Web state machines are usually implemented using JavaScript and are often leveraged by the popular XState library. But the concept of state machines works well in almost any language, surprisingly, including HTML and CSS. This article will demonstrate how to build a state machine using HTML and CSS. I recently built a website with constraints for "clientless JavaScript" and I need a unique interactive feature.
The key is to use<input type="radio">
and<form></form>
Elements to save state. The status is toggled or reset via another radio button or reset button, which can be located anywhere on the page as it connects to the same<form></form>
Label. I call this combination a single-choice reset controller, which will be explained in more detail at the end of the article. You can add more complex states using other form/input pairs. It's kind of like a checkbox trick, and eventually, the :checked
selector in CSS will do the UI work, but this is logically more advanced. I ended up using the template language (Nunjucks) in this post to maintain its manageability and configurability.
Traffic light state machine
Any state machine explanation must include mandatory traffic light examples. Below is a working traffic light that uses state machines in HTML and CSS. Clicking Next will advance the status. The code in this Pen is post-processed from the state machine template to fit the Pen. We will introduce the code later in a more readable way.
Hide/Show form information
Traffic lights are not the most practical daily UI. How to use The goal is to create a common component to control the desired state of the page. "Page status" refers to the required state of the page, and "machine status" refers to the internal state of the controller itself. The above figure shows this general-purpose state machine with four states (A, B, C, and D). Its full controller state machine is shown below. It is built using three single-choice reset controller bits. Combine three such controllers together to form a state machine with eight internal machine states (three independent radio buttons that are on or off). "Machine State" is written as a combination of three radio buttons (i.e. M001 or M101). To convert from initial M111 to M011, uncheck the selected state of the radio button for that bit by clicking another radio button in the same group. To convert back, click the attached to the bit For reusability, the component is built using the Nunjucks template macro. This allows it to be put into any page to add a state machine with the required valid state and transitions. There are four required subcomponents: The controller is built with three empty form tags and three radio buttons. The {% endmacro %} The logic for connecting the above controller to the page state is written in CSS. Checkbox tricks Use similar techniques to use checkboxes to control sibling or descendant elements. The difference here is that the button that controls the state is not tightly coupled to the element it selects. The logic below selects based on the "checked" state of the three controller radio buttons and any descendant elements with class Changing the status of a page requires a user's click or keystroke. To change a single bit of the machine state, the user clicks the radio button for the same form and radio group that is connected to the bits of the controller. To reset it, the user clicks the reset button for the form connected to the same radio button. Radio buttons or reset buttons are displayed only based on the status they are in. Any conversion macros that are validly converted are added to HTML. Multiple transformations can be placed anywhere on the page. All currently inactive transitions will be hidden. The above three components are sufficient. Any element that depends on a state should apply the class so that it can be hidden in other states. This can get very confusing. The following macros are used to simplify this process. If the given element should only be displayed in state A, the {{showA()}} macro adds the state to be hidden. The following are the markers for the traffic light example. Template macros are imported on the first line of the file. CSS logic is added to the header, and the controller is at the top of the body. The state class is located on each light of the View the demo The state machine component here contains up to four states, which is sufficient for many use cases, especially if multiple independent state machines can be used on a single page. That is, this technique can be used to build a state machine with more than four states. The following table shows how many page states can be built by adding more bits. Note that even-digit folding efficiency is not high, which is why both three and four bits are limited to four page states. The trick of being able to display, hide or control HTML elements anywhere on the page without JavaScript, I call it a single-choice reset controller. Use three This shows a minimal implementation. The hidden radio button and its controlled div need to be a sibling element, but the input is hidden and the user never needs to interact directly with it. It is set by the default selected value, cleared by another radio button, and reset by the form reset button. Just two lines of CSS are required to make it work. This mode works, but I don't recommend using it for all cases. In most cases, JavaScript is the right way to add interactivity to the web. I realized that posting this may be criticized by experts in accessibility and semantic tagging. I'm not an accessibility expert and implementing this pattern can cause problems. Or maybe not. A properly marked button can operate on the page, which is controlled by other hidden inputs, which may work well. Like anything else in the field of accessibility: testing is required. Also, I haven't seen anyone else writing about how to do this, and I think that knowledge is useful - even if it only works for rare or extreme situations. GitHub Repo Woolen cloth? There are two states (A and B) that change from two different locations in the design, affecting changes throughout the UI. This is possible because the empty state is saved
<input type="radio">
Elements and<form></form>
The element is at the top of the marker, so its state can be inferred using a regular sibling selector, and the rest of the UI can be accessed using a descendant selector. There is a loose coupling between the UI and the tag here, which means we can change the state of almost any element on the page from anywhere on the page. General four-state components
<form></form>
the reset button, which will restore the default selected state. Although this machine has eight states in total, only certain transitions are possible. For example, it is not possible to go directly from M111 to M100 because it requires two bits to be flipped. However, if we collapse these eight states into four states so that each page state shares two machine states (e.g., A shared states M111 and M000), then there is only one transition from any page state to any other page state. Reusable four-state components
Controller
checked
property of each radio button is selected by default. Each button is connected to a form, they are independent of each other and have their own radio group name. These inputs are hidden using display: none
, as they are not changed or viewed directly. The states of these three inputs constitute the machine state, and this controller is located at the top of the page. <code>{% macro FSM4S_controller()%}</code>
CSS logic
.M000
. This state machine hides any element with .M000
class by setting display: none !important
. !important
is not an important part of logic here and can be removed; it just prioritizes hiding not being overwritten by other CSS. <code>{%macro FSM4S_css()%} /* Hide M000 (A1) */ input[data-rrc="Bx00"]:not(:checked)~input[data-rrc="B0x0"]:not(:checked)~input[data-rrc="B00x"]:not(:checked)~* .M000 { display: none !important; } /* one section for each of 8 Machine States */ {%endmacro%}</code>
Conversion control
<code>{%macro AtoB(text="B",, classBtn="",classLbl="",classInp="")%} <label>{{text}}</label> {{text}} {%endmacro%}</code>
Status Class
<code>{%macro showA() %} M001 M010 M100 M101 M110 M011 {%endmacro%}</code>
Combine together
.traffic-light
element. The lit signal has the {{showA()}} macro, while the "off" version of the signal has .M000
and .M111
classes to hide it in A state. The status transition button is located at the bottom of the page. <code>{% import "rrc.njk" as rrc %}<meta charset="UTF-8"></code><title> Traffic Light State Machine Example</title><link href="styles/index.processed.css" rel="stylesheet">
{{rrc.FSM4S_css()}}
{{rrc.FSM4S_controller()}}
<div>
<div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
<div></div>
</div>
<div>
<div>
{{rrc.AtoC(text="NEXT", classInp="control-input",
classLbl="control-label",classBtn="control-button")}}
{{rrc.CtoB(text="NEXT", classInp="control-input",
classLbl="control-label",classBtn="control-button")}}
{{rrc.BtoA(text="NEXT", classInp="control-input",
classLbl="control-label",classBtn="control-button")}}
</div>
</div>
</div>
Extend to more states
Single-select reset controller details
<form></form>
Tags and a row of CSS, control buttons and controlled elements can be placed anywhere after the controller. The controlled side uses a hidden radio button that is selected by default. The radio button is connected to an empty one by ID<form></form>
element. The form has a type="reset"
button and another radio input that together form the controller.<code></code>
<label>Show</label> Hide <div>Controlled from anywhere</div>
<code>input[name='rrc-group']:checked .controlled-rrc { display: none; } .hidden { display: none; }</code>
:checked
pseudo-selector connects hidden input to the sibling element it is controlling. It adds a radio input and reset button that can be styled into a single toggle, which is shown in the following image: Accessibility… Should you do this?
The above is the detailed content of A Complete State Machine Made With HTML Checkboxes and CSS. For more information, please follow other related articles on the PHP Chinese website!
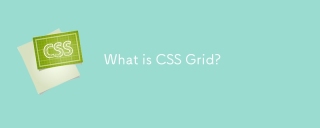
CSS Grid is a powerful tool for creating complex, responsive web layouts. It simplifies design, improves accessibility, and offers more control than older methods.
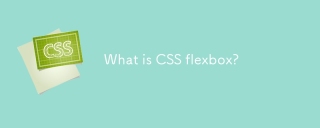
Article discusses CSS Flexbox, a layout method for efficient alignment and distribution of space in responsive designs. It explains Flexbox usage, compares it with CSS Grid, and details browser support.
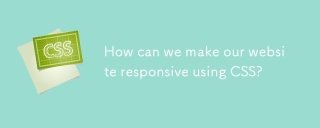
The article discusses techniques for creating responsive websites using CSS, including viewport meta tags, flexible grids, fluid media, media queries, and relative units. It also covers using CSS Grid and Flexbox together and recommends CSS framework
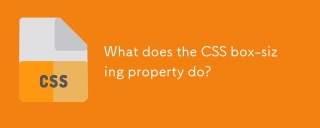
The article discusses the CSS box-sizing property, which controls how element dimensions are calculated. It explains values like content-box, border-box, and padding-box, and their impact on layout design and form alignment.
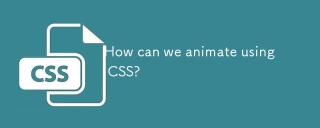
Article discusses creating animations using CSS, key properties, and combining with JavaScript. Main issue is browser compatibility.
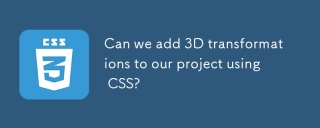
Article discusses using CSS for 3D transformations, key properties, browser compatibility, and performance considerations for web projects.(Character count: 159)
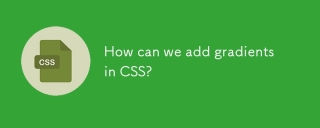
The article discusses using CSS gradients (linear, radial, repeating) to enhance website visuals, adding depth, focus, and modern aesthetics.
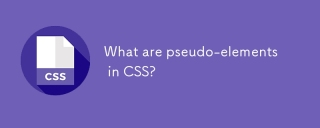
Article discusses pseudo-elements in CSS, their use in enhancing HTML styling, and differences from pseudo-classes. Provides practical examples.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
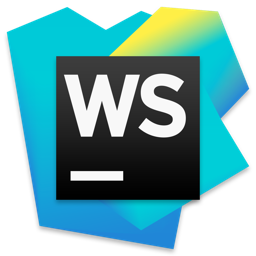
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
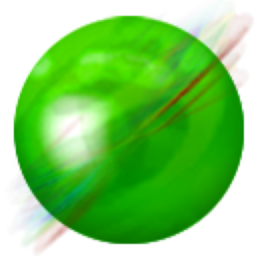
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment
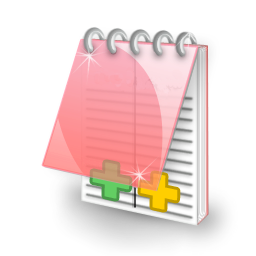
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
