


Javascript array and dictionary usage and traversing object attribute techniques_javascript techniques
Javascript’s array Array is both an array and a dictionary. Let’s take an example to see how to use arrays.
var a = new Array();
a [0] = "Acer";
a[1] = "Dell";
for (var i = 0; i alert(a[i]) ;
}
Let’s take a look at how to use the dictionary.
var computer_price = new Array();
computer_price ["Acer"] = 500;
computer_price["Dell"] = 600;
alert(computer_price["Acer"]);
We can even traverse as above This array (dictionary)
for (var i in computer_price) {
alert(i ": " computer_price[i]);
}
The i here is each key value of the dictionary. The output result is:
Acer: 500
Dell: 600
In addition, computer_price is a dictionary object, and each of its key values is an attribute. In other words, Acer is an attribute of computer_price. We can use it like this:
computer_price.Acer
Let’s look at the simplified declaration of dictionaries and arrays.
var array = [1, 2, 3]; // Array
var array2 = { "Acer": 500, "Dell": 600 }; // Dictionary
alert(array2.Acer); // 500
This declaration of the dictionary is the same as the previous one. In our example, Acer is a key value and can also be used as an attribute of the dictionary object.
Let’s take a look at how to traverse the properties of an object. We can use for in to iterate over the properties of an object.
function Computer(brand, price) {
this .brand = brand;
this.price = price;
}
var mycomputer = new Computer("Acer", 500);
for (var prop in mycomputer) {
alert( "computer[" prop "]=" mycomputer[prop]);
}
In the above code, Computer has two attributes, brand and price. So the output result is:
computer[brand]=Acer
computer[price]=500
The above usage can be used to see what attributes an object has. When you already know that the Computer object has a brand attribute, you can use
mycomputer.brand
or mycomputer[brand]
to get the attribute value.
Summary: Arrays in Javascript can also be used to make dictionaries. The key values of a dictionary are also properties of the dictionary object. When traversing the properties of an object, you can use for in.
Array traversal and properties
Although arrays are objects in JavaScript, there is no good reason to use a for in loop to traverse the array.
Conversely, there are some good reasons not to use for in to iterate over arrays.
Note: Arrays in JavaScript are not associative arrays.
There are only objects in JavaScript to manage key-value correspondence. But associative arrays maintain order, while objects do not.
Since the for in loop enumerates all properties on the prototype chain, the only way to filter these properties is to use the hasOwnProperty function,
so it will be many times slower than an ordinary for loop.
Traversal
In order to achieve the best performance of traversing the array, it is recommended to use the classic for loop.
var list = [1, 2, 3, 4, 5, ... 100000000];
for(var i = 0, l = list.length; i console.log(list[i]);
}
The above code has a processing, which is to cache the length of the array through l = list.length.
Although length is a property of the array, there is a performance overhead in accessing it in each loop.
Maybe the latest JavaScript engines have been optimized in this regard, but we cannot guarantee whether our code will run on these latest engines.
In fact, the way without caching the array length is much slower than the cached version.
length attribute The getter method of the
length attribute will simply return the length of the array, while the setter method will truncate the array.
var foo = [1, 2, 3, 4, 5, 6];
foo.length = 3;
foo; // [1, 2, 3]
foo .length = 6;
foo; // [1, 2, 3]
Translator’s Note:
View the value of foo in Firebug at this time: [1, 2 , 3, undefined, undefined, undefined]
But this result is not accurate. If you view the result of foo in Chrome's console, you will find that it is like this: [1, 2, 3]
Because in In JavaScript, undefined is a variable. Note that a variable is not a keyword, so the meanings of the above two results are completely different.
// Translator's Note: In order to verify, let's execute the following code to see whether the serial number 5 exists in foo.
5 in foo; // Returns false whether in Firebug or Chrome
foo[5] = undefined;
5 in foo; // Returns true whether in Firebug or Chrome
is set to length A smaller value will truncate the array, but increasing the length property has no effect on the array.
Conclusion
For better performance, it is recommended to use a normal for loop and cache the length property of the array.
Using for in to iterate over an array is considered bad coding practice and tends to generate errors and cause performance issues.
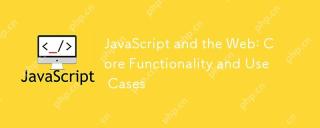
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
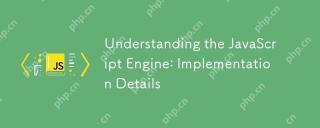
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
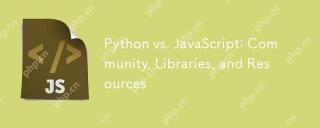
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
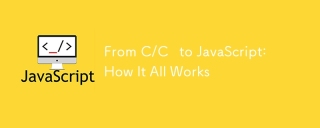
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
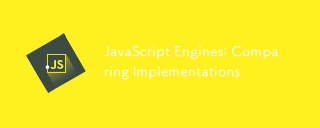
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
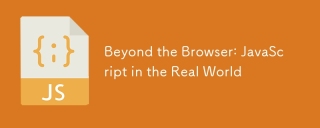
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
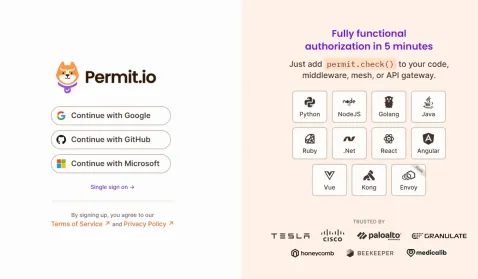
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
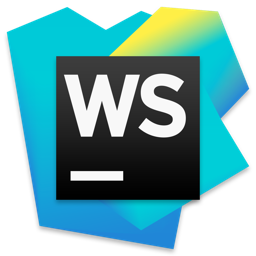
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor