比如说页面的字符处理,js的正则表达式验证等等。下面我就将我自己浅薄的开发经验综合网上的庞杂资源稍稍综合整理一下,省得自己以后要用到时再搜索了。这个系列我会将平时常用的函数归纳整理起来,全当作是抛砖引玉吧。
Code is cheap.看代码:
一、常见的字符串处理函数
// 返回字符的长度,一个中文算2个
String.prototype.ChineseLength = function() {
return this .replace( / [ ^ \x00 - \xff] / g, " ** " ).length;
}
// 去掉字符串两端的空白字符
String.prototype.Trim = function() {
return this .replace( / ( ^ \s + ) | (\s + $) / g, "" );
}
// 去掉字符左端的的空白字符
String.prototype.LeftTrim = function() {
return this .replace( / ( ^ [\s] * ) / g, "" );
}
// 去掉字符右端的空白字符
String.prototype.RightTrim = function() {
return this .replace( / ([\s] * $) / g, "" );
}
/* 忽略大小写比较字符串是否相等
注:不忽略大小写比较用 == 号 */
String.prototype.IgnoreCaseEquals = function(str) {
return this .toLowerCase() == str.toLowerCase();
}
/* 不忽略大小写比较字符串是否相等 */
String.prototype.Equals = function(str) {
return ( this == str);
}
/* 比较字符串,根据结果返回 -1, 0
返回值 相同:0 不相同:-1
*/
String.prototype.CompareTo = function(str) {
if ( this == str) {
return 0 ;
} else
return - 1 ;
}
// 字符串替换
String.prototype.Replace = function(oldValue, newValue) {
var reg = new RegExp(oldValue, " g " );
return this .replace(reg, newValue);
}
// 检查是否以特定的字符串结束
String.prototype.EndsWith = function(str) {
return this .substr( this .length - str.length) == str;
}
// 判断字符串是否以指定的字符串开始
String.prototype.StartsWith = function(str) {
return this .substr( 0 , str.length) == str;
}
// 从左边截取n个字符
String.prototype.LeftSlice = function(n) {
return this .substr( 0 , n);
}
// 从右边截取n个字符
String.prototype.RightSlice = function(n) {
return this .substring( this .length - n);
}
// 统计指定字符出现的次数
String.prototype.Occurs = function(ch) {
// var re = eval("/[^"+ch+"]/g");
// return this.replace(re, "").length;
return this .split(ch).length - 1 ;
}
/* 构造特定样式的字符串,用 包含 */
String.prototype.Style = function(style) {
return " " , this , " " );
}
// 构造类似StringBuilder的函数(连接多个字符串时用到,很方便)
function StringBuilder(str) {
this .tempArr = new Array();
}
StringBuilder.prototype.Append = function(value) {
this .tempArr.push(value);
return this ;
}
StringBuilder.prototype.Clear = function() {
this .tempArr.length = 0 ;
}
StringBuilder.prototype.toString = function() {
return this .tempArr.join( '' );
}
// 字符串常见方法及扩展
function test() {
var testStr = " This is a test string " ;
var testStr2 = " 字符串 " ;
// alert(testStr.Trim());
// alert(testStr.LeftTrim());
// alert(testStr.RightTrim());
// alert(testStr2.ChineseLength());
// alert(testStr2.CompareTo(testStr));
// alert(testStr2.StartsWith("字符串"));
// document.write(testStr2.Style("color:red;width:100px"));
// alert(testStr2.LeftSlice(2));
// alert(testStr.RightSlice(7));
// alert(testStr.Occurs("s"));
/* StringBuilder测试 */
// var testStr3 = new StringBuilder("");
// testStr3.Append("test3\r\n");
// testStr3.Append("test3test3\r\n");
// testStr3.Append("test3");
// alert(testStr3.toString());
// testStr3.Clear();
// alert(testStr3.toString());
}
二、常用的正则表达式
/* -----------------------下面的函数还是涉及到了一些字符串的处理,但是当作正则表达式的一部分看起来更合理----------------------------- */
// 检查字符串是否由数字组成
String.prototype.IsDigit = function () {
var str = this .Trim();
return (str.replace( / \d / g, "" ).length == 0 );
}
// 校验字符串是否为浮点型
String.prototype.IsFloat = function () {
var str = this .Trim();
// 如果为空,则不通过校验
if (str == "" )
return false ;
// 如果是整数,则校验整数的有效性
if (str.indexOf( " . " ) == - 1 ) {
return str.IsDigit();
}
else {
if ( / ^(\-?)(\d+)(.{1})(\d+)$ / g.test(str))
return true ;
else
return false ;
}
}
// 检验是否是负整数
function isNegativeInteger(str) {
// 如果为空,则不通过校验
if (str == "" )
return false ;
if (str.IsDigit()) {
if (parseInt(str, 10 ) < 0 )
return true ;
else
return false ;
}
else
return false ;
}
// 检验是否是负浮点数数
function isNegativeFloat(str) {
// 如果为空,则不通过校验
if (str == "" )
return false ;
if (str.IsFloat()) {
if (parseFloat(str, 10 ) < 0 )
return true ;
else
return false ;
}
else
return false ;
}
// 是否是由字母组成的字符串
function isCharacter(str) {
return ( / ^[A-Za-z]+$ / .test(str));
}
// 是否是字母、数字组成的字符串
function isNumberCharacter(str) {
return ( / ^[A-Za-z0-9]+$ / .test(str));
}
// 是否是email
function isEmail(str) {
return ( / (\S)+[@]{1}(\S)+[.]{1}(\w)+ / .test(str))
}
// 是否是url(评注:网上流传的版本功能很有限,下面这个基本可以满足需求)
function isUrl(str) {
return ( / ([a-zA-z]+:\ / \ / )?[^s]* / .test(str));
}
// 是否是ip地址
function isIpAddress(str) {
return / (\d+)\.(\d+)\.(\d+)\.(\d+) / .test(str);
}
// 是否是汉字组成的字符串
function isChinese(str) {
return ( / ^[\u4e00-\u9fa5]+$ / .test(str));
}
// 是否是双字节字符(包括汉字在内)
function isUnicode(str) {
return ( / ^[\x00-\xff]+$ / .test(str));
}
// 是否是电话号码
function isTelephone(str) {
// 兼容格式: 国家代码(2到3位)-区号(2到3位)(-)?电话号码(7到8位)-分机号(3位)
return ( / ^(([0\+]\d{2,3}-)?(0\d{2,3}))?[-]?(\d{7,8})(-(\d{3,}))?$ / .test(str));
}
// 是否是手机号码
function isMobilePhone(str) {
return ( / ^((\(\d{3}\))|(\d{3}\-))?1[3,5]\d{9}$ / .test(str));
}
// 是否是QQ号码(腾讯QQ号从10000开始)
function isQQNumber(str) {
return ( / ^[1-9][0-9]{4,}$ / .test(str));
}
// 是否是国内的邮政编码(中国邮政编码为6位数字)
function isMailCode(str) {
return ( / \d{6} / .test(str));
}
// 是否是国内的身份证号码
function isIdNumber(str) {
return ( / \d{15}|\d{18} / .test(str));
}
关于正则表达式,网上还有很多的有深度的文章,我这里就拷贝几段常用的代码了,其实学懂了基本的正则知识后普通的验证不过是小菜一碟,不再赘述。
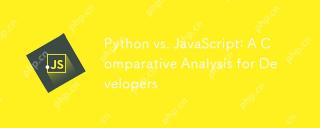
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
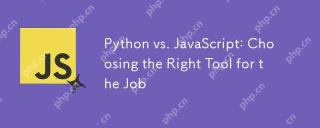
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
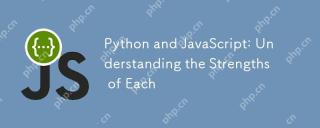
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
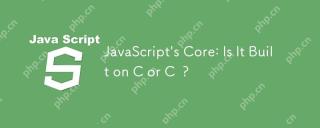
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
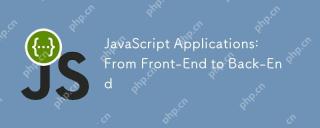
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
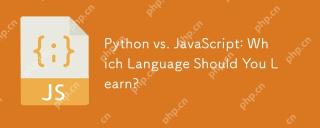
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
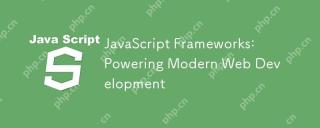
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
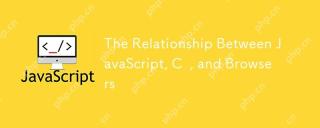
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
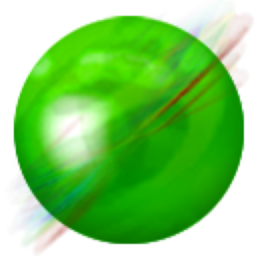
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
