


The right way to extend JavaScript functionality (translation)_javascript tips
I saw the article "JavaScript Weekly Introduction" [Issue 3] this morning, and found an article in it (Extending JavaScript – The Right Way), which I thought was pretty good, translated Come and share it with everyone. This article is not translated word for word. I try to make it as easy to understand as possible.
Original address: Extending JavaScript – The Right Way
The following is the translation
JavaScript has built-in many powerful methods, but sometimes A certain function you need is not available in the built-in method. How can we extend JavaScript functions elegantly?
For example, we want to add a capitalize() method to capitalize the first letter. Usually we write it like this:
if(!String.prototype.capitalize)
{
String.prototype.capitalize = function()
{
return this.slice(0, 1).toUpperCase() this.slice(1).toLowerCase();
}
}
The above code can be used normally, but if there is the following somewhere Code:
var strings = "yay";
for(i in strings) console.log(i ":" strings[i]);
The result we get is this:
0: y
1: a
2: y
capitalize: function () { return this.slice(0, 1).toUpperCase() this.slice(1).toLowerCase(); }
This is obviously not the result we want , the reason why the method we added is output is that the enumerable attribute of the method we added defaults to true.
We can avoid this problem by simply setting the enumeration property (enumerable) to false and use the defineProperty method to extend the functionality:
if(!String.prototype.capitalize)
{
Object.defineProperty(String.prototype, 'capitalize',
{
value: function()
{
return this.slice(0,1).toUpperCase() this.slice(1).toLowerCase();
},
enumerable: false
});
}
Now we run this code again:
var strings = "yay";
for(i in strings) console.log(i ":" strings[i]);
The result we get is:
0: y
1: a
2: y
It should be noted that the lack of output through the loop does not mean that it does not exist. We can pass See the definition in the following code:
var strings = "yay ";
console.log(strings.capitalize)
Will output:
function () { return this.slice(0, 1).toUpperCase() this.slice(1).toLowerCase(); }
It is more flexible to extend JavaScript functions in this way. We can use this way to define our own objects and set some default values.
The following are several other extension methods that you can use in your own projects:
String.pxToInt()
Convert a string like "200px" into the number 200:
if(!String.prototype.pxToInt)
{
Object. defineProperty(String.prototype, 'pxToInt',
{
value: function()
{
return parseInt(this.split('px')[0]);
},
enumerable: false
});
}
String.isHex()
Determine whether a string is expressed in hexadecimal, such as "#CCC" or "#CACACA"
if(!String.prototype.isHex)
{
Object.defineProperty(String.prototype, 'isHex',
{
value: function()
{
return this.substring(0,1) == '#' &&
(this.length == 4 || this.length == 7) &&
/^[0-9a-fA-F] $/.test(this.slice(1));
},
enumerable: false
});
}
String.reverse()
String reverse:
if(!String.prototype.reverse)
{
Object.defineProperty(String.prototype, 'reverse',
{
value: function( )
{
return this.split( '' ).reverse().join( '' );
},
enumerable: false
});
}
String.wordCount()
Count the number of words, separated by spaces
if(!String.prototype.wordCount)
{
Object.defineProperty(String.prototype, 'wordCount',
{
value: function()
{
return this.split(' ').length;
},
enumerable: false
});
}
String. htmlEntities()
HTML tags such as are encoded as special characters
if(!String.prototype.htmlEntities)
{
Object.defineProperty(String.prototype, 'htmlEntities',
{
value: function()
{
return String(this).replace(/&/g, '&').replace(//g, '>').replace(/"/g, '"');
},
enumerable: false
});
}
String.stripTags()
Remove HTML tags:
if(!String.prototype.stripTags)
{
Object.defineProperty(String.prototype, ' stripTags',
{
value: function()
{
return this.replace(/?[^>] >/gi, '');
} ,
enumerable: false
});
}
String.trim()
Remove leading and trailing spaces:
if(!String.prototype.trim)
{
Object.defineProperty(String.prototype, 'trim',
{
value: function()
{
return this.replace(/^s*/, "").replace(/s*$/, "");
},
enumerable: false
});
}
String.stripNonAlpha()
Remove non-alphabetic characters:
if(!String.prototype.stripNonAlpha)
{
Object.defineProperty (String.prototype, 'stripNonAlpha',
{
value: function()
{
return this.replace(/[^A-Za-z ] /g, "");
},
enumerable: false
});
}
Object.sizeof()
Count the size of objects, such as {one: “and”, two: "and"} is 2
if(!Object .prototype.sizeof)
{
Object.defineProperty(Object.prototype, 'sizeof',
{
value: function()
{
var counter = 0;
for(index in this) counter ;
return counter;
},
enumerable: false
});
}
It is quite good to extend the functions of JS native objects in this way, but unless necessary (used a lot in projects), it is not recommended to directly extend functions on native objects, which will cause global variable pollution.
In addition, the pxToInt() method in the article is not necessary. parseInt() in JS can directly complete such a function: parsetInt("200px")===200
There seems to be a problem with the htmlEntities method. Here is another Provide one:
if(!String.prototype.htmlEntities)
{
Object.defineProperty(String.prototype, 'htmlEntities',
{
value: function()
{
var div = document.createElement("div");
if(div.textContent){
div.textContent=this;
}
else{
div.innerText=this;
}
return div.innerHTML;
},
enumerable: false
});
}
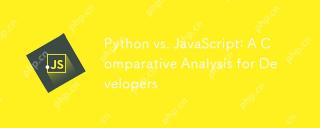
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
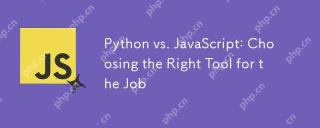
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
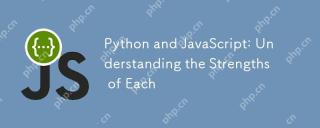
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
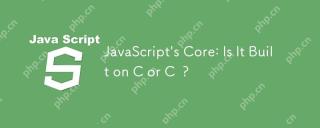
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
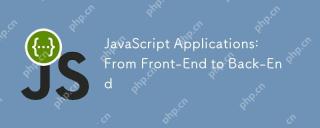
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
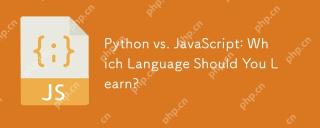
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
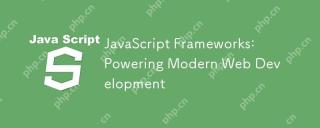
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
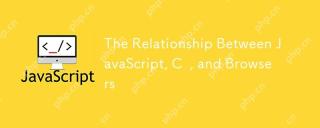
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
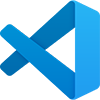
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
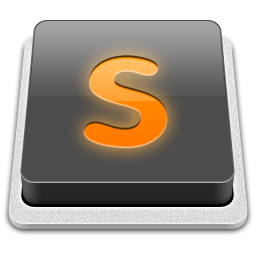
SublimeText3 Mac version
God-level code editing software (SublimeText3)
