{
$this->doXmlString2Xml($string,$xpath);
}
/**
* Adds an additional pear::db_result resultset to $this->xmldoc
*
* @param Object db_result result from a DB-query
* @see doSql2Xml()
* @access public
*/
function addResult($result)
{
$this->doSql2Xml($result);
}
/**
* Adds an aditional resultset generated from an sql-statement
* to $this->xmldoc
*
* @param string sql a string containing an sql-statement.
* @access public
* @see doSql2Xml()
*/
function addSql($sql)
{
/* if there are {} expressions in the sql query, we assume it's an xpath expression to
* be evaluated.
*/
if (preg_match_all ("/\{([^\}]+)\}/i",$sql,$matches))
{
foreach ($matches[1] as $match)
{
$sql = preg_replace("#\{".preg_quote($match)."\}# ", $this->getXpathValue($match),$sql);
}
}
$result = $this->db->query($sql);
//very strange
if (PEAR::isError($result->result)) {
print "You have an SQL-Error:
".$result->result->userinfo;
print "
";
new DB_Error($result->result->code,PEAR_ERROR_DIE);
}
$this->doSql2Xml($result);
}
/**
* Adds an aditional resultset generated from an Array
* to $this->xmldoc
* TODO: more explanation, how arrays are transferred
*
* @param array multidimensional array.
* @access public
* @see doArray2Xml()
*/
function addArray ($array)
{
$parent_row = $this->insertNewResult(&$metadata);
$this->DoArray2Xml($array,$parent_row);
}
/**
* Returns an xml-string with a xml-representation of the resultsets.
*
* The resultset can be directly provided here, or if you need more than one
* in your xml, then you have to provide each of them with add() before you
* call getXML, but the last one can also be provided here.
*
* @param mixed $result result Object from a DB-query
* @return string xml
* @access public
*/
function getXML($result = Null)
{
$xmldoc = $this->getXMLObject($result);
return $xmldoc->dumpmem();
}
/**
* Returns an xml DomDocument Object with a xml-representation of the resultsets.
*
* The resultset can be directly provided here, or if you need more than one
* in your xml, then you have to provide each of them with add() before you
* call getXMLObject, but the last one can also be provided here.
*
* @param mixed $result result Object from a DB-query
* @return Object DomDocument
* @access public
*/
function getXMLObject($result = Null)
{
if ($result) {
$this->add ($result);
}
return $this->xmldoc;
}
/**
* For adding db_result-"trees" to $this->xmldoc
* @param Object db_result
* @access private
* @see addResult(),addSql()
*/
function doSql2Xml($result)
{
if (DB::IsError($result)) {
print "Error in file ".__FILE__." at line ".__LINE__."
\n";
print $result->userinfo."
\n";
new DB_Error($result->code,PEAR_ERROR_DIE);
}
// the method_exists is here, cause tableInfo is only in the cvs at the moment
// BE CAREFUL: if you have fields with the same name in different tables, you will get errors
// later, since DB_FETCHMODE_ASSOC doesn't differentiate that stuff.
$this->LastResult = &$result;
if (!method_exists($result,"tableInfo") || ! ($tableInfo = $result->tableInfo(False)))
{
//emulate tableInfo. this can go away, if every db supports tableInfo
$fetchmode = DB_FETCHMODE_ASSOC;
$res = $result->FetchRow($fetchmode);
$this->nested = False;
$i = 0;
while (list($key, $val) = each($res))
{
$tableInfo[$i]["table"]= $this->tagNameResult;
$tableInfo[$i]["name"] = $key;
$resFirstRow[$i] = $val;
$i++;
}
$res = $resFirstRow;
$FirstFetchDone = True;
$fetchmode = DB_FETCHMODE_ORDERED;
}
else
{
$FirstFetchDone = False;
$fetchmode = DB_FETCHMODE_ORDERED;
}
// initialize db hierarchy...
$parenttable = "root";
$tableInfo["parent_key"]["root"] = 0;
foreach ($tableInfo as $key => $value)
{
if (is_int($key))
{
// if the sql-query had a function the table starts with a # (only in mysql i think....), then give the field the name of the table before...
if (preg_match ("/^#/",$value["table"]) || strlen($value["table"]) == 0) {
$value["table"] = $tableInfo[($key - 1)]["table"] ;
$tableInfo[$key]["table"] = $value["table"];
}
if (!isset($tableInfo["parent_table"]) || is_null($tableInfo["parent_table"][$value]["table"]]))
{
$tableInfo["parent_key"][$value]["table"]] = $key;
$tableInfo["parent_table"][$value]["table"]] = $parenttable;
$parenttable = $value["table"] ;
}
}
//if you need more tableInfo for later use you can write a function addTableInfo..
$this->addTableInfo($key, $value, &$tableInfo);
}
// end initialize
// if user made some own tableInfo data, merge them here.
if ($this->user_tableInfo)
{
$tableInfo = $this->array_merge_clobber($tableInfo,$this->user_tableInfo);
}
$parent['root'] = $this->insertNewResult(&$tableInfo);
//initialize $resold to get rid of warning messages;
$resold[0] = "ThisValueIsImpossibleForTheFirstFieldInTheFirstRow";
while ($FirstFetchDone == True || $res = $result->FetchRow($fetchmode))
{
//FirstFetchDone is only for emulating tableInfo, as long as not all dbs support tableInfo. can go away later
$FirstFetchDone = False;
while (list($key, $val) = each($res))
{
if ($resold[$tableInfo]["parent_key"][$tableInfo][$key]["table"]]] != $res[$tableInfo]["parent_key"][$tableInfo][$key]["table"]]] || !$this->nested)
{
if ($tableInfo["parent_key"][$tableInfo][$key]["table"]] == $key )
{
if ($this->nested || $key == 0)
{
$parent[$tableInfo][$key]["table"]] = $this->insertNewRow($parent[$tableInfo]["parent_table"][$tableInfo][$key]["table"]]], $res, $key, &$tableInfo);
}
else
{
$parent[$tableInfo][$key]["table"]]= $parent[$tableInfo]["parent_table"][$tableInfo][$key]["table"]]];
}
//set all children entries to somethin stupid
foreach($tableInfo["parent_table"] as $pkey => $pvalue)
{
if ($pvalue == $tableInfo[$key]["table"])
{
$resold[$tableInfo]["parent_key"][$pkey]]= "ThisIsJustAPlaceHolder";
}
}
}
if ( $parent[$tableInfo][$key]["table"]] != Null)
{
$this->insertNewElement($parent[$tableInfo][$key]["table"]], $res, $key, &$tableInfo, &$subrow);
}
}
}
$resold = $res;
unset ($subrow);
}
return $this->xmldoc;
}
/**
* For adding whole arrays to $this->xmldoc
*
* @param array
* @param Object domNode
* @access private
* @see addArray()
*/
function DoArray2Xml ($array, $parent) {
while (list($key, $val) = each($array))
{
$tableInfo[$key]["table"]= $this->tagNameResult;
$tableInfo[$key]["name"] = $key;
}
if ($this->user_tableInfo)
{
$tableInfo = $this->array_merge_clobber($tableInfo,$this->user_tableInfo);
}
foreach ($array as $key=>$value)
{
if (is_array($value) ) {
if (is_int($key) )
{
$valuenew = array_slice($value,0,1);
$keynew = array_keys($valuenew);
$keynew = $keynew[0];
}
else
{
$valuenew = $value;
$keynew = $key;
}
$rec2 = $this->insertNewRow($parent, $valuenew, $keynew, &$tableInfo);
$this->DoArray2xml($value,$rec2);
}
else {
$this->insertNewElement($parent, $array, $key, &$tableInfo,&$subrow);
}
}
}
/**
* This method sets the options for the class
* One can only set variables, which are defined at the top of
* of this class.
*
* @param array options to be passed to the class
* @param boolean if the old suboptions should be deleted
* @access public
* @see $nested,$user_options,$user_tableInfo
*/
function setOptions($options,$delete = False) {
//set options
if (is_array($options))
{
foreach ($options as $option => $value)
{
if (isset($this->{$option}))
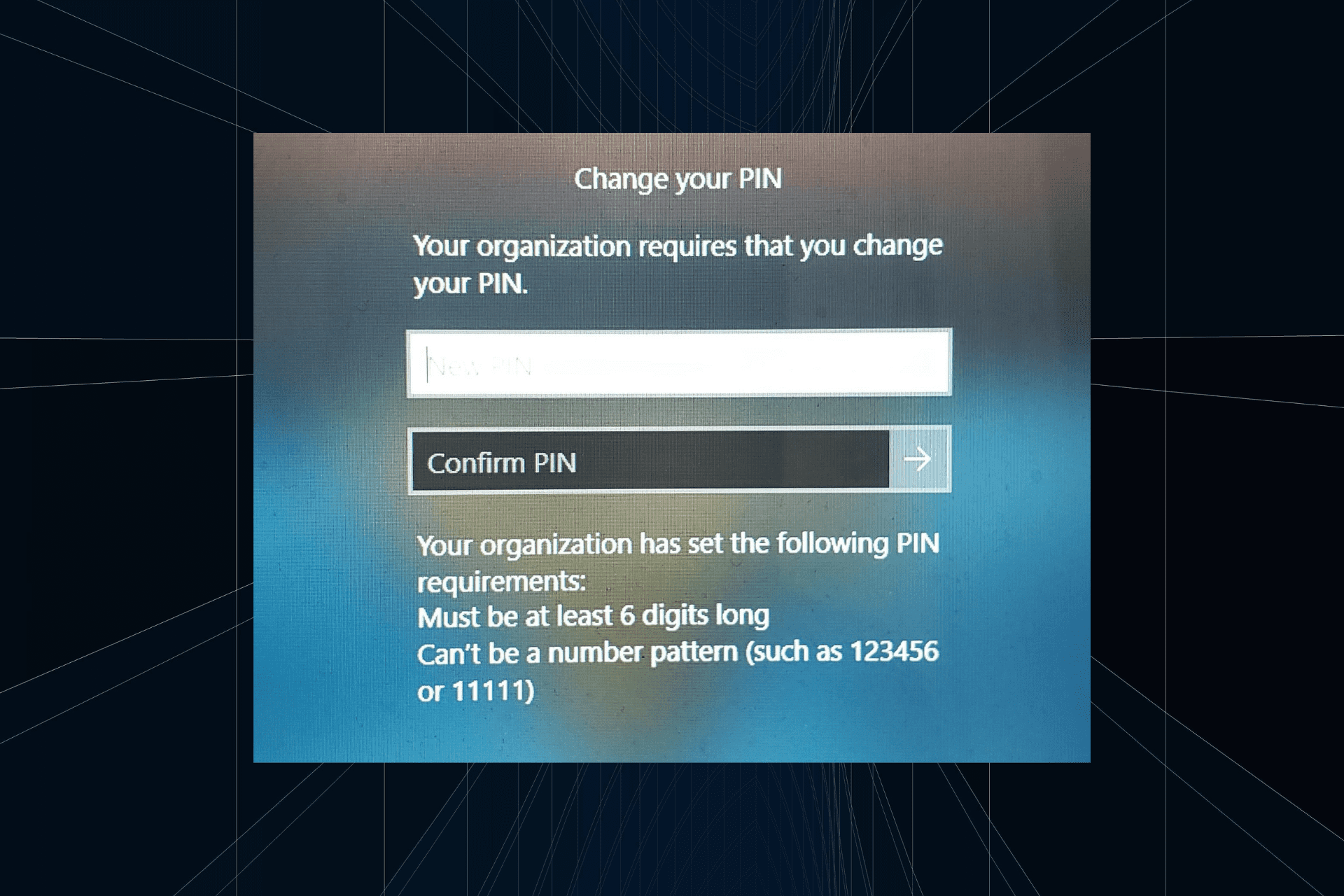
“你的组织要求你更改PIN消息”将显示在登录屏幕上。当在使用基于组织的帐户设置的电脑上达到PIN过期限制时,就会发生这种情况,在该电脑上,他们可以控制个人设备。但是,如果您使用个人帐户设置了Windows,则理想情况下不应显示错误消息。虽然情况并非总是如此。大多数遇到错误的用户使用个人帐户报告。为什么我的组织要求我在Windows11上更改我的PIN?可能是您的帐户与组织相关联,您的主要方法应该是验证这一点。联系域管理员会有所帮助!此外,配置错误的本地策略设置或不正确的注册表项也可能导致错误。即
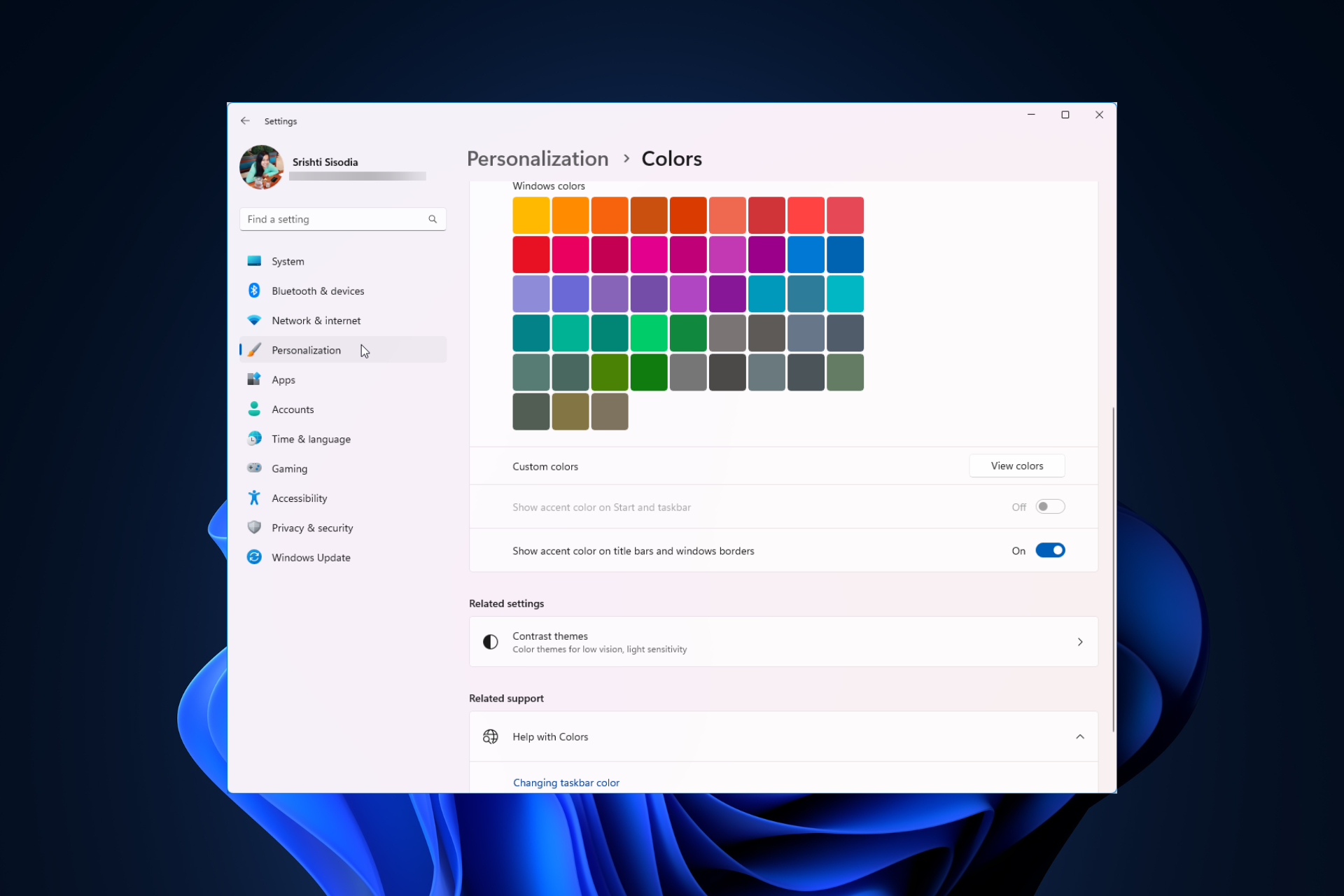
Windows11将清新优雅的设计带到了最前沿;现代界面允许您个性化和更改最精细的细节,例如窗口边框。在本指南中,我们将讨论分步说明,以帮助您在Windows操作系统中创建反映您的风格的环境。如何更改窗口边框设置?按+打开“设置”应用。WindowsI转到个性化,然后单击颜色设置。颜色更改窗口边框设置窗口11“宽度=”643“高度=”500“>找到在标题栏和窗口边框上显示强调色选项,然后切换它旁边的开关。若要在“开始”菜单和任务栏上显示主题色,请打开“在开始”菜单和任务栏上显示主题
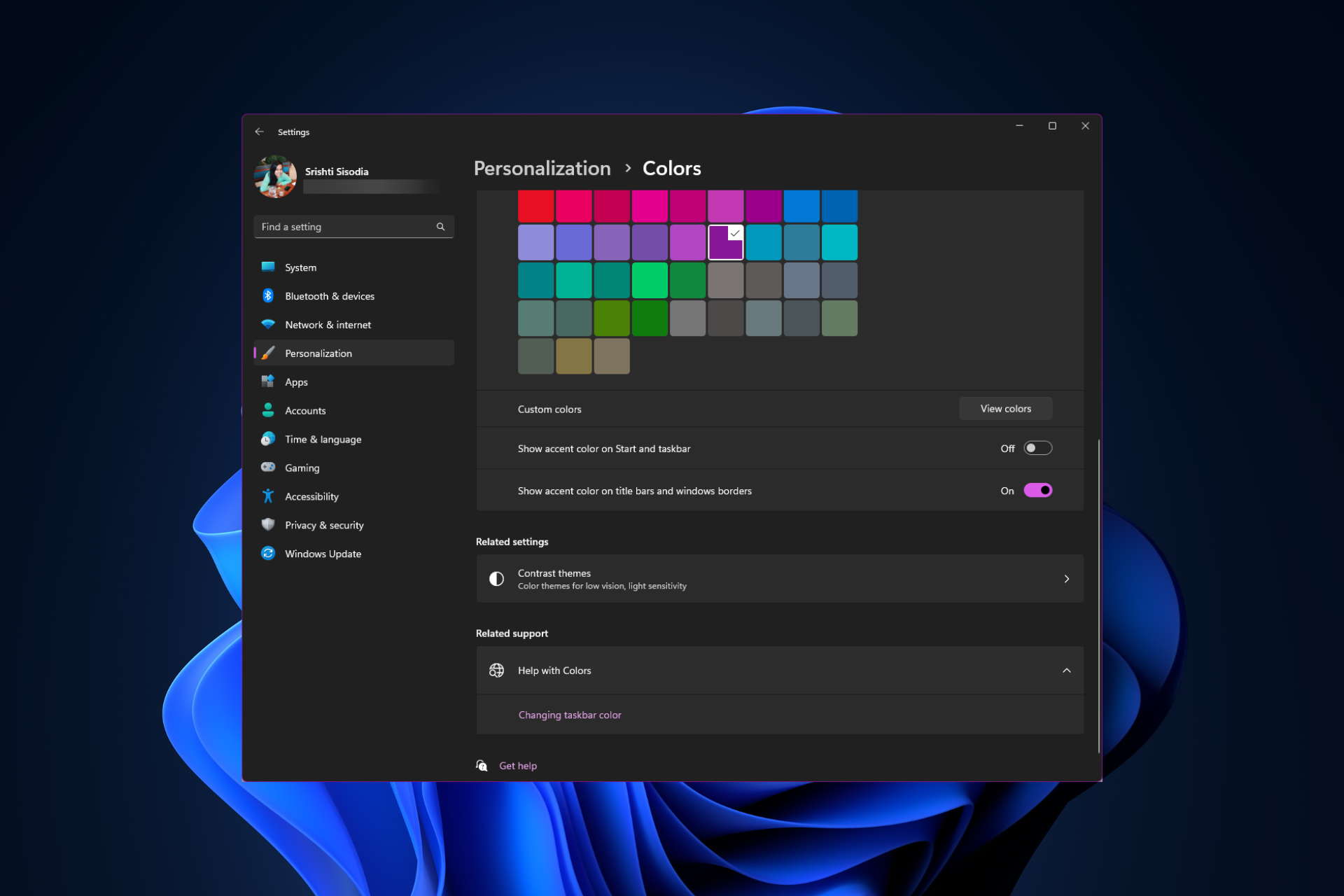
默认情况下,Windows11上的标题栏颜色取决于您选择的深色/浅色主题。但是,您可以将其更改为所需的任何颜色。在本指南中,我们将讨论三种方法的分步说明,以更改它并个性化您的桌面体验,使其具有视觉吸引力。是否可以更改活动和非活动窗口的标题栏颜色?是的,您可以使用“设置”应用更改活动窗口的标题栏颜色,也可以使用注册表编辑器更改非活动窗口的标题栏颜色。若要了解这些步骤,请转到下一部分。如何在Windows11中更改标题栏的颜色?1.使用“设置”应用按+打开设置窗口。WindowsI前往“个性化”,然
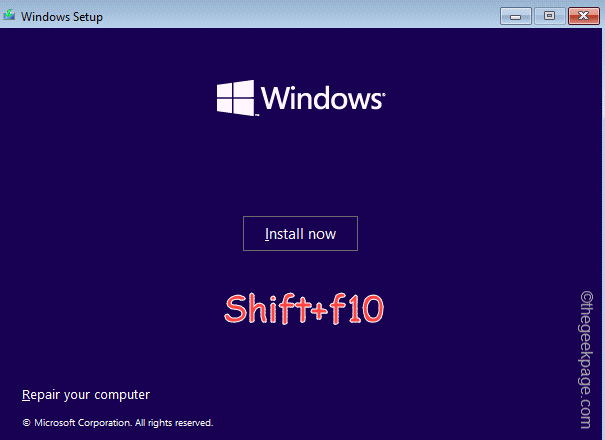
您是否在Windows安装程序页面上看到“出现问题”以及“OOBELANGUAGE”语句?Windows的安装有时会因此类错误而停止。OOBE表示开箱即用的体验。正如错误提示所表示的那样,这是与OOBE语言选择相关的问题。没有什么可担心的,你可以通过OOBE屏幕本身的漂亮注册表编辑来解决这个问题。快速修复–1.单击OOBE应用底部的“重试”按钮。这将继续进行该过程,而不会再打嗝。2.使用电源按钮强制关闭系统。系统重新启动后,OOBE应继续。3.断开系统与互联网的连接。在脱机模式下完成OOBE的所
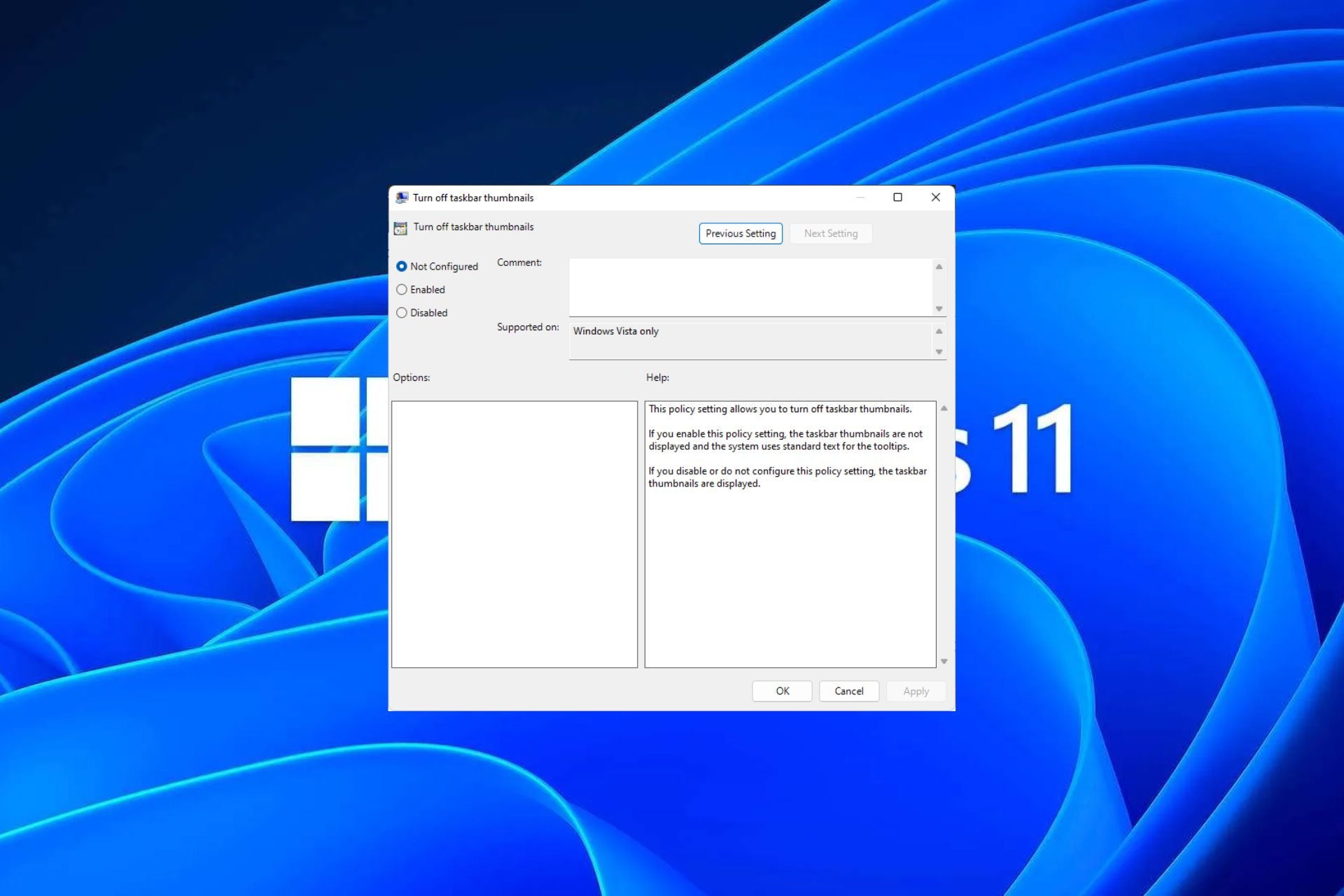
任务栏缩略图可能很有趣,但它们也可能分散注意力或烦人。考虑到您将鼠标悬停在该区域的频率,您可能无意中关闭了重要窗口几次。另一个缺点是它使用更多的系统资源,因此,如果您一直在寻找一种提高资源效率的方法,我们将向您展示如何禁用它。不过,如果您的硬件规格可以处理它并且您喜欢预览版,则可以启用它。如何在Windows11中启用任务栏缩略图预览?1.使用“设置”应用点击键并单击设置。Windows单击系统,然后选择关于。点击高级系统设置。导航到“高级”选项卡,然后选择“性能”下的“设置”。在“视觉效果”选
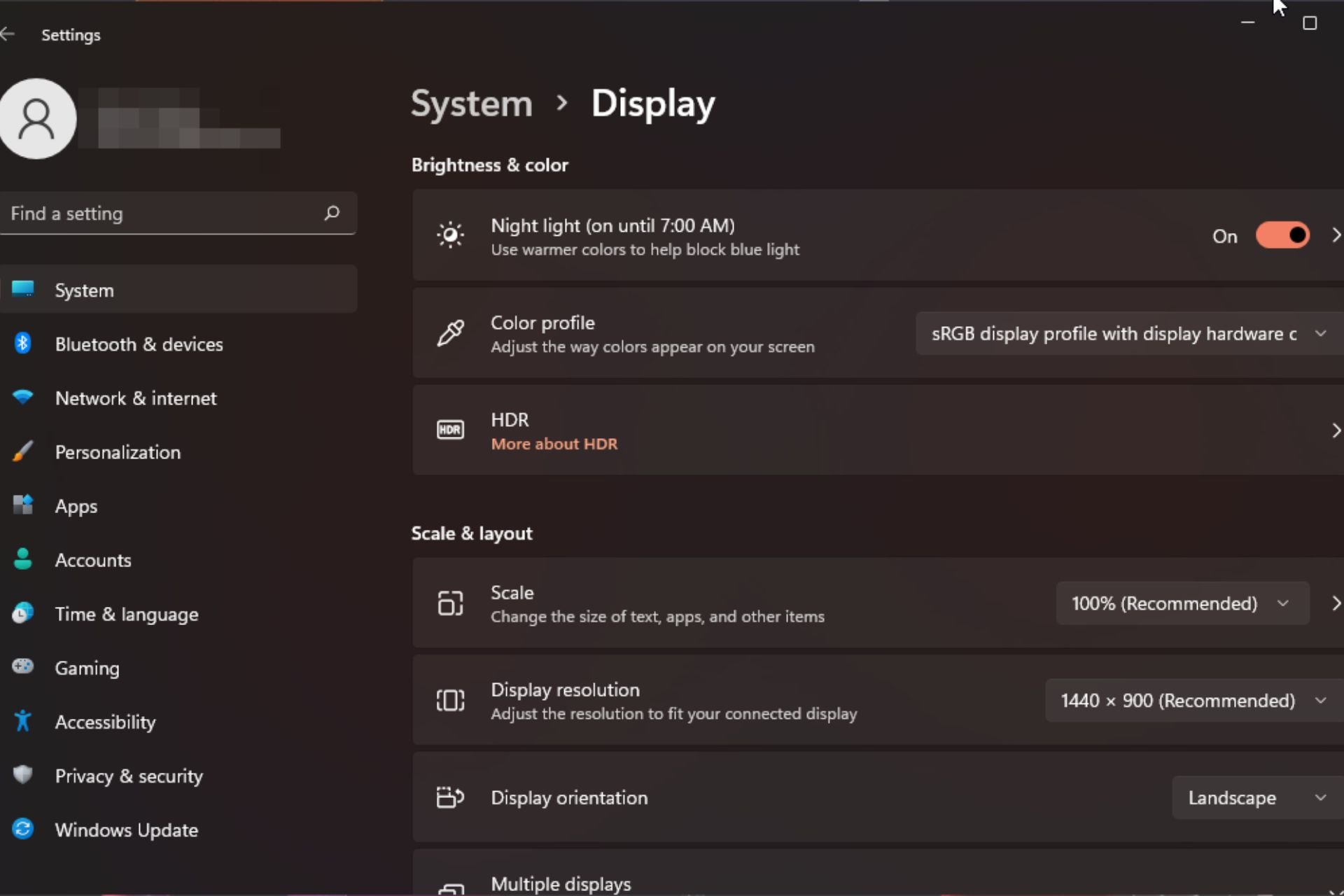
在Windows11上的显示缩放方面,我们都有不同的偏好。有些人喜欢大图标,有些人喜欢小图标。但是,我们都同意拥有正确的缩放比例很重要。字体缩放不良或图像过度缩放可能是工作时真正的生产力杀手,因此您需要知道如何对其进行自定义以充分利用系统功能。自定义缩放的优点:对于难以阅读屏幕上的文本的人来说,这是一个有用的功能。它可以帮助您一次在屏幕上查看更多内容。您可以创建仅适用于某些监视器和应用程序的自定义扩展配置文件。可以帮助提高低端硬件的性能。它使您可以更好地控制屏幕上的内容。如何在Windows11
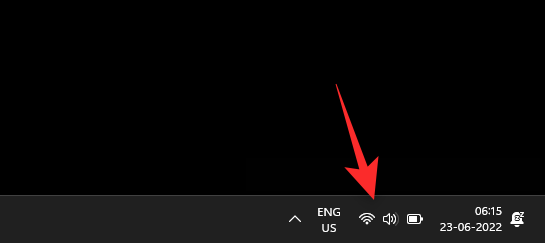
屏幕亮度是使用现代计算设备不可或缺的一部分,尤其是当您长时间注视屏幕时。它可以帮助您减轻眼睛疲劳,提高易读性,并轻松有效地查看内容。但是,根据您的设置,有时很难管理亮度,尤其是在具有新UI更改的Windows11上。如果您在调整亮度时遇到问题,以下是在Windows11上管理亮度的所有方法。如何在Windows11上更改亮度[10种方式解释]单显示器用户可以使用以下方法在Windows11上调整亮度。这包括使用单个显示器的台式机系统以及笔记本电脑。让我们开始吧。方法1:使用操作中心操作中心是访问
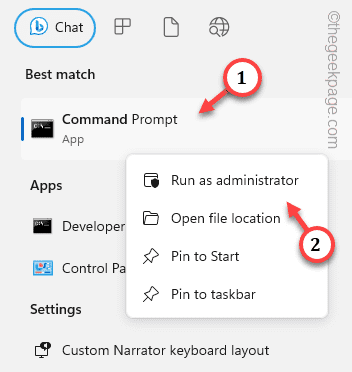
Windows上的激活过程有时会突然转向显示包含此错误代码0xc004f069的错误消息。虽然激活过程已经联机,但一些运行WindowsServer的旧系统可能会遇到此问题。通过这些初步检查,如果这些检查不能帮助您激活系统,请跳转到主要解决方案以解决问题。解决方法–关闭错误消息和激活窗口。然后,重新启动计算机。再次从头开始重试Windows激活过程。修复1–从终端激活从cmd终端激活WindowsServerEdition系统。阶段–1检查Windows服务器版本您必须检查您使用的是哪种类型的W


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
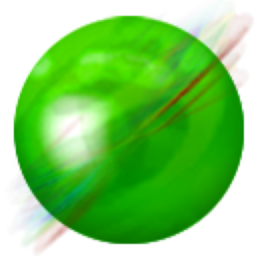
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
