MySQL BLOBs are ideal for storing binary data like images or audio files. 1) They come in four types: TINYBLOB, BLOB, MEDIUMBLOB, and LONGBLOB, each with different size limits. 2) Use them carefully as they can impact database performance and storage. 3) Consider pagination and lazy loading for efficient data retrieval. 4) For security, encrypt sensitive data before storing in BLOBs. 5) Apply best practices like indexing and compression to optimize performance and storage.
When it comes to handling binary data in MySQL, the BLOB (Binary Large OBject) data type is a powerful tool. But why should you care about BLOBs? Well, if you've ever needed to store images, audio files, or any other large binary files in your database, you'll quickly realize that BLOBs are your best friend. They offer flexibility and efficiency in managing such data, but they also come with their own set of challenges and considerations. Let's dive deep into the world of MySQL BLOB data types and explore how you can leverage them effectively in your projects.
So, what exactly is a BLOB in MySQL? Simply put, a BLOB is a data type that can store binary data up to a certain size limit. MySQL offers four types of BLOBs, each with a different maximum storage capacity: TINYBLOB, BLOB, MEDIUMBLOB, and LONGBLOB. The choice of which BLOB to use depends on the size of the data you're working with. For instance, if you're dealing with small files, a TINYBLOB might suffice, whereas for larger files like high-resolution images or video clips, you'd want to go with a LONGBLOB.
Let's get our hands dirty with some code to see how this works. Here's an example of how you might insert an image into a MySQL database using a BLOB:
-- Create a table with a BLOB column CREATE TABLE images ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), image BLOB ); -- Insert an image into the table INSERT INTO images (name, image) VALUES ('example_image', LOAD_FILE('/path/to/your/image.jpg'));
Now, while this might seem straightforward, there are some nuances to consider. For one, storing large files directly in the database can significantly impact performance. The more data you store, the longer queries will take to execute, and the larger your database will grow. This can lead to slower backups, longer recovery times, and increased storage costs. So, before you decide to use BLOBs, weigh the pros and cons carefully.
Another thing to keep in mind is data retrieval. When you need to fetch a BLOB from the database, you're dealing with potentially large amounts of data. Here's how you might retrieve an image from our images
table:
-- Retrieve an image from the table SELECT image FROM images WHERE name = 'example_image';
But what if you're dealing with thousands of images? Fetching them all at once could be a nightmare. This is where pagination and lazy loading come into play. Instead of pulling all the data at once, you can fetch it in smaller chunks, which is much kinder to your database's performance.
Now, let's talk about some advanced use cases. Suppose you're building a web application where users can upload and view images. You might want to store the images in BLOBs and then serve them directly from the database. Here's a simple PHP script to demonstrate this:
<?php $servername = "localhost"; $username = "your_username"; $password = "your_password"; $dbname = "your_database"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Retrieve the image $stmt = $conn->prepare("SELECT image FROM images WHERE name = ?"); $stmt->bind_param("s", $_GET['name']); $stmt->execute(); $stmt->bind_result($image_data); $stmt->fetch(); $stmt->close(); // Set the content type header - in this case image/jpeg header("Content-Type: image/jpeg"); // Output the image echo $image_data; $conn->close(); ?>
This script retrieves an image from the database and sends it directly to the browser. But be warned, serving large files this way can be resource-intensive. You might want to consider using a Content Delivery Network (CDN) or caching mechanisms to improve performance.
What about security? Storing sensitive data in BLOBs can be risky. If your database gets compromised, attackers could potentially access your binary data. To mitigate this, always encrypt sensitive data before storing it in BLOBs. Here's a quick example of how you might encrypt and decrypt data using AES encryption in MySQL:
-- Encrypt data before inserting into the BLOB INSERT INTO secure_images (name, image) VALUES ('secure_image', AES_ENCRYPT(LOAD_FILE('/path/to/your/secure_image.jpg'), 'your_secret_key')); -- Decrypt data when retrieving from the BLOB SELECT AES_DECRYPT(image, 'your_secret_key') AS decrypted_image FROM secure_images WHERE name = 'secure_image';
Encryption adds an extra layer of security, but it also increases the computational overhead. You'll need to find a balance between security and performance that suits your specific use case.
Finally, let's touch on some best practices. Always index your BLOB columns if you plan to search them frequently. This can help speed up queries, but remember that indexing large BLOBs can be resource-intensive. Also, consider using compression to reduce the size of your BLOBs. MySQL supports compression through the COMPRESS
and UNCOMPRESS
functions, which can be a game-changer for storage efficiency.
-- Compress data before inserting into the BLOB INSERT INTO compressed_images (name, image) VALUES ('compressed_image', COMPRESS(LOAD_FILE('/path/to/your/compressed_image.jpg'))); -- Uncompress data when retrieving from the BLOB SELECT UNCOMPRESS(image) AS uncompressed_image FROM compressed_images WHERE name = 'compressed_image';
In conclusion, MySQL BLOBs are a versatile tool for handling binary data, but they require careful consideration and management. From performance impacts to security concerns, there's a lot to think about. By understanding the nuances and applying best practices, you can harness the power of BLOBs to build more robust and efficient applications. Happy coding!
The above is the detailed content of MySQL BLOB Data Type: A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!
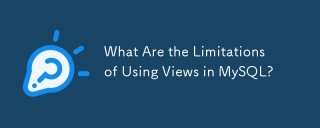
MySQLviewshavelimitations:1)Theydon'tsupportallSQLoperations,restrictingdatamanipulationthroughviewswithjoinsorsubqueries.2)Theycanimpactperformance,especiallywithcomplexqueriesorlargedatasets.3)Viewsdon'tstoredata,potentiallyleadingtooutdatedinforma
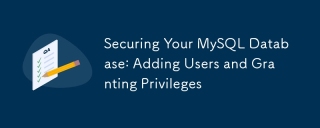
ProperusermanagementinMySQLiscrucialforenhancingsecurityandensuringefficientdatabaseoperation.1)UseCREATEUSERtoaddusers,specifyingconnectionsourcewith@'localhost'or@'%'.2)GrantspecificprivilegeswithGRANT,usingleastprivilegeprincipletominimizerisks.3)
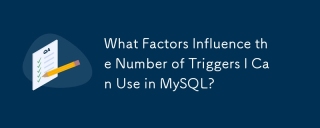
MySQLdoesn'timposeahardlimitontriggers,butpracticalfactorsdeterminetheireffectiveuse:1)Serverconfigurationimpactstriggermanagement;2)Complextriggersincreasesystemload;3)Largertablesslowtriggerperformance;4)Highconcurrencycancausetriggercontention;5)M
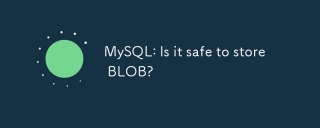
Yes,it'ssafetostoreBLOBdatainMySQL,butconsiderthesefactors:1)StorageSpace:BLOBscanconsumesignificantspace,potentiallyincreasingcostsandslowingperformance.2)Performance:LargerrowsizesduetoBLOBsmayslowdownqueries.3)BackupandRecovery:Theseprocessescanbe
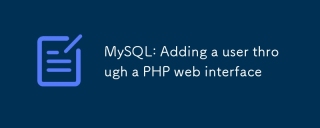
Adding MySQL users through the PHP web interface can use MySQLi extensions. The steps are as follows: 1. Connect to the MySQL database and use the MySQLi extension. 2. Create a user, use the CREATEUSER statement, and use the PASSWORD() function to encrypt the password. 3. Prevent SQL injection and use the mysqli_real_escape_string() function to process user input. 4. Assign permissions to new users and use the GRANT statement.
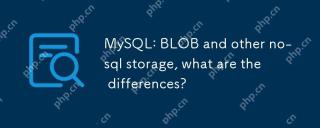
MySQL'sBLOBissuitableforstoringbinarydatawithinarelationaldatabase,whileNoSQLoptionslikeMongoDB,Redis,andCassandraofferflexible,scalablesolutionsforunstructureddata.BLOBissimplerbutcanslowdownperformancewithlargedata;NoSQLprovidesbetterscalabilityand
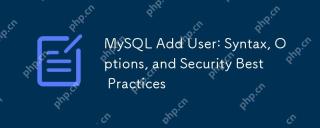
ToaddauserinMySQL,use:CREATEUSER'username'@'host'IDENTIFIEDBY'password';Here'showtodoitsecurely:1)Choosethehostcarefullytocontrolaccess.2)SetresourcelimitswithoptionslikeMAX_QUERIES_PER_HOUR.3)Usestrong,uniquepasswords.4)EnforceSSL/TLSconnectionswith
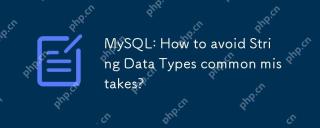
ToavoidcommonmistakeswithstringdatatypesinMySQL,understandstringtypenuances,choosetherighttype,andmanageencodingandcollationsettingseffectively.1)UseCHARforfixed-lengthstrings,VARCHARforvariable-length,andTEXT/BLOBforlargerdata.2)Setcorrectcharacters


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
