When we want to read some information about Event, we are often buried in a large number of attributes, most of which do not run well in most browsers. Here is the event compatibility list.
I am not going to make a list of these attributes, because those situations are too confusing, and it will not be helpful to your learning at all. Before writing the 5 pieces of code, I want to ask 5 questions about the browser.
1. What is the type of event?
2. Which HTML element is the target of the event?
3. Which keys were pressed when the event occurred?
4. Which mouse button was pressed when the Event occurred?
5. Where is the mouse position when the Event occurs?
I have given a very detailed answer to the last question here.
Please note that I have done very strict object checking on these codes. I first created cross-browser access to the event, and then checked for browser support before using each attribute.
1. What is the type of event?
This is a cross-browser question with a standard answer: Use the type attribute to view its properties:
function doSomething(e) {
if (!e) var e = window.event;
alert(e.type);
}
2. Which HTML element is the target of the event?
W3C/Netscape said: target. No, Microsoft said, it's srcElement. Both properties return the HTML element when the event occurred.
function doSomething(e) {
var targ;
if (!e) var e = window.event;
if (e.target) targ = e.target;
else if (e.srcElement) targ = e.srcElement;
if (targ.nodeType == 3) // defeat Safari bug
targ = targ.parentNode;
}
The last two lines of code are specifically for Safari. If an event occurs on an element that contains text, the text node becomes the target of the event rather than the element itself. So we want to check if the target's nodetype is 3 (text node). If it is, we move it to the parent node, the HTML element.
Even if the event is captured or bubbles up (bubbles up), the target/srcElement attribute is still the element where the event occurred earliest.
Other targets
There are many targeting attributes. I discussed currentTarget in the article Event Order and relatedTarget, fromElement and toElement in the article Mouse event.
3. Which keys were pressed when the event occurred?
This question is relatively simple. First get the code of the key (a=65) from the keyCode attribute. After you get the key value, you can know the actual key value through the String.fromCharCode() method, if necessary.
function doSomething(e) {
var code;
if (!e) var e = window.event;
if (e.keyCode) code = e.keyCode;
else if (e.which) code = e.which;
var character = String.fromCharCode(code);
alert('Character was ' character);
}
There are some places here that may make keyboard events difficult to use. For example, the kepress event fires as long as the user presses the key. However, the triggering time of keydown in most browsers is as long as the pressing time. I'm not sure if that's a good idea, but that's what it is.
4. Which mouse button was pressed when the Event occurred?
There are two properties here to know which mouse button was pressed: which and button. Please note that these properties generally do not necessarily work on click. To safely detect which mouse button was pressed, you'd better use the mousedown and mouseup events.
which is an old Netscape property. The value of the left mouse button is 1, the value of the middle button (scroll wheel) is 2, and the value of the right mouse button is 3. There are no problems except for the weak support. In fact, it is often used to detect mouse buttons.
Button attributes are now well recognized. W3C's standard values are as follows:
Left button 0
Middle button 1
Right button 2
Microsoft's standard values are as follows:
Left button 1
Middle button 4
Right button 2
There is no doubt that Microsoft’s standards are better than W3C’s. 0 can mean that no key is pressed, and everything else is unreasonable.
In addition, only in the Microsoft model, the values of the buttons can be combined. For example, 5 means that the "left button and middle button" are pressed together. Not only does IE6 not support merging, but the w3c model is theoretically impossible: you never know whether the left button was pressed.
So in my opinion w3c has made a serious mistake in defining buttons.
Right Click
Fortunately, usually you want to know if the right button was clicked. Because W3C and Microsoft happen to define the button value as 2 on this issue, you can still detect right clicks.
function doSomething(e) {
var rightclick;
if (!e) var e = window.event;
if (e.which) rightclick = (e.which == 3);
else if (e.button) rightclick = (e. button == 2);
alert('Rightclick: ' rightclick); // true or false
}
It should be noted that Macs usually only have one button, Mozilla defines the value of the Ctrl-Click button as 2, so Ctrl-Click will also open the menu. ICab does not yet support mouse button attributes, so you cannot detect right clicks in Opera.
5. Where is the mouse position when the Event occurs?
The problem of mouse position is quite serious. Although there are no less than 6 properties for mouse coordinates, there is still no reliable cross-browser method to find the mouse coordinates.
The following are these 6 sets of coordinates:
1. clientX, clientY
2. layerX, layerY
3. offsetX, offsetY
4. pageX, pageY
5. screenX, screenY
6. x, y
I once explained the issues of pageX/Y and clientX/Y here.
screenX and screenY are the only pair of properties that are cross-browser compatible. They give the coordinates of the mouse across the entire computer screen. Unfortunately, this information alone is not enough: you never need to know where the mouse is on the screen - well, maybe you want to place a new window at the current mouse position.
The other three pairs of attributes are not important, see the description here.
Correct code
The following code can correctly detect the coordinates of the mouse
function doSomething(e) {
var posx = 0;
var posy = 0;
if (!e) var e = window.event;
if (e .pageX || e.pageY) {
posx = e.pageX;
posy = e.pageY;
}
else if (e.clientX || e.clientY) {
posx = e.clientX document.body.scrollLeft
document.documentElement.scrollLeft;
posy = e.clientY document.body.scrollTop
document.documentElement.scrollTop;
}
// posx and posy contain the mouse position relative to the document
// Do something with this information
}
Original text here: http://www.quirksmode.org /js/events_properties.html
Please give me some advice on my twitter: @rehawk
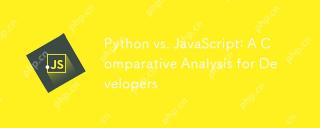
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
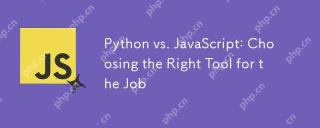
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
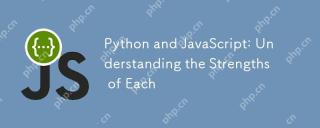
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
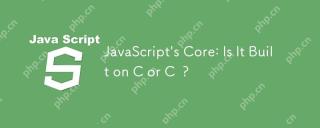
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
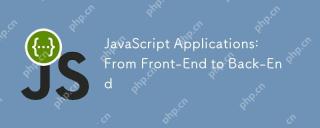
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
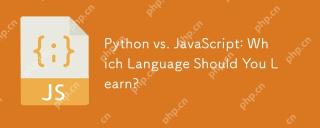
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
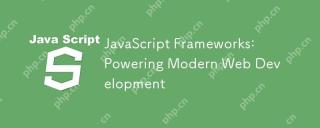
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
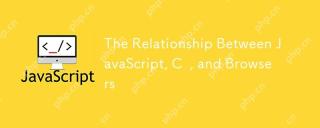
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
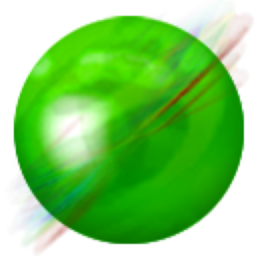
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
