List comprehension is a concise syntax in Python for creating new lists. 1) It generates lists through expressions and iterable objects, and the code is concise and efficient. 2) The working principle includes iteration, expressions and optional filtering steps. 3) The basic usage is simple and intuitive, while the advanced usage can handle complex logic. 4) Common errors include syntax and logic errors, which can be solved by step-by-step debugging and printing intermediate results. 5) It is recommended to use generator expressions for performance optimization to avoid overcomplexity and pay attention to code readability.
introduction
In the film industry, the word "premiere" always sounds so dazzling and full of expectations. What we are going to discuss today is not movies, but "premiere" in programming - a list comprehension in Python. List comprehension is a very powerful feature in the Python language, which can make the code more concise and efficient. This article will take you into the mystery of list comprehension, from basic to advanced usage, to performance optimization and best practices, ensuring you can master this technique and use it flexibly in actual development.
Review of basic knowledge
To understand list comprehension, you first need to review the list and iterators in Python. Lists are one of the most commonly used data structures in Python, which can store a series of ordered elements. The iterator allows us to iterate through these elements and perform various operations. List comprehension is based on this mechanism, providing a concise way to generate new lists.
Core concept or function analysis
Definition and function of list comprehension
List Comprehension is a concise syntax for creating new lists. Its function is to generate a new list through an expression, combined with an iterable object. The advantages are that the code is concise, readable, and the execution efficiency is usually relatively high.
A simple example is as follows:
# Generate a list of squares of 1 to 10 squares = [x**2 for x in range(1, 11)] print(squares) # Output: [1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
How it works
The working principle of list comprehension can be decomposed into the following steps:
- Iteration : Iterate over an iterable object (such as lists, ranges, etc.).
- Expression : Apply an expression to each element to generate a new value.
- Filter (optional): Filter elements according to conditions, only elements that meet the conditions will be added to the new list.
For example:
# Generate a list of even numbers from 1 to 10 even_numbers = [x for x in range(1, 11) if x % 2 == 0] print(even_numbers) # Output: [2, 4, 6, 8, 10]
In implementation, the list comprehension actually creates a new list object and adds the generated elements to it in turn. It is worth noting that list comprehensions are lazy to evaluate and only calculate the results when needed, which can improve performance in some cases.
Example of usage
Basic usage
The basic usage of list comprehension is very simple and intuitive. Here is a simple example:
# Generate a list of 1 to 10 numbers = [x for x in range(1, 11)] print(numbers) # Output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Advanced Usage
List comprehensions can also handle more complex logic, such as nested loops and multi-conditional filtering. Here is a more complex example:
# Generate a combination of all possible (a, b, c) such that a^2 b^2 = c^2, and a, b, c are between 1 and 20 pythagorean_triples = [(a, b, c) for a in range(1, 21) for b in range(1, 21) for c in range(1, 21) if a**2 b**2 == c**2] print(pythagorean_triples) # output: [(3, 4, 5), (4, 3, 5), (5, 12, 13), (6, 8, 10), (8, 6, 10), (12, 5, 13), (15, 8, 17), (8, 15, 17), (17, 8, 15), (9, 12, 15), (12, 9, 15), (10, 24, 26), (24, 10, 26), (20, 21, 29), (21, 20, 29)]
Common Errors and Debugging Tips
Common errors when using list comprehensions include syntax and logical errors. For example:
- Syntax error : Forgot to use square brackets, or use incorrect syntax in the expression.
- Logical error : The conditional filtering is incorrect, resulting in the generated list not meeting expectations.
Debugging Tips:
- Step-by-step debugging : Split the list comprehension into multiple steps to gradually verify the correctness of each part.
- Print intermediate results : Add print statements to the list comprehension to check whether the intermediate results meet expectations.
Performance optimization and best practices
List comprehensions are generally better than traditional for loops in performance because they are closer to Python's internal implementation. However, in some cases, list comprehension may cause memory usage to increase, as it generates the entire list at once.
Here are some performance optimizations and best practices:
- Use generator expressions : If you don't need to generate the entire list at once, you can use generator expressions to save memory. For example:
# Use generator expression to generate squares_gen from 1 to 10 = (x**2 for x in range(1, 11)) for square in squares_gen: print(square)
Avoid overly complex list comprehensions : Although list comprehensions can handle complex logic, overly complex expressions can reduce readability and maintainability. If necessary, you can consider using traditional for loops or functional programming methods.
Code readability : Make sure the list comprehension is concise and clear, avoiding too deep nesting or using too complex expressions. Good naming and commenting can greatly improve the readability of the code.
In actual development, list comprehension is a very useful tool, but it is necessary to choose the most appropriate implementation method according to the specific situation. Through the study of this article, you should be able to flexibly use list comprehension in your code to improve the simplicity and efficiency of your code.
The above is the detailed content of What does a premiere do?. For more information, please follow other related articles on the PHP Chinese website!

Adobe PremierePro can handle various video formats, including MP4, AVI, ProRes, DNxHD, etc. 1) It supports a variety of codecs, making it easy to import and output videos. 2) Use the SDK to programmatically control the import and output process. 3) Media browser helps preview and select formats. 4) Proxy files can optimize high-resolution video processing. 5) Keeping files organized and regularly backed up are best practices for handling different formats.

Premierescelebratecreativityacrossvariousfields,notjustmovies.1)Moviepremieresfeatureredcarpetsandpress.2)Theateropeningsareintimatewithcast-audienceinteractions.3)TVserieslaunchesengagefanscommunally.4)Musicalbumunveilsofferunique,immersiveexperienc

AdobePremiereProintegratesseamlesslywithAdobeCreativeCloudapplications,enhancingproductivityandcreativeworkflow.1)UseDynamicLinktoworkbetweenPremiereProandAfterEffectswithoutrendering.2)EditimagesdirectlyinPhotoshopwithchangesreflectingbackinPremiere

AdobePremiereProalternativesincludeDaVinciResolve,FinalCutProX,HitFilmExpress,Lightworks,andShotcut.1)DaVinciResolveexcelsincolorgradingwitharobustfreeversion.2)FinalCutProXisidealforMacuserswithitsmagnetictimeline.3)HitFilmExpressisgreatforvisualeff

Theterm"premiere"insoftwaredevelopmentreferstothefirstreleaseordeploymentofasoftwareapplication.Preparingforasoftwarepremiereinvolves:1)Thoroughtesting,includingunit,integration,anduseracceptancetestingtoensurefunctionality;2)Performanceopt

A"premiere"isthefirstpublicshowingofaproduct,whilea"release"makesitavailabletothegeneralpublic.1)Apremieregeneratesbuzzandinitialfeedback.2)Areleasefollowstestingandrefinement,makingtheproductwidelyaccessible.

AdobePremiereProiswidelyusedinthefilmindustryforeditingandpost-production.Itsupportsbasiccutstocomplexeffects,integrateswithotherAdobetools,offerscollaborativefeatures,andrequiresoptimizationforlargeprojects.

In Python, methods that "premiere" a function or class include definition, parameter handling, error handling, and performance optimization. 1. Define a simple function, such as premiere_function, to show the basic functions. 2. Add parameters and error handling, such as premiere_function_with_params, to ensure the input is correct. 3. Optimize performance, such as optimized_premiere_function_with_params, use type annotations and f-string to improve efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
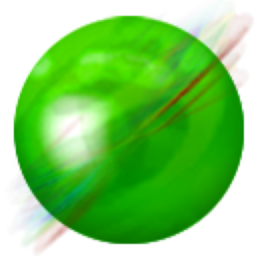
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
