Clever 1: Function
Function is a rare talent in JavaScript code.
♥ It can place code segments and encapsulate relatively independent functions.
♥ It can also implement classes and inject OOP ideas.
jQuery is a function, you can also think of it as a class (haha, it is a class in itself).
(function(){
var jQuery = function() {
// Function body
}
window.jQuery = window.$ = jQuery;
})();
console.log(jQuery);
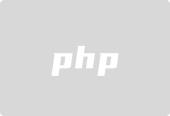
The empty function above is the so-called constructor. The constructor is a basic method of a class in object-oriented languages.
Clever 2: Extended Prototype
What is a prototype object? I will give you a blog post that you can read about
http://www.jb51.net/article/32857.htm.
JavaScript binds a prototype attribute to all functions, and this attribute points to a prototype object. We define the inherited properties and methods of the class in the prototype object.
The prototype object is the basic mechanism for JavaScript to implement inheritance.
(function(){
var jQuery = function () {
// Function body
}
jQuery.fn = jQuery.prototype = {
// Extended prototype object
jquery: "1.8.3",
test: function() {
console.log('test');
}
}
window.jQuery = window.$ = jQuery;
})();
(new jQuery()).test();
Clever 3: Use the factory method to create an instance
The above method must be called using the following method, so A lot of objects will be generated, thus wasting memory consumption.
(new jQuery()).test();
The jQuery source code uses a very soft method, which is also a familiar factory method, for calling.
(function(){
var jQuery = function () {
// Function body
return jQuery.fn.init();
}
jQuery.fn = jQuery.prototype = {
// Extended prototype object
jquery : "1.8.3",
init: function() {
return this;
},
test: function() {
console.log('test');
}
}
window.jQuery = window.$ = jQuery;
})();
jQuery().test();
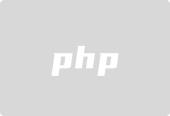
Imagination 1: Let the jQuery function body directly return the object - I use this
(function(){
var jQuery = function() {
return this;
}
jQuery.fn = jQuery.prototype = {
//Extended prototype object
jquery: "1.8.3",
test: function() {
console.log('test');
}
}
window.jQuery = window.$ = jQuery;
})();
console.log(jQuery());
Output Result
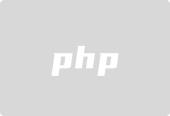
Found that this here points to the Window object.
Imagination 2: Let the jQuery function body directly return an instance of the class.
(function(){
var jQuery = function() {
return new jQuery();
}
jQuery.fn = jQuery.prototype = {
// Extended prototype object
jquery: "1.8.3",
test: function() {
console.log('test');
}
}
window.jQuery = window.$ = jQuery;
})();
console.log(jQuery());
Output results
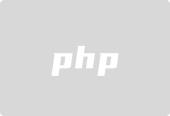
I found that the above is a recursive infinite loop and a memory overflow occurred.
Clever 4: Separate scopes
Thinking 1: What is the this scope returned by the init() method?
(function(){
var jQuery = function() {
// Function body
return jQuery.fn.init();
}
jQuery.fn = jQuery.prototype = {
// Extended prototype object
jquery: "1.8.3",
init: function() {
this.init_jquery = '2.0';
return this ;
}
}
window.jQuery = window.$ = jQuery;
})();
console.log(jQuery().jquery);
console.log (jQuery().init_jquery);
Output result
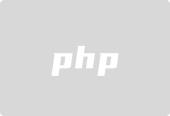
This scope in the init() method: the this keyword refers to the init() function The object where the scope is located can also access the function of the upper-level object jQuery.fn object. ——This kind of thinking will destroy the independence of scope, which is likely to have a negative impact on the jQuery framework.
Thought 2: How to separate this in init() from the jQuery.fn object? ——Instantiate the init initialization type.
(function(){
var jQuery = function () {
// Function body
return new jQuery.fn.init();
}
jQuery.fn = jQuery.prototype = {
// Extended prototype object
jquery: "1.8.3",
init: function() {
this.init_jquery = '2.0';
return this;
}
}
window.jQuery = window .$ = jQuery;
})();
console.log(jQuery().jquery);
console.log(jQuery().init_jquery);
The output result
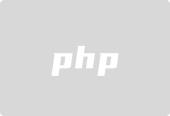
is initialized by instantiating the init() type, limiting this in the init() method, only active within the init() function, and preventing it from going out of scope.
Ingenious 5: Prototype passing
Thinking 1: In Ingenious 4, we separate this in init() from the jquery.fn object. So how can we ensure "Smart 4" and still access the jQuery prototype object? ——Prototype transfer.
Let jQuery’s prototype object override the prototype object of the init() constructor.
jQuery.fn.init.prototype = jQuery.fn;
All codes:
(function(){
var jQuery = function() {
// Function body
return new jQuery.fn.init();
}
jQuery.fn = jQuery.prototype = {
// Extended prototype object
jquery: "1.8.3",
init: function() {
this.init_jquery = '2.0';
return this;
}
}
jQuery.fn.init.prototype = jQuery.fn;
window.jQuery = window.$ = jQuery;
})();
console.log(jQuery() .jquery);
console.log(jQuery().init_jquery);
Output result
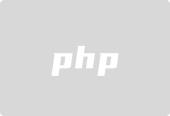
Miaoqi
Point the prototype pointer of the init() object to jQuery.fn. ——In this way, this in init() inherits the methods and properties defined by the jQuery.fn prototype object.
Summary Thank you bloggers for your messages, especially puni, who introduced me to a good book. It would be great if you could add some more.