


Explain the concept of "escape analysis" in Go and how it affects performance.
Escape analysis is a crucial optimization technique used by the Go compiler to determine where to allocate memory for variables. It essentially helps decide whether a variable should be allocated on the stack or the heap. In Go, the stack is faster and more efficient because it's automatically managed, while the heap requires manual garbage collection, which can lead to performance overhead.
The process involves analyzing whether a variable's lifetime "escapes" the scope of the function it's declared in. If a variable does not escape its function scope, it can safely be allocated on the stack. However, if a variable needs to be accessed outside the function, it must be allocated on the heap to ensure it survives beyond the function call.
The impact on performance is significant:
- Stack Allocation: When variables are allocated on the stack, they are typically faster to allocate and deallocate. This is because the stack operations are performed using simple pointer arithmetic, and the memory is automatically reclaimed when the function returns.
- Heap Allocation: Variables allocated on the heap take longer to allocate and require garbage collection, which can introduce pauses in the application. However, heap allocation is necessary when a variable's lifetime needs to extend beyond the function's scope.
Effective escape analysis can lead to better performance by reducing the need for heap allocations and garbage collection, resulting in a more efficient use of memory and CPU resources.
What specific techniques can be used to optimize escape analysis in Go programming?
To optimize escape analysis in Go programming, developers can employ several specific techniques:
-
Minimize Heap Allocations:
- Avoid using variables that escape to the heap unnecessarily. For example, instead of returning a pointer to a local variable, consider returning the value directly.
- Use stack-allocated slices and maps when possible. For instance,
make([]int, 0, 10)
can be stack-allocated if used within a function.
-
Use Inline Functions:
- Inlining can help keep variables on the stack. The Go compiler often inlines small functions automatically, but you can encourage this by keeping functions small and simple.
-
Avoid Closures with Escaping Variables:
- Be cautious with closures, especially when they capture variables that would otherwise be stack-allocated. If a closure captures a local variable and escapes the function, the variable will be moved to the heap.
-
Utilize the
go build -gcflags="-m"
Command:- This command provides detailed output about escape analysis during compilation. It helps identify which variables are escaping and why, allowing developers to refactor code to prevent unnecessary heap allocations.
-
Optimize Structs and Interfaces:
- Be mindful of how structs and interfaces are used. Passing large structs by value can prevent them from escaping, whereas passing pointers to structs can cause them to be allocated on the heap.
By applying these techniques, developers can help the Go compiler make more efficient memory allocation decisions, leading to improved application performance.
How does escape analysis influence memory allocation and garbage collection in Go?
Escape analysis plays a pivotal role in influencing memory allocation and garbage collection in Go:
-
Memory Allocation:
- Stack vs. Heap: The primary decision influenced by escape analysis is whether a variable should be allocated on the stack or the heap. Variables that do not escape their scope are allocated on the stack, which is faster and more efficient. Variables that escape are allocated on the heap, which is slower due to the overhead of dynamic memory management.
- Allocation Speed: Stack allocations are typically performed using simple pointer arithmetic and are much faster than heap allocations, which involve searching for free memory and updating allocation metadata.
-
Garbage Collection:
- Reduced Heap Pressure: By minimizing the number of heap allocations through effective escape analysis, the pressure on the garbage collector is reduced. Fewer objects on the heap mean less work for the garbage collector to do, leading to fewer pauses in the application.
- Garbage Collection Pauses: When variables are allocated on the heap, they become candidates for garbage collection. The garbage collector periodically scans the heap to identify and reclaim memory occupied by objects that are no longer reachable. Effective escape analysis can help reduce the frequency and duration of these pauses by keeping more objects on the stack.
In summary, escape analysis directly impacts the efficiency of memory management in Go by optimizing where variables are allocated and reducing the overhead associated with garbage collection.
In what scenarios might escape analysis lead to performance degradation in Go applications?
While escape analysis is generally beneficial, there are scenarios where it might lead to performance degradation in Go applications:
-
False Positives:
- Sometimes, the Go compiler may mistakenly determine that a variable escapes when it doesn't, leading to unnecessary heap allocations. This can happen with complex data structures or when using certain language features like reflection.
-
Over-Optimization:
- Aggressive optimization techniques aimed at reducing heap allocations can sometimes lead to increased complexity in the code. This added complexity might not always result in better performance and can make the code harder to maintain.
-
Interface Usage:
- When variables are assigned to interfaces, the Go compiler might not be able to determine whether the variable escapes, leading to conservative heap allocations. This can result in more heap allocations than necessary, especially if the interface is used in a way that would not typically cause an escape.
-
Large Function Scopes:
- In functions with large scopes, the compiler might have difficulty determining whether variables escape. This can result in more variables being allocated on the heap than necessary, increasing garbage collection overhead.
-
Use of Closures:
- Closures can cause variables to escape if they capture local variables that would otherwise be stack-allocated. If closures are used extensively in performance-critical sections of code, this can lead to increased heap allocations and garbage collection pressure.
Understanding these scenarios helps developers make informed decisions about when and how to apply optimizations related to escape analysis, ensuring that the balance between performance and code maintainability is maintained.
The above is the detailed content of Explain the concept of "escape analysis" in Go and how it affects performance.. For more information, please follow other related articles on the PHP Chinese website!
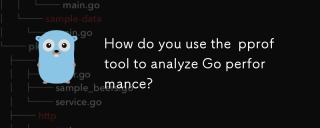
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
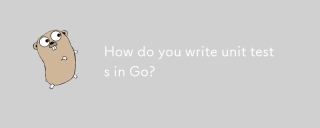
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
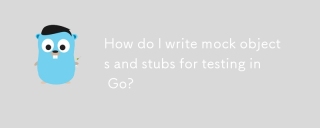
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
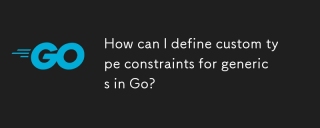
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
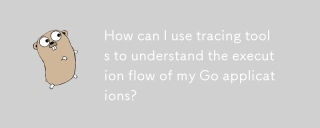
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
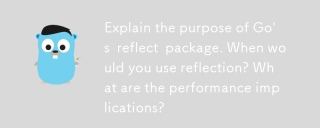
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
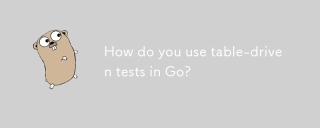
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
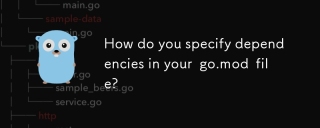
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
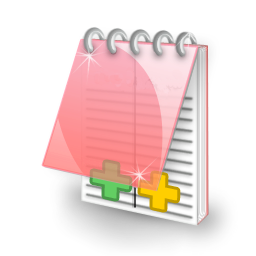
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
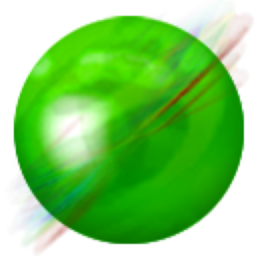
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
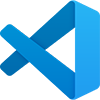
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
