Docker and Kubernetes are key tools for modern software development and deployment. Docker simplifies application packaging and deployment through containerization, while Kubernetes is used for large-scale container orchestration and management. Using Docker and Kubernetes can significantly improve the scalability and management efficiency of your application.
introduction
Docker and Kubernetes have become indispensable tools in modern software development and deployment. They not only simplify the packaging and deployment process of applications, but also greatly improve the scalability and management efficiency of applications. This article will take you into the core concepts of Docker and Kubernetes, how they work, and their best practices in real-world applications. By reading this article, you will learn how to use Docker containerized applications and how to use Kubernetes for large-scale container orchestration and management.
Review of basic knowledge
The core of Docker and Kubernetes is container technology. Containers are a lightweight virtualization technology that allows you to run applications in an isolated environment. Docker provides an easy way to package your app and its dependencies so that it can run anywhere. Kubernetes is an open source container orchestration system that can automatically deploy, scale and manage containerized applications.
Before using Docker and Kubernetes, you need to understand some basic concepts, such as image (Image), container (Container), Pod, Service, etc. These concepts are the basis for understanding and using Docker and Kubernetes.
Core concept or function analysis
The definition and function of Docker
Docker is a containerized platform that allows it to run in any Docker-enabled environment by packaging applications and their dependencies into a mirror. The advantage of Docker is that it provides a high degree of portability and consistency, and the application behavior is consistent in both development and production environments.
# Example: Create a simple Docker image FROM ubuntu:latest RUN apt-get update && apt-get install -y nginx CMD ["nginx", "-g", "daemon off;"]
This Dockerfile shows how to create an image containing Nginx. This way, you can make sure that the app runs the same way anywhere.
The definition and function of Kubernetes
Kubernetes is a system for automated deployment, scaling, and managing containerized applications. It makes managing large-scale containers easier and more efficient by providing a range of abstractions and APIs. The core concepts of Kubernetes include Pod, Service, Deployment, etc. These concepts help you manage and expand applications.
# Example: Kubernetes Deployment Configuration File apiVersion: apps/v1 kind: Deployment metadata: name: nginx-deployment spec: replicas: 3 selector: matchLabels: app: nginx template: metadata: labels: app: nginx spec: containers: - name: nginx image: nginx:latest Ports: - containerPort: 80
This YAML file defines a Deployment named nginx-deployment, which creates three Pods running Nginx.
How Docker works
The working principle of Docker can be divided into the following steps:
- Mirror construction : Define the application and its dependencies through Dockerfile to build a mirror.
- Container Run : Start a container from a mirror, a running mirror instance.
- Container Management : Docker provides a series of commands to manage the life cycle of a container, such as starting, stopping, deleting, etc.
Docker uses Union File System to implement hierarchical storage of images, which allows images to share common tiers, thus saving storage space.
How Kubernetes works
The working principle of Kubernetes can be divided into the following aspects:
- Scheduling : Kubernetes assigns Pods to nodes in the cluster through a scheduler.
- Management : Kubernetes manages the life cycle of a Pod through a controller to ensure that the Pod runs as expected.
- Service Discovery : Kubernetes provides service discovery and load balancing functions through Service, so that Pods can communicate with each other.
Kubernetes uses etcd as its distributed key-value store to ensure consistency in cluster state.
Example of usage
Basic usage of Docker
The basic usage of Docker includes building images, running containers, and managing containers. Here is a simple example:
# Build the image docker build -t my-nginx. # Run container docker run -d -p 8080:80 my-nginx # View running container docker ps
This example shows how to build an Nginx image and run a container locally.
Basic usage of Kubernetes
The basic usage of Kubernetes includes creating Deployment, Service, and Pods. Here is a simple example:
# Create a Deployment kubectl apply -f nginx-deployment.yaml # Create Service kubectl expose deployment nginx-deployment --type=LoadBalancer --port=80 # Check Pod status kubectl get pods
This example shows how to create an Nginx Deployment and Service in Kubernetes.
Advanced Usage
Advanced usage of Docker includes multi-stage construction, Docker Compose, etc. Here is an example of a multi-stage build:
# Multi-stage construction example FROM golang:1.16 AS builder WORKDIR /app COPY . . RUN CGO_ENABLED=0 GOOS=linux go build -a -installsuffix cgo -o app . FROM alpine:latest WORKDIR /root/ COPY --from=builder /app/app . CMD ["./app"]
This Dockerfile shows how to use multi-stage builds to reduce image size.
Advanced usage of Kubernetes includes using Helm for application deployment, using Istio for service mesh management, etc. Here is an example of deploying an application using Helm:
# Add Helm repo add stable https://charts.helm.sh/stable # Install and apply helm install my-nginx stable/nginx-ingress
This example shows how to quickly deploy an Nginx Ingress controller using Helm.
Common Errors and Debugging Tips
Common errors when using Docker and Kubernetes include image building failure, container failure, Pod failure to schedule, etc. Here are some debugging tips:
- Mirror build failed : Check every line in the Dockerfile to make sure the command is correct. Use
docker build --no-cache
to rebuild the image. - The container cannot be started : view the container logs and use
docker logs <container_id></container_id>
to find error information. - Pod cannot be scheduled : Check the events of the Pod and use
kubectl describe pod <pod_name></pod_name>
to find the reason for the scheduling failure.
Performance optimization and best practices
Performance optimization and best practices are very important when using Docker and Kubernetes. Here are some suggestions:
- Mirror Optimization : Use multi-stage builds to reduce image size and reduce transfer and storage costs.
- Resource management : reasonably set Pod resource requests and restrictions in Kubernetes to avoid resource waste and competition.
- Monitoring and logging : Use Prometheus and Grafana to monitor cluster status, use ELK stack to manage logs, and promptly discover and resolve problems.
In practical applications, the performance optimization of Docker and Kubernetes needs to be adjusted according to specific business needs. Here is an example of optimizing the image size:
# Example for optimizing image size FROM golang:1.16 AS builder WORKDIR /app COPY . . RUN CGO_ENABLED=0 GOOS=linux go build -a -installsuffix cgo -o app . FROM scratch COPY --from=builder /app/app . CMD ["./app"]
This Dockerfile uses scratch
as the base image, further reducing the image size.
In short, Docker and Kubernetes are powerful tools for modern application deployment and management. By gaining insight into their core concepts and working principles, you can better utilize them to improve the reliability and scalability of your application. In practical applications, continuous learning and practice are the key to mastering these tools.
The above is the detailed content of Docker and Kubernetes: A Technical Deep Dive. For more information, please follow other related articles on the PHP Chinese website!

The ways Docker can simplify development and operation and maintenance processes include: 1) providing a consistent environment to ensure that applications run consistently in different environments; 2) optimizing application deployment through Dockerfile and image building; 3) using DockerCompose to manage multiple services. Docker implements these functions through containerization technology, but during use, you need to pay attention to common problems such as image construction, container startup and network configuration, and improve performance through image optimization and resource management.

The relationship between Docker and Kubernetes is: Docker is used to package applications, and Kubernetes is used to orchestrate and manage containers. 1.Docker simplifies application packaging and distribution through container technology. 2. Kubernetes manages containers to ensure high availability and scalability. They are used in combination to improve application deployment and management efficiency.

Docker solves the problem of consistency in software running in different environments through container technology. Its development history has promoted the evolution of the cloud computing ecosystem from 2013 to the present. Docker uses Linux kernel technology to achieve process isolation and resource limitation, improving the portability of applications. In development and deployment, Docker improves resource utilization and deployment speed, supports DevOps and microservice architectures, but also faces challenges in image management, security and container orchestration.

Docker and virtual machines have their own advantages and disadvantages, and the choice should be based on specific needs. 1.Docker is lightweight and fast, suitable for microservices and CI/CD, fast startup and low resource utilization. 2. Virtual machines provide high isolation and multi-operating system support, but they consume a lot of resources and slow startup.

The core concept of Docker architecture is containers and mirrors: 1. Mirrors are the blueprint of containers, including applications and their dependencies. 2. Containers are running instances of images and are created based on images. 3. The mirror consists of multiple read-only layers, and the writable layer is added when the container is running. 4. Implement resource isolation and management through Linux namespace and control groups.

Docker simplifies the construction, deployment and operation of applications through containerization technology. 1) Docker is an open source platform that uses container technology to package applications and their dependencies to ensure cross-environment consistency. 2) Mirrors and containers are the core of Docker. The mirror is the executable package of the application and the container is the running instance of the image. 3) Basic usage of Docker is like running an Nginx server, and advanced usage is like using DockerCompose to manage multi-container applications. 4) Common errors include image download failure and container startup failure, and debugging skills include viewing logs and checking ports. 5) Performance optimization and best practices include mirror optimization, resource management and security improvement.

The steps to deploy containerized applications using Kubernetes and Docker include: 1. Build a Docker image, define the application image using Dockerfile and push it to DockerHub. 2. Create Deployment and Service in Kubernetes to manage and expose applications. 3. Use HorizontalPodAutoscaler to achieve dynamic scaling. 4. Debug common problems through kubectl command. 5. Optimize performance, define resource limitations and requests, and manage configurations using Helm.

Docker is an open source platform for developing, packaging and running applications, and through containerization technology, solving the consistency of applications in different environments. 1. Build the image: Define the application environment and dependencies through the Dockerfile and build it using the dockerbuild command. 2. Run the container: Use the dockerrun command to start the container from the mirror. 3. Manage containers: manage container life cycle through dockerps, dockerstop, dockerrm and other commands.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
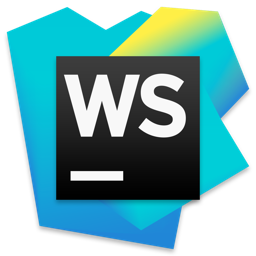
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
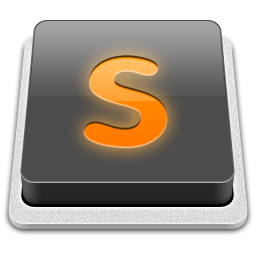
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
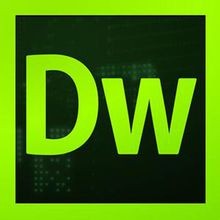
Dreamweaver CS6
Visual web development tools
