Summary of basic knowledge of javascript Array object_javascript skills
My summary of ideas for Array objects is: 5 methods, 3 moves, 12 formulas
1. Declaring the 5th method: only for one-dimensional arrays, of course, there are also two-dimensional and three-dimensional arrays, so I won’t explain it here.
var a = new Array();
var a = new Array;
var a = new Array(10);//Create an Array object and specify the number of items in the array
var a = new Array("red","blue ","green");
var a = ["red"," blue"," green"];
2. Attribute 3 tricks: constructor, length, prototype
constructor represents a function that creates an object. The constructor property is a member of all objects that have a prototype. They include all JScript native objects except Global and Math objects. The constructor property holds a reference to the function that constructs a specific object instance. For example:
x = new String("Hi");
if (x.constructor == String)
// Process (condition is true).
or
function MyFunc {
// Function body.
}
y = new MyFunc;
if (y.constructor == MyFunc)
// Process (condition is true).
Length represents the length of the array and how many items there are. I won’t write an example here.
prototype returns a reference to the prototype of the object type. Use the prototype property to provide a basic set of functionality for an object's class. New instances of an object "inherit" the operations assigned to the object's prototype.
For example, add a method to an Array object that returns the value of the largest element in the array. To accomplish this, declare the function, add it to Array.prototype, and use it.
function array_max( ){
var i, max = this[0];
for (i = 1; i {
if(max max = this[i] ;
}
return max;
}
Array.prototype.max = array_max;
var x = new Array(1, 2, 3, 4, 5, 6);
var y = x.max( );
After this code is executed, y saves the maximum value in the array x, or 6.
3. Method 12: concat method | join method | pop method | push method | reverse method | shift method | unshift method | slice method | splice method | sort method | toString method | valueOf method
//1.concat(): Returns a new array, which is a combination of two or more arrays.
var a1 = [1,2,3,4];
var a2 = a1.concat("5",'6');
alert(a2); //Result: 1,2 ,3,4,5,6
//2.join(): Returns a string value that contains all elements of the arrays connected together, with the elements separated by the specified delimiter.
var a1 = [1,2,3,4];
var a2 = a1.join();
var a3 = a1.join(",");
var a4 = a1 .join("|");
alert(a2); //Result: 1,2,3,4
alert(a3); //Result: 1,2,3,4
alert (a4); //Result: 1|2|3|4
//3.pop(): Remove the last element in the array and return the element. If the array is empty, undefined will be returned.
var a1 = [1,2,3,4];
var item = a1.pop();
alert(item); //Result: 4
alert(a1);/ /Result: 1,2,3
//4.push(): Add (append) new elements to an array and return the new length value of the array. The push method will add new elements in the order they appear. If one of the arguments is an array, the array is added to the array as a single element. If you want to combine elements from two or more arrays, use the concat method.
var a1 = [1,2,3,4];
a1.push(5);
a1.push("6");
alert(a1);//Result: 1,2,3,4,5,6
//5.reverse(): Returns an Array object with the order of elements reversed.
var a1 = [1,2,3,4];
a1.reverse();
alert(a1);//Result: 4,3,2,1
//6 .shift(): Removes the first element in the array and returns that element.
var a1 = [1,2,3,4];
a1.shift();
alert(a1);//Result: 2,3,4
//7.unshift (): Insert the specified element into the beginning of the array and return the array.
var a1 = [1,2,3,4];
a1.unshift(5);
alert(a1);//Result: 5,1,2,3,4
//8.slice(): Returns a segment of an array. a1.slice(start, [end]), the slice method copies up to, but does not include, the element specified by end. If start is negative, treat it as length start, where length is the length of the array. If end is negative, it is treated as length end, where length is the length of the array. If end is omitted, the slice method copies until the end of arrayObj. If end appears before start, no elements are copied to the new array.
var a1 = [1,2,3,4];
var a2 = a1.slice(-1);
var a3 = a1.slice(0,-1);
var a4 = a1.slice(1);
alert(a2);//Result: 4
alert(a3);//Result: 1,2,3
alert(a4);//Result :2,3,4
//9.splice(): Remove one or more elements from an array, if necessary, insert a new element at the position of the removed element, and return the removed element .
//arrayObj.splice(start, deleteCount, [item1[, item2[, . . . [,itemN]]]])
var a1 = [1,2,3,4];
var a2 = a1.splice(1,0);
var a3 = a1.splice(1,1);
var a4 = a1.splice(1,1,"5");
alert (a2);//Result:
alert(a3);//Result: 2
alert(a1);//Result: 1,5,4
//10.sort(): Return An Array object whose elements have been sorted.
var a1 = [2,3,1,4,"b","a"];
var a2 = a1.sort();
alert(a2);//Result: 1, 2,3,4,a,b
//11.toString(): Returns the string representation of the object.
var a1 = [1,2,3,4,"b","a"];
var a2 = a1.toString();
alert(a2);//Result: 1, 2,3,4,b,a
//12.valueOf(): Returns the original value of the specified object.
var a1 = [1,2,3,4,"b","a"];
var a2 = a1.valueOf();
alert(a2);//Result: 1, 2,3,4,b,a
indexOf extension:
if (!Array.prototype.indexOf) Array.prototype.indexOf = function(item, i) {
i || (i = 0);
var length = this.length;
if (i for (; i if (this[i] === item ) return i;
return -1;
};
function testIndexOf()
{
var a =[1,2,3,4];
alert(a. indexOf(3));
}
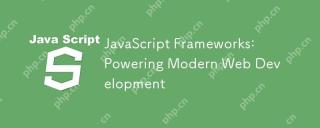
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
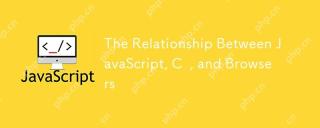
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
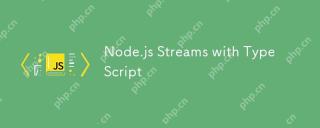
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
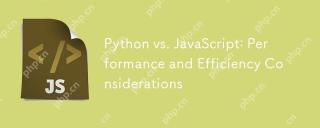
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
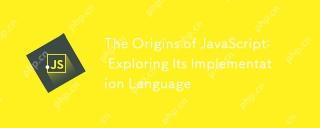
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
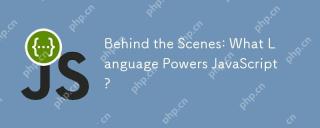
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
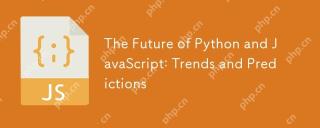
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
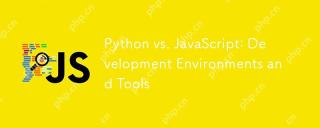
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
