function CCPry(){
//
// Determine the browser type
//
this.Browser ={
"isMozilla":(typeof document.implementation != 'undefined') && (typeof document.implementation.createDocument != 'undefined') && (typeof HTMLDocument!='undefined'),
"isIE":window.ActiveXObject ? true : false,
" isFirefox":navigator.userAgent.toLowerCase().indexOf("firefox")!=-1,
"isOpera":navigator.userAgent.toLowerCase().indexOf("opera")!=-1
};
//
//Return DOM object based on element ID
//
this.$Id=function(id){
return document.getElementById(id);
};
//
//Return the DOM object based on the element ID
//
this.Id=function(id){
var DomObjId=this.$Id(id );
if(!DomObjId){ throw new Error("No Object!");}
//Return or set the innerHTML attribute of the object
this.Html=function(html){
if(!this.IsUndefined(DomObjId.innerHTML))
{
if(!this.IsNull(html)){
DomObjId.innerHTML=html;
}
else{ return DomObjId .innerHTML };
}
else{ throw new Error("Object does not support the property innerHTML!");};
};
//Return or set the innerText property of the object
this.Text=function(text){
if(!this.IsUndefined(DomObjId.innerText))
{
if(!this.IsNull(text)){
DomObjId.innerText= text;
}
else{ return DomObjId.innerText };
}
else{ throw new Error("Object does not support the property innerText!");};
};
//Return or set the value attribute of the object
this.Val=function(val){
if(!this.IsUndefined(DomObjId.value))
{
if(!this. IsNull(val)){
DomObjId.value=val;
}
else{ return DomObjId.value };
}
else{ throw new Error("Object does not support the property value!");};
}
return this;
};
//
//Return the DOM object according to the element Name
//
this .$Name=function(name){
return document.getElementsByName(name);
};
//
//Determine whether the string is empty or null
/ /
this.IsNullOrEmpty=function( str ) {
if(str==null){
return true;
}
else{
if(str.lengthreturn true;
}
}
return false;
};
//
//Determine whether the string is empty
//
this.IsEmpty=function( str ) {
if(str.lengthreturn true;
}
return false;
};
//
//Determine whether the string is null
//
this.IsNull=function( str ) {
if(str==null){
return true;
}
return false;
};
//
//Determine whether it is a function
//
this.IsFunction=function( fn ) {
return typeof(fn)==" function";
};
//
//Judge whether it is an object
//
this.IsObject=function( fn ) {
return typeof(fn) ==="object";
};
//
//Determine whether it is a string
//
this.IsString=function( fn ) {
return typeof(fn )=="string";
};
//
//Determine whether it is a numeric type
//
this.IsNumber=function( fn ) {
return typeof(fn)=="number";
};
//
//Determine whether it is a Boolean type
//
this.IsBoolean=function( fn ) {
return typeof(fn)=="boolean";
};
//
//Determine whether it is undefined
//
this.IsUndefined=function ( fn ) {
return typeof(fn)=="undefined";
};
//
//Determine whether it is a date type
//
this .IsDate=function( fn ) {
return fn.constructor==Date;
};
//
//Return Ajax object
//
this. Ajax=function(s){
//Construct XMLHttpRequest object
GetXmlHttpRequest=function(){
var xmlHttpRequest;
if(window.XMLHttpRequest) { xmlHttpRequest = new XMLHttpRequest(); }
else if(window.ActiveXObject) {
try { xmlHttpRequest = new ActiveXObject("Msxml2.XMLHTTP"); }
catch (e){ xmlHttpRequest = new ActiveXObject("Microsoft.XMLHTTP"); }
}
if (!xmlHttpRequest) {
alert("Failed to create XMLHttpRequest object");
return null;
}
return xmlHttpRequest;
};
//Default Ajax configuration
ajaxSettings={
url: "/ajax/ProcessAjax.ashx",
method: "POST",
timeout: 0,
mimeType: "application/x-www-form-urlencoded",
async: true,
data: null,
datatype: "html",
charset: "utf-8",
accepts : {
xml: "application/xml, text/xml",
html: "text/html",
script: "text/javascript, application/javascript",
json: "application /json, text/javascript",
text: "text/plain",
_default: "*/*"
},
///
/// Call function
///
OnStart:function(){
//start
},
///
/ //Callback function after successful request
///
/// Server returns data
OnSuccess:function(msg ){
//success
},
///
/// Operation exception call function
///
/// Exception information
OnException:function(msg){
//exception
},
///
/// 请求超时后调用函数
///
OnTimeout:function(){
//timeout
},
///
/// 请求完成后调用函数
///
OnComplate:function(){
//complate
}
};
if(this.IsObject(s)){
/*检测传入对象*/
ajaxSettings.url = (!this.IsUndefined(s.url) && this.IsString(s.url)) ? s.url : ajaxSettings.url;
ajaxSettings.method =(!this.IsUndefined(s.method) && this.IsString(s.method)) ? s.method : ajaxSettings.method;
ajaxSettings.timeout = (!this.IsUndefined(s.timeout) && this.IsNumber(s.timeout)) ? s.timeout : ajaxSettings.timeout;
ajaxSettings.mimeType= (!this.IsUndefined(s.mimeType) && this.IsString(s.mimeType)) ? s.mimeType : ajaxSettings.mimeType;
ajaxSettings.async = (!this.IsUndefined(s.async) && this.IsBoolean(s.async)) ? s.async : ajaxSettings.async;
ajaxSettings.data = (!this.IsUndefined(s.data) && this.IsString(s.data)) ? s.data : ajaxSettings.data;
ajaxSettings.datatype = (!this.IsUndefined(s.datatype) && this.IsString(s.datatype)) ? s.datatype: ajaxSettings.datatype;
ajaxSettings.charset =(!this.IsUndefined(s.charset) && this.IsString(s.charset)) ? s.charset: ajaxSettings.charset;
ajaxSettings.OnStart =(!this.IsUndefined(s.OnStart) && this.IsFunction(s.OnStart)) ? s.OnStart : ajaxSettings.OnStart;
ajaxSettings.OnSuccess =(!this.IsUndefined(s.OnSuccess) && this.IsFunction(s.OnSuccess)) ? s.OnSuccess : ajaxSettings.OnSuccess;
ajaxSettings.OnException=(!this.IsUndefined(s.OnException)&&this.IsFunction(s.OnException))?s.OnException:ajaxSettings.OnException;
ajaxSettings.OnTimeout = (!this.IsUndefined(s.OnTimeout) && this.IsFunction(s.OnTimeout)) ? s.OnTimeout : ajaxSettings.OnTimeout;
ajaxSettings.OnComplate=(!this.IsUndefined(s.OnComplate) && this.IsFunction(s.OnComplate)) ? s.OnComplate : ajaxSettings.OnComplate;
//赋值xmlhttp,传入XMLHttpRequest对象
var xmlhttp=GetXmlHttpRequest();
var requestDone=false;
try
{
//根据POST或GET方法判断xmlhttp.send()需要传入什么参数
if (ajaxSettings.data && ajaxSettings.method.toUpperCase() == "GET" ) {
ajaxSettings.url = (ajaxSettings.url.match(/?/) ? "&" : "?") ajaxSettings.data;
ajaxSettings.data = null;
}
xmlhttp.open(ajaxSettings.method,ajaxSettings.url,ajaxSettings.async);
xmlhttp.setRequestHeader("Content-Type", ajaxSettings.mimeType);
xmlhttp.setRequestHeader("X-Requested-With", "XMLHttpRequest"); // 设置标题表明一个XMLHttpRequest的请求
xmlhttp.setRequestHeader("Accept",ajaxSettings.datatype && ajaxSettings.accepts[ ajaxSettings.datatype ] ?ajaxSettings.accepts[ ajaxSettings.datatype ] ", */*" :ajaxSettings.accepts._default);
if(ajaxSettings.timeout>0){
var timer=setTimeout(function(){requestDone=true;xmlhttp.abort();},ajaxSettings.timeout);
}
var xmlreadystatechange=function ()
{
if(requestDone){ ajaxSettings.OnTimeout(); } //timeout
else if(xmlhttp.readyState==4) //success
{
if (timer) { clearTimeout(timer); timer = null;}
if (xmlhttp.status==200 || xmlhttp.status=="success")
{
switch(ajaxSettings.datatype.toLowerCase())
{
case "html":
ajaxSettings.OnSuccess(xmlhttp.responseText);
break;
case "xml":
ajaxSettings.OnSuccess(xmlhttp.responseXML);
break;
}
}
ajaxSettings.OnComplate(); //complate
if (ajaxSettings.async&&xmlhttp){ xmlhttp=null; }
}
else{ajaxSettings.OnStart();} //start
}
xmlhttp.onreadystatechange=xmlreadystatechange;
xmlhttp.send(ajaxSettings.data);
if (!ajaxSettings.async){ xmlreadystatechange();xmlhttp=null; }
}
catch(e){
ajaxSettings.OnException(e.message); //exception
}
}
return this;
}
//
// 拆分JSON对象并以特定符号连接组成字符串
//
this.SplitJson=function(jsonObj,splitSign){
var jsonStr='',signLastIndex;
for (jo in jsonObj)
{
jsonStr = (jo "=" jsonObj[jo] splitSign) ;
}
signLastIndex=jsonStr.lastIndexOf(splitSign);
if(signLastIndex!=-1){
jsonStr=jsonStr.substring(0,signLastIndex);
}
return jsonStr;
}
//
// JS图片缩略
//
this.DrawImage=function(ImgD,iwidth,iheight){
var flag=false;
var image=new Image();
image.src=ImgD.src;
if(image.width>0 && image.height>0)
{
flag=true;
if(image.width/image.height>= iwidth/iheight)
{
if(image.width>iwidth)
{
ImgD.width=iwidth;
ImgD.height=(image.height*iwidth)/image.width;
}
else
{
ImgD.width=image.width;
ImgD.height=image.height;
}
}
else
{
if(image.height>iheight)
{
ImgD.height=iheight;
ImgD.width=(image.width*iheight)/image.height;
}
else
{
ImgD.width=image.width;
ImgD.height=image.height;
}
}
}
}
//
// Intercept the string and include Chinese processing
//
this.SubString=function(str, len, hasDot){
var newLength = 0;
var newStr = "";
var chineseRegex = /[^x00-xff]/g;
var singleChar = "";
var strLength = str.replace (chineseRegex,"**").length;
for(var i = 0;i {
singleChar = str.charAt(i).toString();
if(singleChar.match(chineseRegex) != null)
{
newLength = 2;
}
else
{
newLength ;
}
if( newLength > len)
{
break;
}
newStr = singleChar;
}
if(hasDot && strLength > len)
{
newStr = "";
}
return newStr;
}
//
// Replace all
//
this.ReplaceAll=function(Str,oldString,newString){
return Str.replace(new RegExp(oldString,"gm"),newString);
},
//
// Get URL parameters, if invalid, return undfined
//
this.RequestQueryString=function(url){
var qIndex=url.indexOf('?');
var queryObj={};
if(qIndex!=-1){
var queryStr=url.substring(qIndex 1,url.length);
if(queryStr.indexOf('&')!=-1){
var arrQuery = new Array();
arrQuery=queryStr .split('&');
for(arrStr in arrQuery){
paramKey=arrQuery[arrStr].substring(0,arrQuery[arrStr].indexOf("=")).toLowerCase();
paramValue=arrQuery[arrStr].substring(arrQuery[arrStr].indexOf("=") 1,arrQuery[arrStr].length);
queryObj[paramKey]=paramValue
}
}
else{
paramKey=queryStr.substring(0,queryStr.indexOf("=")).toLowerCase();
paramValue=queryStr.substring(queryStr.indexOf("=") 1,queryStr. length);
queryObj[paramKey]=paramValue;
}
}
return queryObj;
}
}
window.$CC=new CCPry();
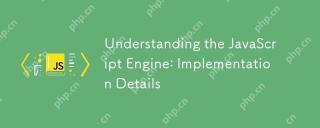
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
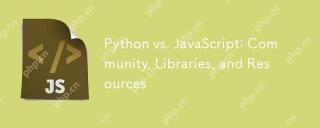
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
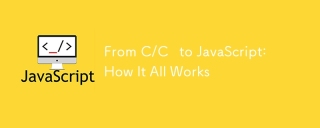
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
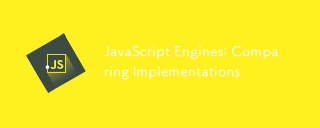
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
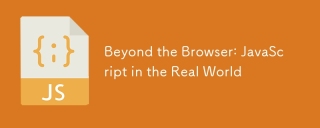
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
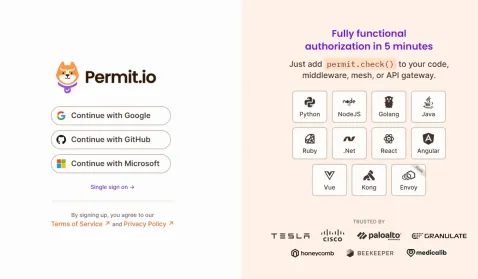
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
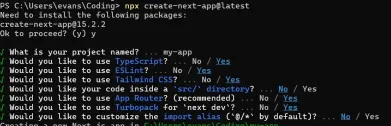
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
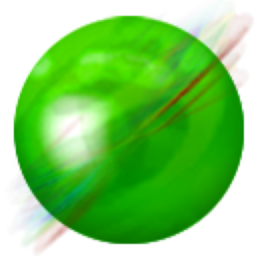
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
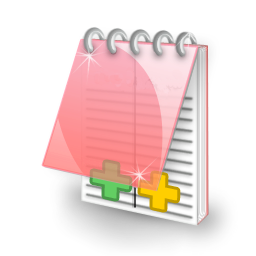
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
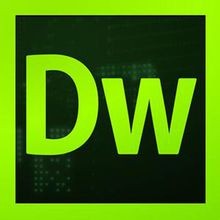
Dreamweaver CS6
Visual web development tools