Modern web application development often involves loose coupling between front-end and back-end, frequently handled by separate teams. This can lead to synchronization challenges. A solution is to simulate the back-end API, creating a "fake" server that mimics the expected behavior. This process is known as mocking. Ideally, mocking allows front-end development to proceed independently, without direct back-end dependency. While multiple mocking approaches exist, this can be daunting.
This article demonstrates effective API mocking and its implementation in new or existing applications using a framework-agnostic approach (applicable to any framework or vanilla JavaScript).
Mirage: A Client-Side Mocking Solution
We'll utilize MirageJS, a client-side mocking framework. Mirage operates within the browser, intercepting XMLHttpRequest
and Fetch
requests to simulate API responses. It provides a user-friendly interface and simplifies the mocking process.
We'll build a simple to-do application using Vue (though the approach is framework-independent).
Setting up Mirage
Install Mirage:
# npm npm i miragejs -D # Yarn yarn add miragejs -D
Optimal folder structure:
<code>/ ├── public ├── src │ ├── api │ │ └── mock │ │ ├── fixtures │ │ │ └── get-tasks.js │ │ └── index.js │ └── main.js ├── package.json └── package-lock.json</code>
Create api/mock/index.js
:
// api/mock/index.js import { Server } from 'miragejs'; export default function ({ environment = 'development' } = {}) { return new Server({ environment, routes() { // Routes defined here }, }); }
In your application's bootstrap file (e.g., src/main.js
for Vue):
// main.js import createServer from './api/mock/index'; if (process.env.NODE_ENV === 'development') { createServer(); }
This conditional ensures Mirage is excluded from production builds.
How Mirage Works
Mirage is client-side, using the Pretender library. Pretender intercepts requests, preventing them from reaching the actual server and routing them to the simulated Mirage server. DevTools' Network tab won't show Mirage requests, as they're handled internally.
Handling Requests
Let's create a /api/tasks
endpoint to fetch to-do items (using axios):
// components/tasks.vue export default { async created() { try { const { data } = await axios.get('/api/tasks'); this.tasks = data.tasks; } catch (e) { console.error(e); } } };
Initially, Mirage will log an error indicating an undefined route. We address this by adding the route handler in api/mock/index.js
:
// api/mock/index.js routes() { this.get('/api/tasks', () => ({ tasks: [ { id: 1, text: "Feed the cat" }, { id: 2, text: "Wash the dishes" }, ], })); },
Mirage supports various HTTP methods (get
, post
, patch
, put
, delete
, options
).
Dynamic Data with Mirage's Data Layer
For dynamic data, Mirage provides a seeds
hook and a lightweight database. Let's refactor:
// api/mock/index.js seeds(server) { server.db.loadData({ tasks: [ { id: 1, text: "Feed the cat" }, { id: 2, text: "Wash the dishes" }, ], }); }, routes() { this.get('/api/tasks', (schema) => { return schema.db.tasks; }); this.post('/api/tasks', (schema, request) => { const task = JSON.parse(request.requestBody).data; return schema.db.tasks.insert(task); }); this.delete('/api/tasks/:id', (schema, request) => { const id = request.params.id; return schema.db.tasks.remove(id); }); }
The schema
parameter in route handlers provides access to the Mirage database. The request
parameter contains request details.
Integrating Mirage into Existing Applications
To integrate Mirage into an existing application, use the passthrough()
method to handle unhandled requests:
routes() { this.get('/api/tasks', () => { ... }); this.passthrough(); // Handle unhandled requests }
You can also specify a custom urlPrefix
:
routes() { this.urlPrefix = 'https://devenv.ourapp.example'; this.get('/tasks', () => { ... }); }
This allows seamless transition between Mirage and the real API.
Conclusion
MirageJS offers a streamlined, client-side solution for API mocking, eliminating the need for external processes or server-side knowledge. Its ease of use and powerful features make it a valuable tool for front-end developers. Refer to the official MirageJS documentation for more advanced features and tutorials. A working example can be found on GitHub.
The above is the detailed content of Don't Wait! Mock the API. For more information, please follow other related articles on the PHP Chinese website!
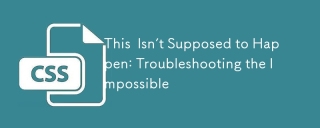
What it looks like to troubleshoot one of those impossible issues that turns out to be something totally else you never thought of.
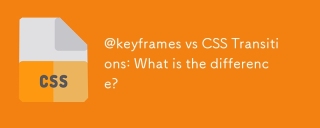
@keyframesandCSSTransitionsdifferincomplexity:@keyframesallowsfordetailedanimationsequences,whileCSSTransitionshandlesimplestatechanges.UseCSSTransitionsforhovereffectslikebuttoncolorchanges,and@keyframesforintricateanimationslikerotatingspinners.
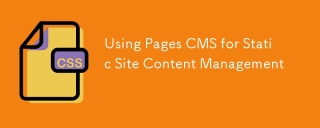
I know, I know: there are a ton of content management system options available, and while I've tested several, none have really been the one, y'know? Weird pricing models, difficult customization, some even end up becoming a whole &
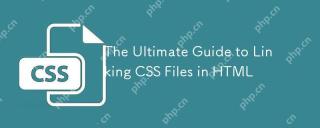
Linking CSS files to HTML can be achieved by using elements in part of HTML. 1) Use tags to link local CSS files. 2) Multiple CSS files can be implemented by adding multiple tags. 3) External CSS files use absolute URL links, such as. 4) Ensure the correct use of file paths and CSS file loading order, and optimize performance can use CSS preprocessor to merge files.
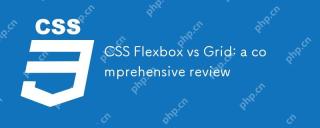
Choosing Flexbox or Grid depends on the layout requirements: 1) Flexbox is suitable for one-dimensional layouts, such as navigation bar; 2) Grid is suitable for two-dimensional layouts, such as magazine layouts. The two can be used in the project to improve the layout effect.
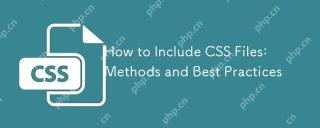
The best way to include CSS files is to use tags to introduce external CSS files in the HTML part. 1. Use tags to introduce external CSS files, such as. 2. For small adjustments, inline CSS can be used, but should be used with caution. 3. Large projects can use CSS preprocessors such as Sass or Less to import other CSS files through @import. 4. For performance, CSS files should be merged and CDN should be used, and compressed using tools such as CSSNano.
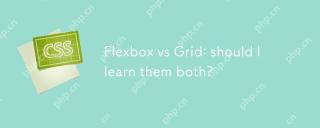
Yes,youshouldlearnbothFlexboxandGrid.1)Flexboxisidealforone-dimensional,flexiblelayoutslikenavigationmenus.2)Gridexcelsintwo-dimensional,complexdesignssuchasmagazinelayouts.3)Combiningbothenhanceslayoutflexibilityandresponsiveness,allowingforstructur
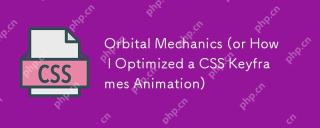
What does it look like to refactor your own code? John Rhea picks apart an old CSS animation he wrote and walks through the thought process of optimizing it.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
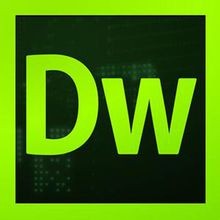
Dreamweaver CS6
Visual web development tools
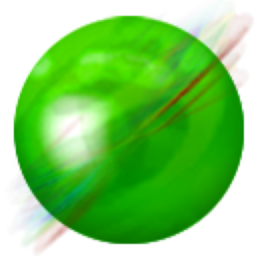
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
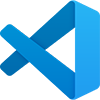
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
