


SQL Deep Dive: Mastering Window Functions, Common Table Expressions (CTEs), and Stored Procedures
SQL provides three powerful functions: window functions, common table expressions (CTEs), and stored procedures. 1. Window functions allow grouping and sorting operations without changing the data set. 2.CTEs provide temporary result sets to simplify complex queries. 3. Stored procedures are precompiled SQL code blocks that can be executed repeatedly to improve efficiency and consistency.
introduction
In a data-driven world, SQL is not just a query language, but also an art. Today, we will dive into three powerful features in SQL: window functions, common table expressions (CTEs), and stored procedures. Through this article, you will learn how to use these tools to deal with complex data problems, improve your SQL skills, and be at ease in data analysis and management.
Review of basic knowledge
The charm of SQL lies in its simplicity and powerful functions. Window functions allow you to group and sort data without changing the data set. CTEs provide a temporary result set way to make complex queries more readable and managed. Stored procedures are precompiled SQL code blocks that can be executed repeatedly to improve efficiency and consistency.
Core concept or function analysis
Window Functions
Window functions are a magical tool in SQL that allows you to group and sort data without changing the data set. They are very useful in data analysis because they can help you calculate moving averages, rankings, cumulative sums, and more.
SELECT Employee_id, Salarary, AVG(salary) OVER (PARTITION BY department) AS avg_department_salary, RANK() OVER (ORDER BY salary DESC) AS salary_rank FROM employees;
In this example, we calculate the average salary for each employee’s department and rank the employees based on their salary. The power of window functions is that they allow you to perform multiple calculations in the same query without using subqueries or self-joins.
Common Table Expressions (CTEs)
CTEs are temporary result sets in SQL that simplify the structure of complex queries and make the code more readable and maintained. CTEs are particularly useful in recursive queries because they can refer to themselves.
WITH RECURSIVE employee_hierarchy AS ( SELECT employee_id, manager_id, 0 AS level FROM employees WHERE manager_id IS NULL UNION ALL SELECT e.employee_id, e.manager_id, eh.level 1 FROM employees e JOIN employee_hierarchy eh ON e.manager_id = eh.employee_id ) SELECT * FROM employee_hierarchy;
In this example, we use CTE to build the hierarchy of employees. The recursive nature of CTE allows us to easily traverse the entire employee tree without writing complex self-connection queries.
Stored procedures
Stored procedures are precompiled blocks of SQL code that can be executed repeatedly. They are useful when you need to perform complex logic or improve performance because they reduce network traffic and compile time.
CREATE PROCEDURE get_employee_details(IN emp_id INT) BEGIN SELECT e.employee_id, e.first_name, e.last_name, d.department_name FROM Employees e JOIN departments d ON e.department_id = d.department_id WHERE e.employee_id = emp_id; END;
In this example, we create a stored procedure to get employee details. The advantage of stored procedures is that they can encapsulate complex logic and can be called multiple times, improving code reusability and consistency.
Example of usage
Basic usage
The basic usage of window functions is very simple. You can use the OVER
clause to define the window and use various aggregate functions to calculate the result.
SELECT product_id, sale_date, sale_amount, SUM(sale_amount) OVER (PARTITION BY product_id ORDER BY sale_date) AS running_total FROM sales;
In this example, we calculate the accumulated sales for each product. PARTITION BY
clause groups data, and ORDER BY
clause defines the order of windows.
The basic usage of CTEs is also very simple. You can use the WITH
keyword to define a CTE and then reference it in subsequent queries.
WITH top_sellers AS ( SELECT product_id, SUM(sale_amount) AS total_sales FROM sales GROUP BY product_id ORDER BY total_sales DESC LIMIT 10 ) SELECT * FROM top_sellers;
In this example, we use CTE to find the 10 products with the highest sales. CTE makes query structure clearer and easier to manage.
The basic usage of stored procedures is also very simple. You can use the CREATE PROCEDURE
statement to define a stored procedure, and then use the CALL
statement to call it.
CALL get_employee_details(1);
In this example, we call the previously defined stored procedure to get employee details with employee ID 1.
Advanced Usage
Advanced usage of window functions includes using ROWS
or RANGE
clauses to define the scope of a window, and using LAG
and LEAD
functions to access the data of the front and back rows.
SELECT product_id, sale_date, sale_amount, LAG(sale_amount) OVER (PARTITION BY product_id ORDER BY sale_date) AS previous_sale, LEAD(sale_amount) OVER (PARTITION BY product_id ORDER BY sale_date) AS next_sale FROM sales;
In this example, we use LAG
and LEAD
functions to get the previous and next sales of each product. Such advanced usage can help you perform more complex data analysis.
Advanced usage of CTEs includes using recursive CTEs to process hierarchical data, and using multiple CTEs to simplify complex queries.
WITH RECURSIVE category_hierarchy AS ( SELECT category_id, parent_category_id, 0 AS level FROM categories WHERE parent_category_id IS NULL UNION ALL SELECT c.category_id, c.parent_category_id, ch.level 1 FROM categories c JOIN category_hierarchy ch ON c.parent_category_id = ch.category_id ), product_categories AS ( SELECT p.product_id, ch.category_id, ch.level FROM products p JOIN category_hierarchy ch ON p.category_id = ch.category_id ) SELECT * FROM product_categories;
In this example, we use recursive CTE to build the hierarchy of the product category, and then use another CTE to associate the product with its category. Such advanced usage can help you deal with complex hierarchical data.
Advanced usage of stored procedures includes the use of cursors, exception handling, and transaction management to implement complex business logic.
CREATE PROCEDURE update_employee_salary(IN emp_id INT, IN new_salary DECIMAL(10, 2)) BEGIN DECLARE exit handler for sqlexception BEGIN ROLLBACK; RESIGNAL; END; START TRANSACTION; UPDATE employees SET salary = new_salary WHERE employee_id = emp_id; COMMIT; END;
In this example, we create a stored procedure to update employee salaries. Stored procedures use transaction management and exception handling to ensure data consistency and integrity.
Common Errors and Debugging Tips
A common mistake when using window functions is to forget to use the OVER
clause. This causes the SQL engine to fail to parse window functions correctly.
-- Error Example SELECT Employee_id, Salarary, AVG(salary) -- Missing OVER clause FROM employees;
To avoid this error, make sure that the OVER
clause is always included when using the window function.
When using CTEs, a common mistake is to forget to define all required columns in the CTE. This will cause subsequent queries to fail to correctly reference data in the CTE.
-- Error Example WITH top_sellers AS ( SELECT product_id -- total_sales column missing FROM sales GROUP BY product_id ORDER BY total_sales DESC LIMIT 10 ) SELECT * FROM top_sellers;
To avoid this error, make sure to include all required columns when defining the CTE.
A common mistake when using stored procedures is forgetting to handle exceptions. This may cause stored procedures to fail to roll back the transaction correctly when they encounter an error.
-- Error example CREATE PROCEDURE update_employee_salary(IN emp_id INT, IN new_salary DECIMAL(10, 2)) BEGIN UPDATE employees SET salary = new_salary WHERE employee_id = emp_id; END;
To avoid this error, make sure to include exception handling and transaction management in the stored procedure.
Performance optimization and best practices
When using window functions, a key point in performance optimization is to select the appropriate window frame. Using ROWS
or RANGE
clauses can significantly improve query performance because they can reduce the computational volume of window functions.
-- Optimization Example SELECT product_id, sale_date, sale_amount, SUM(sale_amount) OVER (PARTITION BY product_id ORDER BY sale_date ROWS BETWEEN UNBOUNDED PRECEDING AND CURRENT ROW) AS running_total FROM sales;
In this example, we use the ROWS
clause to define the window framework, which can improve query performance.
When using CTEs, a key point in performance optimization is to avoid using complex calculations in CTEs. CTEs are temporary result sets that may affect query performance if they contain complex calculations.
-- Optimization example WITH sales_summary AS ( SELECT product_id, SUM(sale_amount) AS total_sales FROM sales GROUP BY product_id ) SELECT * FROM sales_summary;
In this example, we put complex calculations outside of CTE to improve query performance.
When using stored procedures, a key point in performance optimization is to avoid using cursors in stored procedures. Cursors can cause performance degradation because they need to process data line by line.
-- Optimization example CREATE PROCEDURE update_employee_salaries() BEGIN UPDATE employees SET salary = salary * 1.1; END;
In this example, we avoid using cursors and use batch update operations to improve performance.
When writing SQL code, best practices include using meaningful alias, annotating code, and keeping the code readable and maintainable.
-- Best Practice Example SELECT e.employee_id AS emp_id, -- use meaningful alias e.first_name, -- comment code e.last_name, d.department_name -- Keep code readability and maintainability FROM Employees e JOIN departments d ON e.department_id = d.department_id;
By following these best practices, you can write more efficient and more maintainable SQL code.
In the process of exploring SQL, we not only mastered the basic and advanced usage of window functions, CTEs and stored procedures, but also learned how to avoid common errors and optimize performance. I hope this article can help you better understand and apply these powerful SQL features and achieve greater success in data analysis and management.
The above is the detailed content of SQL Deep Dive: Mastering Window Functions, Common Table Expressions (CTEs), and Stored Procedures. For more information, please follow other related articles on the PHP Chinese website!

SQL indexes can significantly improve query performance through clever design. 1. Select the appropriate index type, such as B-tree, hash or full text index. 2. Use composite index to optimize multi-field query. 3. Avoid over-index to reduce data maintenance overhead. 4. Maintain indexes regularly, including rebuilding and removing unnecessary indexes.
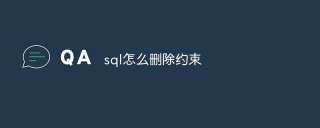
To delete a constraint in SQL, perform the following steps: Identify the constraint name to be deleted; use the ALTER TABLE statement: ALTER TABLE table name DROP CONSTRAINT constraint name; confirm deletion.
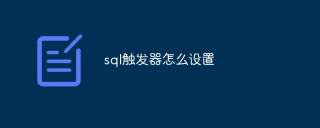
A SQL trigger is a database object that automatically performs specific actions when a specific event is executed on a specified table. To set up SQL triggers, you can use the CREATE TRIGGER statement, which includes the trigger name, table name, event type, and trigger code. The trigger code is defined using the AS keyword and contains SQL or PL/SQL statements or blocks. By specifying trigger conditions, you can use the WHERE clause to limit the execution scope of a trigger. Trigger operations can be performed in the trigger code using the INSERT INTO, UPDATE, or DELETE statement. NEW and OLD keywords can be used to reference the affected keyword in the trigger code.
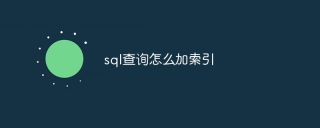
Indexing is a data structure that accelerates data search by sorting data columns. The steps to add an index to an SQL query are as follows: Determine the columns that need to be indexed. Select the appropriate index type (B-tree, hash, or bitmap). Use the CREATE INDEX command to create an index. Reconstruct or reorganize the index regularly to maintain its efficiency. The benefits of adding indexes include improved query performance, reduced I/O operations, optimized sorting and filtering, and improved concurrency. When queries often use specific columns, return large amounts of data that need to be sorted or grouped, involve multiple tables or database tables that are large, you should consider adding an index.
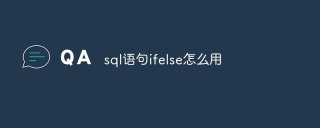
The IFELSE statement is a conditional statement that returns different values based on the conditional evaluation result. Its syntax structure is: IF (condition) THEN return_value_if_condition_is_true ELSE return_value_if_condition_is_false END IF;.
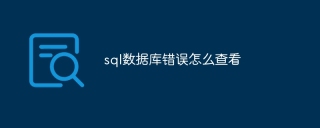
The methods for viewing SQL database errors are: 1. View error messages directly; 2. Use SHOW ERRORS and SHOW WARNINGS commands; 3. Access the error log; 4. Use error codes to find the cause of the error; 5. Check the database connection and query syntax; 6. Use debugging tools.
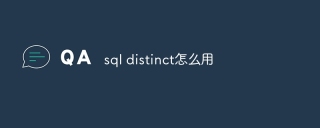
The DISTINCT operator is used to exclude duplicate rows in SQL queries and returns only unique values. It is suitable for scenarios such as obtaining a list of unique values, counting the number of unique values, and using it in combination with GROUP BY.
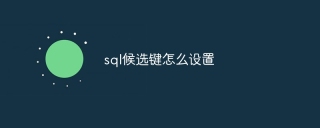
Methods to set candidate keys in SQL: Determine a unique identification column; create a primary key using the PRIMARY KEY constraint; add a unique constraint using the UNIQUE constraint; create a unique index. Setting candidate keys ensures data integrity, improves query performance, and prevents data duplication.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
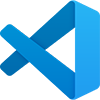
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),