During the summer vacation, I also worked on an ext4 web desktop, and more JavaScript stuff. For javascript, I only knew document.getElementById() and alert() before, but now I am starting to understand it more deeply. If there is anything wrong with this article, please point it out.
Regarding JavaScript, it is based on objects, so it does not have the concept of classes. Therefore, if you want to implement inheritance, you can only use JavaScript's prototype mechanism to achieve it. (Actually, this is wrong. Thanks to @Memory Forest’s tip, you can also use apply and call to achieve it)
Because JavaScript does not have a special mechanism to implement classes, so it can only be nested with the help of its functions. Mechanism to simulate implementation classes. In JavaScript, a function can contain variables or other functions. In this case, we can use variables as attributes of the class and internal functions as member methods. Then, the outer function can be regarded as a class.
1. First, let’s write an animal class. In fact, it is a function, but we can regard it as the constructor of this class
function Animal(){
console.log('Call the constuctor.');
}
2. Then we can use the new keyword to create an instance of the myClass class
var cat = new Animal();
In this way, we have created an instance obj. Run it and use the relevant The debugging tool can see the debugging information of Call the constructor. This proves that we successfully created the class.
3. Then, next, we can also add parameters to the constructor, such as:
function Animal(name){
this.name = name;
}
In this way, we can create instances and access classes The attributes are
function myClass(name){
this.name = name;
}
var cat = new myClass("Kate");
alert(cat.name);
In this way, you can access The attribute name of instance cat is.
4. Everyone knows that animals can jump and eat, so how do we add methods to them? See below
Method 1: Declare the method directly in the constructor
function Animal(name){
this.name = name; this.jump = function(){
alert (this.name " is jumping...");
};
this.eat = function(){
alert (this.name " is eating...");
};
}
var cat = new Animal("Kate");
alert(cat.name);
cat.jump();
cat.eat();
Method 2: Use prototype to add methods to the class
function Animal(name){
this.name = name;
}
Animal.prototype = {
type : 'cat',
jump : function(){
alert (this.name " is jumping...");
},
eat : function(){
alert (this.name " is eating...");
}
}
var cat = new Animal("Kate");
alert(cat.name);
alert(cat.type);
cat.jump();
cat.eat();
Similarly, we also You can use the same method to add new attributes to a class, such as type....
5. What we talked about above is how to create a class in javascript and how to add attributes and methods to the class. Next, Let's talk about how to implement class inheritance.
To achieve inheritance, we can implement class inheritance through prototype. First, we must declare a Dog class (if you still don’t understand, please re-read the relevant content above) and let it inherit the Animal class.
function Dog(){};
Dog. prototype = new Animal("Henry");
Then we can instantiate a new dog and try to call its method to see if it succeeds?
function Dog(){};
Dog.prototype = new Animal("Henry");
var dog = new Dog();
dog.jump();
dog.eat();
Obviously, if the code is correct, you should be able to see the prompts "Henry is jumping...", "Henry is eating...".
6. Now that class inheritance has been implemented, another problem must come to mind, and that is the problem of polymorphism.
Polymorphism means that the same operation, function, or process can be applied to multiple types of objects and obtain different results. Different objects can produce different results when receiving the same message. This phenomenon is called polymorphism.
Through inheritance, the subclass has inherited the methods of the parent class, but to achieve polymorphism, the methods of the subclass must be rewritten.
In order to express it more clearly, we create another Pig class and inherit the Animal class. I won’t tell you how to create it. The created code should look like this.
function Dog(){};
Dog. prototype = new Animal("Henry");
function Pig(){};
Pig.prototype = new Animal("Coco");
var dog = new Dog();
dog .jump();
dog.eat();
var pig = new Pig();
pig.jump();
pig.eat();
After running, because it inherits the Animal class, the result will definitely be "XX is jumping...", "XX is eating...", then what we want to achieve is to rewrite the method. We can implement method overriding in the following ways.
function Dog(){};//Create dog Class
Dog.prototype = new Animal("Henry");
//Override dog method
Dog.prototype.jump = function(){
alert("Hi, this is " this.name ", I'm jumping...")
};
Dog.prototype.eat = function(){
alert("Henry is eating a bone now.");
};
function Pig(){};//Create pig subclass
Pig.prototype = new Animal("Coco");
//Override pig method
Pig.prototype .jump = function(){
alert("I'm sorry. " this.name " can not jump.");
};
Pig.prototype.eat = function(){
alert("Hi, I'm " this.name ", I'm eating something delicious.");
}
var dog = new Dog();
dog.jump();
dog.eat();
var pig = new Pig();
pig.jump();
pig.eat();
Run it, yes Isn't it possible to rewrite the method? ?
6. So, if I instantiate a dog, I want to add attributes and methods to this dog separately. How should I do it? Look below
var dog = new Dog();
//Add attributes and methods
dog.type = "Doberman Pinscher";
dog.shout = function(){
alert("I'm a " this.type ".");
}
dog.jump();
dog.eat();
//Call new method
dog.shout();
7. Well, this article is written here. I believe that beginners should have a certain understanding of class creation and inheritance. If you have any questions, you can leave a message. Thanks for the advice.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
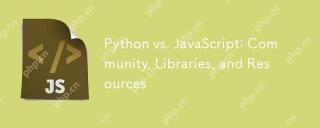
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
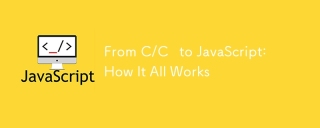
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
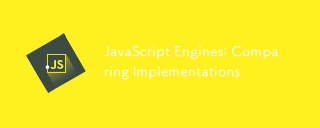
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
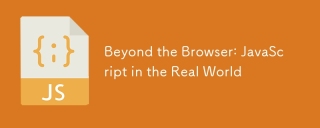
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
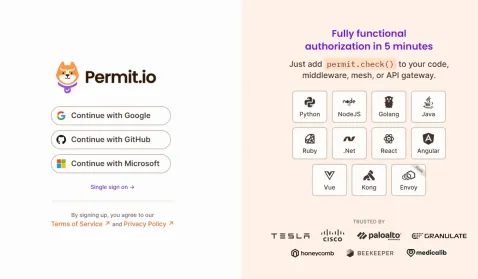
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
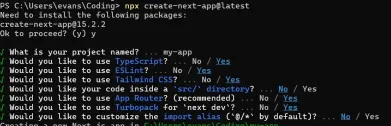
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
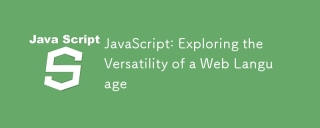
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
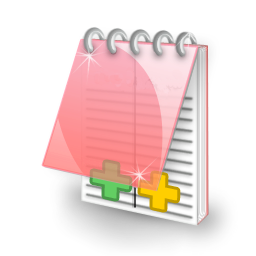
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
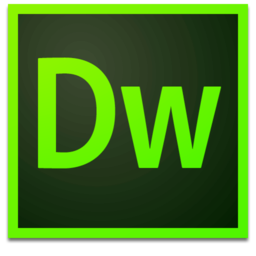
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor