


The Boilerplate Code Required for React Projects: Reducing Setup Overhead
To reduce setup overhead in React projects, use tools like Create React App (CRA), Next.js, Gatsby, or starter kits, and maintain a modular structure. 1) CRA simplifies setup with a single command. 2) Next.js and Gatsby offer more features but a learning curve. 3) Starter kits provide comprehensive setups. 4) A modular structure enhances maintainability and scalability.
In the world of React development, one of the most frequent challenges developers face is managing the boilerplate code that comes with setting up a new project. This overhead can be a significant barrier, especially for newcomers or when working on quick prototypes. So, how can we reduce the setup overhead in React projects?
Let's dive into the world of React and explore various strategies and tools that can help us minimize the boilerplate code, making our development process smoother and more efficient.
When I first started with React, the sheer amount of setup required for even a simple project was overwhelming. I remember spending hours configuring webpack, setting up Babel, and ensuring all dependencies were correctly managed. It's a common pain point, but over time, I've discovered several approaches that drastically cut down on this setup time.
One of the most effective ways to reduce setup overhead is by using Create React App (CRA). CRA is a tool developed by the React team that sets up a new React project with a single command. It handles all the configuration for you, including webpack, Babel, and other essential tools. Here's how you can get started with CRA:
npx create-react-app my-app cd my-app npm start
This simplicity is a game-changer, especially for beginners. However, CRA comes with its own set of limitations. For instance, it's not as flexible as a custom setup, and ejecting from CRA to gain more control can be a complex process. If you need more customization, you might want to look into alternatives like Next.js or Gatsby, which offer more out-of-the-box features while still providing a streamlined setup process.
Next.js, for example, is particularly useful for server-side rendering and static site generation. It comes with built-in routing and a lot of optimizations out of the box. Here's a quick example of how to start a Next.js project:
npx create-next-app my-next-app cd my-next-app npm run dev
Gatsby, on the other hand, is excellent for content-driven sites and offers a rich plugin ecosystem. Starting a Gatsby project is just as straightforward:
npx gatsby new my-gatsby-app cd my-gatsby-app npm run develop
Both Next.js and Gatsby provide more features than CRA but might introduce a learning curve due to their additional capabilities. It's essential to weigh the trade-offs and choose the tool that best fits your project's needs.
Another approach to reducing boilerplate is to use a starter kit or a boilerplate project. There are numerous open-source projects on GitHub that you can clone and use as a starting point. For instance, the react-boilerplate
project offers a more comprehensive setup with Redux, React Router, and other commonly used libraries. Here's how you can use it:
git clone https://github.com/react-boilerplate/react-boilerplate.git my-react-app cd my-react-app npm install npm start
While starter kits can save time, they often come with more code than you might need, which can be overwhelming. It's crucial to understand the components and configurations included in these kits to avoid unnecessary complexity.
In my experience, one of the most overlooked aspects of reducing setup overhead is maintaining a clean and modular project structure. By organizing your code into clear, reusable components and using tools like ESLint and Prettier, you can ensure that your project remains maintainable and easy to navigate. Here's a simple example of a modular component structure:
// src/components/Button.js import React from 'react'; const Button = ({ onClick, children }) => ( <button onClick={onClick}> {children} </button> ); export default Button; // src/components/Header.js import React from 'react'; import Button from './Button'; const Header = ({ onLogin }) => ( <header> <h1 id="My-App">My App</h1> <Button onClick={onLogin}>Login</Button> </header> ); export default Header;
This approach not only reduces the initial setup time but also makes your project more scalable and easier to maintain in the long run.
When it comes to performance optimization, tools like react-fast-refresh
can significantly improve your development experience by providing faster feedback loops. Additionally, using modern React features like hooks can help reduce the amount of boilerplate code you need to write. For example, instead of using class components with lifecycle methods, you can use functional components with hooks:
// Before: Class Component import React, { Component } from 'react'; class Counter extends Component { constructor(props) { super(props); this.state = { count: 0 }; } increment = () => { this.setState({ count: this.state.count 1 }); }; render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.increment}>Increment</button> </div> ); } } // After: Functional Component with Hooks import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); };
In conclusion, reducing setup overhead in React projects is all about finding the right balance between simplicity and customization. Whether you choose to use tools like Create React App, Next.js, or Gatsby, or opt for a starter kit, the key is to understand your project's requirements and choose the approach that best suits your needs. By maintaining a clean, modular structure and leveraging modern React features, you can significantly streamline your development process and focus more on building great applications.
The above is the detailed content of The Boilerplate Code Required for React Projects: Reducing Setup Overhead. For more information, please follow other related articles on the PHP Chinese website!

useState()iscrucialforoptimizingReactappperformanceduetoitsimpactonre-rendersandupdates.Tooptimize:1)UseuseCallbacktomemoizefunctionsandpreventunnecessaryre-renders.2)EmployuseMemoforcachingexpensivecomputations.3)Breakstateintosmallervariablesformor

Use Context and useState to share states because they simplify state management in large React applications. 1) Reduce propdrilling, 2) The code is clearer, 3) It is easier to manage global state. However, pay attention to performance overhead and debugging complexity. The rational use of Context and optimization technology can improve the efficiency and maintainability of the application.

Using incorrect keys can cause performance issues and unexpected behavior in React applications. 1) The key is a unique identifier of the list item, helping React update the virtual DOM efficiently. 2) Using the same or non-unique key will cause list items to be reordered and component states to be lost. 3) Using stable and unique identifiers as keys can optimize performance and avoid full re-rendering. 4) Use tools such as ESLint to verify the correctness of the key. Proper use of keys ensures efficient and reliable React applications.

InReact,keysareessentialforoptimizinglistrenderingperformancebyhelpingReacttrackchangesinlistitems.1)KeysenableefficientDOMupdatesbyidentifyingadded,changed,orremoveditems.2)UsinguniqueidentifierslikedatabaseIDsaskeys,ratherthanindices,preventsissues

useState is often misused in React. 1. Misunderstand the working mechanism of useState: the status will not be updated immediately after setState. 2. Error update status: SetState in function form should be used. 3. Overuse useState: Use props if necessary. 4. Ignore the dependency array of useEffect: the dependency array needs to be updated when the state changes. 5. Performance considerations: Batch updates to states and simplified state structures can improve performance. Correct understanding and use of useState can improve code efficiency and maintainability.

Yes,ReactapplicationscanbeSEO-friendlywithproperstrategies.1)Useserver-siderendering(SSR)withtoolslikeNext.jstogeneratefullHTMLforindexing.2)Implementstaticsitegeneration(SSG)forcontent-heavysitestopre-renderpagesatbuildtime.3)Ensureuniquetitlesandme

React performance bottlenecks are mainly caused by inefficient rendering, unnecessary re-rendering and calculation of component internal heavy weight. 1) Use ReactDevTools to locate slow components and apply React.memo optimization. 2) Optimize useEffect to ensure that it only runs when necessary. 3) Use useMemo and useCallback for memory processing. 4) Split the large component into small components. 5) For big data lists, use virtual scrolling technology to optimize rendering. Through these methods, the performance of React applications can be significantly improved.

Someone might look for alternatives to React because of performance issues, learning curves, or exploring different UI development methods. 1) Vue.js is praised for its ease of integration and mild learning curve, suitable for small and large applications. 2) Angular is developed by Google and is suitable for large applications, with a powerful type system and dependency injection. 3) Svelte provides excellent performance and simplicity by compiling it into efficient JavaScript at build time, but its ecosystem is still growing. When choosing alternatives, they should be determined based on project needs, team experience and project size.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
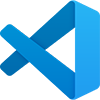
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
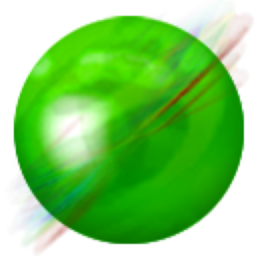
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
