The first time I swallowed it wholeheartedly, I didn't ask for a clear explanation, and I suddenly felt enlightened. However, when I went to bed at night, I found many problems and didn't understand anything. After reading it a second time, I found that it was like this. After using it for a few days, I found that I was still relying on memory when writing by hand, so the next time, the next time...
It is unreliable to figure out things by memory alone, and my mind will go blank after a long time. Especially many technical ideas and principles, if you just don’t practice them, even if you think about them very clearly at the time, you will forget them after a long time. Furthermore, some things on the Internet can only be said to provide a convenient way to view them. It is better to summarize them yourself afterwards. After all, most of them are personal summaries. Some concepts are difficult to explain clearly, and two people are talking about the same thing. , generally speaking, the steps and chapters are different, so it is easy to form cross memories. The more cross memories, the more confusing it will be. It's better to look at things with a skeptical attitude. Try it out and you'll know what it's like. Books with guaranteed high quality or official stuff are good sources.
While you can still see clearly now and your mind is still clear, record it and make a memo. The conceptual stuff is in the book to reduce misunderstanding in the future. Write the example by hand and verify it, and then draw a picture so that you can understand it at a glance later.
1. Encapsulation
Object definition: ECMA-262 defines an object as: "a collection of unordered attributes, where attributes can include basic values, objects or functions" .
Create objects: Each object is created based on a reference type. This reference type can be a native type (Object, Array, Date, RegExp, Function, Boolean, Number, String) or a self- Define type.
1. Constructor pattern
function Person(name, age) {
this.name = name;
this.age = age;
this.sayName = function() {
alert(this.name);
}
}
Object instances can be created using the new operator through the above constructor.
var zhangsan = new Person('zhangsan', 20);
var lisi = new Person('lisi', 20);
zhangsan.sayName();//zhangsan
lisi.sayName (); //lisi
Creating an object through new goes through 4 steps
1. Create a new object; [var o = new Object();]
2. Assign the scope of the constructor to the new object (so this points to the new object); [Person.apply(o)] [Person’s original this points to window]
3. Execute the code in the constructor (add attributes to this new object);
4. Return the new object.
Steps to restore new through code:
function createPerson(P) {
var o = new Object();
var args = Array.prototype.slice.call(arguments, 1);
o.__proto__ = P.prototype;
P.prototype.constructor = P;
P.apply(o, args);
}
Test the new create instance method
var wangwu = createPerson(Person, 'wangwu', 20) ;
wangwu.sayName();//wangwu
2. Prototype mode
Prototype object concept: Whenever you create a new function, A prototype attribute is created for the function according to a specific set of rules. This attribute points to the prototype object of the function. By default, all prototype objects automatically get a constructor property, which contains a pointer to the function where the prototype property is located. Through this constructor, you can continue to add other properties and methods to the prototype object. After creating a custom constructor, its prototype object will only obtain the constructor property by default; as for other methods, they are inherited from Object. When a constructor is called to create a new instance, the instance will contain a pointer (internal property) that points to the prototype object of the constructor. ECMA-262 5th Edition calls this pointer [[Prototype]]. There is no standard way to access [[Prototype]] in scripts, but Firefox, Safari, and Chrome support an attribute __proto__ on every object; in other implementations, this attribute is completely invisible to scripts. The really important point to make clear, though, is that the connection exists between the instance and the constructor's prototype object, not between the instance and the constructor.
This paragraph basically outlines the relationship between constructors, prototypes, and examples. The following figure shows it more clearly
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.country = 'chinese';
Person.prototype.sayCountry = function() {
alert(this.country);
}
var zhangsan = new Person('zhangsan', 20);
var lisi = new Person('lisi', 20);
zhangsan.sayCountry(); //chinese
lisi.sayCountry(); //chinese
alert(zhangsan.sayCountry = = lisi.sayCountry); //true
Note: The prototype object of the constructor is mainly used to allow multiple object instances to share the properties and methods it contains. But this is also where problems tend to occur. If the prototype object contains a reference type, then the reference type stores a pointer, so value sharing will occur. As follows:
Person.prototype.friends = ['wangwu' ]; //Person adds an array type
zhangsan.friends.push('zhaoliu'); //Zhang San’s modification will affect Li Si
alert(zhangsan.friends); //wangwu,zhaoliu
alert(lisi.friends); //wangwu, zhaoliu Li Si also has one more
3. Use the constructor mode and prototype mode in combination
This mode is to use The most widespread and recognized way to create custom types. Constructor pattern is used to define instance properties, while prototype pattern is used to define methods and shared properties. In this way, each instance has its own copy of the instance attributes and shares references to methods, which saves memory to the maximum extent.
The modified prototype mode is as follows:
function Person(name, age) {
this.name = name;
this.age = age;
this.friends = ['wangwu'];
}
Person.prototype.country = 'chinese';
Person.prototype.sayCountry = function() {
alert(this.country);
}
var zhangsan = new Person(' zhangsan', 20);
var lisi = new Person('lisi', 20);
zhangsan.friends.push('zhaoliu');
alert(zhangsan.friends); / /wangwu,zhaoliu
alert(lisi.friends); //wangwu
2. Inheritance
Basic concepts of inheritance
ECMAScript mainly relies on the prototype chain Implement inheritance (you can also inherit by copying properties).
The basic idea of the prototype chain is to use prototypes to let one reference type inherit the properties and methods of another reference type. The relationship between constructors, prototypes, and examples is: each constructor has a prototype object, the prototype object contains a pointer to the constructor, and the instance contains an internal pointer to the prototype. So, by making the prototype object equal to an instance of another type, the prototype object will contain a pointer to the other prototype, and accordingly, the other prototype will also contain this pointer to the other constructor. If another prototype is an instance of another type, then the above relationship still holds, and so on, layer by layer, a chain of instances and prototypes is formed. This is the basic concept of the prototype chain.
It is confusing to read and difficult to understand. Verify directly through examples.
1. Prototype chain inheritance
function Parent() {
this.pname = 'parent';
}
Parent.prototype.getParentName = function() {
return this.pname;
}
function Child() {
this.cname = 'child';
}
//The child constructor prototype is set to an instance of the parent constructor, forming a prototype chain so that Child has the getParentName method
Child .prototype = new Parent();
Child.prototype.getChildName = function() {
return this.cname;
}
var c = new Child();
alert(c.getParentName()); //parent
Illustration:
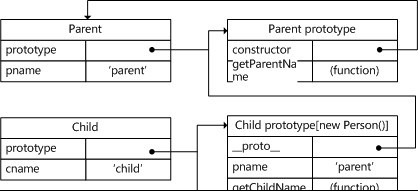
Prototype chain problem, if the parent class includes a reference type, Child.prototype = new Parent() will bring the reference type in the parent class to In the prototype of a subclass, prototype properties of reference type values will be shared by all instances. The problem comes back to section [1, 2].
2. Combination inheritance - the most commonly used inheritance method
Combination inheritance is a combination of prototype chain and borrowed constructor (apply, call) technologies. The idea is to use the prototype chain to achieve inheritance of prototype properties and methods, and to achieve inheritance of instance properties by borrowing constructors. In this way, methods can be defined on the prototype to achieve function reuse, and each instance can be guaranteed to have its own attributes.
function Parent(name) {
this.name = name;
this.colors = ['red', 'yellow'];
}
Parent.prototype.sayName = function() {
alert(this.name);
}
function Child(name, age) {
Parent.call(this, name); //Call Parent() for the second time
this.age = age;
}
Child.prototype = new Parent(); //The first time Parent() is called, the properties of the parent class will be
Child.prototype.sayAge = function() {
alert(this.age );
}
var c1 = new Child('zhangsan', 20);
var c2 = new Child('lisi', 21);
c1.colors.push( 'blue');
alert(c1.colors); //red,yellow,blue
c1.sayName(); //zhangsan
c1.sayAge(); //20
alert(c2.colors); //red,yellow
c2.sayName(); //lisi
c2.sayAge(); //21
combination inheritance The problem is that the supertype constructor is called twice each time: once when creating the subtype prototype, and once inside the subtype constructor. This will cause the rewriting of attributes. The subtype constructor contains the attributes of the parent class, and the prototype object of the subclass also contains the attributes of the parent class.
3. Parasitic combination inheritance - the most perfect inheritance method
The so-called parasitic combination inheritance means inheriting properties by borrowing constructors and inheriting methods through the hybrid form of the prototype chain. The basic idea behind it is: instead of calling the supertype's constructor to specify the prototype of the subclass, all we need is a copy of the supertype's prototype
function extend(child, parent) {
var F = function(){}; //Define an empty constructor
F.prototype = parent.prototype; //Set as the prototype of the parent class
child.prototype = new F(); //Set the prototype of the subclass as an instance of F, forming a prototype chain
child .prototype.constructor = child; //Reassign the subclass constructor pointer
}
function Parent(name) {
this.name = name;
this.colors = [' red', 'yellow'];
}
Parent.prototype.sayName = function() {
alert(this.name);
}
function Child(name, age) {
Parent.call(this, name);
this.age = age;
}
extend(Child, Parent); //Implement inheritance
Child. prototype.sayAge = function() {
alert(this.age);
}
var c1 = new Child('zhangsan', 20);
var c2 = new Child( 'lisi', 21);
c1.colors.push('blue');
alert(c1.colors); //red,yellow,blue
c1.sayName(); //zhangsan
c1.sayAge(); //20
alert(c2.colors); //red,yellow
c2.sayName(); //lisi
c2.sayAge() ; //21