Netflix uses React as the main framework for its web applications. 1) React's flexibility and efficiency are the main reasons why Netflix chose it. 2) React's componentization and virtual DOM technology help Netflix manage complex UI and improve rendering performance. 3) Netflix uses React's ecosystem and community support to iterate and scale quickly. 4) They combine Redux for global state management and develop custom Hook processing business logic. 5) Netflix optimizes application performance through lazy loading, code segmentation and performance monitoring tools.
Netflix primarily uses React for its web application. While Vue.js is a popular framework, Netflix has heavily invested in React, leveraging its ecosystem and community support to build their complex, high-performance user interfaces.
Netflix's technology stack: React selection and application
Netflix's technology choice has always been the focus of industry attention, especially in the use of front-end frameworks. Today we will talk about why Netflix chose React and how it can play its advantages in practical applications.
The charm of React and the choice of Netflix
There are many reasons why Netflix chose React, but the most important thing is because of React's flexibility and efficiency. React's component development model allows Netflix to better manage complex UI logic, while its virtual DOM technology greatly improves rendering performance. React's advantages are particularly obvious in a platform like Netflix that requires handling large amounts of user interaction and data flow.
I remember at a technology sharing session, Netflix's front-end engineers mentioned that one of the important reasons they chose React is the richness of its ecosystem. The React community is very active and provides a large number of third-party libraries and tools, which is a huge advantage for a platform like Netflix that requires rapid iteration and expansion.
// A simple React component example import React, { useState } from 'react'; const MovieList = ({ movies }) => { const [selectedMovie, setSelectedMovie] = useState(null); Return ( <div> <h1 id="Movie-List">Movie List</h1> <ul> {movies.map(movie => ( <li key={movie.id} onClick={() => setSelectedMovie(movie)}> {movie.title} </li> ))} </ul> {selectedMovie && <MovieDetails movie={selectedMovie} />} </div> ); }; const MovieDetails = ({ movie }) => ( <div> <h2 id="movie-title">{movie.title}</h2> <p>{movie.description}</p> </div> );
This simple component shows the basic usage of React. Netflix will perform more complex component design and state management according to needs in actual applications.
Application of React on Netflix
Netflix not only uses React to build its master site, it also widely applies React in its internal tools and management systems. For example, Netflix's recommendation algorithm and user interface are built on React, which allows them to quickly respond to user behavior and provide personalized content recommendations.
During the development of Netflix, they also combined Redux to manage global state, which makes complex application state management more controllable and maintainable. Netflix engineers have also developed some custom React Hooks to handle specific business logic, which demonstrates React's flexibility and scalability.
// Custom React Hook example import { useState, useEffect } from 'react'; const useMovieRecommendations = (userId) => { const [recommendations, setRecommendations] = useState([]); useEffect(() => { const fetchRecommendations = async () => { const response = await fetch(`/api/recommendations?userId=${userId}`); const data = await response.json(); setRecommendations(data); }; fetchRecommendations(); }, [userId]); return recommendations; };
This custom Hook shows how Netflix can leverage the features of React to simplify complex business logic.
Performance optimization and best practices
Netflix pays great attention to performance optimization when using React. They used React's lazy loading and code segmentation techniques to reduce initial loading time, and also used React.memo and useMemo to optimize the rendering performance of components.
In actual development, Netflix engineers also use performance monitoring tools to monitor application performance in real time and optimize based on monitoring data. This not only improves the user experience, but also reduces the load on the server.
// Performance optimization example import React, { lazy, Suspense } from 'react'; const MovieDetails = lazy(() => import('./MovieDetails')); const App = () => ( <Suspense fallback={<div>Loading...</div>}> <MovieDetails /> </Suspense> );
This example shows how to use React's lazy loading and Suspense to optimize the loading performance of your application.
Summary and reflection
Netflix chose React not only because of its technological advantages, but also because of the richness of its ecosystem and the activity of its community. In practical applications, Netflix fully utilizes React's flexibility and efficiency to build a complex and high-performance user interface.
However, there are some challenges to choose React. For example, React's learning curve is relatively steep, which may take some time for new developers to adapt. In addition, React's ecosystem, although rich, also means more time is spent selecting and integrating the right tools and libraries.
Overall, Netflix's technology choices provide us with a good reference case for how to select and apply a front-end framework in a real-life project. I hope that through this article, you can have a deeper understanding of Netflix's technology stack and learn from it in your own projects.
The above is the detailed content of Does Netflix use Vue or React?. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
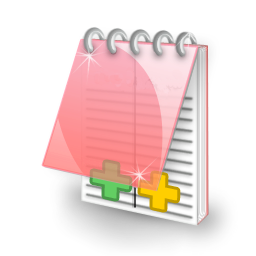
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
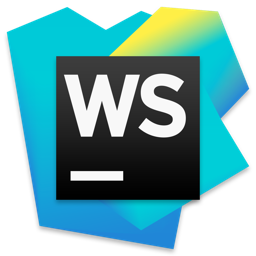
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment