


Docker with Kubernetes: Container Orchestration for Enterprise Applications
How to use Docker and Kubernetes to orchestrate containers for enterprise applications? Implement it by creating a Docker image and pushing it to the Docker Hub. Create a Deployment and Service in Kubernetes to deploy the application. Use Ingress to manage external access. Apply performance optimization and best practices such as multi-stage construction and resource constraints.
introduction
In modern enterprise application development, containerization technology has become an indispensable part, and Docker and Kubernetes are undoubtedly the two giants in this field. Today we are going to explore how to use Docker and Kubernetes to perform container orchestration of enterprise applications. Through this article, you will learn how to build an efficient, scalable containerized application environment from scratch and master some practical tips and best practices.
Review of basic knowledge
Docker is an open source containerized platform that allows developers to package applications and their dependencies into a portable container, thus simplifying application deployment and management. Kubernetes (K8s for short) is an open source container orchestration system that can automatically deploy, scale and manage containerized applications.
Before using Docker and Kubernetes, it is necessary to understand some basic concepts, such as containers, mirrors, pods, service, etc. These concepts are the basis for understanding and using these two tools.
Core concept or function analysis
The definition and function of Docker and Kubernetes
Docker packages applications and their dependencies into a separate unit through container technology, allowing applications to run in any Docker-enabled environment. This greatly simplifies the deployment and migration process of applications. Kubernetes provides higher-level abstraction and automation management functions based on Docker containers. It manages hundreds of containers, ensuring high availability and scalability of applications.
A simple Docker example:
# Build a simple Docker image docker build -t myapp:v1. # Run Docker container docker run -d -p 8080:80 myapp:v1
One of the basic concepts of Kubernetes is a Pod, which is the smallest deployable unit, usually containing one or more containers. Here is a simple Kubernetes Pod definition file:
apiVersion: v1 kind: Pod metadata: name: myapp-pod spec: containers: - name: myapp-container image: myapp:v1 Ports: - containerPort: 80
How it works
The working principle of Docker is mainly to implement container isolation and resource management through the namespace and control groups of the Linux kernel. The Docker image is a read-only template that contains the application and its dependencies. The container is a writable layer started from the image and runs on the Docker engine.
Kubernetes works more complexly, and it manages the life cycle of a Pod through a series of controllers and schedulers. The core components of Kubernetes include API Server, Controller Manager, Scheduler, etcd, etc. API Server is responsible for handling API requests, Controller Manager is responsible for running the controller, Scheduler is responsible for scheduling the Pods to the appropriate node, and etcd is a distributed key-value store that saves the state of the cluster.
When using Kubernetes, it is important to note its complexity and learning curve. Beginners may find the concepts and configuration files of Kubernetes difficult to understand, but once you master these basics, you can take advantage of the power of Kubernetes.
Example of usage
Basic usage
Let's start with a simple example showing how to deploy a basic web application using Docker and Kubernetes.
First, we need to create a Docker image:
FROM nginx:alpine COPY index.html /usr/share/nginx/html
Then, build and push the image to Docker Hub:
docker build -t mywebapp:v1 . docker push mywebapp:v1
Next, create a Deployment and Service in Kubernetes:
apiVersion: apps/v1 kind: Deployment metadata: name: mywebapp spec: replicas: 3 selector: matchLabels: app: mywebapp template: metadata: labels: app: mywebapp spec: containers: - name: mywebapp image: mywebapp:v1 Ports: - containerPort: 80 --- apiVersion: v1 kind: Service metadata: name: mywebapp-service spec: selector: app: mywebapp Ports: - protocol: TCP port: 80 targetPort: 80 type: LoadBalancer
Advanced Usage
In practice, we may need more complex configurations, such as using ConfigMap and Secret to manage configuration and sensitive information, or using Ingress to manage external access. Here is an example of using Ingress:
apiVersion: networking.k8s.io/v1 kind: Ingress metadata: name: mywebapp-ingress spec: Rules: - host: mywebapp.example.com http: paths: - path: / pathType: Prefix backend: service: name: mywebapp-service port: number: 80
Common Errors and Debugging Tips
When using Docker and Kubernetes, you may encounter some common problems, such as image pull failure, Pod startup failure, etc. Here are some debugging tips:
- Use
docker logs
to view container logs to help diagnose problems. - Use
kubectl describe pod
to view the details of the pod, including events and status. - Use
kubectl logs
to view container logs in the Pod.
Performance optimization and best practices
In practical applications, how to optimize the performance of Docker and Kubernetes is a key issue. Here are some suggestions:
- Use multi-stage builds to reduce image size, thus speeding up image pulling and deployment.
- Use resource constraints and requests to ensure that the Pod does not over-consuming node resources.
- Use Horizontal Pod Autoscaler (HPA) to automatically scale Pods to cope with traffic changes.
It is also very important to keep the code readable and maintainable when writing Dockerfile and Kubernetes configuration files. Here are some best practices:
- Use the
.dockerignore
file in the Dockerfile to exclude unnecessary files. - Use comments and tags in Kubernetes configuration files to improve readability.
- Use tools such as Helm or Kustomize to manage and reuse Kubernetes configurations.
Overall, Docker and Kubernetes provide powerful tools to manage and deploy enterprise applications. Through the introduction and examples of this article, you should have mastered how to use these two tools to build an efficient and scalable containerized application environment. Hopefully these knowledge and skills will work in your actual project.
The above is the detailed content of Docker with Kubernetes: Container Orchestration for Enterprise Applications. For more information, please follow other related articles on the PHP Chinese website!

Best practices for using Docker on Linux include: 1. Create and run containers using dockerrun commands, 2. Use DockerCompose to manage multi-container applications, 3. Regularly clean unused images and containers, 4. Use multi-stage construction to optimize image size, 5. Limit container resource usage to improve security, and 6. Follow Dockerfile best practices to improve readability and maintenance. These practices can help users use Docker efficiently, avoid common problems and optimize containerized applications.

Using Docker on Linux can improve development and deployment efficiency. 1. Install Docker: Use scripts to install Docker on Ubuntu. 2. Verify the installation: Run sudodockerrunhello-world. 3. Basic usage: Create an Nginx container dockerrun-namemy-nginx-p8080:80-dnginx. 4. Advanced usage: Create a custom image, build and run using Dockerfile. 5. Optimization and Best Practices: Follow best practices for writing Dockerfiles using multi-stage builds and DockerCompose.

The core of Docker monitoring is to collect and analyze the operating data of containers, mainly including indicators such as CPU usage, memory usage, network traffic and disk I/O. By using tools such as Prometheus, Grafana and cAdvisor, comprehensive monitoring and performance optimization of containers can be achieved.

DockerSwarm can be used to build scalable and highly available container clusters. 1) Initialize the Swarm cluster using dockerswarminit. 2) Join the Swarm cluster to use dockerswarmjoin--token:. 3) Create a service using dockerservicecreate-namemy-nginx--replicas3nginx. 4) Deploy complex services using dockerstackdeploy-cdocker-compose.ymlmyapp.

How to use Docker and Kubernetes to perform container orchestration of enterprise applications? Implement it through the following steps: Create a Docker image and push it to DockerHub. Create Deployment and Service in Kubernetes to deploy applications. Use Ingress to manage external access. Apply performance optimization and best practices such as multi-stage construction and resource constraints.

Docker FAQs can be diagnosed and solved through the following steps: 1. View container status and logs, 2. Check network configuration, 3. Ensure that the volume mounts correctly. Through these methods, problems in Docker can be quickly located and fixed, improving system stability and performance.

Docker is a must-have skill for DevOps engineers. 1.Docker is an open source containerized platform that achieves isolation and portability by packaging applications and their dependencies into containers. 2. Docker works with namespaces, control groups and federated file systems. 3. Basic usage includes creating, running and managing containers. 4. Advanced usage includes using DockerCompose to manage multi-container applications. 5. Common errors include container failure, port mapping problems, and data persistence problems. Debugging skills include viewing logs, entering containers, and viewing detailed information. 6. Performance optimization and best practices include image optimization, resource constraints, network optimization and best practices for using Dockerfile.

Docker security enhancement methods include: 1. Use the --cap-drop parameter to limit Linux capabilities, 2. Create read-only containers, 3. Set SELinux tags. These strategies protect containers by reducing vulnerability exposure and limiting attacker capabilities.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
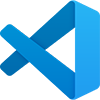
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
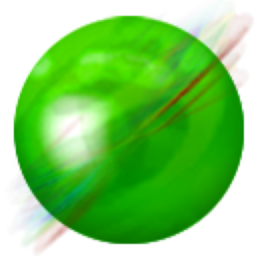
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
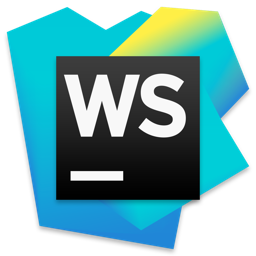
WebStorm Mac version
Useful JavaScript development tools