Using Visual Studio: Developing Software Across Platforms
Cross-platform development with Visual Studio is feasible, and by supporting frameworks like .NET Core and Xamarin, developers can write code at once and run on multiple operating systems. 1) Create .NET Core projects and use their cross-platform capabilities, 2) Use Xamarin for mobile application development, 3) Use asynchronous programming and code reuse to optimize performance to ensure efficient operation and maintainability of applications.
introduction
In today's world of software development, cross-platform development has become a trend. Whether you are developing mobile applications, desktop applications, or web applications, it is very important to be able to run your software on different operating systems. As an integrated development environment (IDE), Visual Studio not only performs well on the Windows platform, but also supports cross-platform development through various tools and extensions. This article will take you into the deep understanding of how to use Visual Studio for cross-platform software development to help you master this skill.
By reading this article, you will learn how to use Visual Studio for cross-platform development, understand its strengths and challenges, and master some practical tips and best practices.
Review of basic knowledge
Visual Studio is a powerful IDE that supports multiple programming languages and development frameworks. Its main advantage lies in its integrated debugging tools, code editor and project management capabilities. Cross-platform development usually involves the use of different programming languages and frameworks, such as C#, .NET Core, Xamarin, etc.
In cross-platform development, common technologies include:
- .NET Core : An open source cross-platform framework that allows developers to write applications that can run on Windows, Linux, and macOS in languages such as C# and F#.
- Xamarin : A framework for building cross-platform mobile applications that allow developers to use C# and .NET to develop iOS and Android applications.
- Visual Studio Code : A lightweight code editor that supports multiple programming languages and platforms, and is often used for cross-platform development.
Core concept or function analysis
The definition and role of cross-platform development
Cross-platform development refers to the development method of writing code once and then running on multiple operating systems. Its main function is to reduce development and maintenance costs and improve code reusability. Visual Studio makes it easier for developers to achieve this by supporting a variety of cross-platform frameworks and tools.
For example, web applications developed using .NET Core can run on Windows, Linux, and macOS without major code modifications.
How it works
The main way Visual Studio supports cross-platform development is through the integration of different development frameworks and tools. For example, the .NET Core project can be created and debugged in Visual Studio, while the Xamarin project allows developers to write iOS and Android applications in C#.
When using .NET Core, Visual Studio compiles the code to an intermediate language (IL) and is then executed on different platforms by the .NET Core runtime. This allows the code to run on different operating systems without recompiling.
// .NET Core example using System; namespace HelloWorld { class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } } }
When using Xamarin, Visual Studio compiles C# code into native code for iOS and Android, thereby enabling cross-platform mobile application development.
// Xamarin example using Xamarin.Forms; namespace MyXamarinApp { public class App: Application { public App() { MainPage = new ContentPage { Content = new StackLayout { VerticalOptions = LayoutOptions.Center, Children = { new Label { HorizontalTextAlignment = TextAlignment.Center, Text = "Welcome to Xamarin.Forms!" } } } }; } } }
Example of usage
Basic usage
The basic steps of cross-platform development with Visual Studio include creating projects, writing code, and debugging. Here is an example of creating a web application using .NET Core:
// .NET Core Web Application Example using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.AspNetCore.Http; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; namespace WebApplication1 { public class Startup { public void ConfigureServices(IServiceCollection services) { } public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapGet("/", async context => { await context.Response.WriteAsync("Hello World!"); }); }); } } public class Program { public static void Main(string[] args) { CreateHostBuilder(args).Build().Run(); } public static IHostBuilder CreateHostBuilder(string[] args) => Host.CreateDefaultBuilder(args) .ConfigureWebHostDefaults(webBuilder => { webBuilder.UseStartup<Startup>(); }); } }
This example shows how to create a simple web application using .NET Core and debug and run it in Visual Studio.
Advanced Usage
In cross-platform development, it is often necessary to deal with specific functions of different platforms. For example, when developing mobile applications using Xamarin, you may need to use platform-specific APIs to implement certain features. Here is an example of implementing platform-specific functionality using Xamarin.Forms and dependency injection:
// Xamarin.Forms platform-specific feature example using Xamarin.Forms; namespace MyXamarinApp { public class App: Application { public App() { MainPage = new ContentPage { Content = new StackLayout { VerticalOptions = LayoutOptions.Center, Children = { new Button { Text = "Click me", Command = new Command(async () => { var result = await DependencyService.Get<IPlatformService>().GetPlatformInfo(); await DisplayAlert("Platform Info", result, "OK"); }) } } } }; } } public interface IPlatformService { Task<string> GetPlatformInfo(); } // Implement IPlatformService interface in iOS and Android projects} // iOS implements using MyXamarinApp.iOS; using Foundation; [assembly: Xamarin.Forms.Dependency(typeof(PlatformService))] namespace MyXamarinApp.iOS { public class PlatformService : IPlatformService { public async Task<string> GetPlatformInfo() { return await Task.FromResult("iOS: " UIDevice.CurrentDevice.SystemVersion); } } } // Android implements using MyXamarinApp.Droid; using Android.OS; [assembly: Xamarin.Forms.Dependency(typeof(PlatformService))] namespace MyXamarinApp.Droid { public class PlatformService : IPlatformService { public async Task<string> GetPlatformInfo() { return await Task.FromResult("Android: "BuildConfig.VersionName); } } }
This example shows how to use dependency injection and platform-specific implementations to handle the functionality of different platforms.
Common Errors and Debugging Tips
Common errors in cross-platform development include:
- Platform compatibility issues : APIs and functions of different platforms may vary and need to be handled carefully.
- Dependency management issues : Dependency management methods may be different on different platforms, and it is necessary to ensure that all dependencies are configured correctly.
- Performance issues : Cross-platform applications may perform differently on different platforms and need to be optimized.
Debugging skills include:
- Remote debugging features using Visual Studio : You can remotely connect to devices on different platforms for debugging.
- Use logs and monitoring tools : Add logs to your code to help locate problems.
- Using emulators and virtual machines : Use emulators and virtual machines to test during development to simulate environments on different platforms.
Performance optimization and best practices
Performance optimization and best practices are very important in cross-platform development. Here are some suggestions:
- Using asynchronous programming : In .NET Core and Xamarin, using asynchronous programming can improve application responsiveness and performance.
// Asynchronous programming example public async Task<string> GetDataAsync() { // Simulation time-consuming operation await Task.Delay(1000); return "Data"; }
- Optimize dependencies and libraries : Ensure that only necessary dependencies and libraries are introduced, reducing application size and startup time.
- Code reuse and modularity : Reuse code as much as possible to improve the maintainability and testability of the code.
// Code reuse example public class DataService { public async Task<string> GetDataAsync() { // Implement data acquisition logic} } public class ViewModel { private readonly DataService _dataService; public ViewModel(DataService dataService) { _dataService = dataService; } public async Task LoadDataAsync() { var data = await _dataService.GetDataAsync(); // Process data} }
- Performance testing and optimization : Use performance analysis tools to identify bottlenecks in your application and optimize.
With these methods and techniques, you can efficiently develop cross-platform in Visual Studio to create high-performance, maintainable software applications.
The above is the detailed content of Using Visual Studio: Developing Software Across Platforms. For more information, please follow other related articles on the PHP Chinese website!

The difference between VisualStudioProfessional and Enterprise is in the functionality and target user groups. The Professional version is suitable for professional developers and provides functions such as code analysis; the Enterprise version is for large teams and has added advanced tools such as test management.

VisualStudio is suitable for large projects, VSCode is suitable for projects of all sizes. 1. VisualStudio provides comprehensive IDE functions, supports multiple languages, integrated debugging and testing tools. 2.VSCode is a lightweight editor that supports multiple languages through extension, has a simple interface and fast startup.

VisualStudio is a powerful IDE developed by Microsoft, supporting multiple programming languages and platforms. Its core advantages include: 1. Intelligent code prompts and debugging functions, 2. Integrated development, debugging, testing and version control, 3. Extended functions through plug-ins, 4. Provide performance optimization and best practice tools to help developers improve efficiency and code quality.

The differences in pricing, licensing and availability of VisualStudio and VSCode are as follows: 1. Pricing: VSCode is completely free, while VisualStudio offers free community and paid enterprise versions. 2. License: VSCode uses a flexible MIT license, and the license of VisualStudio varies according to the version. 3. Usability: VSCode is supported across platforms, while VisualStudio performs best on Windows.

VisualStudio supports the entire process from code writing to production deployment. 1) Code writing: Provides intelligent code completion and reconstruction functions. 2) Debugging and testing: Integrate powerful debugging tools and unit testing framework. 3) Version control: seamlessly integrate with Git to simplify code management. 4) Deployment and Release: Supports multiple deployment options to simplify the application release process.

VisualStudio offers three license types: Community, Professional and Enterprise. The Community Edition is free, suitable for individual developers and small teams; the Professional Edition is annually subscribed, suitable for professional developers who need more functions; the Enterprise Edition is the highest price, suitable for large teams and enterprises. When selecting a license, project size, budget and teamwork needs should be considered.

VisualStudio is suitable for large-scale project development, while VSCode is suitable for projects of all sizes. 1. VisualStudio provides comprehensive development tools, such as integrated debugger, version control and testing tools. 2.VSCode is known for its scalability, cross-platform and fast launch, and is suitable for fast editing and small project development.

VisualStudio is suitable for large projects and Windows development, while VSCode is suitable for cross-platform and small projects. 1. VisualStudio provides a full-featured IDE, supports .NET framework and powerful debugging tools. 2.VSCode is a lightweight editor that emphasizes flexibility and extensibility, and is suitable for various development scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
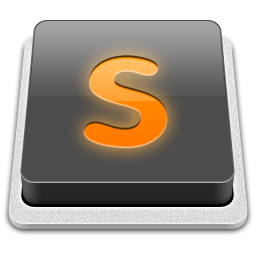
SublimeText3 Mac version
God-level code editing software (SublimeText3)
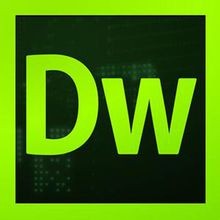
Dreamweaver CS6
Visual web development tools
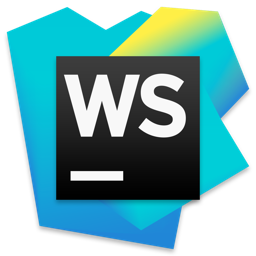
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
