Streaming BLOBs is indeed better than direct storage because it reduces memory usage and improves performance. 1) By gradually reading and processing files, database bloat and performance degradation are avoided. 2) Streaming requires more complex code logic and may increase the number of I/O operations.
When dealing with large files, using BLOB (Binary Large OBject) type data storage and streaming in MySQL are two common choices. So, is streaming BLOBs really better than storing them directly? There is no simple answer to this question, because it depends on your specific needs and usage scenarios. However, in many cases, streaming does have its advantages.
The main advantage of streaming BLOB is that it can significantly reduce memory usage and improve performance. Suppose you have an application that needs to process a large number of video files, and if you choose to store these files directly in the database, it may cause the database to bloat and performance degradation. Instead, streaming allows you to read and process files step by step without loading the entire file into memory, which is especially important for large files.
For example, I have processed a large number of pictures uploaded by users in a project. Initially, we stored these images directly in the BLOB field of the MySQL database, but we quickly encountered performance issues. Database query speeds become very slow, and server memory is often filled. After switching to streaming, we were able to process these images without affecting database performance, and the user experience was significantly improved.
Of course, streaming also has some potential challenges and points to be paid attention to. First, streaming requires more complex code logic to manage the reading and writing process of data. Second, if your application needs to access these BLOB data frequently, streaming may increase the number of I/O operations, which affects performance.
Here is a simple example showing how to use streaming in MySQL to process BLOB data:
import java.io.InputStream; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; public class BlobStreamingExample { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/your_database"; String user = "your_username"; String password = "your_password"; try (Connection conn = DriverManager.getConnection(url, user, password)) { String sql = "SELECT image_data FROM images WHERE id = ?"; try (PreparedStatement pstmt = conn.prepareStatement(sql)) { pstmt.setInt(1, 1); // Suppose we want to get the image with id 1 try (ResultSet rs = pstmt.executeQuery()) { if (rs.next()) { InputStream inputStream = rs.getBinaryStream("image_data"); // Here you can gradually read and process the data in the inputStream// For example, write data to a file or perform other processing byte[] buffer = new byte[1024]; int bytesRead; while ((bytesRead = inputStream.read(buffer)) != -1) { // Process the read data System.out.println("Read " bytesRead " bytes"); } inputStream.close(); } } } } catch (Exception e) { e.printStackTrace(); } } }
This example shows how to read BLOB data from a database and process it step by step through streaming. In practical applications, you may need to adjust the code according to specific needs, such as writing the read data to a file or performing other processing.
When using streaming, there are also some best practices and performance optimization strategies to consider. For example, try to use buffered streams to reduce the number of I/O operations and avoid frequent opening and closing of streams. In addition, if your application needs to access BLOB data frequently, consider using a caching mechanism to improve performance.
In general, streaming BLOB does have its advantages when processing large files, but it also needs to weigh its advantages and disadvantages based on the specific application scenario. With reasonable design and optimization, you can take advantage of streaming while avoiding its potential pitfalls.
The above is the detailed content of MySQL: Streaming of BLOBS is better than storing them?. For more information, please follow other related articles on the PHP Chinese website!
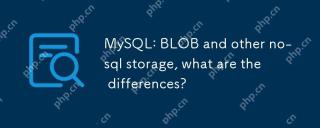
MySQL'sBLOBissuitableforstoringbinarydatawithinarelationaldatabase,whileNoSQLoptionslikeMongoDB,Redis,andCassandraofferflexible,scalablesolutionsforunstructureddata.BLOBissimplerbutcanslowdownperformancewithlargedata;NoSQLprovidesbetterscalabilityand
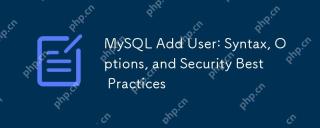
ToaddauserinMySQL,use:CREATEUSER'username'@'host'IDENTIFIEDBY'password';Here'showtodoitsecurely:1)Choosethehostcarefullytocontrolaccess.2)SetresourcelimitswithoptionslikeMAX_QUERIES_PER_HOUR.3)Usestrong,uniquepasswords.4)EnforceSSL/TLSconnectionswith
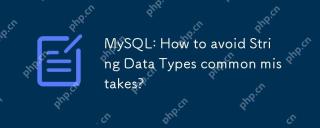
ToavoidcommonmistakeswithstringdatatypesinMySQL,understandstringtypenuances,choosetherighttype,andmanageencodingandcollationsettingseffectively.1)UseCHARforfixed-lengthstrings,VARCHARforvariable-length,andTEXT/BLOBforlargerdata.2)Setcorrectcharacters
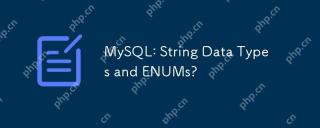
MySQloffersechar, Varchar, text, Anddenumforstringdata.usecharforfixed-Lengthstrings, VarcharerForvariable-Length, text forlarger text, AndenumforenforcingdataAntegritywithaetofvalues.
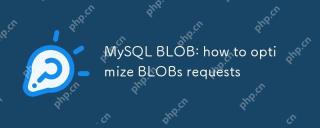
Optimizing MySQLBLOB requests can be done through the following strategies: 1. Reduce the frequency of BLOB query, use independent requests or delay loading; 2. Select the appropriate BLOB type (such as TINYBLOB); 3. Separate the BLOB data into separate tables; 4. Compress the BLOB data at the application layer; 5. Index the BLOB metadata. These methods can effectively improve performance by combining monitoring, caching and data sharding in actual applications.
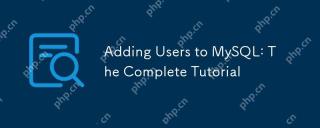
Mastering the method of adding MySQL users is crucial for database administrators and developers because it ensures the security and access control of the database. 1) Create a new user using the CREATEUSER command, 2) Assign permissions through the GRANT command, 3) Use FLUSHPRIVILEGES to ensure permissions take effect, 4) Regularly audit and clean user accounts to maintain performance and security.
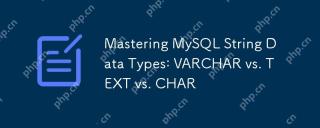
ChooseCHARforfixed-lengthdata,VARCHARforvariable-lengthdata,andTEXTforlargetextfields.1)CHARisefficientforconsistent-lengthdatalikecodes.2)VARCHARsuitsvariable-lengthdatalikenames,balancingflexibilityandperformance.3)TEXTisidealforlargetextslikeartic
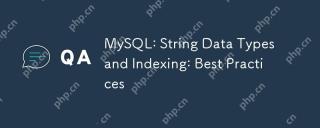
Best practices for handling string data types and indexes in MySQL include: 1) Selecting the appropriate string type, such as CHAR for fixed length, VARCHAR for variable length, and TEXT for large text; 2) Be cautious in indexing, avoid over-indexing, and create indexes for common queries; 3) Use prefix indexes and full-text indexes to optimize long string searches; 4) Regularly monitor and optimize indexes to keep indexes small and efficient. Through these methods, we can balance read and write performance and improve database efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
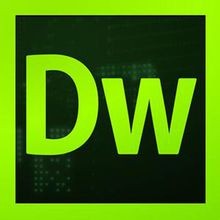
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
