DLL ActiveX control WEB page call example_javascript skills
Due to the needs of the project, I started to learn and research VC, DLL and ActiveX controls. I found a lot of information online, but none was available or there was no example that could be understood and run. No way, just do your own research. Hard work pays off, and you will eventually achieve success. Haha, now I have summarized my own learning and dedicated it to those who need it.
DLL (dynamic link library): divided into WIN32 DLL and MFC DLL
ActiveX: divided into ATL control and MFC control (also a DLL)
WEB: JAVASCRIPT call-> ActiveX call-> DLL Completes the addition operation and returns the value, which is displayed on the page.
2. Development (VS2008)
1. DLL library writing:
File -> New -> WIN32 console -> Fill in the project name -> Select DLL -> Empty project -"Finish.
(1) In the solution panel, add a header file testdll.h, content:
extern "C"
{
DECLDIR int Add( int a, int b );
DECLDIR void Function( void );
}
#endif
(2) In the solution panel, add an implementation file testdll.cpp, content:
Copy the code
The code is as follows :
return( a b );
}
DECLDIR void Function( void )
{
std::cout < ;}
}
(3) Optional. Create a new WIN32 console class and test this DLL.
File -> New -> WIN32 Console -> Fill in the project name -> Select the console program -> Empty project -> Complete.
In the solution panel, add an implementation file loaddll.cpp content:
The code is as follows:
FunctionFunc _FunctionFunc;
cout // L means to use the UNICODE character set, which must be consistent with the character set of the project.
HINSTANCE hInstLibrary = LoadLibrary(L"E:\Project\VS\LoadDll\Release\TestDll.dll");
if (hInstLibrary == NULL)
{
cout FreeLibrary(hInstLibrary);
}else{
cout }
_AddFunc = (AddFunc)GetProcAddress(hInstLibrary, "Add");
_FunctionFunc = (FunctionFunc)GetProcAddress(hInstLibrary, "Function");
if ((_AddFunc == NULL) || (_FunctionFunc == NULL))
{
FreeLibrary(hInstLibrary);//Release
}else{
cout }
cout _FunctionFunc(); //
cin.get (); // Get focus so that the program will not flash by.
FreeLibrary(hInstLibrary);//After calling, release the memory.
return(1);
}
2. ActiveX control implementation:
Here we choose ATL control implementation instead of MFC ActiveX.
File -> New -> ATL Project -> Fill in the project name ("FROMYANTAI") -> Select the dynamic link library (DLL) -> Complete.
After completion, many header H files and CPP implementation files will be generated in the "Solution Explorer" on the right. These are the default and do not need to be modified.
(1) Add an ALT simple object: Click the project name (the name just given) with the mouse and select - "Add class -" select ATL simple object.
Next step, give a name: "ytiicrj" -> Next step: Leave everything else unchanged, in support, select "Connection Point" and "IE Object Support" -> Complete.
The next step is to add a method to "ytiicrj" so that it can be called by the WEB page. Select "iytiicrj" in "Class View" (there is a gray key icon), right-click and add -> Add method. Name the method "GetContent" -"Select IN for parameter attributes, select LONG for parameter type, parameter name A -"Add; continue; select IN for parameter attributes, select LONG for parameter type, parameter name B -"Add; continue; select OUT and parameter attributes RETVAL, select LONG* for parameter type and parameter name out –》Add---》Click to complete.
This adds one (in the last line) to the ytiicrj.H header file:
STDMETHOD(GetContent)(LONG a, LONG b, LONG* out);
And in the ytiicrj.CPP file Added an implementation class:
STDMETHODIMP CCaluNumCtrl::GetContent( LONG a, LONG b, LONG* out)
{
// TODO: Add implementation code here
return S_OK;
}
(2), in In the ytiicrj.H file, call the DLL class library. The code is as follows:
// CaluNumCtrl.h: Statement of ytiicrj The bold part is the specific implementation, and the others are untouched.
#pragma once
#include "resource.h " // Main symbol
#include
#include "AtlActiveX_i.h"
#include "_ICaluNumCtrlEvents_CP.h"
#if defined(_WIN32_WCE) && ! defined(_CE_DCOM) && !defined(_CE_ALLOW_SINGLE_THREADED_OBJECTS_IN_MTA)
#error "Single-threaded COM objects are not properly supported on Windows CE platforms (such as Windows Mobile platforms that do not provide full DCOM support). Define _CE_ALLOW_SINGLE_THREADED_OBJECTS_IN_MTA to force ATL to support the creation of single-threaded objects. COM object implementation and allows the use of its single-threaded COM object implementation. The threading model in the rgs file has been set to "Free" because this model is the only threading model supported by non-DCOM Windows CE platforms. "
#endif
// ytiicrj
class ATL_NO_VTABLE Cytiicrj:
//Add a line: Security prompt is removed, -- when running the browser call, no security question will be prompted.
public IObjectSafetyImpl
public IConnectionPointContainerImpl
public CProxy_ICaluNumCtrlEvents
public IObjectWithSiteImpl
public IDispatchImpl
{
public:
//The following three lines of implementation definition.
typedef int (*AddFunc)(int,int); //Type definition, corresponding to the DLL ADD method. Func is customized and can be written as you wish.
HINSTANCE hInstLibrary;
AddFunc _AddFunc; //Class mapping
Cytiicrj()
{
//Start calling the DLL for calculation.
hInstLibrary = LoadLibrary(L"TestDll.dll");//Place the written DLL file in the directory generated by this project
if (hInstLibrary == NULL)
{
FreeLibrary( hInstLibrary);//Resource release
}else{
}
//Call the method and return the method handle.
_AddFunc = (AddFunc)GetProcAddress(hInstLibrary, "Add");
}
DECLARE_REGISTRY_RESOURCEID(IDR_CALUNUMCTRL)
BEGIN_COM_MAP(Cytiicrj)
COM_INTERFACE_ENTRY(ICaluNumCtrl)
COM_INTERFACE_ENTRY(IDispatch)
COM_INTERFACE_ENTRY(IConnectionPointContainer)
COM_INTERFACE_ENTRY(IObjectWithSite)
//Add a line: Disable the security prompt, -- when running the browser call, no security questions will be prompted.
COM_INTERFACE_ENTRY(IObjectSafety)
END_COM_MAP()
BEGIN_CONNECTION_POINT_MAP(Cytiicrj)
CONNECTION_POINT_ENTRY(__uuidof(_ICaluNumCtrlEvents))
END_CONNECTION_POINT_MAP()
DECLARE_PROTECT_FINAL_CONSTRUCT()
HRESULT FinalConstruct()
{
return S_OK;
}
void FinalRelease()
{
FreeLibrary(hInstLibrary);
}
public:
STDMETHOD(GetContent)(LONG a, LONG b, LONG* out);
};
OBJECT_ENTRY_AUTO(__uuidof(CaluNumCtrl), Cytiicrj)
(3) Go back to the ytiicrj.PP file and add the implementation code as follows:
STDMETHODIMP CCaluNumCtrl::GetContent(LONG a, LONG b, LONG* out)
{
// TODO: Add implementation code here
int sum = this-> ;_AddFunc(static_cast
*out = static_cast
this->_AtlFinalRelease();
return S_OK ;
}
(4) Generate DLL:
This step is very simple, select Release mode, click on the project to generate (it will prompt you to select REG32 registration, then select it). This generates a lot of files in the Release directory, and what we want is a DLL file.
3. DLL and ATL ActiveX control DLL are packaged as CAB files:
For example: after generating test.CAB, the WEB page will prompt for download and installation.
(1) First define the setup.inf file: it describes the downloaded content and target directory as well as the version number and corresponding DLL file. This needs to be written manually. My content is as follows (please modify the name accordingly):
[version]
; version signature (same for both NT and Win95) do not remove
signature="$CHICAGO$"
AdvancedINF=2.0
[Add.Code]
AtlActiveX.dll=AtlActiveX.dll
TestDll.dll=TestDll.dll
setup.inf=setup.inf
[install.files]
AtlActiveX.dll=AtlActiveX.dll
TestDll.dll=TestDll.dll
setup.inf=setup.inf
[AtlActiveX.dll]
clsid={4AE870B5-C7FB-4171-A47E-7F57AFD86F67}
file-win32-x86 =thiscab
FileVersion=1,0,0,1
DestDir=11
RegisterServer=yes
[TestDll.dll]
file-win32-x86=thiscab
DestDir=11
FileVersion=1,0,0,1
RegisterServer=yes
[setup.inf]
file=thiscab
[RegisterFiles]
%AtlActiveX.dll
; end of INF file
(2) Integrate resources:
Put all the DLLs used in one directory including the setup.inf file, and then run: IExpress command to generate the CAB package .
After running, select the first, next, select the third, next, add files (select your DLL and INF files), next, select an output directory and create a CAB file name, then select Second option, next step, select the second option and then OK. This generates a CAB file.
(3) The WEB page calls the ActiveX control to perform addition operations:
Write a test.htm web page and put the CAB file in a directory. The content of test.htm is as follows:
Description: codeBase="test .CAB#version=9,0,0,1" codeBase represents the relative or absolute path of the file; version represents the version number. If this number is the same as the version number of the INF file, then the page will not be downloaded the second time, otherwise every time downloaded every time. CLSID is the serial number generated by the ActiveX project, which can be found in the *.rgs file of the project.
Okay. All steps are completed. Now you run test.htm, the ActiveX control is prompted, you choose to allow it, and then you can call the addition operation.
This is just a simple example. In the DLL, you can implement your own application.
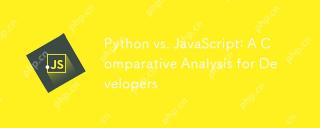
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
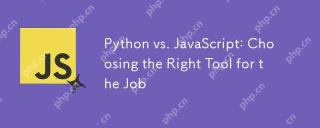
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
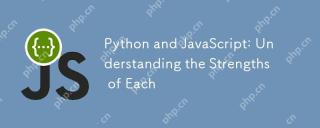
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
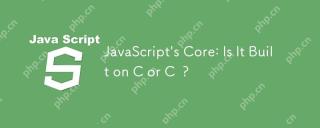
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
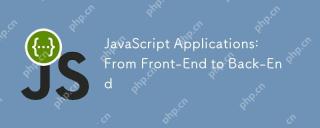
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
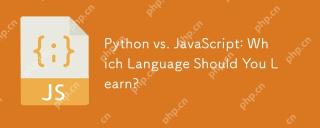
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
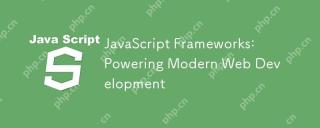
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
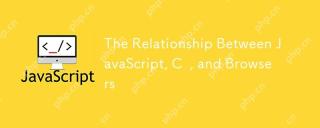
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
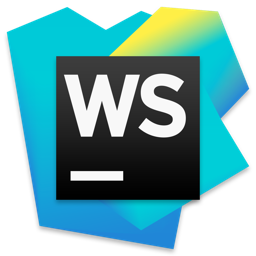
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
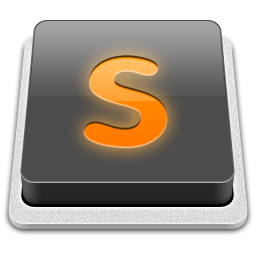
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
