1. Create a new demo.aspx page.
2. First add a reference to the background file demos.aspx.cs of the page.
using System.Web.Services;
3. Method call without parameters. Please note that this version cannot be lower than .net framework 2.0. 2.0 is no longer supported.
Backend code:
[WebMethod]
public static string SayHello()
{
return "Hello Ajax!";
}
JS code:
$(function() {
$("#btnOK").click(function() {
$.ajax({
//To use the post method
type: "Post",
//The page where the method is located and the method name
url: "Demo.aspx/SayHello",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function(data) {
//The returned data uses data.d to obtain the content
alert(data.d);
},
error: function(err) {
alert(err);
}
});
//Disable button Submit
return false;
});
});
Page code:
3. Method call with parameters
Backend code:
[WebMethod]
public static string GetStr(string str, string str2)
{
return str str2;
}
JS code:
$(function() {
$("#btnOK").click(function() {
$.ajax({
type: "Post",
url: "demo.aspx/GetStr",
//The method parameters must be written correctly, str is the name of the formal parameter, str2 is the name of the second formal parameter
data: "{'str':'I am','str2':'XXX'}",
contentType: "application/json; charset=utf-8 ",
dataType: "json",
success: function(data) {
//The returned data is obtained using data.d
alert(data.d);
},
error: function(err) {
alert(err);
}
});
//Disable button submission
return false;
} );
});
The operation effect is as follows:
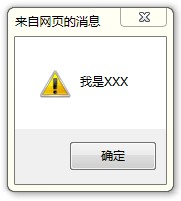
4. Return array method
Backend code:
[WebMethod]
public static List GetArray()
{
List li = new List();
for (int i = 0 ; i < 10; i )
li.Add(i "");
return li;
}
JS code:
$(function() {
$("#btnOK"). click(function() {
$.ajax({
type: "Post",
url: "demo.aspx/GetArray",
contentType: "application/json; charset=utf- 8",
dataType: "json",
success: function(data) {
//Clear ul before inserting
$("#list").html("");
//Recursively obtain data
$(data.d).each(function() {
//Insert the results into li
$("#list").append("
" this "");
});
alert(data.d);
},
error: function(err) {
alert(err);
}
});
//Disable button submission
return false;
});
});
Run result chart:
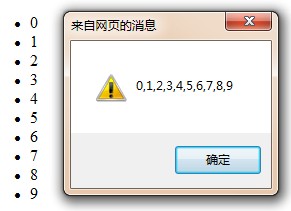