How do you manage global styles in a framework-based project?
In a framework-based project, managing global styles effectively involves a combination of organizing the style files, leveraging the framework's built-in features, and maintaining a consistent approach throughout the project lifecycle. Here are some strategies to consider:
-
Centralized Style Files: In many frameworks like React, Vue.js, and Angular, it's common to create a global style file (e.g.,
styles.css
orglobal.scss
) that is imported into the main application file. This approach helps keep all global styles in one place, making it easier to maintain and update. - Modular Approach: Even within a global style file, styles can be organized into modules. For instance, you can have sections for typography, colors, spacing, etc. This modular approach within the global styles helps keep the file organized and makes it easier to find and modify specific styles.
- Framework-Specific Features: Utilize framework-specific features like CSS-in-JS in React (e.g., styled-components), scoped styles in Vue.js, or style encapsulation in Angular. These features can help manage the scope of styles more effectively.
- CSS Preprocessors: Using CSS preprocessors like Sass or Less can be beneficial. They offer features like nesting, variables, and mixins that help in keeping the styles DRY (Don't Repeat Yourself) and maintainable.
- Version Control and Documentation: Ensure that changes to global styles are tracked using version control systems like Git, and document the purpose and impact of these changes. This helps maintain the history and reasoning behind style decisions.
By following these strategies, you can manage global styles in a way that is scalable, maintainable, and consistent across the project.
What are the best practices for maintaining consistent global styles across different components?
Maintaining consistent global styles across different components is crucial for a cohesive user interface. Here are some best practices to achieve this:
- Define a Design System: Create a comprehensive design system that outlines typography, colors, spacing, and other visual elements. This system should be followed across all components to ensure consistency.
- Use CSS Variables: CSS custom properties (variables) allow you to define reusable values for properties. By using variables for colors, fonts, and other common properties, you can easily update these values globally.
- Consistent Naming Conventions: Establish a naming convention for classes and other style selectors. This helps developers understand and use the styles more easily, reducing the chances of creating conflicting or redundant styles.
- Modular and Reusable Components: Build components that are modular and reusable. By using these components consistently across the application, you ensure that the same styles are applied uniformly.
- Regular Audits and Reviews: Conduct regular style audits to check for consistency and adherence to the design system. Peer reviews during development can also help catch any deviations from the established styles early.
- Automated Testing for Styles: Use tools like CSS regression testing to automatically check if changes to global styles have unintended effects on the UI. This can help maintain consistency over time.
By adhering to these practices, you can ensure that your global styles remain consistent and cohesive across all components of your project.
How can you override global styles for specific components without affecting the rest of the project?
Overriding global styles for specific components while keeping the rest of the project unaffected is a common requirement in web development. Here are some effective methods to achieve this:
-
Scoped Styles: Many modern frameworks like Vue.js and Angular support scoped styles. By adding the
scoped
attribute to a style tag, you can ensure that the styles only apply to the component they are defined in.<style scoped> .button { background-color: #ff0000; } </style>
-
CSS-in-JS: In frameworks like React, using CSS-in-JS libraries such as styled-components or emotion allows you to define styles directly within your components. These styles are automatically scoped to the component.
import styled from 'styled-components'; const StyledButton = styled.button` background-color: #ff0000; `;
-
Specificity and Selectors: Use more specific selectors to override global styles. For example, you can use a class name combined with the component's class to target it specifically.
.my-component .button { background-color: #ff0000; }
-
Inline Styles: While not always the best practice, inline styles can be used to override global styles for a specific element. This method has the highest specificity and will override other styles.
<button style="background-color: #ff0000;">Click me</button>
-
Shadow DOM: In web components, you can use the Shadow DOM to encapsulate styles. Styles defined within the Shadow DOM do not affect the rest of the document.
<template> <style> .button { background-color: #ff0000; } </style> <button class="button">Click me</button> </template>
By using these methods, you can effectively override global styles for specific components without impacting the rest of your project.
What tools or plugins can help in managing and organizing global styles effectively in a framework?
Several tools and plugins can help in managing and organizing global styles effectively within a framework. Here are some popular options:
- Sass/SCSS: While not a tool per se, Sass and SCSS are CSS preprocessors that offer powerful features like variables, nesting, and mixins. They are widely used in frameworks to manage and organize styles more effectively.
-
Styled-Components (React): This CSS-in-JS library allows you to write actual CSS code within your JavaScript. It automatically scopes styles to components and provides a way to manage global styles through the
createGlobalStyle
function.import { createGlobalStyle } from 'styled-components'; const GlobalStyle = createGlobalStyle` body { margin: 0; padding: 0; font-family: 'Arial', sans-serif; } `;
-
CSS Modules: CSS Modules are a popular way to locally scope CSS in frameworks like React. They allow you to write CSS in separate files and import them into your components, ensuring that styles are scoped to the component.
/* Button.module.css */ .button { background-color: #ff0000; }
import styles from './Button.module.css'; function Button() { return <button className={styles.button}>Click me</button>; }
-
PostCSS: PostCSS is a tool for transforming CSS with JavaScript. It can be used with plugins like
postcss-preset-env
to use modern CSS features andpostcss-nested
for nesting selectors, helping to organize styles more effectively. - Stylelint: This is a linter for CSS that helps maintain consistent style rules across your project. It can be configured to enforce specific style conventions and catch errors in your CSS.
- Prettier: While primarily a code formatter, Prettier can also format CSS, ensuring that your style files are consistently formatted, which aids in readability and maintenance.
- CSS Regression Testing Tools: Tools like Percy or BackstopJS can help you test and ensure that changes to global styles do not break the UI. They provide visual regression testing to catch unintended style changes.
By leveraging these tools and plugins, you can more effectively manage and organize global styles within your framework-based project, ensuring a more maintainable and scalable codebase.
The above is the detailed content of How do you manage global styles in a framework-based project?. For more information, please follow other related articles on the PHP Chinese website!
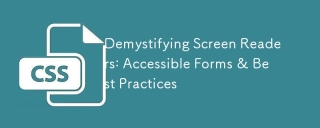
This is the 3rd post in a small series we did on form accessibility. If you missed the second post, check out "Managing User Focus with :focus-visible". In
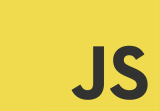
This tutorial demonstrates creating professional-looking JavaScript forms using the Smart Forms framework (note: no longer available). While the framework itself is unavailable, the principles and techniques remain relevant for other form builders.
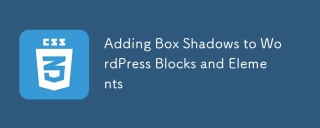
The CSS box-shadow and outline properties gained theme.json support in WordPress 6.1. Let's look at a few examples of how it works in real themes, and what options we have to apply these styles to WordPress blocks and elements.
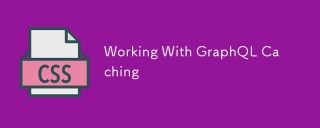
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
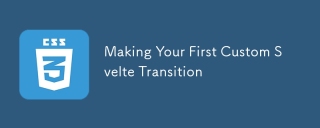
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
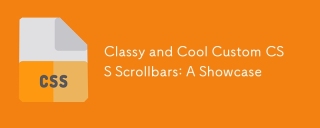
In this article we will be diving into the world of scrollbars. I know, it doesn’t sound too glamorous, but trust me, a well-designed page goes hand-in-hand
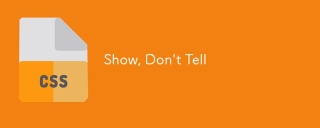
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
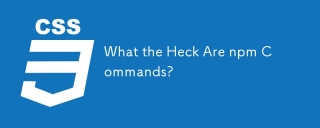
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
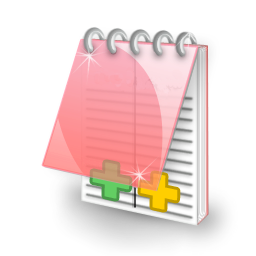
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Linux new version
SublimeText3 Linux latest version