Combining Bootstrap and Sass can achieve more advanced styles and theme customization: 1. Modify Bootstrap variables to change theme colors; 2. Simplify CSS writing with Sass nesting rules; 3. Use Sass' mixing and functions to create complex styles, such as buttons of different colors; 4. Pay attention to the compilation and import order of Sass files to avoid common errors; 5. Optimize performance and maintain code through the rational use of Sass functions and tools.
introduction
In modern front-end development, Bootstrap has become an indispensable tool, which provides us with the convenience of building responsive websites quickly. However, when we want to customize and optimize our design more deeply, using the default style of Bootstrap may not meet our needs. This is the time for Sass to come on. By combining Bootstrap and Sass, we can achieve more advanced style and theme customization. This article will take you into a deep understanding of how to use Sass to improve Bootstrap's styling and theme customization capabilities, helping you create a unique user interface.
Review of basic knowledge
Bootstrap is a front-end framework based on HTML, CSS and JavaScript. It provides rich components and styles to help developers quickly build beautiful website interfaces. Sass is a CSS preprocessor. It makes CSS writing more efficient and maintainable by introducing functions such as variables, nested rules, and mixins.
When using Bootstrap, we usually directly reference its precompiled CSS files, but if we want to customize the style more deeply, Sass becomes our right-hand assistant. With Sass, we can easily modify Bootstrap variables, rewrite its styles, and even create our own themes.
Core concept or function analysis
The combination of Bootstrap and Sass
Bootstrap itself supports Sass, and we can use its Sass file by downloading the source code of Bootstrap. These files contain all style definitions of Bootstrap and can be customized with Sass variables and blends.
For example, we can modify the color variable of Bootstrap to change the theme color of the entire website:
// Modify the main tone of Bootstrap $primary: #3498db; $secondary: #2ecc71; // Import Bootstrap's Sass file @import "bootstrap/scss/bootstrap";
In this way, we can easily change the default style of Bootstrap without writing CSS from scratch.
How Sass works
Sass converts Sass code to standard CSS by compiling. The power of Sass is that it allows us to use functions such as variables, nested rules, mixing, etc., which are not possible in standard CSS.
For example, we can use Sass' nesting rules to simplify writing of CSS:
.nav { ul { list-style: none; padding: 0; margin: 0; } li { display: inline-block; } }
After compilation, this Sass code will generate the following CSS:
.nav ul { list-style: none; padding: 0; margin: 0; } .nav li { display: inline-block; }
In this way, we can organize our style code more clearly, improving readability and maintainability.
Example of usage
Basic usage
Let's start with a simple example showing how to customize the button style of Bootstrap using Sass:
// Custom button style $btn-padding-y: 0.5rem; $btn-padding-x: 1rem; $btn-font-size: 1rem; $btn-border-radius: 0.25rem; // Import Bootstrap's Sass file @import "bootstrap/scss/bootstrap"; // Custom button color.custom-btn { @extend .btn; background-color: #3498db; border-color: #3498db; &:hover { background-color: #2980b9; border-color: #2980b9; } }
In this example, we first modified the button variable of Bootstrap, then imported the Sass file of Bootstrap, and finally defined a custom button style.
Advanced Usage
In more advanced usage, we can use the mix and functions of Sass to create more complex styles. For example, we can create a blend to generate buttons of different colors:
// Define a mix of generated buttons @mixin button-variant($background, $border, $hover-background, $hover-border) { background-color: $background; border-color: $border; &:hover { background-color: $hover-background; border-color: $hover-border; } } // Use mix to generate buttons of different colors.btn-primary { @include button-variant(#3498db, #3498db, #2980b9, #2980b9); } .btn-secondary { @include button-variant(#2ecc71, #2ecc71, #27ae60, #27ae60); }
In this way, we can easily generate buttons of different colors without repeatedly writing style code.
Common Errors and Debugging Tips
There are some common problems you may encounter when using Sass and Bootstrap. For example, if you modify the Sass variable but don't see any effect, it may be that you forgot to recompile the Sass file. Make sure you are using the correct compile commands, such as sass input.scss output.css
.
Another common problem is the import order of Sass files. If you import Bootstrap's Sass file and then define your own style, it may cause your style to be overwritten by Bootstrap's default style. The solution to this problem is to make sure your custom styles are imported after Bootstrap, or to override Bootstrap's styles with higher CSS priority.
Performance optimization and best practices
There are some performance optimizations and best practices to note when using Sass and Bootstrap. First, avoid overuse of nested rules, as this may generate unnecessary CSS selectors that affect performance. Secondly, the rational use of Sass variables and mixing can improve the maintainability and reusability of the code.
In actual projects, we can use tools such as Autoprefixer to add browser prefixes to ensure that our styles can be displayed normally in different browsers. At the same time, using the CSS compression tool can reduce the final generated CSS file size and improve page loading speed.
In general, combining Bootstrap and Sass allows us to implement more advanced style and theme customization in front-end development. By using Sass’s capabilities properly, we can write and maintain our style code more efficiently to create a unique user interface.
The above is the detailed content of Bootstrap with Sass: Advanced Styling & Theming Techniques. For more information, please follow other related articles on the PHP Chinese website!
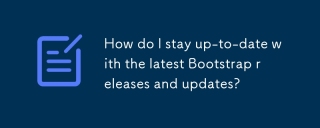
The article discusses strategies for staying updated with Bootstrap releases, accessing official documentation, best practices for integration, and community resources for discussion.
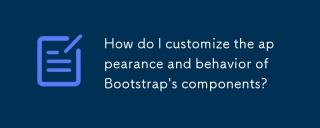
Article discusses customizing Bootstrap's appearance and behavior using CSS variables, Sass, custom CSS, JavaScript, and component modifications. It also covers best practices for modifying styles and ensuring responsiveness across devices.
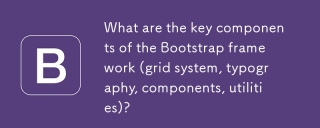
Article discusses key Bootstrap components: grid system, typography, components, and utilities. Focuses on enhancing responsive design and interactive UI creation.
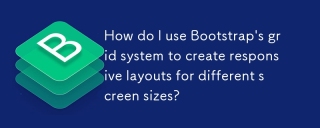
Article discusses using Bootstrap's grid system for responsive layouts across devices, detailing structure, customization, and testing tools.
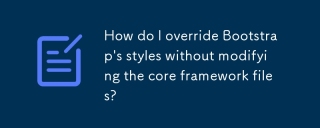
The article discusses methods to override Bootstrap's styles using custom CSS, focusing on creating separate files, using specificity, and best practices for organization.
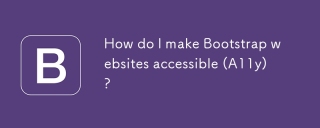
The article discusses making Bootstrap websites accessible by adhering to WCAG standards, using semantic HTML, ensuring proper contrast, enabling keyboard navigation, implementing ARIA, and conducting regular audits.
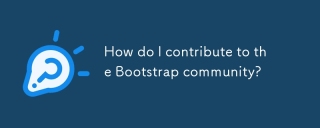
The article outlines ways to contribute to Bootstrap, including code submissions, documentation improvements, bug reporting, and community engagement. It provides detailed steps for submitting pull requests and reporting issues.
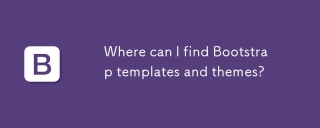
The article discusses sources for Bootstrap templates and themes, both free and premium. It covers customization and lists reputable sites for downloads.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
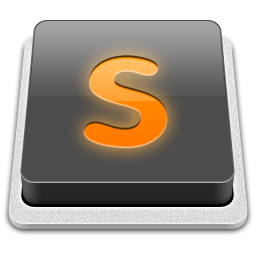
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment