Event bus is a design pattern (although we will discuss JavaScript here, it is a design pattern in any language) that can be used to simplify communication between different components. It can also be considered a publish/subscribe or pubsub.
The idea is that components can listen to the event bus to understand when their operations are performed. For example, the Tab Panel component may listen for events indicating that it changes the active tab. Of course, this may be caused by clicking one of the tabs, so it is handled entirely within that component. However, with the event bus, some other elements can tell the tab to make changes. Imagine an error in which form submission causes the user to receive an alert in a specific tab, so the form sends a message to the event bus, instructing the tab component to change the active tab to a tab containing the error. This is how it looks on the event bus.
The pseudo-code for this case is as follows…
// Tab component Tabs.changeTab = id => { // Change the DOM operation of the active tab. }; MyEventBus.subscribe("change-tab", Tabs.changeTab(id)); // Other components... // Something happens, and then: MyEventBus.publish("change-tab", 2);
Do you need a JavaScript library to implement this? (Tip Question: You never need a JavaScript library). Well, there are many options:
- PubSubJS
- EventEmitter3
- Postal.js
- jQuery even supports custom events, which is highly relevant to this pattern.
Also, check out Mitt, a library with only 200 bytes of gzip. This simple pattern inspired people to solve it on their own in a way that was as concise as possible.
Let's do it ourselves! We don't use any third-party libraries at all, but instead take advantage of the event listening system that is already built into JavaScript, addEventListener
we are all familiar with and love.
First, some context
The addEventListener
API in JavaScript is a member function of EventTarget
class. The reason we can bind the click event to the button is that the prototype interface of ( HTMLButtonElement
) is indirectly inherited from EventTarget
.
Unlike most other DOM interfaces, you can use the new
keyword to create EventTarget
directly . It is supported in all modern browsers, but only recently. As we can see in the screenshot above, Node
inherits EventTarget
, so all DOM nodes have method addEventListener
.
Tips
I recommend using an extremely lightweight Node type as our event listening bus: HTML comments ( <!-- -->
).
For browser rendering engines, HTML comments are just comments in the code and have no functionality other than providing descriptive text to developers. However, since comments are still written in HTML, they end up appearing in the DOM as real nodes and have their own prototype interface— Comment
—which inherits from Node
.
The Comment
class can be created directly from new
, just like EventTarget
:
const myEventBus = new Comment('my-event-bus');
We can also use the ancient but widely supported document.createComment
API. It requires a data parameter, i.e. the content of the comment. It can even be an empty string:
const myEventBus = document.createComment('my-event-bus');
Now we can use dispatchEvent
to issue an event, which accepts an Event
object. To pass user-defined event data, use CustomEvent
, where the detail
field can be used to contain any data.
myEventBus.dispatchEvent( new CustomEvent('event-name', { detail: 'event-data' }) );
Internet Explorer 9-11 supports CustomEvent
, but no version supports new CustomEvent
. Using document.createEvent
to simulate it is complex, so if IE support is important to you, there is a way to populate it.
Now we can bind the event listener:
myEventBus.addEventListener('event-name', ({ detail }) => { console.log(detail); // => event-data });
If the event is intended to fire only once, we can use { once: true }
for one-time binding. Other options are not suitable here. To remove the event listener, we can use the native removeEventListener
.
debug
The number of events bound to a single event bus can be very large. If you forget to delete them, there may also be a memory leak. What if we want to know how many events are bound to myEventBus
?
myEventBus
is a DOM node, so DevTools in the browser can check it. There we can find the event in the Elements → Event Listener tab. Be sure to uncheck "Ancestor" to hide events bound to document
and window
.
Example
One disadvantage is that the syntax of EventTarget
is slightly verbose. We can write a simple wrapper for it. Here is a demonstration in TypeScript:
class EventBus<detailtype any> { private eventTarget: EventTarget; constructor(description = '') { this.eventTarget = document.appendChild(document.createComment(description)); } on(type: string, listener: (event: CustomEvent<detailtype> ) => void) { this.eventTarget.addEventListener(type, listener); } once(type: string, listener: (event: CustomEvent<detailtype> ) => void) { this.eventTarget.addEventListener(type, listener, { once: true }); } off(type: string, listener: (event: CustomEvent<detailtype> ) => void) { this.eventTarget.removeEventListener(type, listener); } emit(type: string, detail?: DetailType) { return this.eventTarget.dispatchEvent(new CustomEvent(type, { detail })); } } // Usage const myEventBus = new EventBus<string> ('my-event-bus'); myEventBus.on('event-name', ({ detail }) => { console.log(detail); }); myEventBus.once('event-name', ({ detail }) => { console.log(detail); }); myEventBus.emit('event-name', 'Hello'); // => Hello Hello myEventBus.emit('event-name', 'World'); // => World</string></detailtype></detailtype></detailtype></detailtype>
The following demonstration provides compiled JavaScript.
That's it! We just created a dependency-free event listening bus where one component can notify another component of changes to trigger an action. Doing such operations does not require a complete library, and the possibility of it being turned on is unlimited.
The above is the detailed content of Let's Create a Lightweight Native Event Bus in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
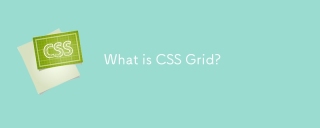
CSS Grid is a powerful tool for creating complex, responsive web layouts. It simplifies design, improves accessibility, and offers more control than older methods.
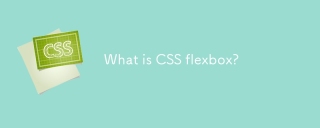
Article discusses CSS Flexbox, a layout method for efficient alignment and distribution of space in responsive designs. It explains Flexbox usage, compares it with CSS Grid, and details browser support.
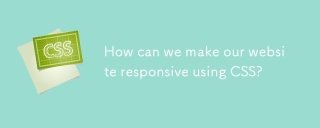
The article discusses techniques for creating responsive websites using CSS, including viewport meta tags, flexible grids, fluid media, media queries, and relative units. It also covers using CSS Grid and Flexbox together and recommends CSS framework
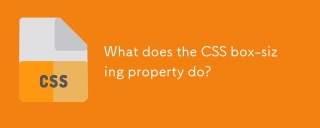
The article discusses the CSS box-sizing property, which controls how element dimensions are calculated. It explains values like content-box, border-box, and padding-box, and their impact on layout design and form alignment.
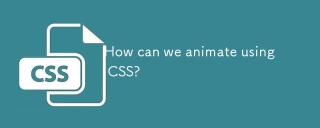
Article discusses creating animations using CSS, key properties, and combining with JavaScript. Main issue is browser compatibility.
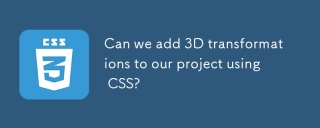
Article discusses using CSS for 3D transformations, key properties, browser compatibility, and performance considerations for web projects.(Character count: 159)
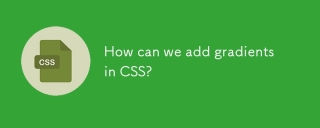
The article discusses using CSS gradients (linear, radial, repeating) to enhance website visuals, adding depth, focus, and modern aesthetics.
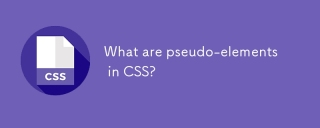
Article discusses pseudo-elements in CSS, their use in enhancing HTML styling, and differences from pseudo-classes. Provides practical examples.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
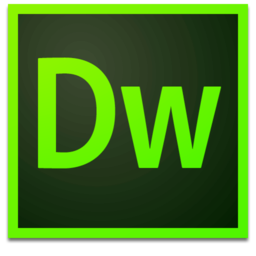
Dreamweaver Mac version
Visual web development tools
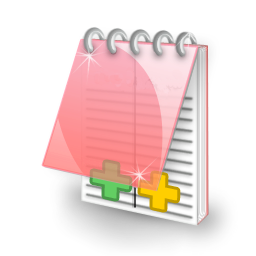
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
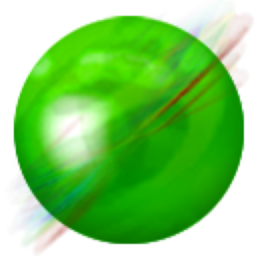
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
