phpMyAdmin FAQ solutions include: 1. Login failed: Check the database connection information in the username, password, and configuration file. 2. Insufficient permissions: Use MySQL's GRANT statement to adjust permissions. 3. SQL syntax error: double-check SQL statements and use phpMyAdmin's SQL query window to test and debug.
introduction
It’s really a headache to encounter the problem of phpMyAdmin! As a veteran developer, I know how important it is to find the right way when dealing with these problems. The purpose of this article is to help everyone solve common problems and errors encountered when using phpMyAdmin. Whether you are a newbie who is new to phpMyAdmin or a veteran who has been using it for a while, here are the solutions you need. After reading this article, you will be able to deal with various challenges of phpMyAdmin more calmly.
In my career, phpMyAdmin has accompanied me through countless projects like an old friend. Although it is very powerful, sometimes it can have some minor problems. Today, I would like to share with you some of the experience and skills I have accumulated, hoping to help you who are worried about this.
Review of basic knowledge
phpMyAdmin is a free and open source tool, mainly used for the management of MySQL and MariaDB. It provides an intuitive way to manipulate databases through the web interface, which is ideal for users who are not familiar with command line operations.
When using phpMyAdmin, it is helpful to understand some basic concepts, such as databases, tables, fields, etc. These concepts are crucial in daily database management. In addition, the installation and configuration of phpMyAdmin also requires certain technical support, and ensuring the correct setting of the server environment is the key.
Core concept or function analysis
Functions and functions of phpMyAdmin
The main function of phpMyAdmin is to manage MySQL databases through the web interface. It allows users to create, modify, delete databases and tables, execute SQL queries, import and export data, etc. Its advantages lie in its ease of use and comprehensive functionality, making database management easier and more efficient.
For example, here is a simple example showing how to create a new table with phpMyAdmin:
<?php // Suppose we have connected to the MySQL database $conn = new mysqli("localhost", "username", "password", "database"); // Check the connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // SQL statement $sql = "CREATE TABLE MyGuests ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, firstname VARCHAR(30) NOT NULL, lastname VARCHAR(30) NOT NULL, email VARCHAR(50), reg_date TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP )"; // Execute SQL statement if ($conn->query($sql) === TRUE) { echo "Table MyGuests was created successfully"; } else { echo "Create table error: " . $conn->error; } $conn->close(); ?>
This example shows how to create a table in phpMyAdmin via PHP code. Through this process, you can see how phpMyAdmin simplifies database operations.
How it works
phpMyAdmin works by interacting with a MySQL database through a web server such as Apache or Nginx. It converts the user's actions into SQL queries and then sends them to the database server for execution. The results are then displayed to users through the web interface.
Understanding how it works can help us locate the problem faster when dealing with phpMyAdmin's problem. For example, network connection problems, permission settings errors, or SQL syntax errors may be the reasons why phpMyAdmin cannot work properly.
Example of usage
Basic usage
In phpMyAdmin, the most common operation is browsing and editing data in the database. Here is a simple example showing how to import a CSV file into a database through phpMyAdmin:
<?php // Suppose we have connected to the MySQL database $conn = new mysqli("localhost", "username", "password", "database"); // Check the connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // CSV file path $file = 'data.csv'; // Open the CSV file if (($handle = fopen($file, 'r')) !== FALSE) { // Read the first line of the CSV file as the header $header = fgetcsv($handle, 1000, ','); // Create SQL statement to insert data while (($data = fgetcsv($handle, 1000, ',')) !== FALSE) { $num = count($data); $sql = "INSERT INTO MyGuests (firstname, lastname, email) VALUES ('" . $data[0] . "', '" . $data[1] . "', '" . $data[2] . "')"; $conn->query($sql); } fclose($handle); } $conn->close(); ?>
This example shows how to import data from a CSV file into a database through PHP code. In actual operation, phpMyAdmin provides a more intuitive interface to complete this task.
Advanced Usage
In phpMyAdmin, there are some advanced features that can help us manage our database more effectively. For example, batch operations, SQL query optimization, database backup and recovery, etc. Here is an example showing how to perform database backup via phpMyAdmin:
<?php // Suppose we have connected to the MySQL database $conn = new mysqli("localhost", "username", "password", "database"); // Check the connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } // Create backup file $backup_file = 'backup.sql'; // Open the backup file $fp = fopen($backup_file, 'w'); // Get all table names $tables = array(); $result = $conn->query('SHOW TABLES'); while($row = mysqli_fetch_row($result)){ $tables[] = $row[0]; } // Iterate through all tables and generate backup SQL statement foreach($tables as $table) { $result = $conn->query('SELECT * FROM '.$table); $num_fields = mysqli_num_fields($result); $return = ''; $return.= 'DROP TABLE IF EXISTS '.$table.';'; $row2 = mysqli_fetch_row($conn->query('SHOW CREATE TABLE '.$table)); $return.= "\n\n".$row2[1].";\n\n"; for ($i = 0; $i < $num_fields; $i ) { while($row = mysqli_fetch_row($result)) { $return.= 'INSERT INTO '.$table.' VALUES('; for($j=0; $j<$num_fields; $j ) { $row[$j] = addslashes($row[$j]); $row[$j] = str_replace("\n","\\n",$row[$j]); if (isset($row[$j])) { $return.= '"'.$row[$j].'"' ; } else { $return.= '""'; } if ($j<($num_fields-1)) { $return.= ','; } } $return.= ");\n"; } } $return.="\n\n\n"; fwrite($fp, $return); } fclose($fp); $conn->close(); ?>
This example shows how to generate a database backup file through PHP code. In practice, phpMyAdmin provides a simpler way to accomplish this task.
Common Errors and Debugging Tips
When using phpMyAdmin, common errors include login failure, insufficient permissions, SQL syntax errors, etc. Here are some common errors and their solutions:
- Login failed : Check whether the username and password are correct, and make sure the database connection information in the phpMyAdmin configuration file is correct.
- Insufficient permissions : Ensure that the current user has sufficient permissions to perform the required operations, and the permissions can be adjusted through the MySQL GRANT statement.
- SQL syntax error : Check the SQL statement carefully to ensure the syntax is correct, and you can use phpMyAdmin's SQL query window for testing and debugging.
During debugging, you can get more information by viewing the error log of phpMyAdmin. Error logs are usually located in the installation directory of phpMyAdmin. Viewing these logs can help us quickly locate problems.
Performance optimization and best practices
Performance optimization and best practices are also very important when using phpMyAdmin. Here are some suggestions:
- Optimize SQL query : Ensure the efficiency of SQL statements when executing large queries. You can use the EXPLAIN statement to analyze the execution plan of the query, find out the bottlenecks and optimize it.
- Database index : The rational use of indexes can significantly improve query speed. Make sure to index on frequently queried fields, but also be careful that too many indexes can affect the performance of insertion and update operations.
- Regular backup : It is very important to back up the database regularly and prevent data loss. phpMyAdmin provides convenient backup and recovery functions and is recommended to use them regularly.
- Code readability : When writing code related to phpMyAdmin, it is very important to keep the code readability and maintenance. Using meaningful variable names and comments can help other developers understand and maintain code.
In my career, I have found that these best practices not only improve the efficiency of phpMyAdmin, but also reduce the occurrence of errors. Hope these suggestions help you to be more handy when using phpMyAdmin.
The above is the detailed content of phpMyAdmin Troubleshooting: Solving Common Issues and Errors. For more information, please follow other related articles on the PHP Chinese website!

The security reinforcement strategies of phpMyAdmin include: 1. Use HTTPS to ensure communication encryption; 2. Restrict access through IP whitelist or user authentication; 3. Implement a strong password policy; 4. Disable unnecessary functions to reduce the attack surface; 5. Configure log audits to monitor and respond to threats. These measures have jointly improved the security of phpMyAdmin.

phpMyAdmin realizes team collaboration and user management through user and permission systems. 1) Create a user and assign permissions: Use the CREATEUSER and GRANT commands. 2) Use role management to simplify permission assignment: create roles and assign them to users. 3) Follow the principle of minimum authority and regularly audit permissions to ensure security.

When processing large data sets, the import and export of phpMyAdmin can be optimized through the following steps: 1. Use batch import to reduce memory usage; 2. Increase memory and execution time limits to avoid overflow and timeouts; 3. Compress files and optimize SQL statements to improve performance; 4. Consider using command line tools to process super-large data sets. This can significantly improve data processing efficiency.

phpMyAdmin provides a variety of advanced features to improve database management efficiency. 1. The SQL query editor allows writing and executing complex queries and optimizing table structure. 2. The data export and import functions support backup and migration of data. 3. Performance optimization improves efficiency through indexing and query optimization, and regular maintenance keeps the database running efficiently.

How to make phpMyAdmin more automated and personalized? It can be achieved through the following steps: 1. Use SQL or PHP scripts to automate general tasks, such as database backup and report generation; 2. Customize by modifying configuration files and interfaces to improve user experience and work efficiency.

phpMyAdmin FAQ solutions include: 1. Login failed: Check the database connection information in the username, password and configuration file. 2. Insufficient permissions: Use MySQL's GRANT statement to adjust permissions. 3. SQL syntax error: double-check SQL statements and use phpMyAdmin's SQL query window to test and debug.

phpMyAdmin can perform advanced query and data operations through the following methods: 1. Use JOIN operations to combine multiple table data, such as combining customer and order tables. 2. Use subqueries to nest queries to filter data of specific conditions. 3. Use window functions to perform data analysis, such as ranking customer orders. 4. Use the EXPLAIN command to optimize query performance, avoid common errors and improve efficiency.

The steps to optimize phpMyAdmin performance include: 1. Adjust the configuration file, such as setting compressed transmission and limiting the number of rows displayed per page; 2. Optimizing MySQL query and cache settings; 3. Use EXPLAIN analysis query plan to find out performance bottlenecks. Through these methods, the response speed and processing power of phpMyAdmin can be significantly improved and database management efficiency can be improved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
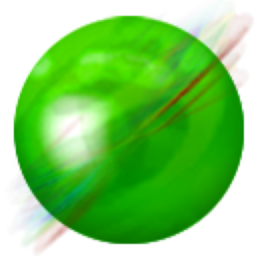
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
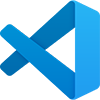
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),