


Ext JS 4 official document three - Overview and practice of class system_Basic knowledge
Ext JS 4 restructures the class system from the bottom up. This is the first major refactoring of the class system in the history of Ext JS. The new architecture is applied to almost every Ext JS 4 class, so it is very important that you have a certain understanding of it before you start coding.
This manual is intended for any developer who wants to create new classes or extend existing classes in Ext JS 4. It is divided into 4 parts:
Part 1: "Overview" -- explains the steps to create a robust class system Necessity
Part 2: "Naming Conventions" -- discusses the best naming conventions for classes, methods, properties, variables, and files
Part 3: "Practice" -- provides detailed step-by-step instructions Code Examples
Part 4: "Error Handling and Debugging" -- Provides very useful tips and tricks on how to handle exceptions
1. Overview
Ext JS 4 has over 300 classes, and to date we have a large community of over 200,000 developers from all over the world and from a variety of programming backgrounds. For a framework of this scale, we face a huge challenge to provide a common code architecture:
Friendly and easy to learn
Fast to develop, easy to debug, simple to deploy
Well organized and scalable And maintainable
JavaScript is an untyped, prototype-oriented language, and one of the most powerful features of this language is flexibility. It can accomplish the same job in a variety of different ways, using a variety of coding styles and techniques. However, this feature comes with an unforeseen cost. Without a unified structure, JavaScript code is difficult to understand, maintain, and reuse.
Class-based programming, in other words, using the most popular OOP model. Class-based languages are usually strongly typed, provide encapsulation, and have standard coding conventions. In general, when developers follow a unified set of coding rules, the code they write is more likely to be predictable, scalable, and extensible. However, they do not have the same dynamic capabilities as languages like JavaScript.
Each method has their own advantages and disadvantages, but can we take advantage of the advantages of both and hide the disadvantages? The answer is yes, we have implemented this solution in Ext JS 4.
2. Naming Conventions
Always use consistent naming conventions based on classes, namespaces and file names in your code. This will help keep your code easy to read. Organized, structured and readable.
1) Classes
Class names can contain only alphanumeric characters, numbers are not allowed in most cases unless they belong to a technical term. Do not use underscores, hyphens, or any other non-alphanumeric characters. For example:
MyCompany.useful_util.Debug_Toolbar is not allowed
MyCompany.util.Base64 is OK
The class name should be placed into the appropriate namespace by using the dot expression (.) attribute of the object middle. At a minimum, class names should have a unique top-level namespace. For example:
MyCompany.data.CoolProxy
MyCompany.Application
Both the top-level namespace and class names should use camel case. In addition, everything else should be all lowercase. For example:
MyCompany.form.action.AutoLoad
Classes not published by Sencha's Ext JS cannot use Ext as the top-level namespace.
Abbreviations should also follow the camel case naming convention mentioned above. For example:
Ext.data.JsonProxy replaces Ext.data.JSONProxy
MyCompany.util.HtmlParser replaces MyCompary.parser.HTMLParser
MyCompany.server.Http replaces MyCompany.server.HTTP
2) Source file
The names of classes are directly mapped to the file paths where they are stored. Therefore, each file can only have one class, for example:
Ext.util.Observable is stored in / to/src/Ext/util/Observable.js
Ext.form.action.Submit is stored in /to/src/Ext/form/action/Submit.js
MyCompany.chart.axis.Numeric is stored in / to/src/MyCompany/chart/axis/Numeric.js
The path /to/src is the root directory of your application's classes, and all classes should be placed in this common root directory.
3) Methods and variables
Similar to class names, method and variable names can only contain alphanumeric characters, numbers are not allowed in most cases unless they belong to a technical term . Do not use underscores, hyphens, or any other non-alphanumeric characters.
Method and variable names should always be camelCase as well, this also applies to abbreviations.
Example:
Acceptable method names: encodeUsingMd5(), getHtml() instead of getHTML(), getJsonResponse() instead of getJSONResponse(), parseXmlContent() instead of parseXMLContent()
Acceptable Variable names: var isGoodName, var base64Encoder, var xmlReader, var httpServer
4) Attribute
The class attribute name completely follows the same naming convention as the above methods and variables, except for static constants.
Static class attributes, i.e. constants, should be all capitalized, for example:
Ext.MessageBox.YES = "Yes"
Ext.MessageBox.NO = "No"
MyCompany.alien.Math.PI = " 4.13"
3. Practice
1. Declaration
1.1) Old method
If you have used any previous version of Ext JS, you must be familiar with using Ext.extend to create a class:
var MyWindow = Ext.extend(Object, { ... });
This method makes it easy to create a new class that inherits from other classes , however other than direct inheritance, we don't have a good API for creating other aspects of classes such as configuration, static configuration, and mixin classes, which we will review in detail later.
Let's look at another example:
My.cool.Window = Ext.extend(Ext.Window, { ... });
In this example, we want to create a new one with Namespace class and let it inherit Ext.Window. There are two problems that need to be solved here:
My.cool must be an existing namespace object so that we can assign Window as its property
Ext.Window Must exist and be loaded so that it can be referenced
The first point is usually solved with Ext.namespace (alias is Ext.ns). This method recursively creates non-existing objects, and the annoying thing is that you always have to Just remember to add them before Ext.extend:
Ext.ns('My.cool');My.cool.Window = Ext.extend(Ext.Window, { ... });
However The second problem is not easy to solve, because Ext.Window may depend on many other classes. It may directly or indirectly inherit from those dependent classes, and these dependent classes may depend on other classes. For this reason, applications written before Ext JS 4 usually import the entire library file ext-all.js, although only a small part of it may be required.
1.2) New method
Ext JS 4 eliminates all these shortcomings, you just need to remember that the only way to create a class is: Ext.define, its basic syntax is as follows:
Ext.define( className, members, onClassCreated);
className: Class name
members is a large object, representing a collection of class members, a series of key-value pairs
onClassCreated is an optional callback function , is called when all dependencies of the class are ready and the class is completely created. This callback function is useful in many situations, which we will discuss in depth in Part 4.
Example:
Ext.define('My. sample.Person',{
name: 'Unknown',
constructor: function(name) { >eat: function(foodType) {
alert(this.name " is eating: " foodType); }});
var aaron = Ext.create('My.sample.Person', 'Aaron') ;
aaron.eat("Salad"); // alert("Aaron is eating: Salad");
Note that we created a My using the Ext.create() method Instance of .sample.Person. Of course we can also use the new keyword (new My.sample.Person()), however we recommend that you develop the habit of always using Ext.create as it can take advantage of the dynamic loading feature. For more information about dynamic loading, see the Ext JS 4 Getting Started Guide.
In Ext JS 4, we introduced a dedicated config attribute, which will be used by the powerful preprocessor class Ext.Class before the class is created. Processing has the following characteristics:
Configuration is completely encapsulated from other class members The getter and setter methods of each config attribute will be automatically generated in the class prototype, if these methods are not defined in the class At the same time, an apply method will be generated for each config attribute, and the automatically generated setter method will call this apply method before setting the value internally. If you want to run custom logic before setting the value, you can override the apply method. If apply does not return a value, the setter method will not set the value. Let’s look at the applyTitle method below:
The following example defines a new class:
Copy the code
Ext.define('My.own.Window', { /**@readonly*/
isWindow: true,
config: {
title: 'Title Here',
bottomBar: {
enabled: true,
height: 50,
resizable: false } },
constructor: function(config) {
this.initConfig(config); },
applyTitle: function(title) {
if (!Ext.isString(title) || title.length === 0) {
alert('Error: Title must be a valid non-empty string');
} else {
return title;
} },
applyBottomBar: function(bottomBar) {
if (bottomBar && bottomBar.enabled) {
if (!this.bottomBar) {
return Ext.create('My.own.WindowBottomBar', bottomBar);
} else {
this.bottomBar.setConfig(bottomBar);
}
}
}});
下面是如何使用这个新类的例子:
var myWindow = Ext.create('My.own.Window', {
title: 'Hello World',
bottomBar: {
height: 60 }});
alert(myWindow.getTitle()); // alerts "Hello World"
myWindow.setTitle('Something New');
alert(myWindow.getTitle()); // alerts "Something New"
myWindow.setTitle(null); // alerts "Error: Title must be a valid non-empty string"
myWindow.setBottomBar({ height: 100 }); // Bottom bar's height is changed to 100
3. 静态配置
静态配置成员可以使用statics属性来定义:
Ext.define('Computer', {
statics: {
instanceCount: 0,
factory: function(brand) {
// 'this' in static methods refer to the class itself
return new this({brand: brand}); } },
config: {
brand: null},
constructor: function(config) {
this.initConfig(config);
// the 'self' property of an instance refers to its class
this.self.instanceCount ; }});
var dellComputer = Computer.factory('Dell');var appleComputer = Computer.factory('Mac');
alert(appleComputer.getBrand());
// using the auto-generated getter to get the value of a config property. Alerts "Mac"
alert(Computer.instanceCount);
// Alerts "2"
四. 错误处理和调试
Ext JS 4包含了一些有用的特性,可以帮助你调试和错误处理:
你可以使用Ext.getDisplayName()方法来获取任何方法的显示名称,这是特别有用的,当抛出错误时,可以用来在错误描述里显示类名和方法名:
throw new Error('[' Ext.getDisplayName(arguments.callee) '] Some message here');
当错误从由Ext.define()定义的类的任何方法中抛出时,如果你使用的基于WebKit的浏览器(Chrome或者Safari)的话,你会在调用堆栈中看到方法名和类名。例如,下面是从Chrome中看到的堆栈信息:
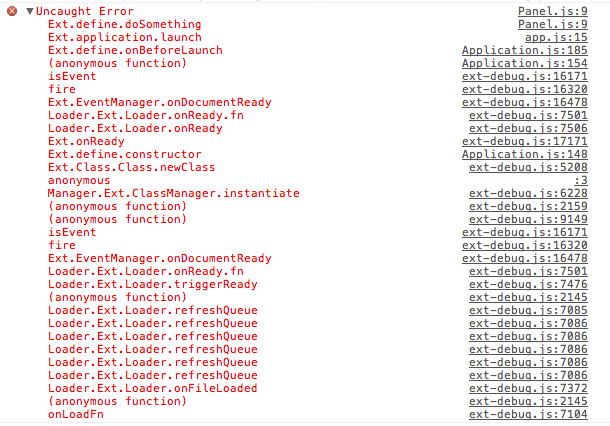
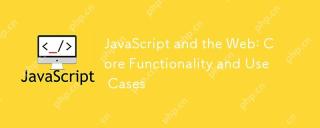
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
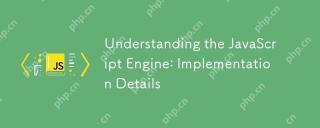
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
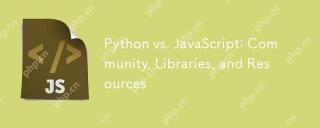
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
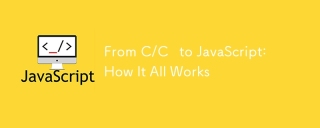
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
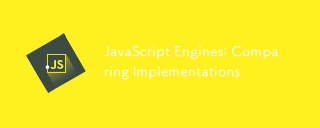
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
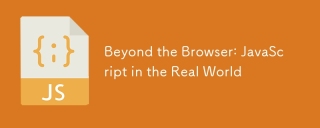
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
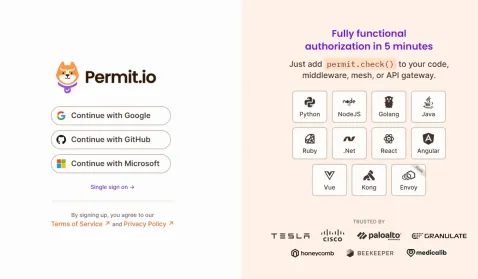
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool