Yii Security Hardening: Protecting Your Applications from Vulnerabilities
In the Yii framework, the application can be protected by the following steps: 1) Enable CSRF protection, 2) Implement input verification, and 3) Use output escape. These measures protect against CSRF, SQL injection and XSS attacks by embedding CSRF tokens, defining verification rules and automatic HTML escapes, ensuring the security of the application.
introduction
In today's online world, security is not just an option, it's a must. As an experienced developer, I know the importance of security reinforcement when developing applications using the Yii framework. This article will explore in-depth how to use the Yii framework to protect your application from various vulnerabilities. Whether you are a beginner or an experienced developer, after reading this article, you will master a range of practical security strategies and techniques to ensure your Yii application is more solid.
Review of basic knowledge
Yii is a high-performance PHP framework that has been designed with security in mind. Understanding Yii's security features, such as CSRF protection, input verification, and output escaping, is the basis for building secure applications. Yii's security components provide multiple ways to protect your application from common web attacks, such as SQL injection, XSS attacks, etc.
When using Yii, it is crucial to be familiar with its built-in security features. For example, Yii's yii\web\Request
class provides automatic protection against CSRF attacks, while yii\filters\AccessControl
helps you manage user permissions and access control.
Core concept or function analysis
Definition and function of Yii security function
The Yii framework provides a variety of security features to protect your application. The most important ones include:
- CSRF Protection : Yii prevents cross-site request forgery attacks by embedding a CSRF token in each request.
- Input Verification : Yii's model class provides powerful input verification functions to ensure that the data entered by the user meets the expected format.
- Output escape : Yii automatically escapes the output to prevent XSS attacks.
A simple example is how to enable CSRF protection in Yii:
// Enable CSRF protection in your configuration file 'components' => [ 'request' => [ 'enableCsrfValidation' => true, ], ],
How it works
How does Yii's security function work? Let's take a closer look:
CSRF protection : Yii embeds a unique CSRF token in each form and verifies the token when processing a POST request. If the token does not match, Yii will reject the request. This method effectively prevents malicious websites from using the user's identity to perform unauthorized operations.
Input Verification : Yii's model class validates input data by defining rules. For example, the
required
rule ensures that a field cannot be empty, and theemail
rule ensures that a valid email address is entered. When verification fails, Yii will throw an exception to prevent unsafe data from entering the system.Output escape : Yii automatically performs HTML escape when outputting data to prevent XSS attacks. For example,
Html::encode()
method converts special characters to HTML entities, ensuring that malicious code cannot be executed.
Example of usage
Basic usage
Let's look at a simple example of how to use input validation and output escape in Yii:
// Verification rules in model class public function rules() { Return [ [['username', 'password'], 'required'], ['email', 'email'], ]; } // Use output escape in view <?= Html::encode($model->username) ?>
These basic usages ensure that the data entered by the user is safe and that no XSS vulnerabilities are introduced when output.
Advanced Usage
For more complex scenarios, you may need to customize the verification rules or use more advanced security features. For example, how to implement custom validation rules in Yii:
// Custom validation rules public function rules() { Return [ ['password', 'validatePasswordStrength'], ]; } public function validatePasswordStrength($attribute, $params) { if (!preg_match('/^(?=.*[az])(?=.*[AZ])(?=.*\d)[a-zA-Z\d]{8,}$/', $this->$attribute)) { $this->addError($attribute, 'Password must contain at least 8 characters, including uppercase, lowercase, and numbers.'); } }
This example shows how to verify password strength through regular expressions to ensure that the password set by the user is safe enough.
Common Errors and Debugging Tips
Common errors when using Yii include:
- Forgot to enable CSRF protection : This may make your application vulnerable to CSRF attacks. Make sure
enableCsrfValidation
is enabled in the configuration file. - Incorrect input validation : If the verification rules are incomplete, it may cause SQL injection or other security issues. Ensure that all user input is strictly verified.
- Ignore output escapes : Direct output of unescaped data may result in XSS attacks. Always use
Html::encode()
or other escape methods.
Methods to debug these problems include:
- Debugging Tools with Yii : Yii provides powerful debugging tools that can help you identify and fix security issues.
- Logging : Enable detailed logging to help you track and analyze security events.
- Security Testing : Regular security testing is performed to ensure that your application has no new vulnerabilities.
Performance optimization and best practices
In practical applications, the following points need to be considered to optimize the security of Yii applications:
Performance vs. Safety Balance : While security is important, excessive safety measures can affect performance. For example, too many verification rules may increase server load. Find a balance point to ensure both safety and performance are guaranteed.
-
Best Practices :
- Using Yii's built-in security features : Yii's security components have been extensively tested to ensure that these features are used to protect your application.
- Periodic updates : The Yii framework and its dependency libraries will release security updates regularly to ensure that your app always uses the latest version.
- Code review : Regular code reviews are performed to ensure no new security vulnerabilities are introduced.
- User Education : Educate users how to use your app safely, such as setting strong passwords, identifying phishing emails, etc.
Through these strategies and best practices, you can significantly improve the security of your Yii app and protect your app from vulnerabilities.
The above is the detailed content of Yii Security Hardening: Protecting Your Applications from Vulnerabilities. For more information, please follow other related articles on the PHP Chinese website!
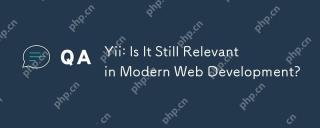
Yiiremainsrelevantinmodernwebdevelopmentforprojectsneedingspeedandflexibility.1)Itoffershighperformance,idealforapplicationswherespeediscritical.2)Itsflexibilityallowsfortailoredapplicationstructures.However,ithasasmallercommunityandsteeperlearningcu
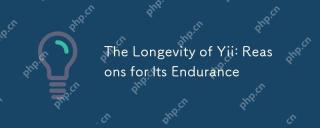
Yii frameworks remain strong in many PHP frameworks because of their efficient, simplicity and scalable design concepts. 1) Yii improves development efficiency through "conventional optimization over configuration"; 2) Component-based architecture and powerful ORM system Gii enhances flexibility and development speed; 3) Performance optimization and continuous updates and iterations ensure its sustained competitiveness.
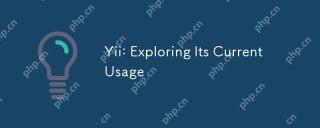
Yii is still suitable for projects that require high performance and flexibility in modern web development. 1) Yii is a high-performance framework based on PHP, following the MVC architecture. 2) Its advantages lie in its efficient, simplified and component-based design. 3) Performance optimization is mainly achieved through cache and ORM. 4) With the emergence of the new framework, the use of Yii has changed.
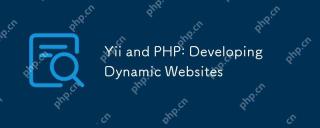
Yii and PHP can create dynamic websites. 1) Yii is a high-performance PHP framework that simplifies web application development. 2) Yii provides MVC architecture, ORM, cache and other functions, which are suitable for large-scale application development. 3) Use Yii's basic and advanced features to quickly build a website. 4) Pay attention to configuration, namespace and database connection issues, and use logs and debugging tools for debugging. 5) Improve performance through caching and optimization queries, and follow best practices to improve code quality.
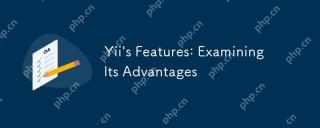
The Yii framework stands out in the PHP framework, and its advantages include: 1. MVC architecture and component design to improve code organization and reusability; 2. Gii code generator and ActiveRecord to improve development efficiency; 3. Multiple caching mechanisms to optimize performance; 4. Flexible RBAC system to simplify permission management.
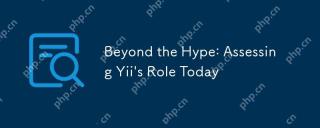
Yii remains a powerful choice for developers. 1) Yii is a high-performance PHP framework based on the MVC architecture and provides tools such as ActiveRecord, Gii and cache systems. 2) Its advantages include efficiency and flexibility, but the learning curve is steep and community support is relatively limited. 3) Suitable for projects that require high performance and flexibility, but consider the team technology stack and learning costs.
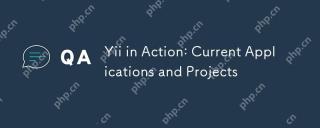
Yii framework is suitable for enterprise-level applications, small and medium-sized projects and individual projects. 1) In enterprise-level applications, Yii's high performance and scalability make it outstanding in large-scale projects such as e-commerce platforms. 2) In small and medium-sized projects, Yii's Gii tool helps quickly build prototypes and MVPs. 3) In personal and open source projects, Yii's lightweight features make it suitable for small websites and blogs.
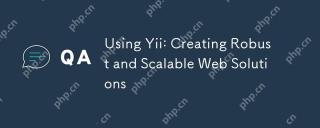
The Yii framework is suitable for building efficient, secure and scalable web applications. 1) Yii is based on the MVC architecture and provides component design and security features. 2) It supports basic CRUD operations and advanced RESTfulAPI development. 3) Provide debugging skills such as logging and debugging toolbar. 4) It is recommended to use cache and lazy loading for performance optimization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
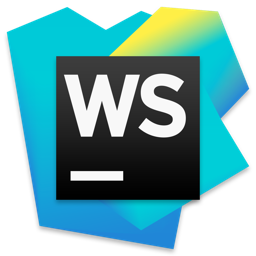
WebStorm Mac version
Useful JavaScript development tools
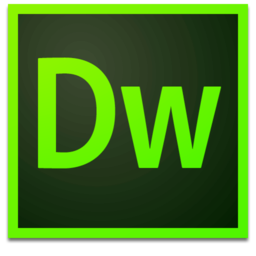
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
