Redis performance optimization can be achieved through the following steps: 1. Configuration adjustment: Set maxmemory, maxmemory-policy and appendfsync parameters. 2. Data structure selection: Select the appropriate data structure, such as strings, hash tables or collections, according to the data access mode. 3. Command optimization: Avoid using high-complexity commands such as KEYS and use SCAN instead. 4. Use Pipeline to reduce network overhead, 5. Implement sharding to improve overall performance, 6. Use cache reasonably to optimize application response speed, 7. Apply data expiration strategies, monitoring and code optimization to ensure efficiency and stability.
introduction
Redis, as a high-performance in-memory database, has become an important part of modern application architecture. Whether you're just starting out with Redis or already relying on it deeply in your project, performance tuning and optimization techniques are key skills you need to master. This article will take you deeper into Redis’s performance optimization journey, allowing you to understand not only how Redis works, but also how to make it run faster and handle more requests.
In this article, we will start with the basic concepts of Redis and gradually deepen into the specific implementation of performance tuning and optimization techniques. You will learn how to improve Redis' performance through configuration adjustment, data structure selection, command optimization and other methods. Whether you are a developer, system administrator, or performance optimization expert, here are the knowledge and skills you need.
Redis basic review
The charm of Redis is its speed and flexibility. As an in-memory database, it can handle various data operations at a response time of microseconds. Here, we quickly review some of the key features of Redis:
- Memory storage : Redis stores data in memory, which makes it read and write extremely fast.
- Diverse data structures : Redis supports a variety of data structures, such as strings, lists, collections, hash tables, etc., which makes it useful in various scenarios.
- Persistence : Although Redis mainly runs in memory, it also provides two persistence mechanisms: RDB and AOF to ensure data security.
Understanding these basic features is critical for subsequent performance optimization, as they directly affect Redis' performance performance.
The core concept of performance tuning
Configuration adjustment
The performance of Redis depends to a large extent on its configuration. Let's look at several key configuration parameters:
- maxmemory : Sets the maximum memory that Redis can use. This is crucial to prevent Redis from crashing due to insufficient memory.
- maxmemory-policy : When maxmemory is reached, Redis will decide how to delete old data based on this policy.
- appendfsync : Controls the frequency of AOF persistence, and this parameter has a direct impact on write performance.
# Example configuration maxmemory 4gb maxmemory-policy allkeys-lru appendfsync everysec
These configurations affect not only Redis' performance, but also its behavior and stability. In practical applications, these parameters need to be adjusted according to specific business needs.
Data structure selection
Redis provides a variety of data structures, each with its unique performance characteristics. Choosing the right data structure can significantly improve performance. For example:
- String : Suitable for simple data storage and counters.
- Hash table : suitable for storing objects and saving memory.
- Collection : Suitable for deduplication and intersection and union operations.
When selecting a data structure, you need to consider the access mode and operation frequency of the data. For example, if you need to frequently deduplicate data, sets may be a better choice.
Command Optimization
Redis command performance varies greatly. Some commands such as SET
and GET
are very efficient, while some such as KEYS
can cause performance problems. Understanding the performance characteristics of these commands and using them reasonably in your application is the key to optimizing Redis performance.
For example, avoid using the KEYS
command to traverse all keys because it is an O(N) complexity operation. Instead, the SCAN
command can be used, which can gradually traverse all keys with lower overhead.
# Use SCAN instead of KEYS SCAN 0 MATCH * COUNT 100
Example of usage
Basic usage
Let's look at a simple example of how to use Redis to store and retrieve user information:
# Set user information SET user:1:name "John Doe" SET user:1:email "john.doe@example.com" # Get user information GET user:1:name GET user:1:email
This example shows the basic usage of Redis, which is simple and efficient.
Advanced Usage
Now, let's look at a more complex example showing how to use Redis' collection to implement the attention feature of a simple social network:
# User 1 Follow User 2 and User 3 SADD user:1: following user:2 user:3 # User 2 Follow User 1 SADD user:2: following user:1 # Get user 1's follower list SMEMBERS user:1:following # Calculate the users who are concerned about SINTER user:1:following user:2:following
This example demonstrates the power of Redis collections and is suitable for scenarios where collection operations need to be handled efficiently.
Common Errors and Debugging Tips
Common errors when using Redis include:
- Memory Leaks : Since Redis runs primarily in memory, it can lead to memory leaks if data is not managed correctly.
- Blocking operations : Some commands such as
KEYS
orSORT
operations with large data volumes may cause Redis to block, affecting performance.
Methods to debug these problems include:
- Use the
INFO
command to view Redis's memory usage and connection status. - Use
MONITOR
command to monitor Redis operations in real time to identify potential performance bottlenecks.
# Check memory usage INFO memory # Real-time monitoring of Redis operations MONITOR
Performance optimization and best practices
Performance optimization
The key to optimizing Redis performance is understanding how it works and bottlenecks. Here are some effective optimization strategies:
- Using Pipeline : Packaging multiple commands can reduce network overhead and improve throughput.
# Send multiple commands using Pipeline MULTI SET user:1:name "John Doe" SET user:1:email "john.doe@example.com" EXEC
Sharding : Distribute data on multiple Redis instances to improve overall performance and capacity.
Cache optimization : Using Redis as the cache layer rationally can significantly improve the response speed of the application.
Best Practices
Here are some best practices when using Redis:
- Data expiration policy : Use the
EXPIRE
command to set the expiration time of the data to prevent memory overflow. - Monitor and log : Regularly monitor Redis's performance metrics and use log analysis tools to find performance bottlenecks.
- Code optimization : In application code, use Redis commands reasonably to avoid unnecessary network requests and data transmission.
With these optimizations and best practices, you can make Redis the most effective in your application while remaining efficient and stable.
In Redis's performance optimization journey, we should not only focus on technical details, but also consider application architecture and data flow from a global perspective. I hope this article can provide you with valuable insights and practical tips, so that you can easily use Redis.
The above is the detailed content of Redis Deep Dive: Performance Tuning & Optimization Techniques. For more information, please follow other related articles on the PHP Chinese website!
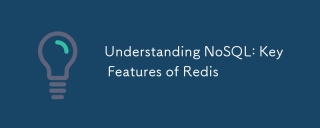
Key features of Redis include speed, flexibility and rich data structure support. 1) Speed: Redis is an in-memory database, and read and write operations are almost instantaneous, suitable for cache and session management. 2) Flexibility: Supports multiple data structures, such as strings, lists, collections, etc., which are suitable for complex data processing. 3) Data structure support: provides strings, lists, collections, hash tables, etc., which are suitable for different business needs.
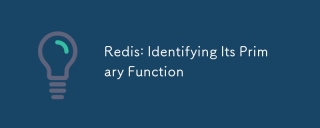
The core function of Redis is a high-performance in-memory data storage and processing system. 1) High-speed data access: Redis stores data in memory and provides microsecond-level read and write speed. 2) Rich data structure: supports strings, lists, collections, etc., and adapts to a variety of application scenarios. 3) Persistence: Persist data to disk through RDB and AOF. 4) Publish subscription: Can be used in message queues or real-time communication systems.

Redis supports a variety of data structures, including: 1. String, suitable for storing single-value data; 2. List, suitable for queues and stacks; 3. Set, used for storing non-duplicate data; 4. Ordered Set, suitable for ranking lists and priority queues; 5. Hash table, suitable for storing object or structured data.
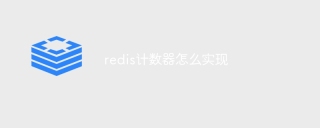
Redis counter is a mechanism that uses Redis key-value pair storage to implement counting operations, including the following steps: creating counter keys, increasing counts, decreasing counts, resetting counts, and obtaining counts. The advantages of Redis counters include fast speed, high concurrency, durability and simplicity and ease of use. It can be used in scenarios such as user access counting, real-time metric tracking, game scores and rankings, and order processing counting.

Use the Redis command line tool (redis-cli) to manage and operate Redis through the following steps: Connect to the server, specify the address and port. Send commands to the server using the command name and parameters. Use the HELP command to view help information for a specific command. Use the QUIT command to exit the command line tool.
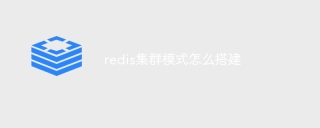
Redis cluster mode deploys Redis instances to multiple servers through sharding, improving scalability and availability. The construction steps are as follows: Create odd Redis instances with different ports; Create 3 sentinel instances, monitor Redis instances and failover; configure sentinel configuration files, add monitoring Redis instance information and failover settings; configure Redis instance configuration files, enable cluster mode and specify the cluster information file path; create nodes.conf file, containing information of each Redis instance; start the cluster, execute the create command to create a cluster and specify the number of replicas; log in to the cluster to execute the CLUSTER INFO command to verify the cluster status; make

To read a queue from Redis, you need to get the queue name, read the elements using the LPOP command, and process the empty queue. The specific steps are as follows: Get the queue name: name it with the prefix of "queue:" such as "queue:my-queue". Use the LPOP command: Eject the element from the head of the queue and return its value, such as LPOP queue:my-queue. Processing empty queues: If the queue is empty, LPOP returns nil, and you can check whether the queue exists before reading the element.
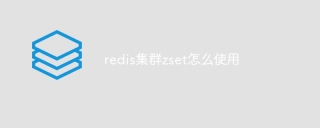
Use of zset in Redis cluster: zset is an ordered collection that associates elements with scores. Sharding strategy: a. Hash sharding: Distribute the hash value according to the zset key. b. Range sharding: divide into ranges according to element scores, and assign each range to different nodes. Read and write operations: a. Read operations: If the zset key belongs to the shard of the current node, it will be processed locally; otherwise, it will be routed to the corresponding shard. b. Write operation: Always routed to shards holding the zset key.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.