Introduction
Need to remove a specific item from a Python list, identified by its position (index)? The built-in pop()
method is your solution. This function efficiently removes an element at a given index and conveniently returns the removed value, providing fine-grained control over your list. Whether you're working with dynamic lists, processing user input, or manipulating data structures, mastering pop()
streamlines your code. Let's delve into its capabilities.
Key Learning Points
- Grasp the purpose and syntax of Python's
pop()
method. - Master removing list elements using
pop()
. - Utilize the index parameter in
pop()
for targeted element removal. - Implement robust error handling when using
pop()
. - Apply
pop()
effectively in diverse coding scenarios.
Table of Contents
- Understanding Python's
pop()
Method - How
pop()
Functions - Negative Indexing with
pop()
-
pop()
and Python Dictionaries - Memory Implications of
pop()
- Performance of
pop()
- Comparing
pop()
andremove()
- Frequently Asked Questions
Understanding Python's pop()
Method
The pop()
method in Python removes an element from a list at a specified index, returning the removed element's value. Unlike remove()
, which requires the element's value, pop()
uses indices, offering precise control over element deletion.
Syntax:
list.pop(index)
-
list
: The target list. -
index
(optional): The index of the element to remove. Omittingindex
removes the last element.
How pop()
Functions
pop()
modifies the list directly (in-place) and returns the removed item. Its behavior varies depending on index specification:
Removing by Index
Specifying an index
removes the element at that position. The remaining elements shift to fill the gap. The removed element is returned.
Mechanism:
- The specified index locates the element.
- The element is removed.
- Subsequent elements shift left.
- The removed element is returned.
Example:
my_list = ['apple', 'banana', 'cherry', 'date'] removed_item = my_list.pop(1) # Removes 'banana' print(removed_item) # Output: banana print(my_list) # Output: ['apple', 'cherry', 'date']
Removing the Last Element
Omitting the index
removes and returns the last element. This is efficient as no element shifting is needed.
Mechanism:
- The last element is identified.
- The element is removed.
- The removed element is returned.
Example:
my_list = [10, 20, 30, 40] removed_item = my_list.pop() # Removes 40 print(removed_item) # Output: 40 print(my_list) # Output: [10, 20, 30]
IndexError
Handling
Attempting to pop()
from an empty list or using an invalid index raises an IndexError
.
-
Empty List:
empty_list.pop()
raisesIndexError: pop from empty list
. -
Invalid Index:
my_list.pop(10)
(ifmy_list
has fewer than 10 elements) raisesIndexError: pop index out of range
.
Negative Indexing with pop()
Python supports negative indexing (counting backward from the end). pop()
works with negative indices: pop(-1)
removes the last element, pop(-2)
the second-to-last, and so on.
Example:
my_list = [100, 200, 300, 400] removed_item = my_list.pop(-2) # Removes 300 print(removed_item) # Output: 300 print(my_list) # Output: [100, 200, 400]
pop()
and Python Dictionaries
pop()
also functions with dictionaries. It removes a key-value pair based on the key and returns the associated value.
Examples:
student = {'name': 'John', 'age': 25, 'course': 'Mathematics'} age = student.pop('age') print(age) # Output: 25 print(student) # Output: {'name': 'John', 'course': 'Mathematics'} # Handling missing keys with a default value: major = student.pop('major', 'Unknown') print(major) # Output: Unknown
Attempting to pop()
a non-existent key without a default value raises a KeyError
.
Memory Implications of pop()
pop()
affects memory due to Python lists' dynamic array nature. Removing a non-last element requires shifting subsequent elements, impacting performance, especially with large lists. Removing the last element is efficient (O(1)).
Performance of pop()
pop()
's efficiency depends on the index:
- Best Case (O(1)): Removing the last element (no index specified).
- Worst Case (O(n)): Removing the first element (index 0).
- Intermediate Cases (O(n)): Removing elements from the middle.
Comparing pop()
and remove()
Both remove elements, but differ significantly:
Feature |
pop() Method |
remove() Method |
---|---|---|
Action | Removes by index, returns removed element | Removes by value, no return value |
Index/Value | Uses index | Uses value |
Return Value | Returns removed element | None |
Error Handling |
IndexError for invalid index or empty list |
ValueError if value not found |
Conclusion
pop()
is a versatile tool for list manipulation, offering precise control over element removal and value retrieval. Understanding its behavior, efficiency, and potential errors ensures efficient and robust code.
Frequently Asked Questions
Q1. What if I omit the index in pop()
? It removes and returns the last element.
Q2. Can I use pop()
on an empty list? No, it raises an IndexError
.
Q3. How does pop()
handle negative indices? It removes elements from the end, counting backward.
Q4. Can pop()
be used with strings or tuples? No, only with lists.
Q5. Does pop()
remove all occurrences of an element? No, only the element at the specified index.
The above is the detailed content of Understanding Python pop() Method. For more information, please follow other related articles on the PHP Chinese website!
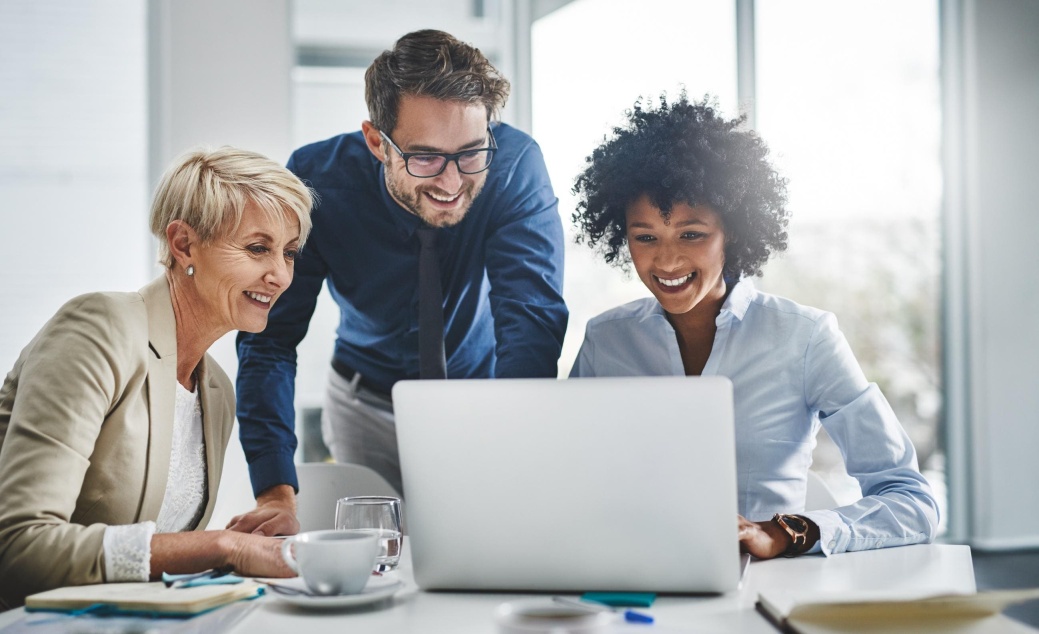
For those of you who might be new to my column, I broadly explore the latest advances in AI across the board, including topics such as embodied AI, AI reasoning, high-tech breakthroughs in AI, prompt engineering, training of AI, fielding of AI, AI re
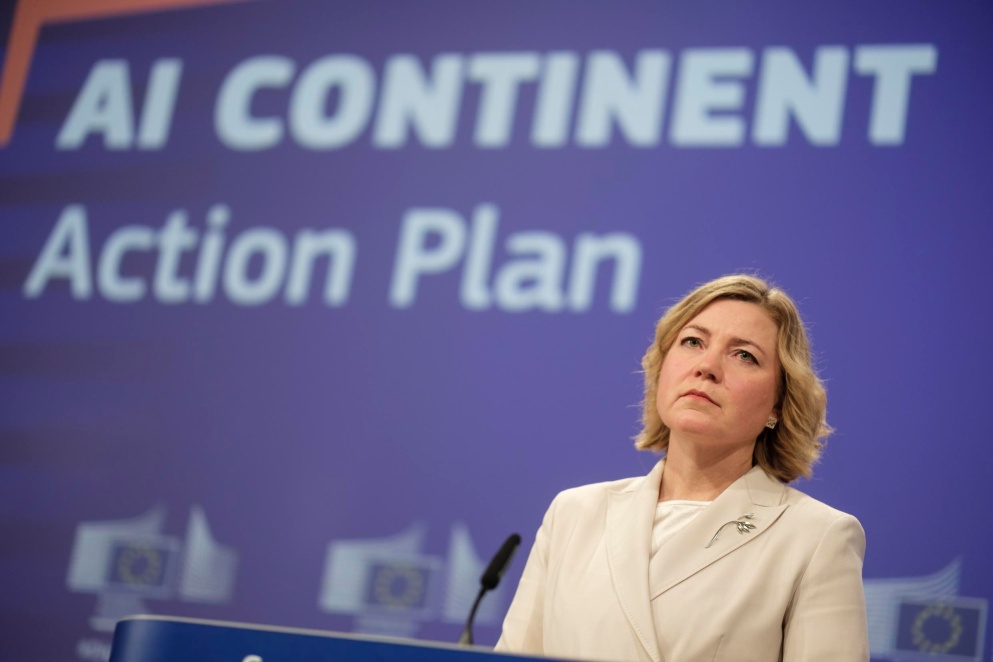
Europe's ambitious AI Continent Action Plan aims to establish the EU as a global leader in artificial intelligence. A key element is the creation of a network of AI gigafactories, each housing around 100,000 advanced AI chips – four times the capaci
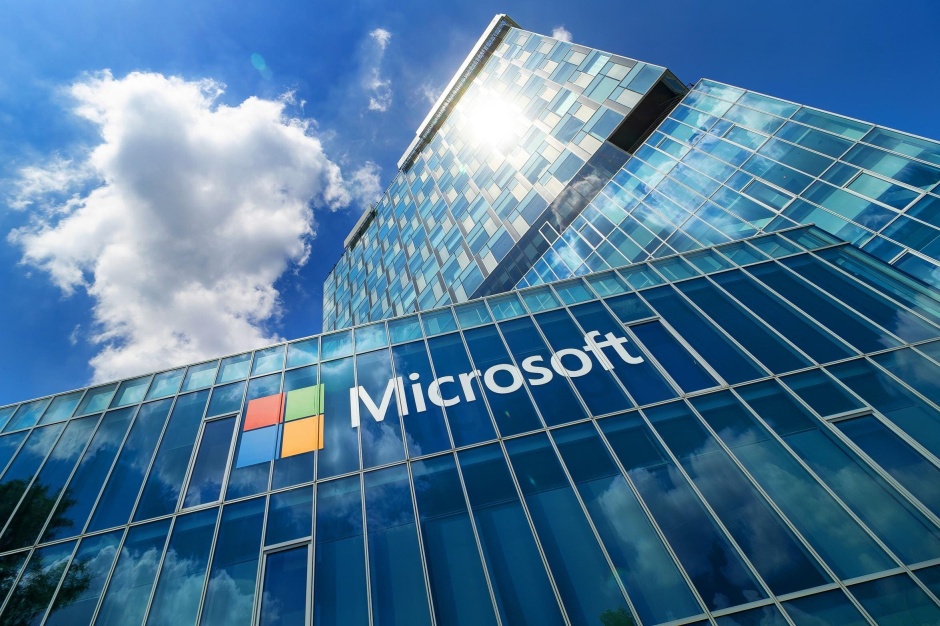
Microsoft's Unified Approach to AI Agent Applications: A Clear Win for Businesses Microsoft's recent announcement regarding new AI agent capabilities impressed with its clear and unified presentation. Unlike many tech announcements bogged down in te
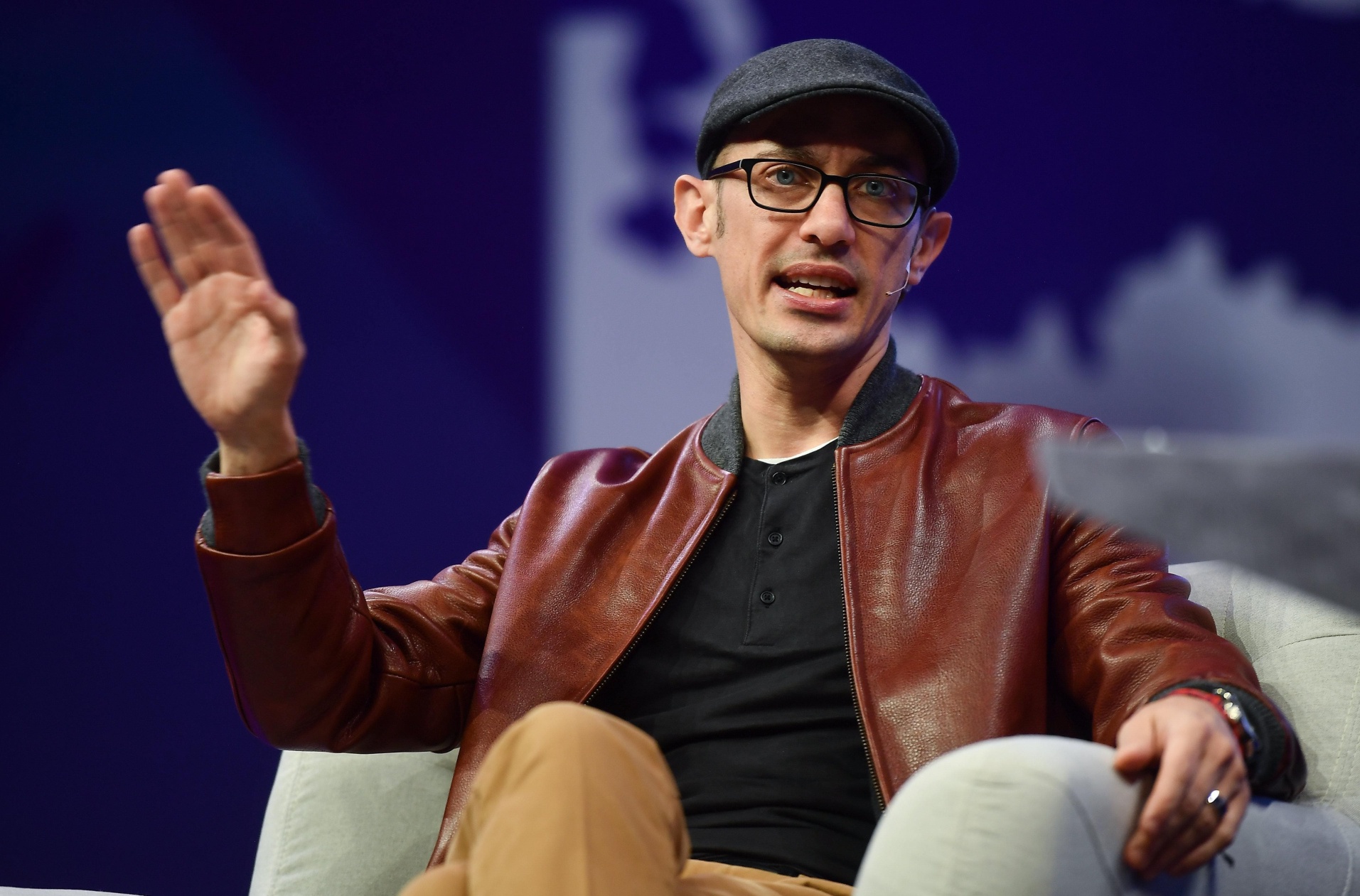
Shopify CEO Tobi Lütke's recent memo boldly declares AI proficiency a fundamental expectation for every employee, marking a significant cultural shift within the company. This isn't a fleeting trend; it's a new operational paradigm integrated into p
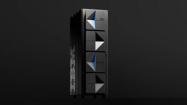
IBM's z17 Mainframe: Integrating AI for Enhanced Business Operations Last month, at IBM's New York headquarters, I received a preview of the z17's capabilities. Building on the z16's success (launched in 2022 and demonstrating sustained revenue grow
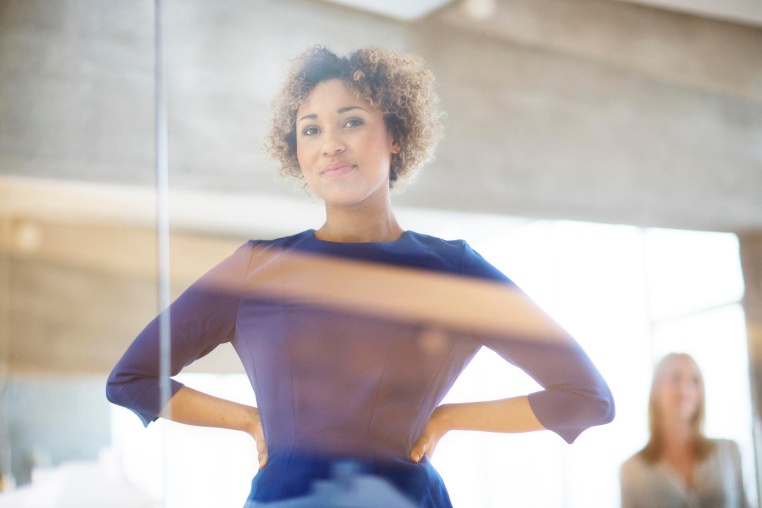
Unlock unshakeable confidence and eliminate the need for external validation! These five ChatGPT prompts will guide you towards complete self-reliance and a transformative shift in self-perception. Simply copy, paste, and customize the bracketed in
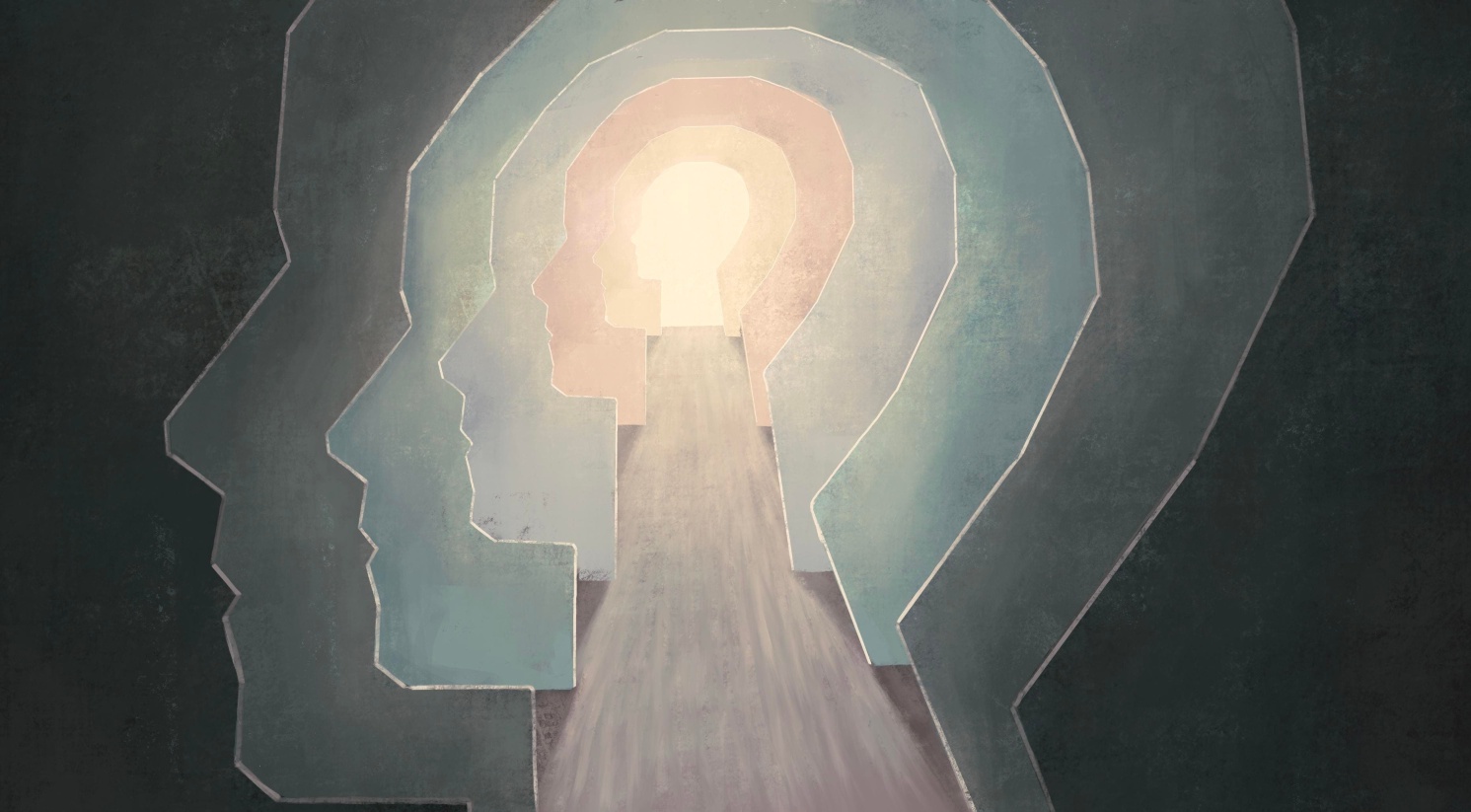
A recent [study] by Anthropic, an artificial intelligence security and research company, begins to reveal the truth about these complex processes, showing a complexity that is disturbingly similar to our own cognitive domain. Natural intelligence and artificial intelligence may be more similar than we think. Snooping inside: Anthropic Interpretability Study The new findings from the research conducted by Anthropic represent significant advances in the field of mechanistic interpretability, which aims to reverse engineer internal computing of AI—not just observe what AI does, but understand how it does it at the artificial neuron level. Imagine trying to understand the brain by drawing which neurons fire when someone sees a specific object or thinks about a specific idea. A
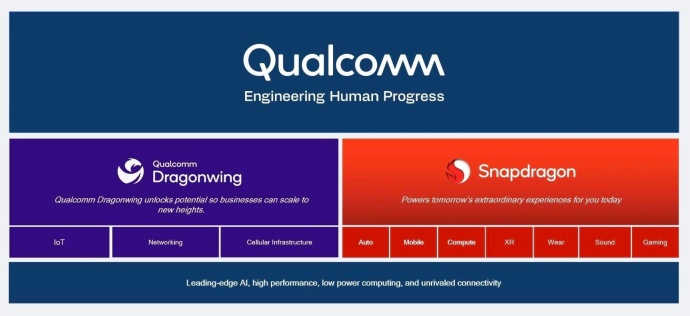
Qualcomm's Dragonwing: A Strategic Leap into Enterprise and Infrastructure Qualcomm is aggressively expanding its reach beyond mobile, targeting enterprise and infrastructure markets globally with its new Dragonwing brand. This isn't merely a rebran


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor
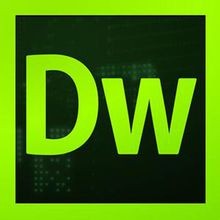
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use