var arr = new Array();
arr['item1 '] = 'the value of item 1 ';
arr['item2'] = 'the value of item 2 ';
alert(arr['item1']);
alert(arr[' item2']);
But the above functions do not meet our actual requirements. In addition, query traversal is inconvenient. We need to expand on the basis of Array.
Below we can use js Array to implement similar hashtable functions,
function Hashtable (){
this.clear = hashtable_clear;
this.containsKey = hashtable_containsKey;
this.containsValue = hashtable_containsValue;
this.get = hashtable_get;
this.isEmpty = hashtable_isEmpty;
this.keys = hashtable_keys;
this.put = hashtable_put;
this.remove = hashtable_remove;
this.size = hashtable_size;
this.toString = hashtable_toString;
this.values = hashtable_values;
this.hashtable = new Array();
}
function hashtable_clear(){
this.hashtable = new Array();
}
function hashtable_containsKey(key) {
var exists = false;
for (var i in this.hashtable) {
if (i == key && this.hashtable[i] != null) {
exists = true;
break;
}
}
return exists;
}
function hashtable_containsValue(value){
var contains = false;
if (value != null) {
for (var i in this.hashtable) {
if (this.hashtable[i] == value) {
contains = true;
break;
}
}
}
return contains;
}
function hashtable_get(key){
return this.hashtable[key];
}
function hashtable_isEmpty(){
return (this.size == 0) ? true : false;
}
function hashtable_keys(){
var keys = new Array();
for (var i in this.hashtable) {
if (this.hashtable[i] != null)
keys.push(i);
}
return keys;
}
function hashtable_put(key, value){
if (key == null || value == null) {
throw 'NullPointerException {' key '},{' value '}';
}else{
this.hashtable[key] = value;
}
}
function hashtable_remove(key){
var rtn = this.hashtable[key];
//this.hashtable[key] =null;
this.hashtable.splice(key,1);
return rtn;
}
function hashtable_size(){
var size = 0;
for (var i in this.hashtable) {
if (this.hashtable[i] != null)
size ;
}
return size;
}
function hashtable_toString(){
var result = '' ;
for (var i in this.hashtable)
{
if (this.hashtable[i] != null)
result = '{' i '},{' this.hashtable[ i] '}n';
}
return result;
}
function hashtable_values(){
var values = new Array();
for (var i in this. hashtable) {
if (this.hashtable[i] != null)
values.push(this.hashtable[i]);
}
return values;
}
How to use Hastable class:
/ /Instantiate a custom hash table class
var hashTable = new Hashtable();
hashTable.put(0,'abc'); //0 is key, 'abc' is value
hashTable.put(1,'123');
hashTable.put(2,'88a');
hashTable.put(3,'88a');
//Traverse hashtable, equivalent to c# and foreach in java
for (var key in hashTable.keys()){ /* Use keys method*/
alert(hashTable.get(key)); //Traverse value by key
}
//Traverse hashtable, equivalent to foreach in c# and java
for (var key in hashTable.hashtable)){ /* Use hashtable attributes*/
alert(hashTable.get(key)); //Traverse value by key
}
alert(hashTable.containsKey(1)); //Return true
alert(hashTable.containsKey(4)); //Because there is no key with key 4, Return false
alert(hashTable.containsValue('888')); //Return true
alert(hashTable.containsValue('mobidogs')); //Because there is no value with value 'mobidogs', return false
hashTable.remove(1); //Remove the element with key 1
alert(hashTable.containsKey(1)); //Because the element with key 1 has been removed by the upstream reomve() method , so return false
//Other methods about hastable are simple to use, readers can test them themselves (this is omitted)
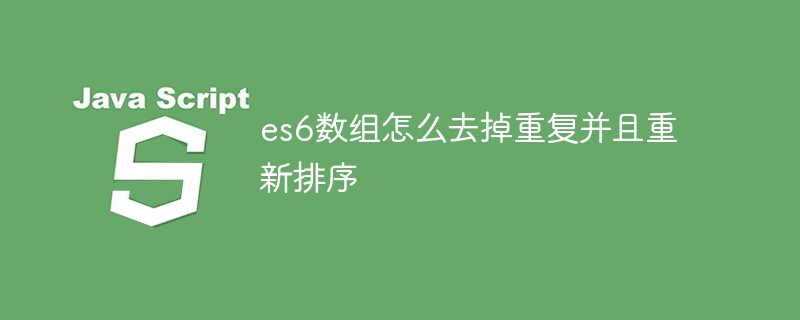
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
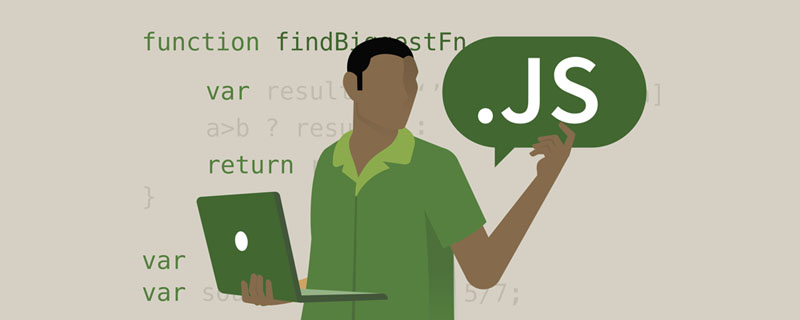
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
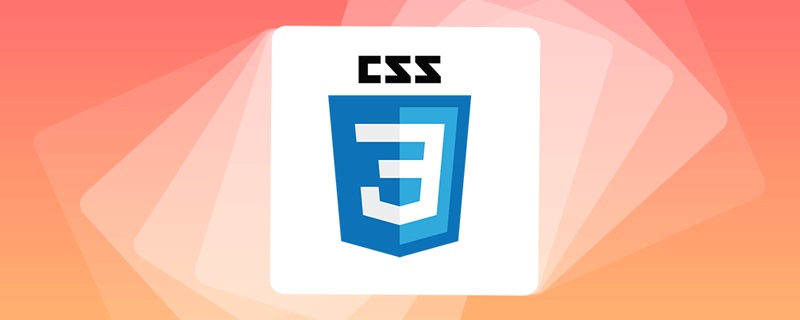
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
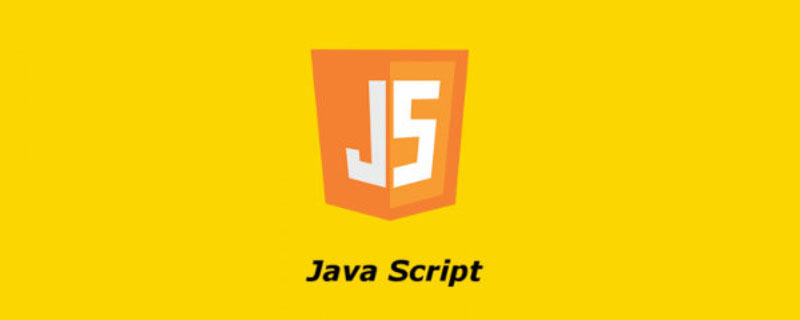
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
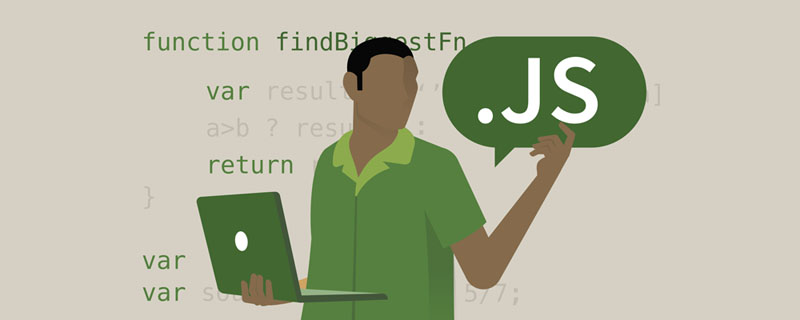
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
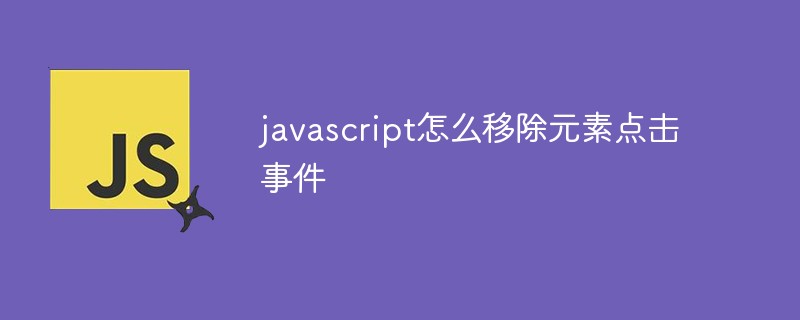
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
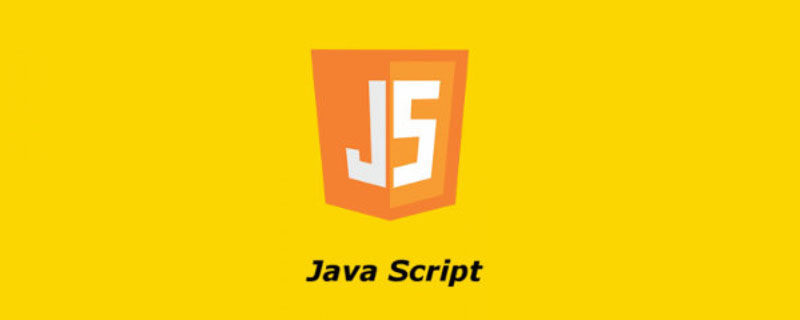
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
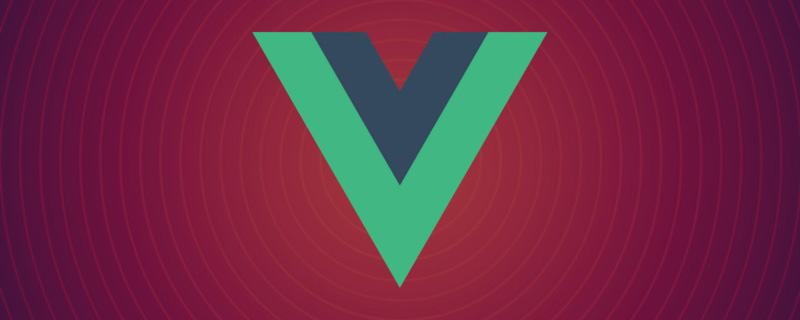
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
