


An event is some action performed by the user or the browser itself. For example, click and mouseover are the names of events. The function corresponding to an event is called an event handler (or event listener). There are several ways to specify handlers for events.
1: HTML event handler.
For example:
I believe this method is currently used more often by all of us, but specifying the event handler in html There are two disadvantages.
(1) First of all: there is a time difference problem. For this example, assuming that the show() function is defined below the button and at the bottom of the page, if the user clicks the button before the page parses the show() function, an error will occur;
(2) The second disadvantage is that html is tightly coupled with javascript code. If you want to change the time handler, you need to change two places: html code and javascript code.
As a result, many developers abandon HTML event handlers and instead use JavaScript to specify event handlers.
Two: Javascript designated event handler
Javascript designated event handler includes three methods:
(1): DOM level 0 event handler
Such as:
var btn=document.getElementById("mybtn"); //Get the Reference of the button
btn.onclick=function(){
alert('clicked');
alert(this.id); // mybtn
in this way The added event handler will be processed during the bubbling phase of the event flow.
Delete the event handler specified by the DOM0 level method:
btn.onclick=null; // Delete the event handler
}
(2):DOM2 level event handler
DOM2 level event Two methods are defined to handle the operation of specifying and removing event handlers: addEventListener() and removeEventListener(). These two methods are included in all DOM nodes, and they both accept 3 parameters: the name of the event to be handled, the function as the event handler, and a Boolean value. If the last parameter is true, it means that the event handler is called in the capture phase; if it is fasle, it means that the event handler is called in the bubbling phase.
For example:
var btn=document.getElementById( "mybtn");
btn.addEventListener("click",function(){
alert(this.id);
},false);
Use DOM2 level The main benefit of event handlers is that you can add multiple event handlers.
For example:
var btn=document.getElementById( "mybtn");
btn.addEventListener("click",function(){
alert(this.id);
},false);
btn.addEventListener("click",function (){
alert("hello world!");
},false);
Two event handlers are added here for the button. The two event handlers are fired in the order they occur.
Time handlers added through addEventListener() can only be removed using removeEventListener(). The parameters passed in when removing are the same as those used when adding. This also means that anonymous functions added through addEventListener() cannot be removed.
For example:
var btn=document.getElementById( "mybtn");
btn.addEventListener("click",function(){
alert(this.id);
},false);
//Remove
btn. removeEventListener("click",function(){ //Writing like this is useless (because the second time is a completely different function than the first time)
alert(this.id);
}, false);
Solution:
var btn=document.getElementById("mybtn");
var hander=function(){
alert(this.id);
};
btn.addEventListener("click" ,hander,false);
btn.removeEventListener("click",hander,false); // Valid
Note: Our third parameter here is all false, which is added during the bubbling stage. In most cases, event handlers are added to the bubbling stage of the event flow, so as to maximize compatibility with various browsers.
3: IE event handler
IE implements two methods similar to those in DOM: attachEvent() and detachEvent(). Both methods accept the same two parameters: event handler name and event handler function. Since IE only supports time bubbling, all event handlers added through attachEvent() will be added to the bubbling stage.
For example:
Three:
var btn=document.getElementById("mybtn");
btn.attachEvent("onclick",function(){
alert("clicked");
})
Note: The first parameter of the attachEvent() function is "onclick", not "click" in the DOM's addEventListener(). The
attachEvent() method can also be used to add multiple event handlers to an element.
For example:
var btn=document.getElementById( "mybtn");
btn.attachEvent("onclick",function(){
alert("clicked");
});
btn.attachEvent("onclick",function() {
alert("hello world!");
});
AttachEvent() is called twice here, adding two different event handlers for the same button . However, unlike DOM methods, these event handlers are not executed in the order in which they were added, but are fired in the reverse order. Click the button in this example: the first thing you see is "hello world", and then "clicked".
Events added using attachEvent() can be removed by detachEvent(), provided that the same parameter.
var btn=document.getElementById("mybtn");
var hander=function(){
alert("clicked");
}
btn.detachEvent("onclick",hander}); // Remove
The above three methods are currently the main event handler methods. When you see this, you will definitely think that since different browsers will have different differences, how to ensure cross-browser event handlers?
In order to handle events in a cross-browser manner, many developers use Javascript libraries that can isolate browser differences, and some developers develop the most appropriate event handling methods themselves.
An EventUtil object is provided here, which can be used to handle differences during browsing:
var EventUtil = {
addHandler: function(element, type, handler){ // This method accepts 3 parameters: the element to be operated on, the event name and the event handler function
if (element.addEventListener){ //Check whether the passed in element has a DOM2 level method
element.addEventListener(type, handler, false); // If it exists, use this method
} else if (element .addEvent){ // If there is an IE method
element.attachEvent("on" type, handler); // then use the IE method. Note that the event type here must be prefixed with "on".
} else { // The last possibility is to use DOM0 level
element["on" type] = hander;
}
},
removeHandler: function(element, type, handler){ // This method is to delete the previously added event handler
if (element.removeEventListener){ //Check whether the passed-in element exists DOM2 level method
element.removeEventListener(type, handler, false); // If it exists, use this method
} else if (element.detachEvent){ // If it exists, IE's method
element.detachEvent("on" type, handler); // Then use the IE method. Note that the event type here must be prefixed with "on".
} else { // The last possibility is to use DOM0 and methods (in modern browsers, the code here should not be executed)
element["on" type] = null;
}
}
};
You can use the EventUtil object as follows:
var btn =document.getElementById("mybtn");
var hander= function(){
alert("clicked");
};
//Omitted here Part of the code is omitted
EventUtil.addHandler(btn,"click",hander);
//Part of the code is omitted here
EventUtil.removeHandler(btn,"click",hander); //Before removal The added event handler
is visible. It is very convenient to use addHandler and removeHandler to add and remove event handlers.
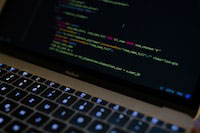
pythonGUI编程简述GUI(GraphicalUserInterface,图形用户界面)是一种允许用户通过图形方式与计算机交互的方式。GUI编程是指使用编程语言来创建图形用户界面。Python是一种流行的编程语言,它提供了丰富的GUI库,使得PythonGUI编程变得非常简单。PythonGUI库介绍Python中有许多GUI库,其中最常用的有:Tkinter:Tkinter是Python标准库中自带的GUI库,它简单易用,但功能有限。PyQt:PyQt是一个跨平台的GUI库,它功能强大,
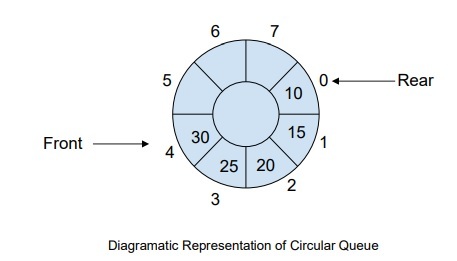
介绍CircularQueue是对线性队列的改进,它被引入来解决线性队列中的内存浪费问题。循环队列使用FIFO原则来插入和删除其中的元素。在本教程中,我们将讨论循环队列的操作以及如何管理它。什么是循环队列?循环队列是数据结构中的另一种队列,其前端和后端相互连接。它也被称为循环缓冲区。它的操作与线性队列类似,那么为什么我们需要在数据结构中引入一个新的队列呢?使用线性队列时,当队列达到其最大限制时,尾指针之前可能会存在一些内存空间。这会导致内存损失,而良好的算法应该能够充分利用资源。为了解决内存浪费
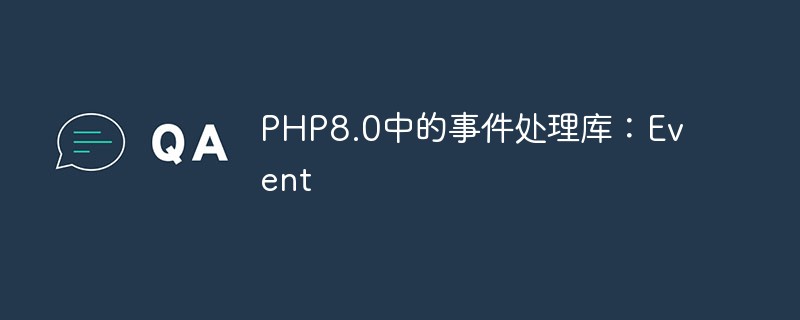
PHP8.0中的事件处理库:Event随着互联网的不断发展,PHP作为一门流行的后台编程语言,被广泛应用于各种Web应用程序的开发中。在这个过程中,事件驱动机制成为了非常重要的一环。PHP8.0中的事件处理库Event将为我们提供一个更加高效和灵活的事件处理方式。什么是事件处理在Web应用程序的开发中,事件处理是一个非常重要的概念。事件可以是任何一种用户行
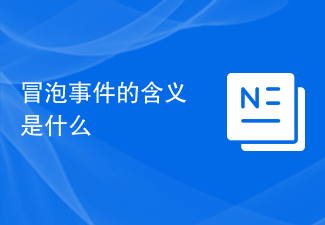
冒泡事件是指在Web开发中,当一个元素上触发了某个事件后,该事件将会向上层元素传播,直到达到文档根元素。这种传播方式就像气泡从底部逐渐冒上来一样,因此被称为冒泡事件。在实际开发中,了解和理解冒泡事件的工作原理对于正确处理事件十分重要。下面将通过具体的代码示例来详细介绍冒泡事件的概念和使用方法。首先,我们创建一个简单的HTML页面,其中包含一个父级元素和三个子
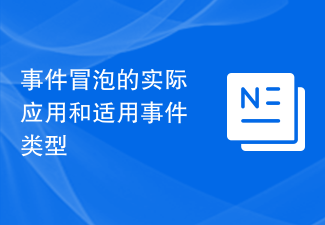
事件冒泡的应用场景及其支持的事件种类事件冒泡是指当一个元素上的事件被触发时,该事件会被传递给该元素的父元素,再传递给该元素的祖先元素,直到传递到文档的根节点。它是事件模型的一种重要机制,具有广泛的应用场景。本文将介绍事件冒泡的应用场景,并探讨它所支持的事件种类。一、应用场景事件冒泡在Web开发中有着广泛的应用场景,下面列举了几个常见的应用场景。表单验证在表单
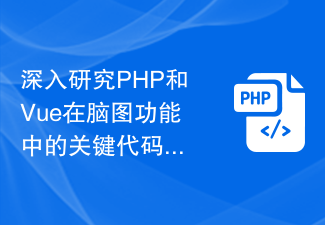
深入研究PHP和Vue在脑图功能中的关键代码实现摘要:本文将深入探讨PHP和Vue在实现脑图功能中的关键代码实现。脑图是一种常用于展示思维结构和关联关系的图形工具,被广泛应用于项目规划、知识管理和信息整理等领域。通过学习PHP和Vue的相关知识,我们可以实现一个简单而功能强大的脑图应用。了解PHPPHP是一种常用的服务器端脚本语言。它具有简单易学、可扩展性强
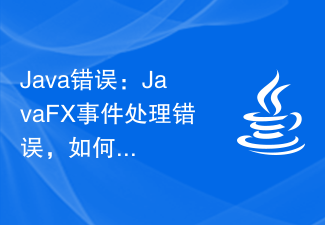
作为一种流行的编程语言,Java在开发过程中经常会遇到各种错误和问题。而其中的JavaFX事件处理错误是一个较为常见的问题,它可能导致应用程序崩溃或失败。本文将介绍JavaFX事件处理错误的原因、解决方法和预防措施,帮助开发人员避免和处理这种错误。错误原因JavaFX事件处理错误通常是由以下原因引起的:1)代码错误:在编写JavaFX代码时,常常会发生代码错
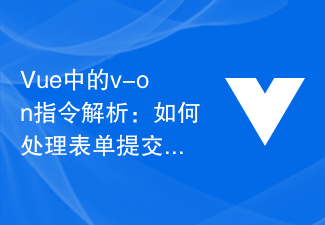
Vue中的v-on指令解析:如何处理表单提交事件在Vue.js中,v-on指令用于绑定事件监听器,可以捕获并处理各种DOM事件。其中,处理表单提交事件是Vue中常见的操作之一。本文将介绍如何使用v-on指令处理表单提交事件,并提供具体的代码示例。首先,需要明确Vue中的表单提交事件指的是当用户点击submit按钮或按下回车键时触发的事件。在Vue中,可以通过


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
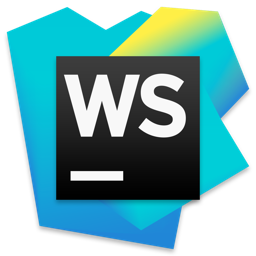
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
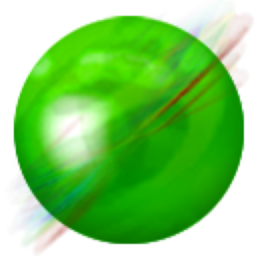
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
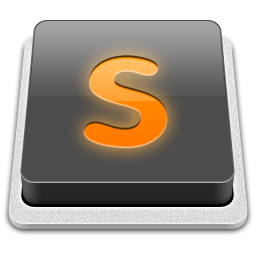
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!
