


Detailed explanation of arguments, callee, caller in javascript_Basic knowledge
What are arguments?
arguments is an array-like object created when a function is called but is not an array object. It stores the parameters actually passed to the function and is not limited to the parameter list declared by the function.
Nima, what do you mean?
Write a demo and see the code below
<!DOCTYPE html> <head> <title>arguments</title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> </head> <body> <script> function obj(){ //利用instanceof判断arguments console.log( 'arguments instanceof Array? ' + (arguments instanceof Array) ); console.log( 'arguments instanceof Object? ' + (arguments instanceof Object) ); console.log(arguments); } obj(); </script> </body> </html>
Run this code and use the chrome debugger to get the following picture
I use instanceof to determine arguments. From the printing effect, arguments are an object.
Then expand the printed arguments. As you can see from the picture above, it includes many attributes, including callee.
Next, we modify the above code and pass parameters to it when calling the obj function, but the obj function has no parameters.
See the specific code below
<!DOCTYPE html> <head> <title>arguments</title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> </head> <body> <script> function obj(){ console.log( 'arguments instanceof Array? ' + (arguments instanceof Array) ); console.log( 'arguments instanceof Object? ' + (arguments instanceof Object) ); console.log(arguments); } //向obj传递参数 obj('monkey','love',24); </script> </body> </html>
Through the chrome debugger, you can get the picture below
As you can see, arguments contains the three parameters "monkey", "love", and 24 that we passed to it.
So, why arguments are the stored parameters actually passed to the function, rather than the parameters declared by the function.
What is a callee?
callee is a member of the arguments object, and its value is "the Function object being executed".
What does it mean?
Let’s write a demo and see the output.
See the code and result diagram below
<!DOCTYPE html> <head> <title>callee</title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> </head> <body> <script> function obj(){ //利用callee console.log(arguments.callee); } obj(); </script> </body> </html>
As you can see from the picture above, arguments.callee is a function pointing to the arguments object, which is obj in this case.
What is caller?
caller is an attribute of the function object, which stores the function that calls the current function.
Note, it is a call. It doesn’t just include closures. If there is no parent function, this is null.
It’s still the same as before, let’s write a demo all the time.
The code is as follows:
<!DOCTYPE html> <head> <title>caller</title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8"/> </head> <body> <script> //child是parent内的函数,并在parent内执行child function parent(){ function child(){ //这里child的父函数就是parent console.log( child.caller ); } child(); } //parent1没有被别人调用 function parent1(){ //这里parent1没有父函数 console.log(parent1.caller); } //parent2调用了child2 function parent2(){ child2(); } function child2(){ console.log(child2.caller); } /*执行 parent里嵌套了child函数 parent1没有嵌套函数 parent2调用了child2,child2不是嵌套在parent2里的函数 */ parent(); parent1(); parent2(); </script> </body> </html>
Open the chrome debugger and you can see the renderings
Based on the code and the above picture, do you understand the caller now?
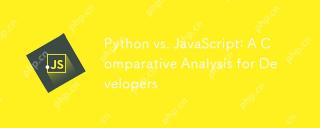
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
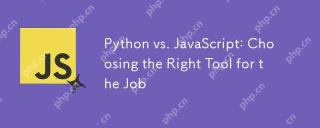
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
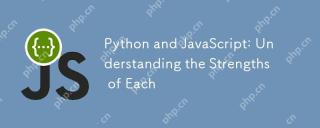
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
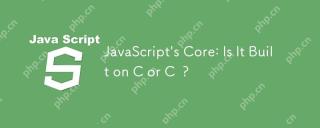
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
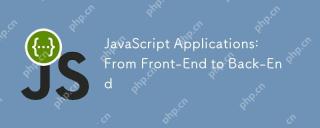
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
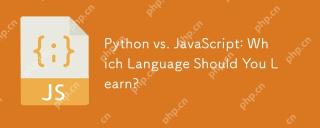
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
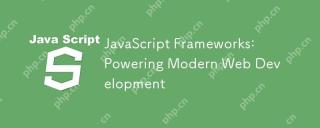
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
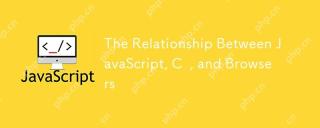
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
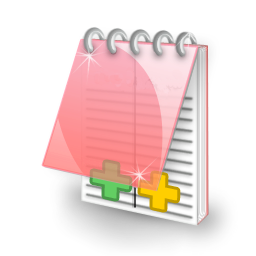
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
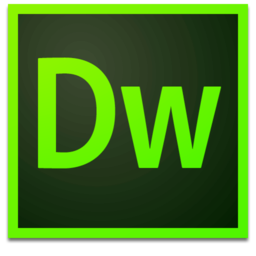
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
