The operation of events is nothing more than the three event methods addEvent, fireEvent, and removeEvent. Generally, lib will make some extensions to the functions provided by the browser to solve problems such as compatibility memory leaks. The third question is how to get the status of domReady.
6.1 Event package
Browser event compatibility is a headache. IE's event is under the global window, while Mozilla's event is that the event source parameters are passed into the callback function. There are many other event handling methods as well.
Jquery provides an event package, which is a bit simpler than what other libs provide, but it is enough to use.
//Wrap the event.
Fix: function(event) {
if (event[expando] == true) return event;//Indicates that the event has been wrapped
//Save the original event and clone one.
var originalEvent = event; ①
event = { originalEvent : originalEvent};
for (var i = this.props.length, prop;i;) {
-i];
event[prop] = originalEvent[prop];
}
event[expando] = true; event.preventDefault = function() { ②
// Run on the original event
if (originalEvent.preventDefault)
originalEvent.preventDefault();
originalEvent.returnValue = false;
} ;
Event.stopPropagation = function() {
🎜> };
// Correct timeStamp
event.timeStamp = event.timeStamp || now();
// Correct target
if (!event.target) ③
event. target = event.srcElement || document;
if (event.target.nodeType == 3)//The text node is the parent node.
Event.target = event.target.parentNode;
// relatedTarget
if (!event.relatedTarget && event.fromElement) event.relatedTarget = event.fromElement == event.target
? event.toElement : event.fromElement;
// Calculate pageX/Y if missing and clientX/Y available
if (event.pageX == null && event.clientX != null) { ⑥
var doc = document.documentElement, body = document.body;
event.pageX = event.clientX
(doc && doc.scrollLeft || body && body.scrollLeft || 0)
- (doc .clientLeft || 0);
event.pageY = event.clientY
(doc && doc.scrollTop || body && body.scrollTop || 0)
- (doc.clientTop || 0);
}
// Add which for key events
if (!event.which && ((event.charCode || event.charCode === 0) ⑦
? event.charCode : event.keyCode))
event.which = event.charCode || event.keyCode;
// Add metaKey to non-Mac browsers
if (!event.metaKey && event.ctrlKey ) ⑧
event.metaKey = event.ctrlKey;
// Add which for click: 1 == left; 2 == middle; 3 == right
// Note: button is not normalized, so don't use it
if (!event.which && event.button) ⑨
event.which = (event.button & 1 ? 1 : (event.button & 2
? 3 : (event .button & 4 ? 2 : 0)));
return event;
},
The above code retains the reference of the original event at ① and clones the original event. Wrap on this clone event. ② Run the preventDefault and stopPropagation methods on the original event to determine whether to prevent the default event action from occurring and whether to stop the bubbling event from being passed upward.
③The target is corrected. srcElement is used in IE. At the same time, for text node events, the target should be passed to its parent node.
④ relatedTarget is only useful for mouseout and mouseover. In IE, it is divided into two Target variables, to and from, but in Mozilla, they are not separated. In order to ensure compatibility, relatedTarget is used to unify them.
⑥ is the coordinate position of the event. This is relative to page. If the page can be scrolled, scroll must be added to its client. In IE, the default 2px body border should also be subtracted.
The ⑦ point is to unify the keys of the keyboard event to the attribute of event.which. The implementation in Ext is ev.charCode || ev.keyCode || 0; ⑨ is to unify the mouse event keys on event.which. One of charCode and ev.keyCode is a character key, and the other is not a character key. ⑨ Use & method to handle compatibility. Ext resolves compatibility issues with the following three lines.
var btnMap = Ext.isIE ? {1:0,4:1,2:2} : (Ext.isSafari ? {1:0,2:1,3:2} : {0:0 ,1:1,2:2}); this.button = e.button ? btnMap[e.button] : (e.which ? e.which-1 : -1);
①②③④⑤⑥⑦⑧⑨⑩
6.2 Event processing
Jquery provides some methods for register, remove, and fire events.
6.2.1 Register
For registering events, jquery provides four methods of registering events: bind, one, toggle, and hover. Bind is the most basic method. One is a method that is registered to run only once, and toggle is a method that is registered to run alternately. Hover is a method of registering mouse hovering.
bind : function(type, data, fn) {
return type == "unload" ? this.one(type, data, fn) : this
.each(function() {// fn || data, fn && data realizes that the data parameter is optional
jQuery.event.add(this, type, fn || data, fn && data);
}); },
Bind for unload Events can only be run once, and other events use the default registration method.
// Bind a one-time event handler function to a specific event (like click) of each matching element.
//On each object, this event handler will only be executed once. Other rules are the same as bind() function.
// This event handler will receive an event object, which can be used to prevent the default behavior (browser).
// If you want to cancel the default behavior and prevent the event from bubbling, this event handling function must return false.
one : function(type, data, fn) {
var one = jQuery.event.proxy(fn || data, function(event) {
jQuery(this).unbind(event, one);
return (fn || data).apply(this , arguments);/this->The current element
});
return this.each(function() {
jQuery.event.add(this, type, one, fn && data);
});
},
One and bind are basically the same. The difference is that when calling jQuery.event.add, the registered event processing function is slightly adjusted. One called jQuery.event.proxy to proxy the incoming event processing function. When an event triggers a call to this agent's function, the event is first deleted from the cache, and then the registered event function is executed. Here is the application of closure, through which the reference of the event function registered by fn is obtained.
//A method to simulate hover events (mouse moves over an object and moves out of the object).
//This is a custom method that provides a "keep in" state for frequently used tasks.
//When the mouse moves over a matching element, the specified first function will be triggered. When the mouse moves out of this element,
/ will trigger the specified second function. Moreover, it will be accompanied by detection of whether the mouse is still in a specific element (for example, an image in a div),
// If so, it will continue to remain in the "hover" state without triggering the move-out event (Fixed a common error using mouseout events).
Hover: function(fnOver, fnOut) {
return this.bind('mouseenter', fnOver).bind('mouseleave', fnOut);
},
Hover is based on bind.
//Calling functions in sequence after each click.
toggle: function(fn) {
var args = arguments, i = 1;
while (i jQuery.event.proxy( fn, args[i ]);//The modified one is still in args
return this.click(jQuery.event.proxy(fn, function(event) {//Assign GUID this.lastToggle = (this.lastToggle || 0) % i; // Previous function event.preventDefault(); // Prevent default action
// Execute the function in the parameters, apply can use array-like parameters
return args[this.lastToggle].apply(this,arguments)||false;
}));
},
The parameters in Toggle can be multiple fn. First code them to generate UUIDs. Then call the click method to register the callback of the agent again. This function is run when the event is triggered. It first calculates the last time the function in the parameter was executed. Then prevent the default action. Then find the next function to run.
//Add commonly used event methods to jquery objects
jQuery.each(
("blur, focus, load, resize, scroll, unload, click, dblclick,"
"mousedown ,mouseup,mousemove,mouseover,mouseout,change,select,"
"submit,keydown,keypress,keyup,error").split(","),
function(i, name) {jQuery.fn [name] = function(fn) {
return fn? this.bind(name, fn) : this.trigger(name);
};});
Jquery adds a common The event handling method includes the click called above. It can be seen here that bind is still called to register. Of course, events can also be triggered through programs.
Many of the above methods are to register events, which ultimately fall into jQuery.event.add(); to complete the registration function. If we use the event method of Dom0 or DOM1, we will use elem.onclick=function(){} to register a handler function for a certain event of the element. The biggest disadvantage of this is that each event is just a processing function. There is an improvement in the dom1 method. We can use elem.addEventListener(type, handle, false) to register multiple handler functions for element events.
This method of processing is not perfect. It would be a bit troublesome if we only run this event once. We need to perform elem.removeEventListener at the end of the event processing function to cancel the event listening. Doing so may cause business problems. What if the first event handling function is triggered again before canceling the event listening?
There is also the browser method, which does not support the registration and processing of custom events, and cannot register the same handler function for multiple events.
jQuery.event = {//Add event to an element.
add : function(elem, types, handler, data) {
if (elem.nodeType == 3 || elem.nodeType == 8) return; // Blank node or comment
// IE It cannot be passed into window, so copy it first.
if (jQuery.browser.msie && elem.setInterval) elem = window;
// Assign a globally unique Id to the handler
if (!handler.guid) handler.guid = this.guid;
// Attach data to handler.data
if (data != undefined) { ①
var fn = handler;
handler =this.proxy(fn,function(){return fn .apply(this,arguments);});
handler.data = data;
}
// Initialize the events of the element. If the value in events is not obtained, initialize data: {} ②
var events =jQuery.data(elem,"events")||jQuery.data(elem,"events",{}),
// If the value in handle is not obtained, initialize data: function() {....} ③
handle = jQuery.data(elem, "handle")|| jQuery.data(elem, "handle" ,
function() {//Handle the second event of a trigger and call an event after the page has been unloaded.
if (typeof jQuery != "undefined"&& !jQuery.event.triggered)
Return jQuery.event.handle.apply(//callee.elem=handle.elem
arguments.callee.elem, arguments);
});
// Add elem as handle attribute, Prevent memory leaks in IE due to no local Event.
handle.elem = elem;
// Use spaces to separate multiple event names, such as jQuery(...).bind("mouseover mouseout", fn);
jQuery.each(types. split(/s /), function(index, type) { ④
// Namespace events are generally not used.
var parts = type.split(".");type = parts[. 0];handler.type = parts[1];
// All handlers bound to the type event of this element
var handlers = events[type]; ⑤
if (!handlers) {// Initialize the event queue if the handler list is not found
handlers = events[type] = {};
// If type is not ready, or the setup execution of ready returns false ⑥
if (!jQuery.event. special[type]|| jQuery.event.special[type].setup
.call(elem, data) === false) {// Call the system event function to register the event
if(elem.addEventListener )elem.addEventListener(type,handle,false);
else if (elem.attachEvent)elem.attachEvent("on" type, handle);
}
}
// Put the handler The form of the id and handler form attribute pair is stored in the handlers list,
// also exists in events[type][handler.guid]
handlers[handler.guid] = handler; ⑦
/ / Globally cache the usage identifier of this event
jQuery.event.global[type] = true;
});
elem = null; // Prevent IE memory leaks.
},
guid: 1,
global: {},
jQuery.event.add uses jQuery.data to organically and orderly combine the event names and processing functions related to the event and store them in the space corresponding to the element in jQuery.cache. Let's analyze the add process with an example: If we receive the following jQuery(e1).bind("mouseover mouseout", fn0);jQuery(e1).bind("mouseover mouseout", fn1) statement.
When jQuery(e1).bind("mouseover mouseout", fn0);, ②③ cannot get the number from the cache, so initialize it first. The cache at this time: {e1_uuid:{events:{},handle:fn}}. Then in ⑤ the mouseover mouseout name will be initialized. The cache at this time: {e1_uuid:{events:{ mouseover:{}, mouseout:{}},handle:fn}}. Register the handler function to the browser event at ⑥. Then ⑦ will add the handler function to the event name. The cache at this time: {e1_uuid:{events:{mouseover:{fn0_uuid:fn0},mouseout:{ fn0_uuid:fn0}},handle:fn}}. Here you can see the role of using proxy to generate uuid for the function.
When jQuery(e1).bind("mouseover mouseout", fn1), ②③ both get data from cache {e1_uuid:{events:{mouseover:{fn0_uuid:fn0},mouseout:{ fn0_uuid: fn0}}, and then get the references of mouseover:{fn0_uuid:fn0}, mouseout:{ fn0_uuid:fn0} in ⑤. Then ⑦ will register the handler function into the event name. The cache at this time: {e1_uuid:{events:{mouseover:{fn0_uuid:fn0, fn1_uuid:fn1,},mouseout:{ fn0_uuid:fn0, fn1_uuid:fn1}},handle:fn}}.
A very important task of jQuery.event.add is to store the registered event functions in an orderly manner. So that the functions of remove and fire events can be found.
//{elem_uuid_1:{events:{mouseover:{fn_uuid:fn1,fn_uuid1:fn2},
//mouseout:{fn_uuid:fn1,fn_uuid1:fn2}},handle:fn}}
6.2.2 trigger
Registered events, such as onclick. Then when the user clicks on this element, the registered event handler of this event will be automatically triggered. But sometimes when we want to use a program to simulate the triggering of an event, we have to use forced triggering of an event. In IE we can use .fireEvent() to achieve this. For example:
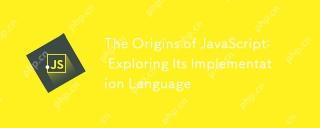
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
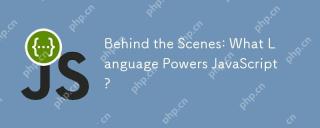
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
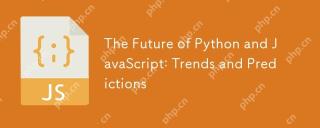
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
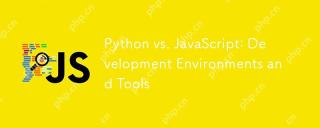
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
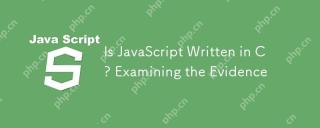
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
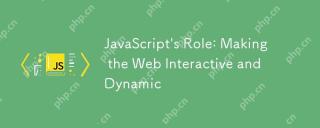
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
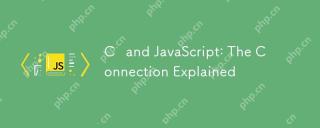
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
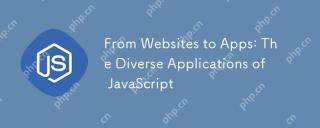
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
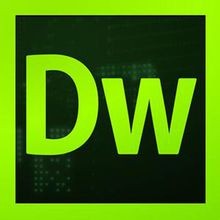
Dreamweaver CS6
Visual web development tools
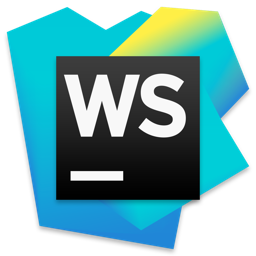
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
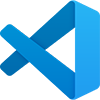
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
