Displaying table data will involve some things, such as showing and hiding fields, data status, paging, etc.
Since our company's products involve a lot of table data display, and each table has a lot of fields, during the work process (with the efforts of several colleagues), we integrated a solution that we thought was relatively lightweight. As shown in the picture: 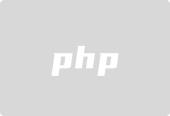
//定义全局命名空间
var GLOBAL = {};
GLOBAL.Namespace = function(str) {
var arr = str.split("."), o = GLOBAL;
for (var i = (arr[0] == 'GLOBAL') ? 1 : 0; i < arr.length; i ) {
o[arr[i]] = o[arr[i]] || {};
o = o[arr[i]];
}
}
GLOBAL.Namespace("zyh");
/*
******************************************************************
jQuery.pager
******************************************************************
*/
(function($) {
$.fn.pager = function(options) {
var opts = $.extend({}, $.fn.pager.defaults, options);
return this.each(function() {
// empty out the destination element and then render out the pager with the supplied options
$(this).empty().append(renderpager(parseInt(options.pagenumber), parseInt(options.pagecount), options.buttonClickCallback, options.rowcount));
// specify correct cursor activity
//$('.pages li').mouseover(function() { document.body.style.cursor = "pointer"; }).mouseout(function() { document.body.style.cursor = "auto"; });
});
};
// render and return the pager with the supplied options
function renderpager(pagenumber, pagecount, buttonClickCallback, rowcount) {
// setup $pager to hold render
var $pager = $('
');
// add in the previous and next buttons
$pager.append(renderButton('|<', pagenumber, pagecount, buttonClickCallback)).append(renderButton('<<', pagenumber, pagecount, buttonClickCallback));
// pager currently only handles 10 viewable pages ( could be easily parameterized, maybe in next version ) so handle edge cases
var startPoint = 1;
var endPoint = 6;
if (pagenumber > 3) {
startPoint = pagenumber - 3;
endPoint = pagenumber 3;
}
if (endPoint > pagecount) {
startPoint = pagecount - 5;
endPoint = pagecount;
}
if (startPoint < 1) {
startPoint = 1;
}
// loop thru visible pages and render buttons
for (var page = startPoint; page <= endPoint; page ) {
var currentButton = $('
' (page) '');
page == pagenumber ? currentButton.addClass('pgCurrent') : currentButton.click(function() { buttonClickCallback(this.firstChild.data); });
currentButton.appendTo($pager);
}
// render in the next and last buttons before returning the whole rendered control back.
$pager.append(renderButton('>>', pagenumber, pagecount, buttonClickCallback)).append(renderButton('>|', pagenumber, pagecount, buttonClickCallback));
$pager.append('
共' pagecount "页");
// if (rowcount != undefined) {
// $pager.append('
共' rowcount "条记录,最多显示600条");
// }
if (rowcount != undefined) {
$pager.append('
' rowcount '条记录 ');
}
return $pager;
}
// renders and returns a 'specialized' button, ie 'next', 'previous' etc. rather than a page number button
function renderButton(buttonLabel, pagenumber, pagecount, buttonClickCallback) {
var $Button = $('
' buttonLabel '');
var destPage = 1;
// work out destination page for required button type
switch (buttonLabel) {
case "|<":
destPage = 1;
break;
case "<<":
destPage = pagenumber - 1;
break;
case ">>":
destPage = pagenumber 1;
break;
case ">|":
destPage = pagecount;
break;
}
// disable and 'grey' out buttons if not needed.
if (buttonLabel == "|<" || buttonLabel == "<<") {
pagenumber <= 1 ? $Button.addClass('pgEmpty') : $Button.click(function() { buttonClickCallback(destPage); });
}
else {
pagenumber >= pagecount ? $Button.addClass('pgEmpty') : $Button.click(function() { buttonClickCallback(destPage); });
}
return $Button;
}
// pager defaults. hardly worth bothering with in this case but used as placeholder for expansion in the next version
$.fn.pager.defaults = {
pagenumber: 1,
pagecount: 1
};
})(jQuery);
/*
======================================== ===========================
//Component function: Move the mouse over the specified target to pop up a drop-down box-- by flowerszhong
//Parameter description:
//target: event object Id
//box: drop-down box Id
//left: left offset from the top coordinate of the event object, the default is 0;
//top: Offset based on the top coordinate of the event object, defaulting to the height of the event object
//overClass: The current status table row maintains the highlighted style
//on: Whether the pop-up box has an arrow
//arrow: Automatically adjusted pointing arrow
======================================== ===========================
*/
var dropbox = function(target, box, left, top, overClass, on, hasArrow) {
var obj, b, p;
if (typeof target == "object")
obj = $(target);
else
obj = $(" #" target);
if (typeof box == "object")
b = $(box);
else
b = $("#" box);
p = obj.parent();
if (top == "undefined") top = obj.height();
var defaults = {
l: left || 0,
t: top | | 0,
overClass: overClass || "",
on: on || "",
hasArrow: hasArrow || ""
},
offset = obj.offset() ,
w = $(window).height(),
selectSet = function(flag) {
//In IE6, prevent the select control from covering the drop-down box
if ($.browser. msie && $.browser.version == "6.0") {
if (flag) {
$("select").css("visibility", "visible");
} else {
$("select").css("visibility", "hidden");
}
}
};
$(window).resize(function() {
w = $(window).height();
offset = obj.offset();
});
$("#arrow").click(function() {
offset = obj.offset();
});
//Bind mouseover event
obj.bind("mouseover", function() {
var diff, arrow, scrollTop;
scrollTop = $(window).scrollTop();
diff = w - (offset.top - scrollTop);
if (on && diff < 145) {
var subTop = 145 - diff;
b.css({ "display": "block", "left": offset.left defaults.l "px", "top": offset.top defaults.t - subTop "px" });
if (hasArrow) {
arrow = b.children("div")[0];
var arrTop = 35 subTop;
$(arrow).css("top", arrTop);
}
} else {
b.css({ "display": "block", "left": offset.left defaults.l "px", "top": offset.top defaults.t "px" });
if (hasArrow) {
arrow = b.children("div")[0];
$(arrow).css("top", "35px");
}
}
b.bind("mouseover", function(event) {
$(this).show();
selectSet(false);
if (overClass) { p .addClass(overClass); }
event.stopPropagation(); //Prevent event bubbling
});
b.bind("mouseout", function(event) {
$(this ).hide();
selectSet(true);
if (overClass) { p.removeClass(overClass); }
event.stopPropagation(); //Prevent event bubbling
}) ;
if (overClass) { p.addClass(overClass); }
selectSet(false);
});
//Bind mouseout event
obj.bind("mouseout" , function() {
b.css("display", "none");
selectSet(true);
if (overClass) { p.removeClass(overClass); }
}) ;
//debugger;
};
GLOBAL.zyh.dropbox = dropbox;
/*
================== ==============================================
/ /Function: Toggle Column table custom column general method, based on jquery.columnmanager component, GLOBAL.zyh.dropbox component
//Parameter description:
//targetTable: Table Id
//columnManagerArgument:jquery. columnmanager requires parameters
//btnSetColumn:
//targetfive:
//left: 0
//top: 0
============== ==================================================
*/
GLOBAL.zyh.toggleTableColumn = function(options) {
var defaults = {
targetTable: '',
columnManagerArgument: {},
btnSetColumn: '',
targetfive: '',
left: 0,
top: 0
}
var settings = $.extend({}, defaults, options);
$('# ' settings.targetTable).columnManager(settings.columnManagerArgument);
GLOBAL.zyh.dropbox(settings.btnSetColumn, settings.targetfive, settings.left, settings.top);
}