I developed a Chrome extension this weekend because I found myself performing the same tasks repeatedly and wanting to automate them. In addition, I am a geek who stays at home to survive the epidemic, so I will devote my accumulated energy to creating things. I've developed some Chrome extensions over the years, and I hope this article will help you get started too. Let's get started!
Create a manifest file
The first step is to create a file named manifest.json
and place it in the project folder. Its function is similar to package.json
, which provides important information about the project for the Chrome web app store, including name, version, required permissions, and more. Here is an example:
{ "manifest_version": 2, "name": "Sample name", "version": "1.0.0", "description": "This is an example description", "short_name": "example name abbreviation", "permissions": ["activeTab", "declarativeContent", "storage", "<all_urls> "], "content_scripts": [ { "matches": ["<all_urls> "], "css": ["background.css"], "js": ["background.js"] } ], "browser_action": { "default_title": "Execute an action when executing an action", "default_popup": "popup.html", "default_icon": { "16": "icons/icon16.png", "32": "icons/icon32.png" } } }</all_urls></all_urls>
You may notice some details - first: the name and description can be filled in as you like.
Permissions depend on what the extension needs to perform. In this example, we use ["activeTab", "declarativeContent", "storage", "<all_urls> "]</all_urls>
, because this particular extension requires information about the active tag, needs to change the page content, needs to access localStorage, and needs to be active on all websites. If it needs to be active on only one website, we can delete the last index of that array.
Chrome's extension documentation lists all permissions and their meanings.
"content_scripts": [ { "matches": ["<all_urls> "], "css": ["background.css"], "js": ["background.js"] } ],</all_urls>
content_scripts
section sets the site where the extension should be active. If you want a separate website, such as Twitter, you can write it as ["https://twitter.com/*"]
. CSS and JavaScript files are everything you need for an extension. For example, my efficient Twitter extension uses these files to overwrite the default appearance of Twitter.
"browser_action": { "default_title": "Execute an action when executing an action", "default_popup": "popup.html", "default_icon": { "16": "icons/icon16.png", "32": "icons/icon32.png" } }
Some contents in browser_action
are also optional. For example, if an extension does not require a popup to implement its functionality, you can delete default_title
and default_popup
. In this case, only the extension's icon is needed. If the extension is only valid on certain websites, Chrome grays out the extension's icon when it is inactive.
debug
Once the manifest file, CSS, and JavaScript files are ready, visit chrome://extensions/
in the browser's address bar and enable developer mode. This will activate the Load Decompressed button to add the extension file. You can also switch whether the developer version of the extension is active.
I highly recommend starting a GitHub repository for version control at this point. This is a great way to save your work.
When updating the extension, it needs to be reloaded from this interface. A small refresh icon will be displayed on the screen. Also, if the extension experiences any errors during development, it will also display an error button with stack trace and more information here.
Popup window function
Fortunately, this is very simple if the extension needs to use a popup that pops up from the extension icon. After specifying the file name with browser_action
in the manifest file, you can use any HTML and CSS you like to build the page, including the images (I tend to use inline SVG).
We may need to add some functionality to the popup. This may require some JavaScript, so make sure you specify the JavaScript file in the manifest file and also link it in your popup file as follows:
In this file, first create the function, we will access the popup DOM like this:
document.addEventListener("DOMContentLoaded", () => { var button = document.getElementById("submit"); button.addEventListener("click", (e) => { console.log(e); }); });
If we create a button in the popup.html
file, assign it an ID called submit
, and then return a console log, you may notice that in fact nothing is logged in the console. This is because we are in different contexts, which means we need to right-click on the popup and open a different set of DevTools.
Now we can access logs and debug! But remember that if anything is set in localStorage, it only exists in the extension's DevTools localStorage; not the user's browser localStorage. (I encountered this problem the first time I tried it!)
Run scripts outside the extension
This is all good, but what if we want to run a script that can access the current tab information? Here are a few ways to do this. I usually call a separate function inside the DOMContentLoaded
event listener:
Example 1: Activate the file
function exampleFunction() { chrome.tabs.executeScript(() => { chrome.tabs.executeScript({ file: "content.js" }); }); }
Example 2: Execute only a small amount of code
This approach is great if you only need to run a small amount of code. However, since it requires passing everything as a string or template literal, it quickly becomes difficult to handle.
function exampleFunction() { chrome.tabs.executeScript({ code: `console.log('hi there');` }); }
Example 3: Activate the file and pass parameters
Remember that extensions and tabs run in different contexts. This makes passing parameters between them not easy. We will nest the first two examples here, passing some code into the second file. I store everything I need in one option, but in order for it to work we will have to stringify the object.
function exampleFunction(options) { chrome.tabs.executeScript( { code: "var options = " JSON.stringify(options) }, function() { chrome.tabs.executeScript({ file: "content.js" }); } ); }
icon
Even though the manifest file only defines two icons, we need two more to formally submit the extension to the Chrome Web Store: a 128-pixel square icon, and an icon I call icon128_proper.png
, which is also 128 pixels, but has some padding between the edges of the image and the icon.
Remember that no matter which icon is used, it needs to look good in the browser's light and dark modes. I usually find my icon on the Noun Project.
Submit to Chrome Web App Store
Now we can go to the Chrome Web Store Developer Console to submit the extension! Click the New Project button and drag and drop the compressed project file into the uploader.
Chrome will ask some questions about extensions here and ask for information about the permissions requested in the extension and why those permissions are needed. Warning: Requesting "activeTab" or "tabs" permissions will take longer to ensure that the code does not perform any abuse.
That's it! This should get you ready to start building Chrome browser extensions! ?
The above is the detailed content of How to Build a Chrome Extension. For more information, please follow other related articles on the PHP Chinese website!
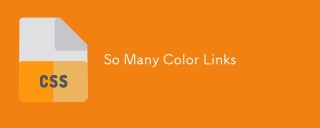
There's been a run of tools, articles, and resources about color lately. Please allow me to close a few tabs by rounding them up here for your enjoyment.
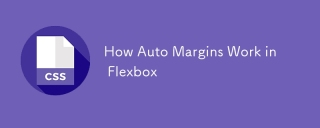
Robin has covered this before, but I've heard some confusion about it in the past few weeks and saw another person take a stab at explaining it, and I wanted
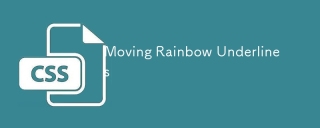
I absolutely love the design of the Sandwich site. Among many beautiful features are these headlines with rainbow underlines that move as you scroll. It's not
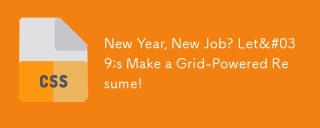
Many popular resume designs are making the most of the available page space by laying sections out in a grid shape. Let’s use CSS Grid to create a layout that
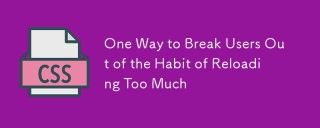
Page reloads are a thing. Sometimes we refresh a page when we think it’s unresponsive, or believe that new content is available. Sometimes we’re just mad at
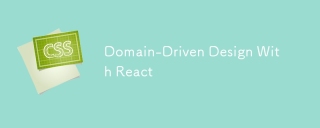
There is very little guidance on how to organize front-end applications in the world of React. (Just move files around until it “feels right,” lol). The truth
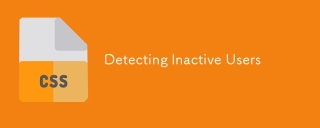
Most of the time you don’t really care about whether a user is actively engaged or temporarily inactive on your application. Inactive, meaning, perhaps they
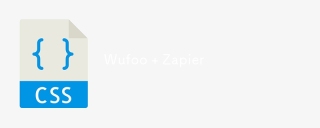
Wufoo has always been great with integrations. They have integrations with specific apps, like Campaign Monitor, Mailchimp, and Typekit, but they also


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
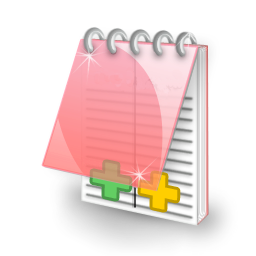
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use