Serverless computing has revolutionized software development, simplifying deployment and DevOps. This tutorial demonstrates building a full-stack serverless application using Cloudflare Workers, a platform that abstracts away scaling and infrastructure. The project, "Repo Hunt," is a daily leaderboard for open-source projects, inspired by Product Hunt and Reddit. The finished site is available here.
Cloudflare Workers, leveraging Cloudflare's global network, delivers extremely low-latency performance regardless of user location. The Wrangler CLI tool simplifies building, uploading, and publishing. This tutorial uses the "Router" template, enabling URL-based routing. The source code is available on GitHub [link-to-github-repo].
Setting Up Your Environment
Install Wrangler:
npm install -g @cloudflare/wrangler
Create a new project using the Router template:
wrangler generate repo-hunt https://github.com/cloudflare/worker-template-router cd repo-hunt
The Router template utilizes Webpack, allowing the inclusion of npm modules. A Router
class handles URL routing. A basic example:
// index.js const Router = require('./router'); addEventListener('fetch', event => { event.respondWith(handleRequest(event.request)); }); async function handleRequest(request) { try { const r = new Router(); r.get('/', () => new Response("Hello, world!")); const resp = await r.route(request); return resp; } catch (err) { return new Response(err); } }
Workers respond to fetch
events, returning a Response
. This tutorial uses routes for the homepage (/
), submission form (/post
), and submission handling (/repo
).
Routing and Templating
The homepage (/
) route renders a list of submitted repositories. We'll start with a simple HTML response:
// index.js const Router = require('./router'); const index = require('./handlers/index'); // ... (rest of index.js) // handlers/index.js const headers = { 'Content-Type': 'text/html' }; const handler = () => { return new Response("Hello, world!", { headers }); }; module.exports = handler;
Use wrangler preview
to test. Next, we'll create a more sophisticated template:
// handlers/index.js const headers = { 'Content-Type': 'text/html' }; const template = require('../templates/index'); // ... (rest of handlers/index.js) // templates/index.js const layout = require('./layout'); const template = () => layout(`<h1 id="Hello-world">Hello, world!</h1>`); module.exports = template; // templates/layout.js const layout = body => `<!-- HTML layout including Bulma CSS -->${body}`; module.exports = layout;
This uses a layout template for consistent styling (Bulma CSS is included).
Data Persistence with Workers KV
Workers KV provides a key-value store for data persistence. We'll store repositories (key: repos:${id}
) and daily lists (key: $date
). Note: Workers KV requires a paid plan.
Basic KV operations:
REPO_HUNT.put("myString", "Hello, world!"); // Setting a value const string = await REPO_HUNT.get("myString"); // Retrieving a value
We'll create classes for managing Repo and Day data:
// store/repo.js const uuid = require('uuid/v4'); class Repo { // ... (Repo class implementation) } module.exports = Repo; // store/day.js const today = () => new Date().toLocaleDateString(); module.exports = { add: async function(id) { // ... }, getRepos: async function() { // ... } };
The Repo
class handles validation and persistence. Day
manages daily repository lists.
Handling User Submissions
A form (GET /post
) allows users to submit repositories. A POST /repo
handler processes submissions:
// handlers/create.js const qs = require('qs'); const Repo = require('../store/repo'); const Day = require('../store/day'); // ... (create handler implementation)
This uses the qs
library to parse form data, creates a Repo
object, saves it to KV, and adds its ID to the daily list.
Rendering Data on the Homepage
The homepage now retrieves and renders repository data:
// handlers/index.js // ... (updated index handler) // templates/index.js // ... (updated template function to render repos)
The Day
module's getRepos
function fetches and instantiates Repo
objects. The template renders each repository.
Deployment
Claim a Workers.dev subdomain:
wrangler subdomain my-subdomain
Deploy the application:
wrangler publish
Create a KV namespace in the Cloudflare dashboard and update wrangler.toml
with the namespace ID:
[[kv-namespaces]] binding = "REPO_HUNT" id = "$yourNamespaceId"
Republish after adding the KV namespace to wrangler.toml
. The application is now live!
Further Development
This tutorial provides a foundation for a serverless application. Future enhancements could include upvoting, comments, and more sophisticated features. Explore the Workers documentation and template gallery for further development ideas.
The above is the detailed content of Building a Full-Stack Serverless Application with Cloudflare Workers. For more information, please follow other related articles on the PHP Chinese website!
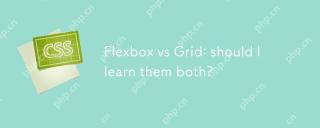
Yes,youshouldlearnbothFlexboxandGrid.1)Flexboxisidealforone-dimensional,flexiblelayoutslikenavigationmenus.2)Gridexcelsintwo-dimensional,complexdesignssuchasmagazinelayouts.3)Combiningbothenhanceslayoutflexibilityandresponsiveness,allowingforstructur
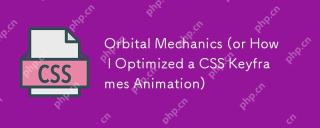
What does it look like to refactor your own code? John Rhea picks apart an old CSS animation he wrote and walks through the thought process of optimizing it.
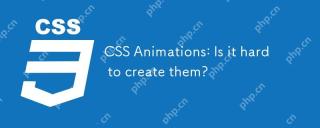
CSSanimationsarenotinherentlyhardbutrequirepracticeandunderstandingofCSSpropertiesandtimingfunctions.1)Startwithsimpleanimationslikescalingabuttononhoverusingkeyframes.2)Useeasingfunctionslikecubic-bezierfornaturaleffects,suchasabounceanimation.3)For
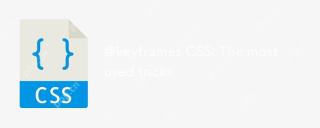
@keyframesispopularduetoitsversatilityandpowerincreatingsmoothCSSanimations.Keytricksinclude:1)Definingsmoothtransitionsbetweenstates,2)Animatingmultiplepropertiessimultaneously,3)Usingvendorprefixesforbrowsercompatibility,4)CombiningwithJavaScriptfo
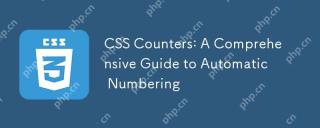
CSSCountersareusedtomanageautomaticnumberinginwebdesigns.1)Theycanbeusedfortablesofcontents,listitems,andcustomnumbering.2)Advancedusesincludenestednumberingsystems.3)Challengesincludebrowsercompatibilityandperformanceissues.4)Creativeusesinvolvecust
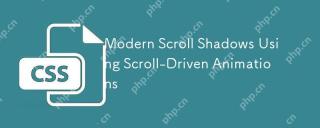
Using scroll shadows, especially for mobile devices, is a subtle bit of UX that Chris has covered before. Geoff covered a newer approach that uses the animation-timeline property. Here’s yet another way.
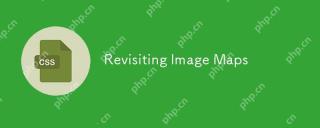
Let’s run through a quick refresher. Image maps date all the way back to HTML 3.2, where, first, server-side maps and then client-side maps defined clickable regions over an image using map and area elements.
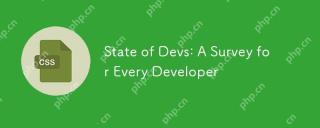
The State of Devs survey is now open to participation, and unlike previous surveys it covers everything except code: career, workplace, but also health, hobbies, and more.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
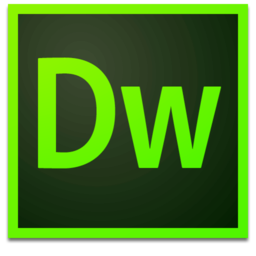
Dreamweaver Mac version
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
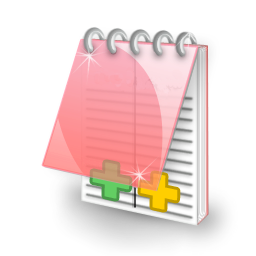
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
