React components go through different stages in their application lifecycle, although what happens behind the scenes may not be obvious.
These stages include:
- Mount
- renew
- uninstall
- Error handling
Each stage has a corresponding method at which specific actions can be performed on components. For example, when fetching data from the network, you might want to call a function that handles API calls in componentDidMount()
method (available in the mount phase).
Understanding different lifecycle approaches is crucial for the development of React applications, as it allows us to trigger operations accurately when needed without being confused with other operations. This article will cover each lifecycle, including the methods available and the types of scenarios we use them.
Mounting phase
Think of mounts as the initial stage of the component life cycle. The component did not exist before the mount occurred - it just flashed through the DOM until the mount occurred and connected it as part of the document.
Once the component is mounted, we can take advantage of many methods: constructor()
, render()
, componentDidMount()
, and static getDerivedStateFromProps()
. Each method has its own purpose, let's take a look in order.
constructor()
constructor()
method is required when setting states directly on the component to bind methods together. It looks like this:
// Once the input component starts mounting... constructor(props) { // ...set some props... super(props); // ...In this case, it is a blank username... this.state = { username: '' }; // ...then bind a method that handles input changes this.handleInputChange = this.handleInputChange.bind(this); }
It is important to know that constructor
is the first method called when creating a component. The component has not been rendered (coming soon), but the DOM already knows it and we can hook it to it before it renders. So this is not where we call setState()
or introduce any side effects, because the component is still in the build phase!
I've written a tutorial on refs before and one thing I noticed is that when using React.createRef()
, you can set ref in constructor
. This is reasonable, because refs is used to change values without props or have to re-render the component with updated values:
constructor(props) { super(props); this.state = { username: '' }; this.inputText = React.createRef(); }
render()
render()
method is where the component's mark is displayed on the front end. The user can see and access it at this time. If you've ever created a React component, you're already familiar with it - even if you don't realize it - because it requires output tags.
class App extends React.Component { // During the mount process, please render the following content! render() { Return ( <div> <p>Hello World!</p> </div> ) } }
But that's not the whole purpose of render()
! It can also be used to render component arrays:
class App extends React.Component { render () { Return [ <h2 id="JavaScript-Tools">JavaScript Tools</h2> , <frontend></frontend>, <backend></backend> ] } }
Even component fragments:
class App extends React.Component { render() { Return ( <react.fragment><p>Hello World!</p></react.fragment> ) } }
We can also use it to render components outside the DOM hierarchy (similar to React Portal):
// We are creating a portal that allows components to move class Portal extends React.Component { // First, we create a div element constructor() { super(); this.el = document.createElement("div"); } // After mount, let's append the child element of the component componentDidMount = () => { portalRoot.appendChild(this.el); }; // If the component is removed from the DOM, then we also remove its child elements componentWillUnmount = () => { portalRoot.removeChild(this.el); }; // Ah, now we can render the component and its child elements render() as needed { const { children } = this.props; return ReactDOM.createPortal(children, this.el); } }
Of course render()
can render numbers and strings...
class App extends React.Component { render () { return "Hello World!" } }
And null or boolean values:
class App extends React.Component { render () { return null } }
componentDidMount()
Does the name componentDidMount()
indicate its meaning? This method is called after the component is mounted (i.e. connected to the DOM). In another tutorial I wrote about getting data in React, this is where you want to make a request to the API to get data.
We can use your fetch method:
fetchUsers() { fetch(`https://jsonplaceholder.typicode.com/users`) .then(response => response.json()) .then(data => this.setState({ users: data, isLoading: false, }) ) .catch(error => this.setState({ error, isLoading: false })); }
Then call the method in the componentDidMount()
hook:
componentDidMount() { this.fetchUsers(); }
We can also add event listeners:
componentDidMount() { el.addEventListener() }
Very concise, right?
static getDerivedStateFromProps()
This is a bit verbose name, but static getDerivedStateFromProps()
is not as complicated as it sounds. It is called before render()
method of the mount phase and before the update phase. It returns an object to update the status of the component, or null
if there is no content to be updated.
To understand how it works, let's implement a counter component that will set a specific value for its counter state. This status will only be updated when the value of maxCount
is higher. maxCount
will be passed from the parent component.
This is the parent component:
class App extends React.Component { constructor(props) { super(props) this.textInput = React.createRef(); this.state = { value: 0 } } handleIncrement = e => { e.preventDefault(); this.setState({ value: this.state.value 1 }) }; handleDecrement = e => { e.preventDefault(); this.setState({ value: this.state.value - 1 }) }; render() { Return ( <react.fragment><p>Max count: { this.state.value }</p> - <counter maxcount="{this.state.value}"></counter></react.fragment> ) } }
We have a button to increase the value of maxCount
, which we pass to Counter
component.
class Counter extends React.Component { state={ Counter: 5 } static getDerivedStateFromProps(nextProps, prevState) { if (prevState.counter <p>Count: {this.state.counter}</p> ) } }
In the Counter
component, we check if counter
is smaller than maxCount
. If so, we set counter
to the value of maxCount
. Otherwise, we do nothing.
Update phase
An update phase occurs when the component's props or state changes. Like mounts, updates also have their own set of available methods, which we will introduce next. That is, it is worth noting that render()
and getDerivedStateFromProps()
will also fire at this stage.
ShouldComponentUpdate()
When the state or props of the component change, we can use shouldComponentUpdate()
method to control whether the component should be updated. This method is called before rendering occurs and when state and props are received. The default behavior is true
. To re-render every time the state or props change, we do this:
shouldComponentUpdate(nextProps, nextState) { return this.state.value !== nextState.value; }
When false
is returned, the component will not be updated, but instead calls render()
method to display the component.
getSnaphotBeforeUpdate()
One thing we can do is capture the state of the component at some point in time, which is what getSnapshotBeforeUpdate()
is designed for. It is called after render()
but before committing any new changes to the DOM. The return value is passed as the third parameter to componentDidUpdate()
.
It takes the previous state and props as parameters:
getSnapshotBeforeUpdate(prevProps, prevState) { // ... }
In my opinion, there are few use cases for this approach. It is a lifecycle method that you may not use often.
componentDidUpdate()
Add componentDidUpdate()
to the method list, where the name roughly says everything. If the component is updated, then we can use this method to hook it at this time and pass it to the component's previous props and state.
componentDidUpdate(prevProps, prevState) { if (prevState.counter !== this.state.counter) { // ... } }
If you have ever used getSnapshotBeforeUpdate()
, you can also pass the return value as a parameter to componentDidUpdate()
:
componentDidUpdate(prevProps, prevState, snapshot) { if (prevState.counter !== this.state.counter) { // .... } }
Uninstallation phase
We almost see the opposite of the mount phase here. As you might expect, the uninstall occurs when the component is cleared from the DOM and is no longer available.
We only have one method here: componentWillUnmount()
This is called before the component is uninstalled and destroyed. This is where we want to perform any necessary cleanup after the component leaves, such as removing event listeners that might be added in componentDidMount()
, or clearing the subscription.
// Delete the event listener componentWillUnmount() { el.removeEventListener() }
Error handling phase
There may be problems in the component, which may cause errors. We've been using error boundaries for a while to help solve this problem. This error boundary component uses some methods to help us deal with possible errors.
getDerivedStateFromError()
We use getDerivedStateFromError()
to catch any errors thrown from the child component, and then we use it to update the state of the component.
class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { return { hasError: true }; } render() { if (this.state.hasError) { Return ( <h1 id="Oops-something-went-wrong">Oops, something went wrong :(</h1> ); } return this.props.children; } }
In this example, when an error is thrown from the child component, the ErrorBoundary
component will show "Oh, some problem has occurred".
componentDidCatch()
While getDerivedStateFromError()
is suitable for updating the state of a component in the event of side effects such as error logging, we should use componentDidCatch()
because it is called during the commit phase, at which time the DOM has been updated.
componentDidCatch(error, info) { // Log errors to service}
Both getDerivedStateFromError()
and componentDidCatch()
can be used in the ErrorBoundary
component:
class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { return { hasError: true }; } componentDidCatch(error, info) { // Log errors to service} render() { if (this.state.hasError) { Return ( <h1 id="Oops-something-went-wrong">Oops, something went wrong :(</h1> ); } return this.props.children; } }
This is the life cycle of React components!
It's a cool thing to understand how React components interact with DOM. It's easy to think that some "magic" will happen, and then something will appear on the page. But the life cycle of React components shows that this madness is orderly, and it aims to give us a lot of control over what happens from the time the component reaches the DOM to the time it disappears.
We cover a lot of things in a relatively short space, but hopefully this gives you a good idea of how React handles components and what capabilities we have at each stage of processing. If you are not clear about anything introduced here, feel free to ask any questions and I'd love to do my best to help!
The above is the detailed content of The Circle of a React Lifecycle. For more information, please follow other related articles on the PHP Chinese website!
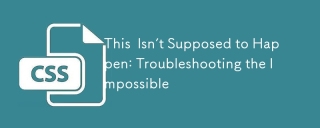
What it looks like to troubleshoot one of those impossible issues that turns out to be something totally else you never thought of.
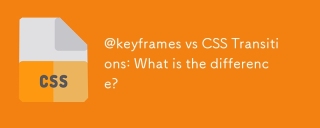
@keyframesandCSSTransitionsdifferincomplexity:@keyframesallowsfordetailedanimationsequences,whileCSSTransitionshandlesimplestatechanges.UseCSSTransitionsforhovereffectslikebuttoncolorchanges,and@keyframesforintricateanimationslikerotatingspinners.
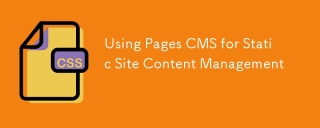
I know, I know: there are a ton of content management system options available, and while I've tested several, none have really been the one, y'know? Weird pricing models, difficult customization, some even end up becoming a whole &
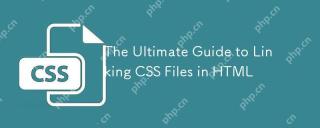
Linking CSS files to HTML can be achieved by using elements in part of HTML. 1) Use tags to link local CSS files. 2) Multiple CSS files can be implemented by adding multiple tags. 3) External CSS files use absolute URL links, such as. 4) Ensure the correct use of file paths and CSS file loading order, and optimize performance can use CSS preprocessor to merge files.
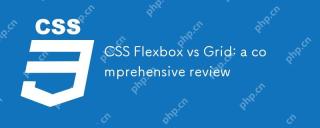
Choosing Flexbox or Grid depends on the layout requirements: 1) Flexbox is suitable for one-dimensional layouts, such as navigation bar; 2) Grid is suitable for two-dimensional layouts, such as magazine layouts. The two can be used in the project to improve the layout effect.
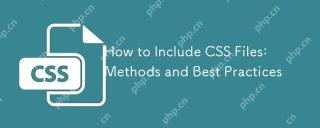
The best way to include CSS files is to use tags to introduce external CSS files in the HTML part. 1. Use tags to introduce external CSS files, such as. 2. For small adjustments, inline CSS can be used, but should be used with caution. 3. Large projects can use CSS preprocessors such as Sass or Less to import other CSS files through @import. 4. For performance, CSS files should be merged and CDN should be used, and compressed using tools such as CSSNano.
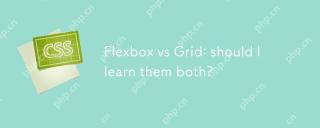
Yes,youshouldlearnbothFlexboxandGrid.1)Flexboxisidealforone-dimensional,flexiblelayoutslikenavigationmenus.2)Gridexcelsintwo-dimensional,complexdesignssuchasmagazinelayouts.3)Combiningbothenhanceslayoutflexibilityandresponsiveness,allowingforstructur
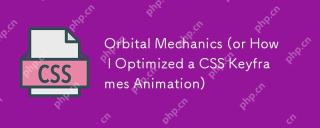
What does it look like to refactor your own code? John Rhea picks apart an old CSS animation he wrote and walks through the thought process of optimizing it.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
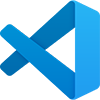
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
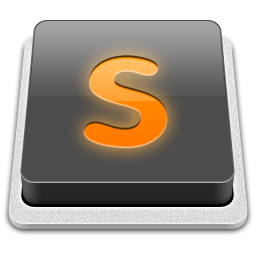
SublimeText3 Mac version
God-level code editing software (SublimeText3)
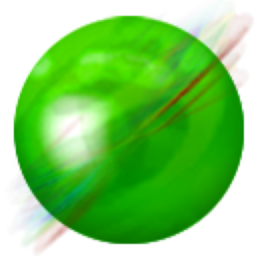
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
