The encoding/binary package in Go is essential for handling binary data, offering functions to read and write data in both big-endian and little-endian formats. 1) It's ideal for network protocols, enabling serialization and deserialization of structured data like packet headers and payloads. 2) For file formats, it efficiently processes binary data record by record, suitable for large datasets. 3) It's designed for high performance but requires careful handling of byte order, alignment, and error checking to avoid common pitfalls.
In the world of Go programming, the encoding/binary
package is a powerful tool for handling binary data. It's like a Swiss Army knife for developers who need to read or write binary data, whether it's for network protocols, file formats, or any other scenario where raw bytes are king. Let's dive into some practical examples to see how this package can be a game-changer in your Go projects.
When working with binary data in Go, the encoding/binary
package is your go-to solution. It provides a set of functions that allow you to read and write binary data in a structured way, supporting both big-endian and little-endian byte orders. This flexibility is crucial when dealing with different systems or protocols that might have different byte order preferences.
Here's a quick example to get the ball rolling:
package main import ( "encoding/binary" "fmt" "bytes" ) func main() { // Create a buffer to write to buf := new(bytes.Buffer) // Write a uint16 in little-endian order binary.Write(buf, binary.LittleEndian, uint16(0x1234)) // Read the uint16 back var num uint16 binary.Read(buf, binary.LittleEndian, &num) fmt.Printf("Read back: 0x%x\n", num) }
This simple example demonstrates how you can write a uint16
value to a buffer and then read it back. The binary.Write
and binary.Read
functions are the core of the package, allowing you to work with different data types seamlessly.
Now, let's explore some more practical scenarios where the encoding/binary
package shines.
Imagine you're working on a network protocol where you need to send and receive structured data. You might have a packet format that includes a header with a magic number, a version, and a payload length, followed by the actual payload. Here's how you could use the encoding/binary
package to handle this:
package main import ( "encoding/binary" "fmt" "bytes" ) // Packet represents our network packet structure type Packet struct { MagicNumber uint32 Version uint16 PayloadLen uint16 Payload []byte } func main() { // Create a sample packet packet := Packet{ MagicNumber: 0x12345678, Version: 1, PayloadLen: 10, Payload: []byte("HelloWorld"), } // Create a buffer to write the packet to buf := new(bytes.Buffer) // Write the packet header binary.Write(buf, binary.BigEndian, packet.MagicNumber) binary.Write(buf, binary.BigEndian, packet.Version) binary.Write(buf, binary.BigEndian, packet.PayloadLen) // Write the payload buf.Write(packet.Payload) // Now let's read the packet back var readPacket Packet readPacket.Payload = make([]byte, packet.PayloadLen) // Read the header binary.Read(buf, binary.BigEndian, &readPacket.MagicNumber) binary.Read(buf, binary.BigEndian, &readPacket.Version) binary.Read(buf, binary.BigEndian, &readPacket.PayloadLen) // Read the payload buf.Read(readPacket.Payload) fmt.Printf("Read back: % v\n", readPacket) }
This example shows how you can use the encoding/binary
package to serialize and deserialize a structured packet. The flexibility to choose between big-endian and little-endian byte orders is particularly useful when working with different systems or protocols.
Another practical use case is working with binary file formats. Let's say you need to read a custom binary file format that contains a series of records, each with a timestamp and a value. Here's how you might approach this:
package main import ( "encoding/binary" "fmt" "os" ) // Record represents a single record in our file type Record struct { Timestamp uint64 Value float64 } func main() { // Open the file file, err := os.Open("data.bin") if err != nil { panic(err) } defer file.Close() // Read records until EOF for { var record Record err := binary.Read(file, binary.LittleEndian, &record) if err != nil { break // EOF or error } fmt.Printf("Timestamp: %d, Value: %f\n", record.Timestamp, record.Value) } }
This example demonstrates how you can use the encoding/binary
package to read binary data from a file, processing it record by record. It's a common pattern when working with binary file formats, allowing you to handle large datasets efficiently.
One of the advantages of using the encoding/binary
package is its performance. It's designed to be fast and efficient, making it suitable for high-performance applications. However, there are some potential pitfalls to be aware of:
- Byte Order Mismatches: If you're working with data from different systems, make sure you're using the correct byte order. Mismatches can lead to incorrect data interpretation.
-
Alignment Issues: Some architectures require specific alignment for certain data types. The
encoding/binary
package doesn't handle alignment automatically, so you need to be careful when working with unaligned data. -
Error Handling: Always check the return values of
binary.Read
andbinary.Write
. Errors can occur if you try to read or write beyond the bounds of your buffer.
In terms of best practices, here are some tips to keep in mind:
-
Use Buffers Wisely: When working with large amounts of data, consider using
bytes.Buffer
orbufio.Reader
/bufio.Writer
to improve performance. - Consistent Byte Order: Choose a byte order and stick with it throughout your application to avoid confusion.
- Error Checking: Always check for errors when reading or writing binary data. It's easy to overlook this, but it's crucial for robustness.
In conclusion, the encoding/binary
package in Go is a versatile tool for working with binary data. Whether you're dealing with network protocols, file formats, or any other binary data scenario, it provides the flexibility and performance you need. By understanding its capabilities and following best practices, you can leverage this package to build efficient and reliable applications. Happy coding!
The above is the detailed content of Go encoding/binary package: Practical examples. For more information, please follow other related articles on the PHP Chinese website!
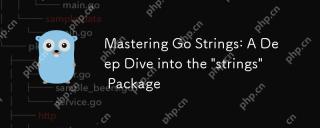
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
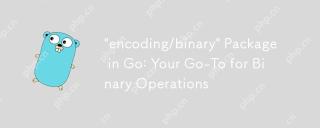
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
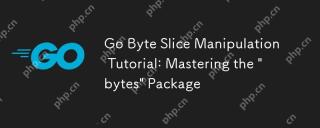
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.
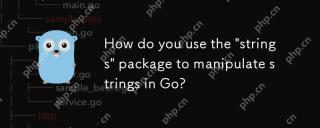
You can use the "strings" package in Go to manipulate strings. 1) Use strings.TrimSpace to remove whitespace characters at both ends of the string. 2) Use strings.Split to split the string into slices according to the specified delimiter. 3) Merge string slices into one string through strings.Join. 4) Use strings.Contains to check whether the string contains a specific substring. 5) Use strings.ReplaceAll to perform global replacement. Pay attention to performance and potential pitfalls when using it.
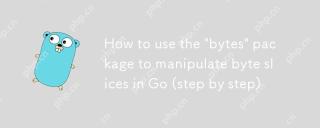
ThebytespackageinGoishighlyeffectiveforbyteslicemanipulation,offeringfunctionsforsearching,splitting,joining,andbuffering.1)Usebytes.Containstosearchforbytesequences.2)bytes.Splithelpsbreakdownbyteslicesusingdelimiters.3)bytes.Joinreconstructsbytesli
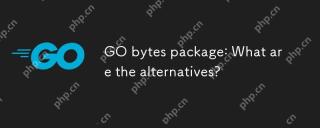
ThealternativestoGo'sbytespackageincludethestringspackage,bufiopackage,andcustomstructs.1)Thestringspackagecanbeusedforbytemanipulationbyconvertingbytestostringsandback.2)Thebufiopackageisidealforhandlinglargestreamsofbytedataefficiently.3)Customstru
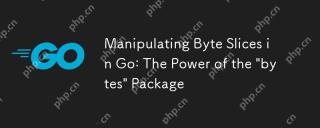
The"bytes"packageinGoisessentialforefficientlymanipulatingbyteslices,crucialforbinarydata,networkprotocols,andfileI/O.ItoffersfunctionslikeIndexforsearching,Bufferforhandlinglargedatasets,Readerforsimulatingstreamreading,andJoinforefficient
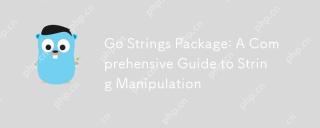
Go'sstringspackageiscrucialforefficientstringmanipulation,offeringtoolslikestrings.Split(),strings.Join(),strings.ReplaceAll(),andstrings.Contains().1)strings.Split()dividesastringintosubstrings;2)strings.Join()combinesslicesintoastring;3)strings.Rep


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
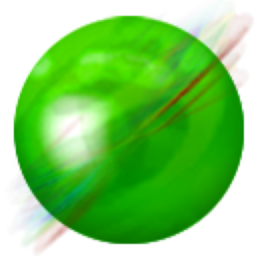
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version
