Seemingly out of the blue, the Gulp processing I had set up for this site started to have a race condition. I’d run my watch command, change some CSS, and the processing would sometimes leave behind some extra files that were meant to be cleaned up during the processing. Like the cleanup tasks happened before the files landed in the file system (or something… I never really got to the bottom of it).
Nevermind about the specifics of that bug. I figured I’d go about solving it by upgrading things to use Gulp 4.x instead of 3.x, and running things in the built-in gulp.series command, which I thought would help (it did).
Getting Gulp 4.x going was a heck of a journey for me, involving me giving up for a year, then reigniting the struggle and ultimately getting it fixed. My trouble was that Gulp 4 requires a CLI version of 2.x while Gulp 3, for whatever reason, used a 3.x version. Essentially I needed to downgrade versions, but after trying a billion things to do that, nothing seemed to work, like there was a ghost version of CLI 3.x on my machine.
I’m sure savvier command-line folks could have sussed this out faster than me, but it turns out running command -v gulp will reveal the file path of where Gulp is installed which revealed /usr/local/share/npm/bin/gulp for me, and deleting it manually from there before re-installing the lastest version worked in getting me back down to 2.x.
Now that I could use Gulp 4.x, I re-wrote my gulpfile.js into smaller functions, each fairly isolated in responsibility. Much of this is pretty unique to my setup on this site, so it’s not meant to be some boilerplate for generic usage. I’m just publishing because it certainly would have been helpful for me to reference as I was creating it.
Things my particular Gulpfile does
- Runs a web server (Browsersync) for style injection and auto-refreshing
- Runs a file watcher (native Gulp feature) for running the right tasks on the right files and doing the above things
- CSS processing
- Sass > Autoprefixer > Minify
- Break stylesheet cache from the templates (e.g.
- Put style.css in the right place for a WordPress theme and clean up files only needed during processing
- JavaScript processing
- Babel > Concatenate > Minify
- Break browser cache for the <script>s</script>
- Clean up unused files created in processing
- SVG processing
- Make an SVG sprite (a block of
s - Name it as a sprite.php file (so it can be PHP-included in a template) and put it somewhere specific
- Make an SVG sprite (a block of
- PHP processing
- Update the Ajax call in the JavaScript to cache-bust when the ads change
Code dump!
const gulp = require("gulp"), browserSync = require("browser-sync").create(), sass = require("gulp-sass"), postcss = require("gulp-postcss"), autoprefixer = require("autoprefixer"), cssnano = require("cssnano"), del = require("del"), babel = require("gulp-babel"), minify = require("gulp-minify"), concat = require("gulp-concat"), rename = require("gulp-rename"), replace = require("gulp-replace"), svgSymbols = require("gulp-svg-symbols"), svgmin = require("gulp-svgmin"); const paths = { styles: { src: ["./scss/*.scss", "./art-direction/*.scss"], dest: "./css/" }, scripts: { src: ["./js/*.js", "./js/libs/*.js", "!./js/min/*.js"], dest: "./js/min" }, svg: { src: "./icons/*.svg" }, php: { src: ["./*.php", "./ads/*.php", "./art-direction/*.php", "./parts/**/*.php"] }, ads: { src: "./ads/*.php" } }; /* STYLES */ function doStyles(done) { return gulp.series(style, moveMainStyle, deleteOldMainStyle, done => { cacheBust("./header.php", "./"); done(); })(done); } function style() { return gulp .src(paths.styles.src) .pipe(sass()) .on("error", sass.logError) .pipe(postcss([autoprefixer(), cssnano()])) .pipe(gulp.dest(paths.styles.dest)) .pipe(browserSync.stream()); } function moveMainStyle() { return gulp.src("./css/style.css").pipe(gulp.dest("./")); } function deleteOldMainStyle() { return del("./css/style.css"); } /* END STYLES */ /* SCRIPTS */ function doScripts(done) { return gulp.series( preprocessJs, concatJs, minifyJs, deleteArtifactJs, reload, done => { cacheBust("./parts/footer-scripts.php", "./parts/"); done(); } )(done); } function preprocessJs() { return gulp .src(paths.scripts.src) .pipe( babel({ presets: ["@babel/env"], plugins: ["@babel/plugin-proposal-class-properties"] }) ) .pipe(gulp.dest("./js/babel/")); } function concatJs() { return gulp .src([ "js/libs/jquery.lazy.js", "js/libs/jquery.fitvids.js", "js/libs/jquery.resizable.js", "js/libs/prism.js", "js/babel/highlighting-fixes.js", "js/babel/global.js" ]) .pipe(concat("global-concat.js")) .pipe(gulp.dest("./js/concat/")); } function minifyJs() { return gulp .src(["./js/babel/*.js", "./js/concat/*.js"]) .pipe( minify({ ext: { src: ".js", min: ".min.js" } }) ) .pipe(gulp.dest(paths.scripts.dest)); } function deleteArtifactJs() { return del([ "./js/babel", "./js/concat", "./js/min/*.js", "!./js/min/*.min.js" ]); } /* END SCRIPTS */ /* SVG */ function doSvg() { return gulp .src(paths.svg.src) .pipe(svgmin()) .pipe( svgSymbols({ templates: ["default-svg"], svgAttrs: { width: 0, height: 0, display: "none" } }) ) .pipe(rename("icons/sprite/icons.php")) .pipe(gulp.dest("./")); } /* END SVG */ /* GENERIC THINGS */ function cacheBust(src, dest) { var cbString = new Date().getTime(); return gulp .src(src) .pipe( replace(/cache_bust=\d /g, function() { return "cache_bust=" cbString; }) ) .pipe(gulp.dest(dest)); } function reload(done) { browserSync.reload(); done(); } function watch() { browserSync.init({ proxy: "csstricks.local" }); gulp.watch(paths.styles.src, doStyles); gulp.watch(paths.scripts.src, doScripts); gulp.watch(paths.svg.src, doSvg); gulp.watch(paths.php.src, reload); gulp.watch(paths.ads.src, done => { cacheBust("./js/global.js", "./js/"); done(); }); } gulp.task("default", watch);
Problems / Questions
- The worst part is that it doesn’t break cache very intelligently. When CSS changes, it breaks the cache on all stylesheets, not just the relevant ones.
- I’d probably just inline SVG icons with PHP include()s in the future rather than deal with spriting.
- The SVG processor breaks if the original SVGs have width and height attributes, which seems wrong.
- Would gulp-changed be a speed boost? As in, only looking at files that have changed instead of all files? Or is it not necessary anymore?
- Should I be restarting gulp on gulpfile.js changes?
- Sure would be nice if all the libs I used were ES6-compatible so I could import stuff rather than having to manually concatenate.
Always so much more that can be done. Ideally, I’d just open-source this whole site, I just haven’t gotten there yet.
The above is the detailed content of Just Sharing My Gulpfile. For more information, please follow other related articles on the PHP Chinese website!
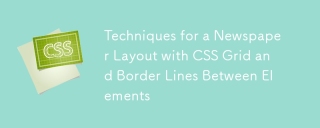
I recently had to craft a newspaper-like design that featured multiple row and column spans with divider lines in between them. Take a look at the mockup
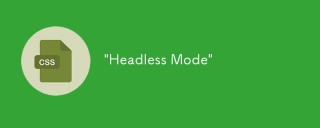
A couple of months ago, we invited Marc Anton Dahmen to show off his database-less content management system (CMS) Automad. His post is an interesting inside
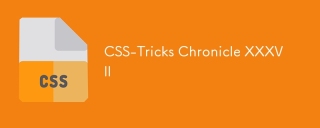
Chronicle posts are opportunities for me to round-up things that I haven't gotten a chance to post about yet, rounded up together. It's stuff like podcasts
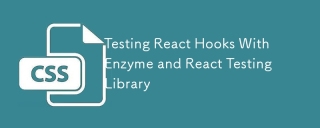
As you begin to make use of React hooks in your applications, you’ll want to be certain the code you write is nothing short of solid. There’s nothing like
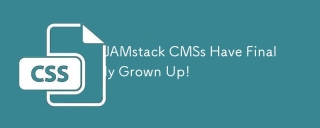
This article is based on Brian's presentation at Connect.Tech 2019. Slides with speaker notes from that presentation are available to download.
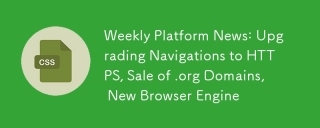
In this week's roundup: DuckDuckGo gets smarter encryption, a fight over the sale of dot org domains, and a new browser engine is in the works.
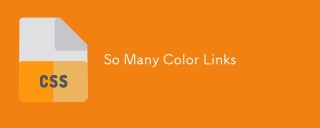
There's been a run of tools, articles, and resources about color lately. Please allow me to close a few tabs by rounding them up here for your enjoyment.
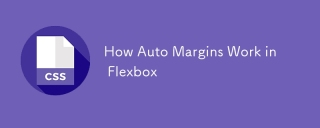
Robin has covered this before, but I've heard some confusion about it in the past few weeks and saw another person take a stab at explaining it, and I wanted


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
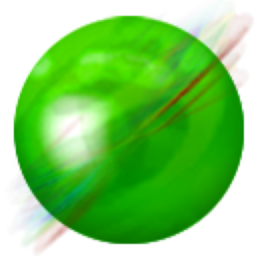
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
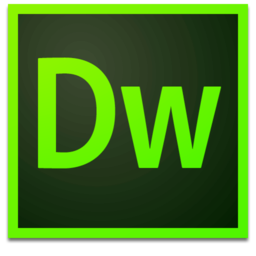
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
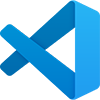
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
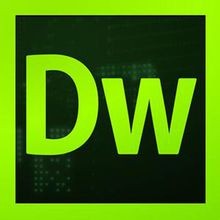
Dreamweaver CS6
Visual web development tools