C Performance Optimization: Techniques for High-Performance Applications
C performance optimization can be achieved through code level, compiler, and runtime optimization. 1) Use inline functions to reduce call overhead. 2) Optimize the loop, such as loop expansion. 3) Use const keyword and modern C features such as std::move to improve efficiency. Through these strategies and best practices, the performance of C programs can be effectively improved.
introduction
In the pursuit of high-performance applications, C, as a powerful programming language, provides a wealth of optimization tools and techniques. Today we will explore various technologies for C performance optimization to help you create efficient and fast applications. Through this article, you will learn how to optimize code from the bottom layer, understand how the compiler works, and how to use the features of modern C to improve program performance.
Review of basic knowledge
Before we start to deepen our optimization, let’s review several key concepts related to performance in C. As a statically typed language, C provides rich underlying control capabilities, including memory management, pointer operations and inline functions. These characteristics give C a unique advantage in performance optimization.
For example, understanding C's memory management mechanism is crucial because improper memory usage can lead to performance bottlenecks. In addition, being familiar with compiler optimization options and linker usage can also help us optimize our code better.
Core concept or function analysis
Definition and role of performance optimization
Performance optimization in C refers to the process of improving program execution efficiency through various technologies and strategies. Its function is to reduce program running time, reduce memory usage, and improve the overall system response speed. Through optimization, we can enable the program to maximize performance under limited resources.
A simple example is to use inline functions to reduce the overhead of function calls:
// Inline function example inline int add(int a, int b) { return ab; } int main() { int result = add(3, 4); return 0; }
How it works
How C Performance Optimization works involves multiple levels, from code-level optimization to compiler and linker optimization to runtime optimization. Code-level optimization includes using appropriate data structures, reducing unnecessary function calls, optimizing loops, etc.
Compiler optimization is performed by analyzing the code and performing automatic optimization, such as loop expansion, dead code elimination, and register allocation. Linker optimization can help us better manage the memory layout of our programs, reduce page errors and improve cache hits.
For example, consider a loop optimization:
// Original loop for (int i = 0; i < n; i) { sum = arr[i]; } // Optimized loop (loop expansion) for (int i = 0; i < n; i = 4) { sum = arr[i] arr[i 1] arr[i 2] arr[i 3]; }
Loop expansion can reduce the overhead of loop control, but it should be noted that this optimization may increase code size, which affects cache performance.
Example of usage
Basic usage
Let's look at a basic performance optimization example, using the const
keyword to improve the execution efficiency of the code:
// Use const to optimize void process(const int* arr, int size) { int sum = 0; for (int i = 0; i < size; i) { sum = arr[i]; } // Use sum }
By using const
we tell the compiler that this data will not be modified, which may enable more optimizations.
Advanced Usage
In more advanced usage, we can take advantage of modern C features such as std::move
and std::forward
to optimize the movement and forwarding of objects:
// Use std::move and std::forward template<typename T> void process(T&& obj) { T temp = std::forward<T>(obj); // Use temp }
This technique can reduce unnecessary copying and improve the efficiency of the program.
Common Errors and Debugging Tips
Common errors during performance optimization include over-optimization, ignoring the readability and maintenance of the code, and performance degradation caused by improper use of optimization techniques. For example, excessive use of inline functions may increase the code size, resulting in a decrease in cache hit rate.
Methods to debug these problems include using performance analysis tools such as gprof
or Valgrind
to identify performance bottlenecks and improve code through step-by-step optimization.
Performance optimization and best practices
In practical applications, performance optimization needs to be combined with specific scenarios and requirements. Here are some optimization strategies and best practices:
Using the right data structure : Choosing the right data structure can significantly improve the performance of the program. For example, using
std::vector
instead ofstd::list
can improve cache friendliness.Reduce unnecessary function calls : Inline functions or use lambda expressions to reduce the overhead of function calls.
Optimize cycles : The execution efficiency of cycles can be improved through technologies such as loop expansion and loop fusion.
Using modern C features : modern C features such as
auto
,constexpr
, andstd::array
can help us write more efficient code.Compiler Optimization : Use compiler optimization options such as
-O3
or-Ofast
to enable more optimizations.Code readability and maintenance : While pursuing performance, do not ignore the readability and maintenance of the code. Good code structure and comments can help the team better understand and maintain the code.
Through these strategies and best practices, we can effectively improve the performance of C programs while maintaining code readability and maintainability. In actual projects, performance optimization is an ongoing process that requires continuous testing, analysis and improvement. Hope this article provides you with useful guidance and helps you create high-performance C applications.
The above is the detailed content of C Performance Optimization: Techniques for High-Performance Applications. For more information, please follow other related articles on the PHP Chinese website!
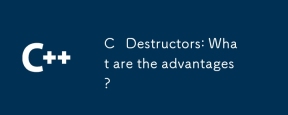
C destructorsprovideseveralkeyadvantages:1)Theymanageresourcesautomatically,preventingleaks;2)Theyenhanceexceptionsafetybyensuringresourcerelease;3)TheyenableRAIIforsaferesourcehandling;4)Virtualdestructorssupportpolymorphiccleanup;5)Theyimprovecode
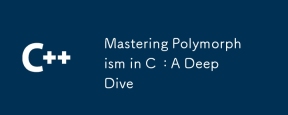
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
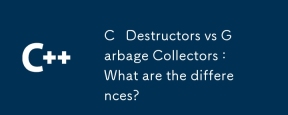
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
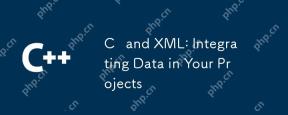
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
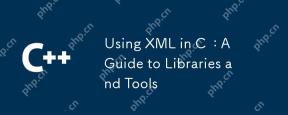
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
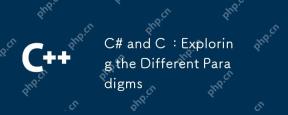
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
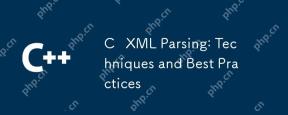
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
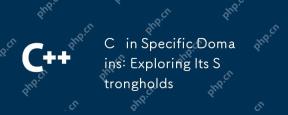
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
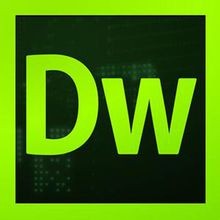
Dreamweaver CS6
Visual web development tools
