Python's Duck Typing: A Flexible Approach to Object-Oriented Programming
Imagine a world where an object's type is secondary to its functionality. This is the essence of duck typing—a powerful paradigm in Python that emphasizes what an object can do, not what it is. This article explores how duck typing enhances Python's object-oriented programming, leading to more readable and adaptable code.
Key Concepts:
- Grasp the core principles of duck typing and its importance in Python.
- Learn practical implementations of duck typing with illustrative examples.
- Understand the advantages of duck typing for creating flexible and maintainable code.
- Recognize potential drawbacks and best practices for effective duck typing.
- Apply duck typing techniques to real-world scenarios for improved code adaptability.
Table of Contents:
- Introduction
- Understanding Duck Typing
- Duck Typing vs. Static Typing
- Advantages of Duck Typing
- Practical Duck Typing Examples
- Error Handling in Duck Typing
- Real-World Applications
- Conclusion
- Frequently Asked Questions
What is Duck Typing?
In duck typing, an object's suitability is determined not by its class but by the methods and attributes it possesses. The idiom "If it walks like a duck and quacks like a duck, then it must be a duck" perfectly encapsulates this concept. If an object exhibits the necessary behavior, it can be used as if it were of a specific type.
Duck Typing vs. Static Typing
Statically-typed languages (like Java or C ) require type declarations at compile time. This ensures type safety but can lead to less flexible and more verbose code. For example, in Java:
List<string> list = new ArrayList(); list.add("Hello");</string>
Python, on the other hand, uses dynamic typing, where type checking happens at runtime. Duck typing takes this further by omitting type checks altogether, focusing solely on the availability of methods:
def add_to_list(obj, item): obj.append(item) my_list = [1, 2, 3] add_to_list(my_list, 4)
add_to_list
functions correctly with any object possessing an append
method, not just lists.
Benefits of Duck Typing
- Flexibility: Duck typing promotes reusable and adaptable code. Any object with the required methods can be passed to a function.
- Simplicity: Eliminates explicit type declarations and interfaces, simplifying code.
- Polymorphism: Different object types can be used interchangeably if they share the same behavior.
- Refactoring Ease: Changing an object's type is straightforward as long as the necessary methods remain.
Examples of Duck Typing
Let's illustrate duck typing with practical examples.
Example 1: Calculating Area
A function to calculate a shape's area only needs to know if the shape object has an area()
method:
class Circle: def __init__(self, radius): self.radius = radius def area(self): return 3.14 * self.radius**2 class Square: def __init__(self, side): self.side = side def area(self): return self.side**2 def print_area(shape): print(f"The area is {shape.area()}") circle = Circle(5) square = Square(4) print_area(circle) print_area(square)
Output:
<code>The area is 78.5 The area is 16</code>
Example 2: Iterating Through Collections
A function to print collection items works with lists, tuples, and sets because they all support iteration:
def print_items(collection): for item in collection: print(item) my_list = [1, 2, 3] my_tuple = (4, 5, 6) my_set = {7, 8, 9} print_items(my_list) print_items(my_tuple) print_items(my_set)
Output:
<code>1 2 3 4 5 6 7 8 9</code>
Handling Errors with Duck Typing
The absence of a required method can lead to runtime errors. Exception handling mitigates this:
def safe_append(obj, item): try: obj.append(item) except AttributeError: print(f"Object {obj} lacks the append method.") my_list = [1, 2, 3] my_string = "hello" safe_append(my_list, 4) safe_append(my_string, 'a')
Output:
<code>Object hello lacks the append method.</code>
Duck Typing in Practice
Many Python libraries utilize duck typing. For instance, the json
module handles JSON serialization flexibly.
Conclusion
Duck typing is a flexible and powerful approach in Python's object-oriented programming. By prioritizing behavior over strict type adherence, it results in cleaner, more maintainable, and adaptable code.
Frequently Asked Questions
Q1: What is duck typing in Python? A: It's a dynamic typing style where an object's suitability is determined by its methods and attributes, not its type.
Q2: How does it differ from static typing? A: Static typing verifies types at compile time; duck typing checks for methods at runtime.
Q3: Why "duck typing"? A: It's derived from the saying: "If it walks like a duck and quacks like a duck, it must be a duck."
The above is the detailed content of Duck Typing in Python. For more information, please follow other related articles on the PHP Chinese website!
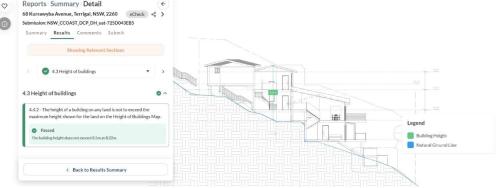
AI Streamlines Wildfire Recovery Permitting Australian tech firm Archistar's AI software, utilizing machine learning and computer vision, automates the assessment of building plans for compliance with local regulations. This pre-validation significan
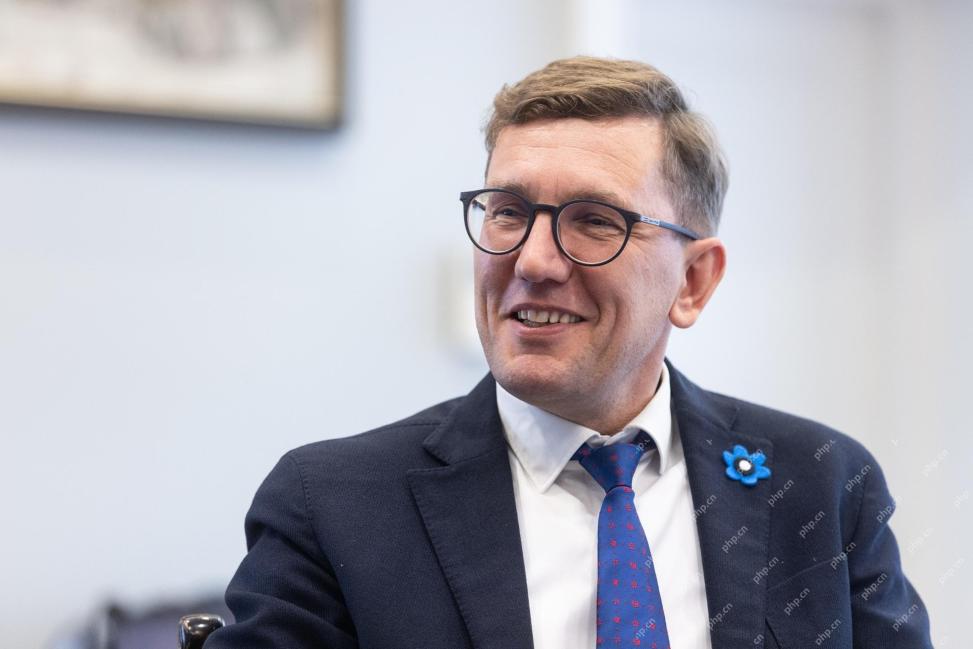
Estonia's Digital Government: A Model for the US? The US struggles with bureaucratic inefficiencies, but Estonia offers a compelling alternative. This small nation boasts a nearly 100% digitized, citizen-centric government powered by AI. This isn't

Planning a wedding is a monumental task, often overwhelming even the most organized couples. This article, part of an ongoing Forbes series on AI's impact (see link here), explores how generative AI can revolutionize wedding planning. The Wedding Pl
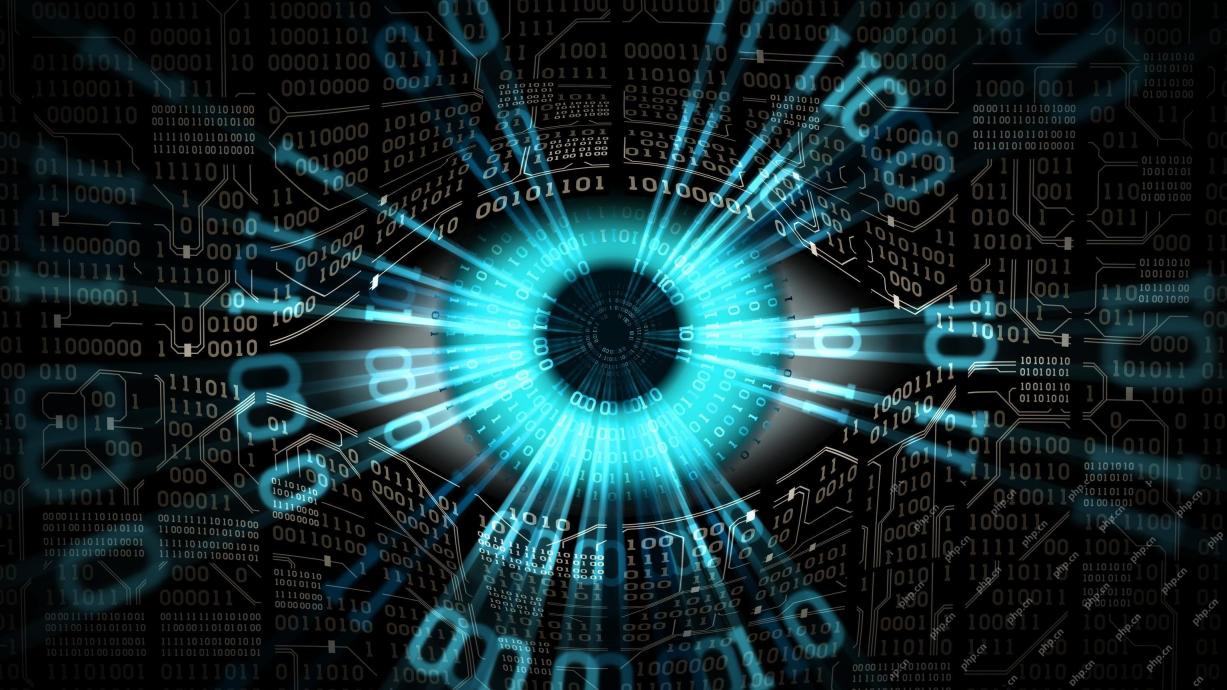
Businesses increasingly leverage AI agents for sales, while governments utilize them for various established tasks. However, consumer advocates highlight the need for individuals to possess their own AI agents as a defense against the often-targeted
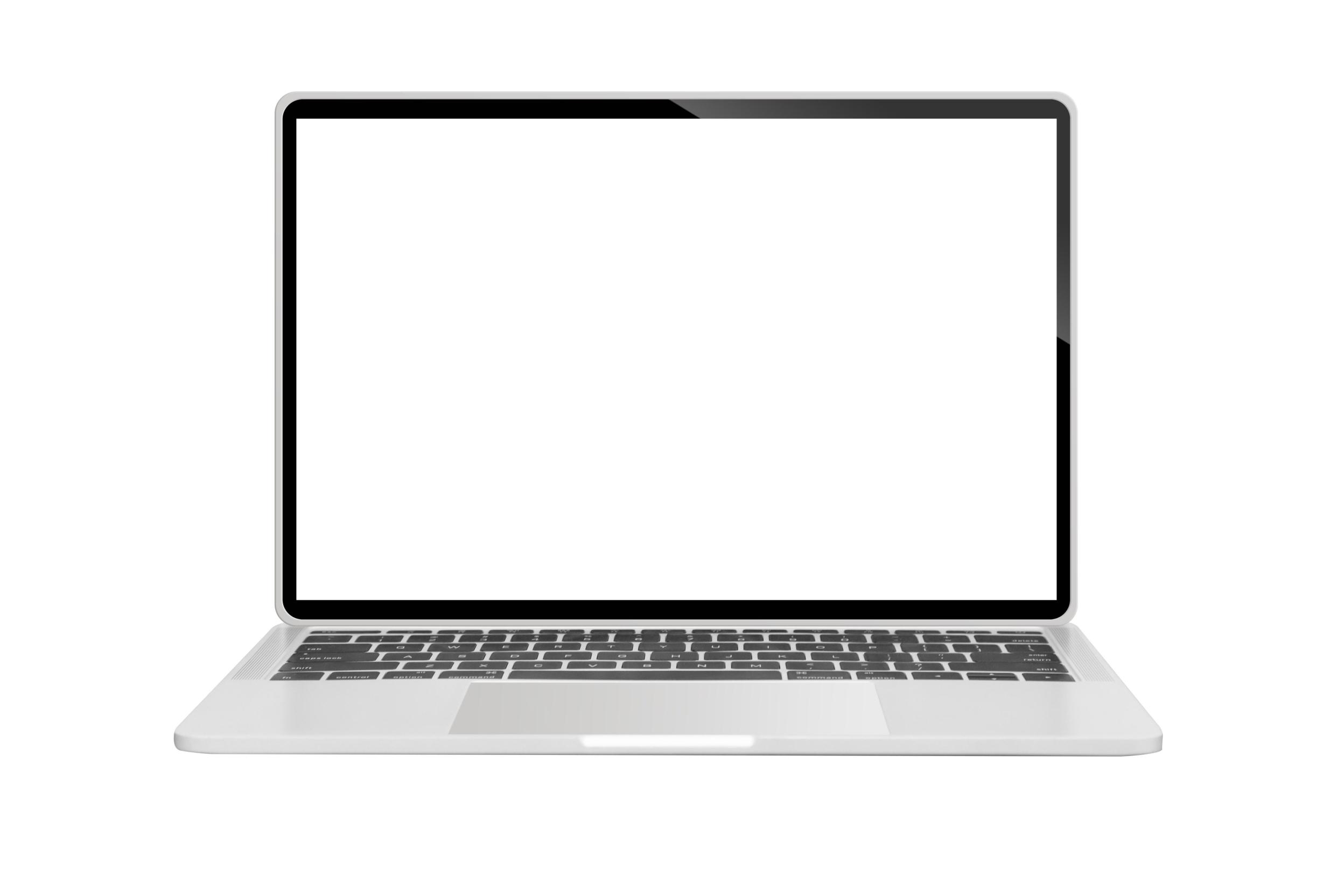
Google is leading this shift. Its "AI Overviews" feature already serves more than one billion users, providing complete answers before anyone clicks a link.[^2] Other players are also gaining ground fast. ChatGPT, Microsoft Copilot, and Pe
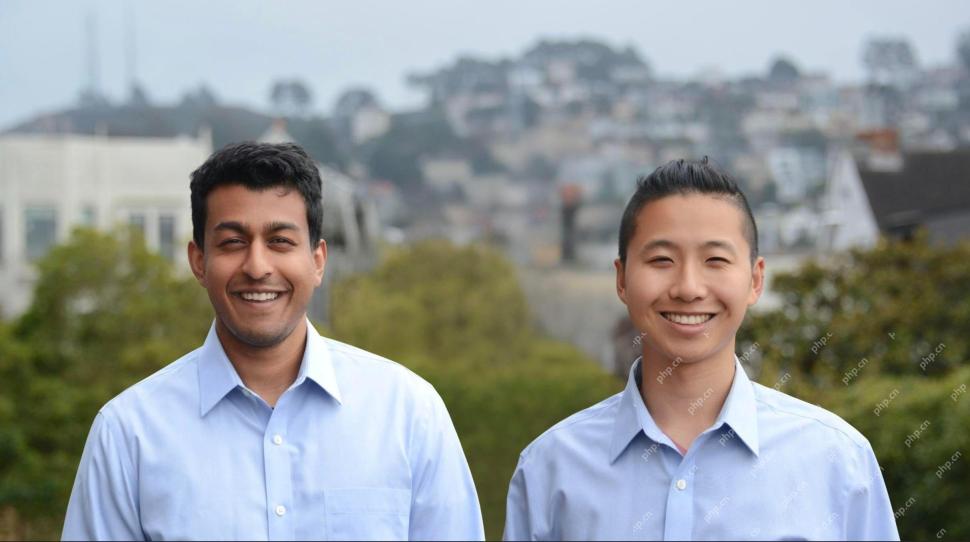
In 2022, he founded social engineering defense startup Doppel to do just that. And as cybercriminals harness ever more advanced AI models to turbocharge their attacks, Doppel’s AI systems have helped businesses combat them at scale— more quickly and
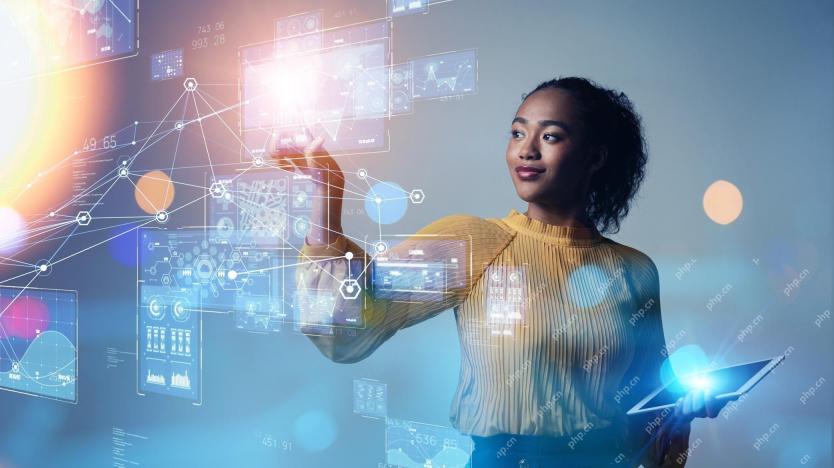
Voila, via interacting with suitable world models, generative AI and LLMs can be substantively boosted. Let’s talk about it. This analysis of an innovative AI breakthrough is part of my ongoing Forbes column coverage on the latest in AI, including
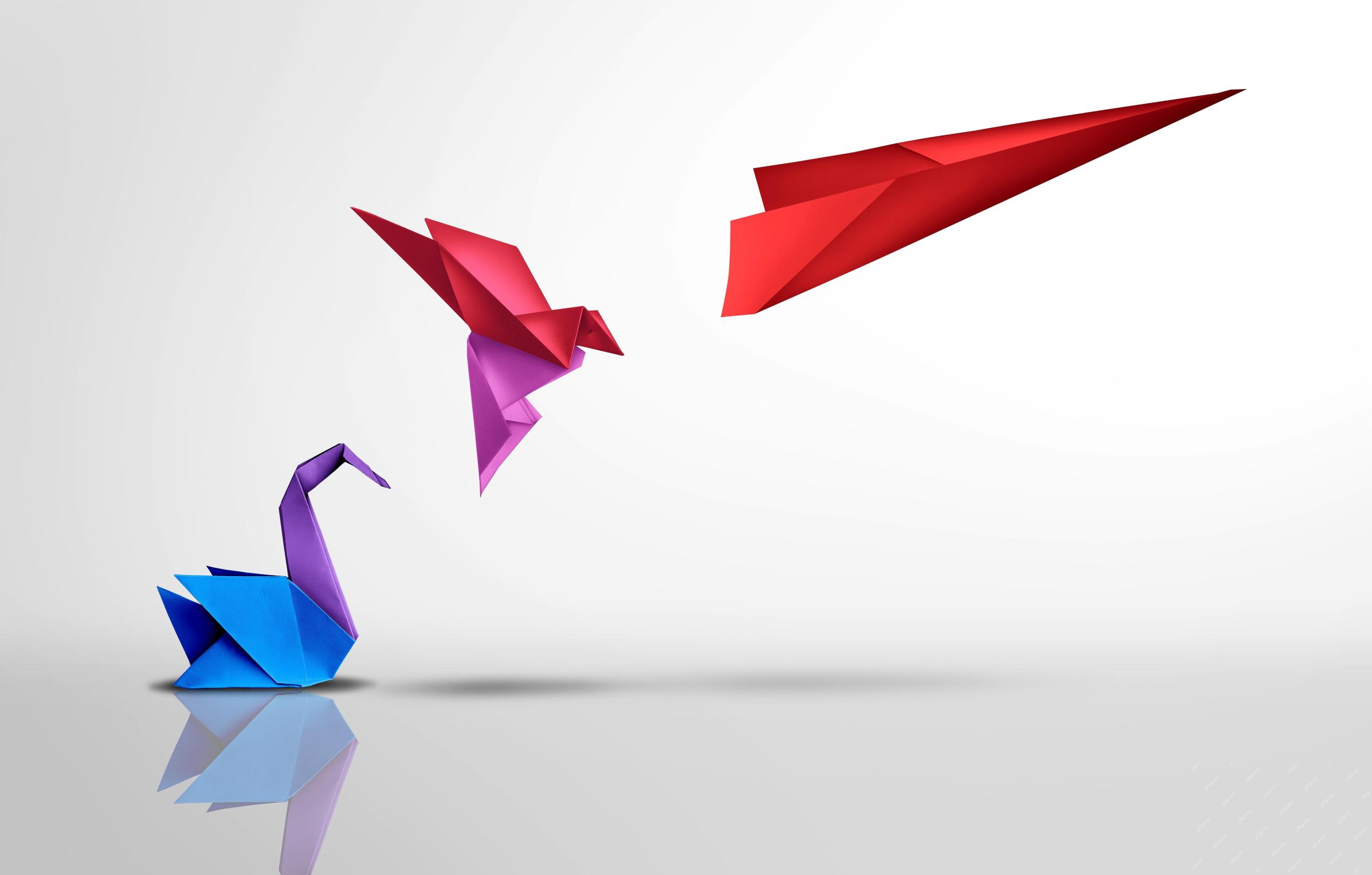
Labor Day 2050. Parks across the nation fill with families enjoying traditional barbecues while nostalgic parades wind through city streets. Yet the celebration now carries a museum-like quality — historical reenactment rather than commemoration of c


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
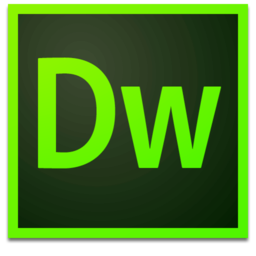
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
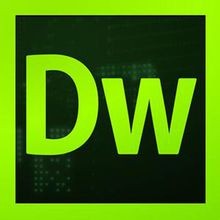
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
