What are prefix indexes in MySQL and when are they useful/problematic?
Prefix indexing is a tool in MySQL used to optimize query performance, reducing the index size by indexing the first N characters of a string field. When using prefix indexes, you need to pay attention to: 1. Select the appropriate prefix length, 2. Avoid query conditions involving the middle or back characters of the string, 3. Use in combination with other index types, 4. Regularly monitor and adjust the index strategy.
introduction
Prefix indexes in MySQL are a powerful tool to optimize query performance. Understanding them can not only improve the query speed of the database, but also avoid some common pitfalls. In this article, we will dive into the concept of prefix indexing, usage scenarios, and possible problems. After reading this article, you will learn how to effectively use prefix indexes in real projects and learn how to avoid possible risks.
Review of basic knowledge
In MySQL, indexing is an important tool to speed up data retrieval. Common index types include B-Tree index, full-text index, etc., while prefix index is a special index for string type fields. Prefix indexes improve query performance by indexing the first few characters of a field, rather than indexing the entire field.
For example, suppose we have a table containing user names. If we only need to query based on the first few characters of the name, using prefix indexes can significantly reduce the size of the index, thereby improving query efficiency.
Core concept or function analysis
Definition and function of prefix index
Prefix index refers to indexing the first N characters of a string type field, rather than indexing the entire field. This indexing method can greatly reduce the size of the index, especially when dealing with long strings. For example, for a VARCHAR field with a length of 255, we can choose to index only the first 10 characters.
CREATE INDEX idx_name ON users (name(10));
This index will index the first 10 characters of the name
field, so that only the prefix part of the index is scanned when querying.
How it works
The prefix index works in that it improves query performance by reducing the length of the index. When we execute a query, MySQL will first look for the matching prefix in the index tree, and then find the corresponding complete record based on the prefix. This method reduces the size of the index, thereby improving query speed.
However, prefix indexing also has some limitations. Because only indexing prefixes, some queries may not be able to fully utilize indexes. For example, if the query condition involves the middle or back characters of a string, the prefix index may not work. Additionally, prefix indexing may result in reduced selectivity, as multiple different strings may have the same prefixes.
Example of usage
Basic usage
Suppose we have a users
table with the name
field, and we want to query based on the first few characters of the name:
CREATE TABLE users ( id INT PRIMARY KEY, name VARCHAR(255) ); CREATE INDEX idx_name ON users (name(10)); SELECT * FROM users WHERE name LIKE 'John%';
In this example, the prefix index idx_name
will index the first 10 characters of the name
field, thus speeding up queries like LIKE 'John%'
.
Advanced Usage
In some cases, we may need to establish a prefix index on multiple fields, or use a prefix index in conjunction with other index types. For example, suppose we have an articles
table containing title
and content
fields, we can index the first 10 characters of title
and the first 20 characters of content
at the same time:
CREATE TABLE articles ( id INT PRIMARY KEY, title VARCHAR(255), content TEXT ); CREATE INDEX idx_title ON articles (title(10)); CREATE INDEX idx_content ON articles (content(20)); SELECT * FROM articles WHERE title LIKE 'Hello%' AND content LIKE '%world%';
In this example, prefix indexing can help speed up queries to title
and content
fields, but it should be noted that queries like LIKE '%world%'
may not fully utilize prefix indexing because it involves the back of the string.
Common Errors and Debugging Tips
Common errors when using prefix indexes include choosing an inappropriate prefix length or using a prefix index in an inappropriate scenario. Here are some common problems and solutions:
- Improper selection of prefix length : The selected prefix length is too short, resulting in insufficient selectivity of the index and inability to effectively speed up the query. The solution is to analyze the data distribution and select an appropriate prefix length.
- Unsuitable query conditions : If the query conditions involve the middle or back characters of the string, the prefix index may not work. The solution is to consider using full-text indexes or other index types, or redesign query conditions.
Performance optimization and best practices
In practical applications, how to optimize the use of prefix indexes is a question worth discussing in depth. Here are some optimization suggestions and best practices:
- Selecting the appropriate prefix length : By analyzing the data distribution and selecting a suitable prefix length can not only ensure the selectivity of the index, but also reduce the size of the index. For example, the following query can be used to analyze the selectivity of prefix length:
SELECT COUNT(DISTINCT LEFT(name, 10)) / COUNT(*) AS selection FROM users;
Combined with other index types : In some cases, prefix indexes can be used in conjunction with other index types. For example, you can create both prefix and full-text indexes on
name
field to meet different query needs.Monitor and adjust : Regularly monitor the use of prefix indexes, and adjust the prefix length or index strategy based on actual query performance. The query plan can be analyzed through
EXPLAIN
statement to determine whether the prefix index is used effectively.Code readability and maintenance : Ensure the readability and maintenance of the code when using prefix indexes. Clearly comment on the reasons for the use of indexes and the basis for selection of prefix lengths for subsequent maintenance and optimization.
In short, prefix indexing is a powerful tool in MySQL, but it needs to be used with caution and optimized in combination with actual requirements and data distribution. Through the introduction and examples of this article, I hope you can better understand and apply prefix indexing and improve database query performance.
The above is the detailed content of What are prefix indexes in MySQL and when are they useful/problematic?. For more information, please follow other related articles on the PHP Chinese website!
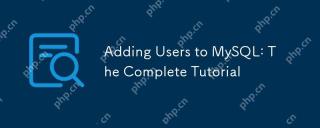
Mastering the method of adding MySQL users is crucial for database administrators and developers because it ensures the security and access control of the database. 1) Create a new user using the CREATEUSER command, 2) Assign permissions through the GRANT command, 3) Use FLUSHPRIVILEGES to ensure permissions take effect, 4) Regularly audit and clean user accounts to maintain performance and security.
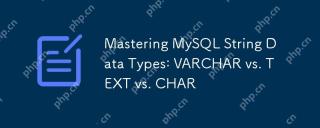
ChooseCHARforfixed-lengthdata,VARCHARforvariable-lengthdata,andTEXTforlargetextfields.1)CHARisefficientforconsistent-lengthdatalikecodes.2)VARCHARsuitsvariable-lengthdatalikenames,balancingflexibilityandperformance.3)TEXTisidealforlargetextslikeartic
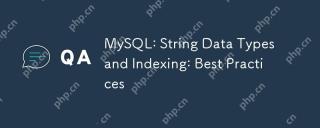
Best practices for handling string data types and indexes in MySQL include: 1) Selecting the appropriate string type, such as CHAR for fixed length, VARCHAR for variable length, and TEXT for large text; 2) Be cautious in indexing, avoid over-indexing, and create indexes for common queries; 3) Use prefix indexes and full-text indexes to optimize long string searches; 4) Regularly monitor and optimize indexes to keep indexes small and efficient. Through these methods, we can balance read and write performance and improve database efficiency.
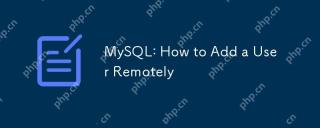
ToaddauserremotelytoMySQL,followthesesteps:1)ConnecttoMySQLasroot,2)Createanewuserwithremoteaccess,3)Grantnecessaryprivileges,and4)Flushprivileges.BecautiousofsecurityrisksbylimitingprivilegesandaccesstospecificIPs,ensuringstrongpasswords,andmonitori
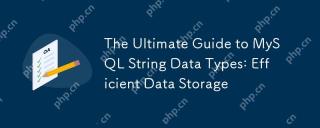
TostorestringsefficientlyinMySQL,choosetherightdatatypebasedonyourneeds:1)UseCHARforfixed-lengthstringslikecountrycodes.2)UseVARCHARforvariable-lengthstringslikenames.3)UseTEXTforlong-formtextcontent.4)UseBLOBforbinarydatalikeimages.Considerstorageov
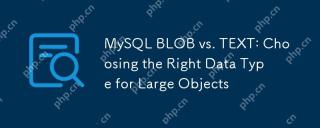
When selecting MySQL's BLOB and TEXT data types, BLOB is suitable for storing binary data, and TEXT is suitable for storing text data. 1) BLOB is suitable for binary data such as pictures and audio, 2) TEXT is suitable for text data such as articles and comments. When choosing, data properties and performance optimization must be considered.
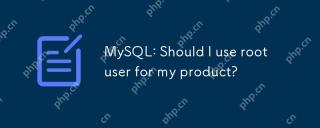
No,youshouldnotusetherootuserinMySQLforyourproduct.Instead,createspecificuserswithlimitedprivilegestoenhancesecurityandperformance:1)Createanewuserwithastrongpassword,2)Grantonlynecessarypermissionstothisuser,3)Regularlyreviewandupdateuserpermissions
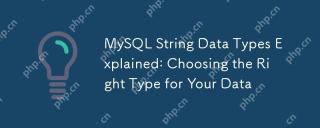
MySQLstringdatatypesshouldbechosenbasedondatacharacteristicsandusecases:1)UseCHARforfixed-lengthstringslikecountrycodes.2)UseVARCHARforvariable-lengthstringslikenames.3)UseBINARYorVARBINARYforbinarydatalikecryptographickeys.4)UseBLOBorTEXTforlargeuns


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
