


js code set (friends who are learning js can take a look)_javascript skills
/**
* @author Super Sha
* QQ:770104121
* E-Mail:supei_shafeng@163.com
* publish date: 2009-3-27
* All Rights Reserved
*/
var JsHelper={}; //Declare a JsHelper root namespace
JsHelper.DOM = {}; //Declare the DOM namespace under the JsHelper directory
JsHelper.Event={}; //Declare the Event event namespace in the JsHelper directory
JsHelper.Browser={}; //Declare the browser-related functions in the JsHelper directory Browser namespace
JsHelper. Ajax={}; //Declare the Ajax-related function namespace in the Jshelper directory
JsHelper.String={}; //Declare the String-related namespace in the JsHelper directory
/*
* $() can input multiple parameters and will return an array of objects
*/
var $=function(){ //Simplified version of the $ method
var elements=new Array ();
if(arguments.length==0){ //If the parameter is empty, return the document element
return document;
}
for (var i = 0; i {
var element = arguments[i];
if (typeof element == string )
{
element = document.getElementById(element);
}
if (arguments.length == 1)
{
return element;
}
elements.push(element);
}
return elements;
}
JsHelper.DOM.$=function()
{
var elements=new Array();
for (var i = 0; i {
var element = arguments[i];
if (typeof element == string )
{
element = document.getElementById(element);
}
if (arguments.length == 1)
{
return element;
}
elements.push(element);
}
return elements;
}
/*
* $Value() can input multiple parameters and will return a Value array of obtained objects
*/
JsHelper.DOM.value=function()
{
var values=new Array() ;
for (var i = 0; i {
var element = arguments[i];
if (typeof(element) == string )
{
var v=document.getElementById(element).value;
}
if(arguments.length==1)
{
return v;
}
values .push(v);
}
return values;
}
/*
makeArray generates an array return for the input parameters. If the parameter is empty, it returns "undefined", otherwise it returns Array
*/
JsHelper.String.makeArray=function()
{
var values=new Array();
if(arguments.length>0){
for ( var i = 0; i {
var element=arguments[i];
if(typeof element == "string")
{
values. push(element);
}
}
}
else
{
return "undefined";
}
return values;
}
/*
* Declare a StringBuilder class to handle connection string performance issues
*/
JsHelper.String.StringBulider={
_strs:new Array(),
append: function( str){ //Add string to attribute _strs
this._strs.push(str);
return this;
},
toString:function(){
if (arguments .length != 0) {
return this._strs.join(arguments[0]); //Return the string after combining the attribute _strs, accepting an optional parameter for the join parameter
} else{
return this._strs.join("");
}
}
};
/*
* $TagName() enters a parameter and will Returns an array of Elements TagNeme objects
*/
JsHelper.DOM.tagName=function()
{
var element=arguments[0];
if(typeof element== string )
{
var tagname=document.getElementsByTagName(element);
}
return tagname;
}
//========== ==================================================
/*
* label: HTML Label ID
* Can only be used with responseText
* Can only be used with GET method
*/
var _xmlhttp;//Declare the global XMLHttpRequest object Example
function Ajax(method, url, label){
this.method = method;
this.url = url;
try {
_xmlhttp = new ActiveXObject("Msxml2.XMLHTTP" );
}
catch (e) {
try {
_xmlhttp = new ActiveXObject("Microsoft.XMLHTTP");
}
catch (s) {
_xmlhttp = new XMLHttpRequest();
}
}
Ajax.prototype.ResponseText = function(){
_xmlhttp.onreadystatechange = this.onComplete;
_xmlhttp.open(this.method, this .url, true)
_xmlhttp.send(null);
}
Ajax.prototype.onComplete = function(){
if (_xmlhttp.readyState == 4) {
if ( _xmlhttp.status == 200) {
$(label).innerHTML = _xmlhttp.responseText;
}
}
}
this.ResponseText();
}
//================================================
/*
* Determine browser type
*/
var ua = navigator.userAgent.toLowerCase();
if (window.ActiveXObject) {
JsHelper. Browser.IE = ua.match(/msie ([d.] )/)[1];
}
else if (document.getBoxObjectFor) {
JsHelper.Browser.Firefox = ua.match( /firefox/([d.] )/)[1];
}
else if (window.MessageEvent && !document.getBoxObjectFor) {
JsHelper.Browser.Chrome = ua.match(/chrome /([d.] )/)[1];
}
else if (window.opera) {
JsHelper.Browser.Opera = ua.match(/opera.([d.] ) /)[1];
}
else if (window.openDatabase) {
JsHelper.Browser.Safari = ua.match(/version/([d.] )/)[1];
}
/*
* Declare an instance of XMLHttpRequest object and return the instance
*/
JsHelper.Ajax.createRequest=function()
{
var xmlhttp=null;
try
{
xmlhttp=new XMLHttpRequest();
}
catch(trymicrosoft){
try {
xmlhttp = new ActiveXObject("Msxml2.XMLHTTP");
}
catch(othermicrosoft){
xmlhttp=new ActiveXObject("Microsoft.XMLHTTP");
}
}
return xmlhttp;
}
/*
* A general AddEventListener function, obj is a DOM element
*/
JsHelper.Event.addEventlistener=function(labelID,eventMode,fn)
{
var obj=JsHelper.DOM. $(labelID);
if (typeof window.addEventListener != undefined ) {
obj.addEventListener(eventMode, fn, false);
}
else
if (typeof document.addEventListener != undefined ) {
obj.addEventListener(eventMode, fn, false);
}
else
if (typeof window.attachEvent != undefined ) {
obj.attachEvent("on " eventMode, fn);
}
else {
return false;
}
return true;
}
/*
* contains a Douglas Crockford's extension of function method. The copyright of the following three functions belongs to Douglas Crockford. Hereby declare
*/
Function.prototype.method = function (name, func) {
this.prototype[ name] = func;
return this;
};
Function.method( inherits , function (parent) {
var d = {}, p = (this.prototype = new parent() );
this.method( base , function uber(name) {
if (!(name in d)) {
d[name] = 0;
}
var f, r, t = d[name], v = parent.prototype;
if (t) {
while (t) {
v = v.constructor.prototype;
t -= 1;
}
f = v[name];
} else {
f = p[name];
if (f == this[name]) {
f = v [name];
}
}
d[name] = 1;
r = f.apply(this, Array.prototype.slice.apply(arguments, [1]));
d[name] -= 1;
return r;
});
return this;
});
Function.method( swiss , function (parent) {
for (var i = 1; i var name = arguments[i];
this.prototype[name] = parent.prototype[name];
}
return this;
});
/*
* A solution to the problem that IE does not support HTMLElement
*/
var DOMElement ={
extend : function(name,fn)
{
if(!document.all)
{
eval("HTMLElement.prototype." name " = fn");
}
else
{
var _createElement = document.createElement;
document.createElement = function(tag)
{
var _elem = _createElement(tag);
eval("_elem." name " = fn");
return _elem;
}
var _getElementById = document.getElementById;
document.getElementById = function(id)
{
var _elem = _getElementById( id);
eval("_elem." name " = fn");
return _elem;
}
var _getElementsByTagName = document.getElementsByTagName;
document.getElementsByTagName = function(tag)
{
var _arr = _getElementsByTagName(tag);
for(var _elem=0;_elem<_arr.length>eval("_arr[_elem]." name " = fn" );
return _arr;
}
}
}
};
/*
* The following is modeled after several DOM query functions of John Resig, the father of jQuery Capabilities
*/
DOMElement.extend("previous",function(){ // similar to previousSibling DOM Function
var elem=this;
do{
elem=elem.previousSibling ;
}while(elem&&elem.nodeType!=1);
return elem;
});
DOMElement.extend("next",function(){ //similar to nextSibling DOm Function
var elem=this;
do{
elem=elem.nextSibling;
}while(elem&&elem.nodeType!=1);
return elem;
});
DOMElement.extend("first",function(num){ //similar to firstChild DOM Function, same as parent
var elem=this;
num=num||1;
for (var i = 0; i elem = elem.firstChild;
}
return (elem && elem.nodeType!=1 ? next(elem):elem);
} );
DOMElement.extend("last",function(num){ //similar to lastChild DOM Function, same as parent
var elem=this;
num=num||1;
for (var i = 0; i elem = elem.lastChild;
}
return (elem && elem.nodeType!=1 ? prev(elem):elem);
});
DOMElement.extend("parent",function(num){ //You can return several num levels of parentNode, for example: parent(2) is equivalent to elem.parent().parent();
var elem=this;
num=num ||1;
for (var i = 0; i if (elem != null) {
elem = elem.parentNode;
}
}
return elem;
});
DOMElement.extend("hasChilds",function(){ //Judge when there are child nodes
if(this!=null && this.hasChildNodes()){
return true;
}
else{
return false;
}
});
DOMElement.extend("text",function(){ //Get the text in the label. If the parameter is not zero, you can set the text version in the label. It is also suitable for input labels
try{ //Solution Solution for Firefox not supporting InnerText
HTMLElement.prototype.__defineGetter__("innerText",function(){
var anyString = "";
var childS = this.childNodes;
for(var i =0; i
anyString = childS[i].tagName == "BR" ? "n : childS[ i].innerText;
}
else if(childS[i].nodeType == 3) {
anyString = childS[i].nodeValue;
}
}
return anyString;
});
}
catch(e){}
if (arguments.length == 1) {
if (this.innerText) {
this.innerText = arguments[0];
}
else {
this.value = arguments[0];
}
}
else {
return this.innerText || this.value;
}
});
DOMElement.extend("html",function(){ //Get the innerHTML of the element. If the parameter is not zero, you can set the text and child nodes within the element
if(arguments.length= =0){
return this.innerHTML;
}
else if(arguments.length==1)
{
this.innerHTML=arguments[0];
}
});
/*
* The following are the operations of className
*/
DOMElement.extend("getClassName",function(){ //Return element className
if(this!=null&&this.nodeType==1){
return this.className.replace(/s /, ).split( );
}
return null;
});
DOMElement.extend("hasClassName",function(){ //Determine whether there is a class class
if(this!=null&&this.nodeType==1){
var classes=this.getClassName();
for(var i=0;i
}
}else{
return false;
}
});
DOMElement.extend("addClass",function(){ //Add classes to elements, you can add multiple classes at once
if( this!=null&&this.nodeType==1){
for (var i = 0; i this.className = (this.className ? : ) arguments[i];
}
return this;
}
return null;
});
DOMElement.extend("removeClass",function(){ //Remove the class, if there are no parameters, then Delete all classes
if (this != null && this.nodeType == 1) {
if (arguments.length == 0) {
this.className = "";
}
else if(arguments.length!=0) {
var classes=this.getClassName();
for (var i = 0; i for (var j = 0; j if (arguments[i]==classes[j]) {
classes = classes.join(" ").replace(arguments[i], ).split(" ");
}
}
}
this.className=classes.join(" ");
}
return this;
}
return null;
});
JsHelper.__toggleflag=false; //Add a judgment switch
DOMElement.extend("toggleClass",function(classname){ //Function called by two clicks Different
if(this!=null && this.nodeType==1){
this.onclick=function(){
if(JsHelper.__toggleflag==false){
this.addClass( classname);
JsHelper.__toggleflag = true;
}else if (JsHelper.__toggleflag == true) {
this.removeClass(classname);
JsHelper.__toggleflag = false;
}
}
}
});
/*
* Add a click method to each object, similar to how jQuery's click method is used
*/
DOMElement. extend("click",function(){
if(this!=null && this.nodeType==1){
if(arguments.length==0){
alert("you have done nothing when you clicked.");
}else{
this.onclick=arguments[0];
}
}
});
/*
* Extend the hover method to each object. This method accepts two functions as parameters
*/
DOMElement.extend("hover",function(){
if(this!=null && this. nodeType==1){
if(arguments.length!=2){
alert("Require two function to be param.");
}else{
this.onmouseover=arguments[ 0];
this.onmouseout=arguments[1];
}
}
});
/*
* Function to add events to each element
* /
DOMElement.extend("addEvent",function(eventtype,fn){
if(document.all){
this.attachEvent("on" eventtype,fn);
}else{
this.addEventListener(eventtype,fn,false);
}
});
/*
* Extend the css method for each element, accepting an attribute and attribute value as parameters
*/
DOMElement.extend("css",function(){
if(this!=null && this.nodeType==1){
if(arguments.length!=2){
alert("Require two function to be param.");
}else{
this.style[arguments[0]]=arguments[1]; //Set the value of the relevant style attribute
return this;
}
}
return null;
});
/*
* //Find and return all elements that exist in a certain class, name is className, type is the HTML tag type
*/
var hasClass = function(name,type){
var r = new Array();
//var re = new RegExp(name," g");
var e=document.getElementsByTagName(type||"*");
for(var i=0;i
for (var j = 0; j if (name== classes[j]) {
r.push(e[i] );
}
}
}
return r;
}
/*
* Returns a collection of specific child element references of an element. If there is no element, call For this method, the default is document
*/
DOMElement.extend("find",function(){
var elem=this||document;
var r=new Array();
if(elem!=null && (elem.nodeType==1||elem.nodeType==9)){
var e=elem.getElementsByTagName(arguments[0]);
for(var i =0;i
}
return r;
}
return null;
}) ;
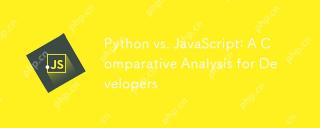
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
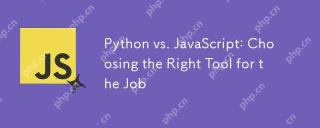
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
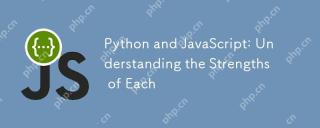
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
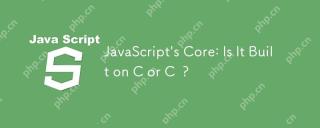
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
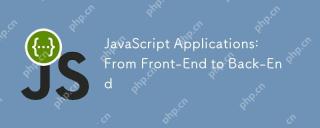
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
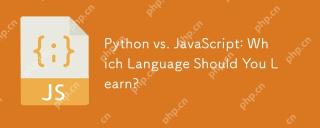
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
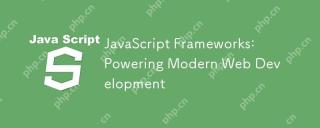
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
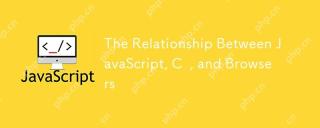
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
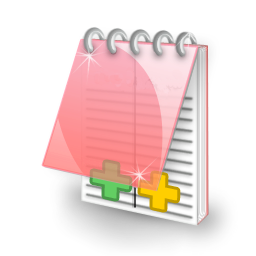
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
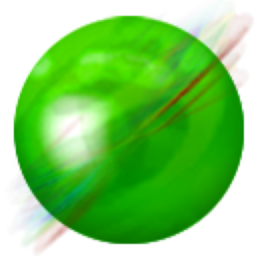
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
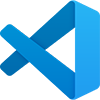
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
