


Attributes is a newly introduced metadata annotation feature in PHP 8 for embedding additional information in code. It is more structured than DocBlocks and can be processed at runtime. Attributes work through reflection mechanism and are suitable for scenarios such as version tagging, routing definition, etc., and can use their own advantages in combination with DocBlocks.
introduction
In the world of PHP 8, Attributes is like a new star, making us old programmers revisit their toolboxes. Today we will talk about these new features and their grudges and hatreds with old friend DocBlocks. After reading this article, you will not only understand the basic usage of Attributes, but also understand their application scenarios and advantages in actual projects.
Review of basic knowledge
Let's start with the basics. Attributes in PHP are metadata annotations that allow us to embed additional information directly into our code, while DocBlocks is a tool that PHP developers have long used to add document annotations. Although both are used to provide additional information, their usage and purpose are very different.
Attributes are like tagging your code, which can directly affect the behavior of the code or provide additional configuration information. They were introduced in PHP 8 and were designed to provide a more structured way to add metadata. In contrast, DocBlocks describes the use, parameters, return values and other information of the code through special format comments, and is mainly used to generate code prompts for API documents or IDEs.
Core concept or function analysis
The definition and function of Attributes
Attributes can be regarded as tags or annotations of code, which can provide additional contextual information without changing the code itself. For example, you can use Attributes to tag a class or method for special processing at runtime or compile time.
#[Attribute] class MyAttribute { public function __construct(public string $value) {} } #[MyAttribute('example')] class MyClass { // ... }
In this example, MyAttribute
is a custom Attribute that can be used to tag MyClass
with the value of the tag being 'example'. This method makes the code more self-described and can be read and used by other tools or frameworks.
How it works
Attributes works through PHP reflection mechanism. Reflection allows us to check and manipulate code structures at runtime, so we can read and process these Attributes. They are stored in PHP's AST (Abstract Syntax Tree) and are recognized and processed when parsing the code.
$reflectionClass = new ReflectionClass(MyClass::class); $attributes = $reflectionClass->getAttributes(MyAttribute::class); foreach ($attributes as $attribute) { $instance = $attribute->newInstance(); echo $instance->value; // Output 'example' }
In this example, we use reflection to get all MyAttribute
instances on MyClass
and read their value
attributes. This way makes Attributes available not only for documents, but also for actual code logic.
Example of usage
Basic usage
Let's look at a simple example showing how to use Attributes to add version information to a class:
#[Attribute] class VersionAttribute { public function __construct(public string $version) {} } #[VersionAttribute('1.0.0')] class MyService { // ... }
In this example, we define a VersionAttribute
and use it to label MyService
class with version tags. This approach is more structured than using DocBlocks and is easier to find and process in your code.
Advanced Usage
Advanced usage of Attributes can include using them in the routing system to define routing rules, or using them in the ORM to define database mappings. Let's look at an example of a routing system:
#[Attribute] class RouteAttribute { public function __construct(public string $path, public string $method) {} } #[RouteAttribute('/users', 'GET')] function getUsers() { // Return to user list} #[RouteAttribute('/users/{id}', 'GET')] function getUser($id) { // Return the information of the specified user}
In this example, we use RouteAttribute
to define routing rules, which is more intuitive and flexible than traditional configuration files or comments.
Common Errors and Debugging Tips
Common errors when using Attributes include forgetting to use #[Attribute]
to tag a custom Attribute, or forgetting to handle possible exceptions when using reflection. Here are some debugging tips:
- Make sure your Attribute class uses the
#[Attribute]
annotation correctly. - When using reflection, remember to handle possible exceptions such as
ReflectionException
. - Use the IDE's code checking feature to ensure that the use of Attributes complies with PHP's syntax rules.
Performance optimization and best practices
In terms of performance optimization, the use of Attributes does not directly affect the execution efficiency of the code, but they may increase the parsing time of the code, especially in large projects. Therefore, the best practice is:
- Use Attributes reasonably to avoid abuse and cause code parsing to be too long.
- Use a cache mechanism to store the parsing results of Attributes when needed, reducing the overhead of repeated parsing.
- Keep Attributes simple and readable and avoid overly complex logic.
In terms of best practices, it is recommended:
- Use Attributes to replace some DocBlocks, especially metadata that needs to be processed at runtime.
- Use combinations of Attributes and DocBlocks, Attributes are used for runtime processing and DocBlocks are used for document generation.
- Maintain maintainability and readability of the code and avoid over-reliance on Attributes to implement complex logic.
Comparison with DocBlocks
Finally, let's compare Attributes and DocBlocks. Attributes provide a more structured metadata annotation method that can be processed at runtime, while DocBlocks is mainly used to generate documents and provide IDE prompts. Both have their advantages and disadvantages:
- Structure and Flexibility : Attributes are more structured and suitable for embedding metadata in code, while DocBlocks are more flexible and suitable for describing the purpose and parameters of the code.
- Runtime processing : Attributes can be read and processed by reflection mechanisms and are suitable for special processing at runtime, while DocBlocks is mainly used for static analysis and document generation.
- Compatibility : Attributes is a new feature of PHP 8, which is not available in older versions of PHP, while DocBlocks can be used across versions.
In general, Attributes and DocBlocks each have their own uses and can be used in a combination in actual projects to give full play to their respective strengths. Hopefully this article helps you better understand and use Attributes in PHP and find their best application scenarios in your project.
The above is the detailed content of What are PHP Attributes (PHP 8 ) and how do they compare to DocBlocks?. For more information, please follow other related articles on the PHP Chinese website!
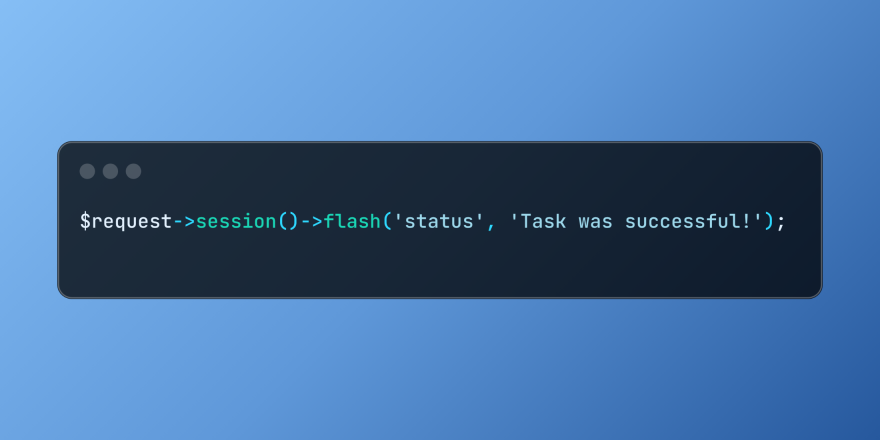
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
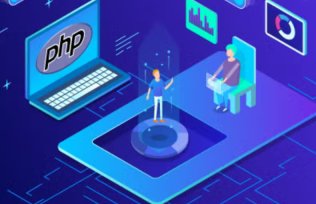
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
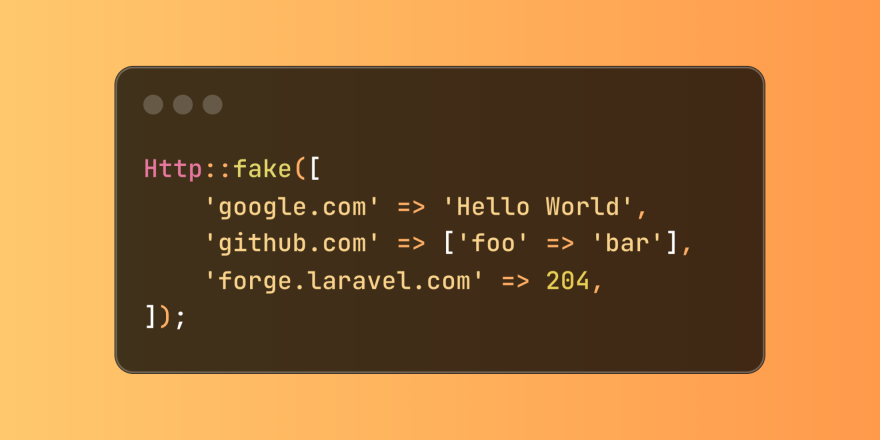
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
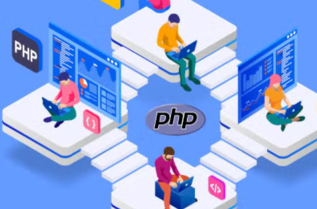
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
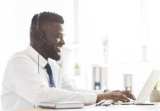
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
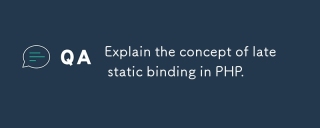
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
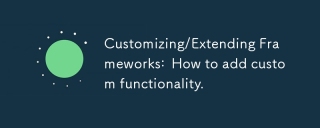
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
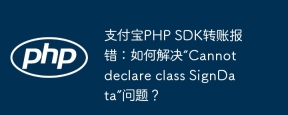
Alipay PHP...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
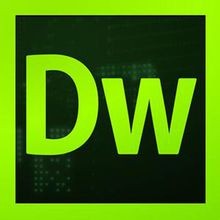
Dreamweaver CS6
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
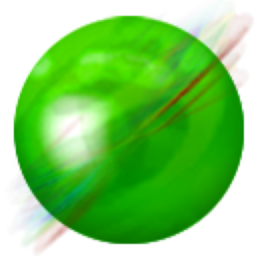
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment