sql cheat sheet
This blog provides comprehensive guidance on the most important SQL commands and operations. It covers basic queries, connections, subqueries, indexes, and more advanced concepts.
Table of contents
- Basics of SQL
- Data definition language (ddl)
- Data operation language (dml)
- Data query language (dql)
- Data Control Language (dcl)
- join in
- Subquery
- index
- Aggregation function
- Grouping and sorting
- trade
- Advanced sql
- Best Practices
Basics of SQL
Structure of SQL query
select column1, column2 from table_name Where condition order by column limit n;
Comment in SQL
- Single comment : -- This is a comment
- Multiple comments :
/* this is a multi-line comment */
Data definition language (ddl)
Create a table
create table table_name ( column1 datatype [constraints], column2 datatype [constraints], ... );
Example:
create table employees ( id int primary key, name varchar(100), age int, hire_date date );
Modify the form
Add column
alter table table_name add column_name datatype;
Delete a column
alter table table_name drop column column_name;
Modify columns
alter table table_name modify column column_name datatype;
Rename table
alter table old_table_name rename to new_table_name;
Delete a table
drop table table_name;
Create an index
create index index_name on table_name (column_name);
Delete the index
drop index index_name;
Data operation language (dml)
Insert data into table
insert into table_name (column1, column2, ...) values (value1, value2, ...);
Example:
insert into employees (id, name, age, hire_date) values (1, 'john doe', 30, '2022-01-01');
Update data in table
update table_name set column1 = value1, column2 = value2, ... where condition;
Example:
update employees set age = 31 where id = 1;
Delete data from table
delete from table_name where condition;
Example:
delete from employees where id = 1;
Data query language (dql)
Select data from the table
select column1, column2, ... from table_name Where condition order by column limit n;
Example:
select * from employees; select name, age from employees where age > 30;
Wildcard
- *: Select all columns
- %: a wildcard with zero or more characters (in the like clause)
- _: Wildcards representing only one character (in the like clause)
Example:
select * from employees where name like 'j%';
Data Control Language (dcl)
Grant permissions
grant permission on object to user;
Example:
grant select, insert on employees to 'user1';
Revoke permissions
revoke permission on object from user;
Example:
revoke select on employees from 'user1';
join in
Inner connection
Returns rows when there are matches in both tables.
Select columns from table1 inner join table2 on table1.column = table2.column;
Left connection (or left outer connection)
Returns all rows in the left table and matched rows in the right table. If it does not match, the columns in the right table will display a null value.
Select columns from table1 left join table2 on table1.column = table2.column;
Right connection (or right external connection)
Returns all rows in the right table and matched rows in the left table. If it does not match, the columns in the left table will display a null value.
Select columns from table1 right join table2 on table1.column = table2.column;
Fully external connection
Returns rows when there is a match in one of the tables.
Select columns from table1 full outer join table2 on table1.column = table2.column;
Subquery
Subquery in select
select column1, (select column2 from table2 where condition) as alias from table1;
Subquery in where
select column1 from table1 where column2 in (select column2 from table2 where condition);
Subquery in from
select alias.column1 from (select column1 from table2 where condition) as alias;
index
Create an index
create index index_name on table_name (column1, column2);
Delete the index
drop index index_name;
Unique index
Make sure that all values in one column (or a group of columns) are unique.
create unique index index_name on table_name (column_name);
Aggregation function
Count
Calculate the number of rows that meet certain criteria.
select count(*) from table_name where condition;
and
Returns the sum of the values in the column.
select sum(column_name) from table_name;
Average voltage
Returns the average value of the values in the column.
select avg(column_name) from table_name;
Minimum and maximum values
Returns the minimum and maximum values in the column.
select min(column_name), max(column_name) from table_name;
Grouping and sorting
Grouping basis
Group rows with the same value into summary rows.
select column1, count(*) from table_name group by column1;
have
Apply group by to filter the group.
select column1, count(*) from table_name group by column1 having count(*) > 5;
Order basis
Sort the result set in ascending or descending order.
select column1, column2 from table_name order by column1 desc;
trade
Start trading
begin transaction;
Conduct a transaction
commit;
Roll back transactions
rollback;
Advanced sql
Case when
Conditional logic in the query.
select column1, case When condition then 'result 1' When condition then 'result 2' else 'default' end as alias from table_name;
United and United All
- union : merges the result sets of two or more queries (delete duplicates).
- union all : merge result sets (retain duplicates).
select column from table1 union select column from table2; select column from table1 union all select column from table2;
Best Practices
- Use join instead of subquery when possible for better performance.
- Index frequently searched columns to speed up queries.
- Avoid select * and specify only the columns you need.
- Use limit on the number of rows returned for large result sets .
- Standardize your data to avoid redundancy and improve consistency.
- Use the where clause instead of filtering the data before aggregation.
- Test query performance, especially for large data sets.
- Use transactions to ensure data consistency, especially operations involving multiple dml statements.
in conclusion
This sql cheat sheet covers all the basic sql commands and techniques required to use a relational database. Whether you are querying, inserting, updating, or connecting data, this guide will help you use SQL more effectively.
The above is the detailed content of SQL Quick Reference: Simplify Database Management. For more information, please follow other related articles on the PHP Chinese website!
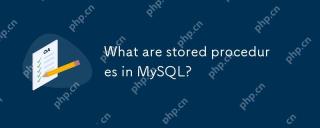
Stored procedures are precompiled SQL statements in MySQL for improving performance and simplifying complex operations. 1. Improve performance: After the first compilation, subsequent calls do not need to be recompiled. 2. Improve security: Restrict data table access through permission control. 3. Simplify complex operations: combine multiple SQL statements to simplify application layer logic.
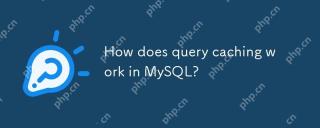
The working principle of MySQL query cache is to store the results of SELECT query, and when the same query is executed again, the cached results are directly returned. 1) Query cache improves database reading performance and finds cached results through hash values. 2) Simple configuration, set query_cache_type and query_cache_size in MySQL configuration file. 3) Use the SQL_NO_CACHE keyword to disable the cache of specific queries. 4) In high-frequency update environments, query cache may cause performance bottlenecks and needs to be optimized for use through monitoring and adjustment of parameters.
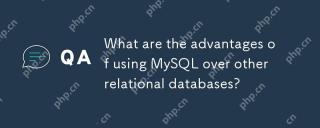
The reasons why MySQL is widely used in various projects include: 1. High performance and scalability, supporting multiple storage engines; 2. Easy to use and maintain, simple configuration and rich tools; 3. Rich ecosystem, attracting a large number of community and third-party tool support; 4. Cross-platform support, suitable for multiple operating systems.
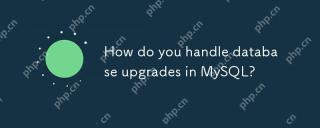
The steps for upgrading MySQL database include: 1. Backup the database, 2. Stop the current MySQL service, 3. Install the new version of MySQL, 4. Start the new version of MySQL service, 5. Recover the database. Compatibility issues are required during the upgrade process, and advanced tools such as PerconaToolkit can be used for testing and optimization.
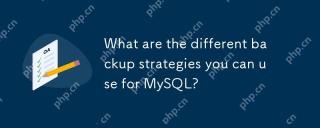
MySQL backup policies include logical backup, physical backup, incremental backup, replication-based backup, and cloud backup. 1. Logical backup uses mysqldump to export database structure and data, which is suitable for small databases and version migrations. 2. Physical backups are fast and comprehensive by copying data files, but require database consistency. 3. Incremental backup uses binary logging to record changes, which is suitable for large databases. 4. Replication-based backup reduces the impact on the production system by backing up from the server. 5. Cloud backups such as AmazonRDS provide automation solutions, but costs and control need to be considered. When selecting a policy, database size, downtime tolerance, recovery time, and recovery point goals should be considered.
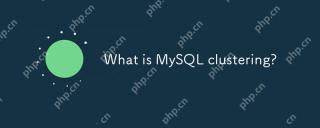
MySQLclusteringenhancesdatabaserobustnessandscalabilitybydistributingdataacrossmultiplenodes.ItusestheNDBenginefordatareplicationandfaulttolerance,ensuringhighavailability.Setupinvolvesconfiguringmanagement,data,andSQLnodes,withcarefulmonitoringandpe
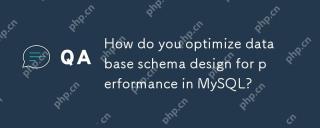
Optimizing database schema design in MySQL can improve performance through the following steps: 1. Index optimization: Create indexes on common query columns, balancing the overhead of query and inserting updates. 2. Table structure optimization: Reduce data redundancy through normalization or anti-normalization and improve access efficiency. 3. Data type selection: Use appropriate data types, such as INT instead of VARCHAR, to reduce storage space. 4. Partitioning and sub-table: For large data volumes, use partitioning and sub-table to disperse data to improve query and maintenance efficiency.
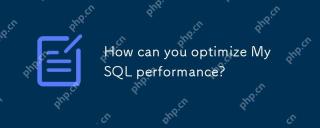
TooptimizeMySQLperformance,followthesesteps:1)Implementproperindexingtospeedupqueries,2)UseEXPLAINtoanalyzeandoptimizequeryperformance,3)Adjustserverconfigurationsettingslikeinnodb_buffer_pool_sizeandmax_connections,4)Usepartitioningforlargetablestoi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
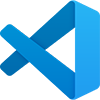
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
