1. Reason:
This article originated from a question in the OSC community about how to use jq to obtain pseudo elements. My first thought is that the powerful CSS Query should be able to obtain pseudo elements.
However, in fact, CSS Query cannot. That is, we cannot obtain the :before pseudo-element through $(":before"), $(dom).find(":before") or document.querySelector(":before").
To do this, I had to re-understand pseudo-elements (Pseudo-elements). Why can't we directly get pseudo elements using JS?
For example, the ::before and ::after pseudo-elements are used to insert content into the head or tail of an element in CSS rendering. They are not bound by the document and do not affect the document itself, but only affect the final style. These added content will not appear in the DOM, but will only be added in the CSS rendering layer.
In fact, pseudo-elements can be rendered by the browser, but they are not DOM elements themselves. It doesn't exist in the document, so JS can't manipulate it directly. jQuery's selectors are all based on DOM elements, so they cannot directly operate pseudo-elements.
How to manipulate the style of pseudo elements?
For this reason, I decided to summarize the methods of controlling pseudo elements in JS for easy reference in the future.
2. What are the pseudo elements:
First of all, let’s briefly talk about what pseudo-elements are. There are six pseudo-elements, namely ::after, ::before, ::first-line, ::first-letter, ::selection, ::backdrop.
The most commonly used pseudo-elements in major web pages are ::after and ::before.
For the semantics of these pseudo-elements, please refer to my other article "CSS Pseudo-Element Selector Summary".
In CSS3, it is recommended that pseudo-elements use two colons (::) syntax instead of one colon (:) in order to distinguish pseudo-classes and pseudo-elements. Most browsers support both presentation syntaxes. Only ::selection can always start with two colons (::). Because IE8 only supports single colon syntax, if you want to be compatible with IE8, the safest way is to use a single colon.
3. Get the attribute value of the pseudo element:
To obtain the attribute value of a pseudo element, you can use the window.getComputedStyle() method to obtain the CSS style declaration object of the pseudo element. Then use the getPropertyValue method or directly use key-value access to obtain the corresponding property value.
Syntax: window.getComputedStyle(element[, pseudoElement])
parameters are as follows:
element (Object): the DOM element where the pseudo element is located;
pseudoElement (String): Pseudo element type. Optional values are: ":after", ":before", ":first-line", ":first-letter", ":selection", ":backdrop";
For example:
// CSS代码 #myId:before { content: "hello world!"; display: block; width: 100px; height: 100px; background: red; } // HTML代码 <div id="myId"></div> // JS代码 var myIdElement = document.getElementById("myId"); var beforeStyle = window.getComputedStyle(myIdElement, ":before"); console.log(beforeStyle); // [CSSStyleDeclaration Object] console.log(beforeStyle.width); // 100px console.log(beforeStyle.getPropertyValue("width")); // 100px console.log(beforeStyle.content); // "hello world!"
Remarks:
1. Both getPropertyValue() and direct key-value access can access CSSStyleDeclaration Object. The differences between them are:
For float attributes, if you use key-value access, you cannot use getComputedStyle(element, null).float directly, but cssFloat and styleFloat;
If you use key-value access directly, the attribute key needs to be written in camel case, such as: style.backgroundColor;
The getPropertyValue() method does not have to be written in camel case (camel case is not supported), for example: style.getPropertyValue("border-top-color");
The getPropertyValue() method is supported in IE9+ and other modern browsers; in IE6~8, you can use the getAttribute() method instead;
2. The default pseudo element is "display: inline". If the display attribute is not defined, even if the width attribute value is explicitly set to a fixed size such as "100px" in CSS, the finally obtained width value is still "auto". This is because the width and height of inline elements cannot be customized. The solution is to modify the display attribute of the pseudo element to "block", "inline-block" or other.
4. Change the style of pseudo-element:
Method 1. Change the class to change the attribute value of the pseudo element:
Give me an example:
// CSS代码 .red::before { content: "red"; color: red; } .green::before { content: "green"; color: green; } // HTML代码 <div class="red">内容内容内容内容</div> // jQuery代码 $(".red").removeClass('red').addClass('green');
Method 2. Use insertRule of CSSStyleSheet to modify the style of pseudo-elements:
Give me an example:
document.styleSheets[0].addRule('.red::before','color: green'); // 支持IE document.styleSheets[0].insertRule('.red::before { color: green }', 0); // 支持非IE的现代浏览器
Method 3. Insert the internal style of
var style = document.createElement("style"); document.head.appendChild(style); sheet = style.sheet; sheet.addRule('.red::before','color: green'); // 兼容IE浏览器 sheet.insertRule('.red::before { color: green }', 0); // 支持非IE的现代浏览器
Or use jQuery:
$('<style>.red::before{color:green}</style>').appendTo('head');
5. Modify the content attribute value of the pseudo element:
Method 1. Use insertRule of CSSStyleSheet to modify the style of the pseudo element:
var latestContent = "修改过的内容"; var formerContent = window.getComputedStyle($('.red'), '::before').getPropertyValue('content'); document.styleSheets[0].addRule('.red::before','content: "' + latestContent + '"'); document.styleSheets[0].insertRule('.red::before { content: "' + latestContent + '" }', 0);
Method 2. Use the data-* attribute of the DOM element to change the value of content:
// CSS代码 .red::before { content: attr(data-attr); color: red; } // HTML代码 <div class="red" data-attr="red">内容内容内容内容</div> // JacaScript代码 $('.red').attr('data-attr', 'green');
六. :before和:after伪元素的常见用法总结:
1. 利用content属性,为元素添加内容修饰:
1) 添加字符串:
使用引号包括一段字符串,将会向元素内容中添加字符串。栗子:
a:after { content: "after content"; }
2) 使用attr()方法,调用当前元素的属性的值:
栗子:
a:after { content: attr(href); } a:after { content: attr(data-attr); }
3)使用url()方法,引用多媒体文件:
栗子:
a::before { content: url(logo.png); }
4) 使用counter()方法,调用计时器:
栗子:
h:before { counter-increment: chapter; cotent: "Chapter " counter(chapter) ". " }
2. 清除浮动:
.clear-fix { *overflow: hidden; *zoom: 1; } .clear-fix:after { display: table; content: ""; width: 0; clear: both; }
3. 特效妙用:
// CSS代码 a { position: relative; display: inline-block; text-decoration: none; color: #000; font-size: 32px; padding: 5px 10px; } a::before, a::after { content: ""; transition: all 0.2s; } a::before { left: 0; } a::after { right: 0; } a:hover::before, a:hover::after { position: absolute; } a:hover::before { content: "\5B"; left: -20px; } a:hover::after { content: "\5D"; right: -20px; } // HTML代码 <a href="#">我是个超链接</a>
4. 特殊形状的实现:
举个栗子:(譬如对话气泡)
// CSS代码 .tooltip { position: relative; display: inline-block; padding: 5px 10px; background: #80D4C8; } .tooltip:before { content: ""; display: block; position: absolute; left: 50%; margin-left: -5px; bottom: -5px; width: 0; height: 0; border-left: 5px solid transparent; border-right: 5px solid transparent; border-top: 5px solid #80D4C8; } // HTML代码 <div class="tooltip">I'm a tooltip.</div>
:before 和 :after 伪元素结合更多CSS3强大的特性,还可完成非常多有趣的特效和 hack ,这里权当抛砖引玉。
六. 一点小小建议:
伪元素的content属性很强大,可以写入各种字符串和部分多媒体文件。但是伪元素的内容只存在于CSS渲染树中,并不存在于真实的DOM中。所以为了SEO优化,最好不要在伪元素中包含与文档相关的内容。
修改伪元素的样式,建议使用通过更换class来修改样式的方法。因为其他两种通过插入行内CSSStyleSheet的方式是在JavaScript中插入字符代码,不利于样式与控制分离;而且字符串拼接易出错。
修改伪元素的content属性的值,建议使用利用DOM的data-*属性来更改。
以上所述是小编给大家介绍的JS控制伪元素的方法汇总,希望对大家有所帮助!
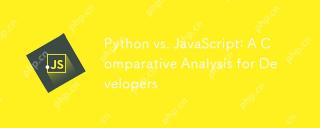
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
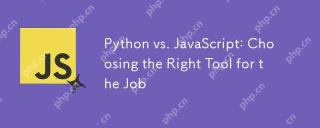
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
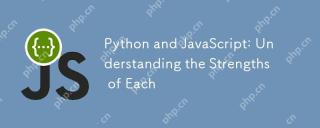
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
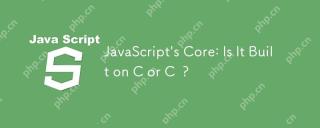
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
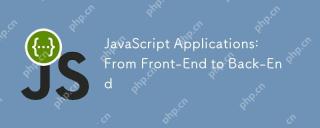
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
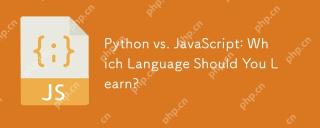
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
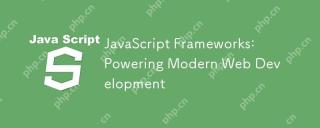
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
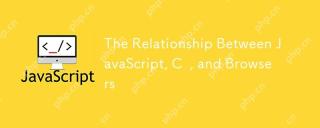
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
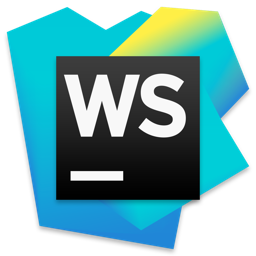
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
