


This article describes how to use Cumul.io to integrate an interactive data visualization layer into your application. To do this, we built a demo application for visualizing Spotify playlist analysis! We use Cumul.io as an interactive dashboard because it makes integration very easy and provides functionality (i.e. custom events) that allows interaction between the dashboard and the application. The application is a simple JavaScript web application with a Node.js server, but you can also use Angular, React, and React Native and use the Cumul.io dashboard to achieve the same functionality if you prefer.
Here, we build a dashboard that displays data from Kaggle Spotify dataset 1921-2020 (160,000+ tracks), as well as data obtained through the Spotify Web API when the user logs in. The dashboard we built is a deep dive into playlists and song features. We have added some Cumul.io custom events that will allow any end user accessing these dashboards to select songs from the chart and add them to their own Spotify playlist. They can also select a song to show more information and play them in the app. The code for the complete application is also available in the public open code base.
Here is a preview of the complete version's final result:
What are Cumul.io custom events and their features?
Simply put, Cumul.io custom events are a way to trigger events from the dashboard for applications that integrate dashboards. You can add custom events to the selected chart in the dashboard and have the application listen for these events.
Why? What's powerful about this tool is that it allows you to reuse data from the Analytics Dashboard (BI Tools) in the application that builds it. It allows you to define actions based on data that can be triggered directly from the integrated dashboard while keeping the dashboard, analytics layer completely separate from the application and can be managed separately.
What they contain: Cumul.io Custom events are attached to the chart , not the entire dashboard. Therefore, the information that the event has is limited to the information that the graph has.
Simply put, an event is a JSON object. This object will contain fields such as the dashboard ID that triggered it, the event name, and some other fields (depending on the chart type that triggered the event). For example, if the event was triggered from a scatter plot, you will receive the x-axis and y-axis values of the point that triggered it. On the other hand, if it is triggered from the table, you will receive a column value for example. See examples of the appearance of these events in different charts:
<code>// 表格中一行中的“添加到播放列表”自定义事件{ "type":"customEvent", "dashboard":"xxxx", "name":"xxxx", "object":"xxxx", "data":{ "language":"en", "columns":[ {"id":"Ensueno","value":"Ensueno","label":"Name"}, {"id":"Vibrasphere","value":"Vibrasphere","label":"Artist"}, {"value":0.406,"formattedValue":"0.41","label":"Danceability"}, {"value":0.495,"formattedValue":"0.49","label":"Energy"}, {"value":180.05,"formattedValue":"180.05","label":"Tempo (bpm)"}, {"value":0.568,"formattedValue":"0.5680","label":"Accousticness"}, {"id":"2007-01-01T00:00:00.000Z","value":"2007","label":"Release Date (Yr)"}, ], "event":"add_to_playlist" } }</code>
<code>//'散点图中一个点上的“歌曲信息”自定义事件{ "type":"customEvent", "dashboard":"xxxx", "name":"xxxx", "object":"xxxx", "data":{ "language":"en", "x-axis":{"id":0.601,"value":"0.601","label":"Danceability"}, "y-axis":{"id":0.532,"value":"0.532","label":"Energy"}, "name":{"id":"xxxx","value":"xxx","label":"Name"}, "event":"song_info" } }</code>
The possibilities of this feature are actually unlimited. Of course, depending on what you want to do, you may need to write a few lines of code, but this is undoubtedly a very powerful tool!
Dashboard
We don't actually cover the dashboard creation process here, but instead focus on the interactive part after integrating it into the application. The dashboard integrated in this walkthrough has been created and enabled for custom events. Of course, you can create your own dashboards and integrate them instead of our pre-built dashboards (you can create a free trial account). But before that, some background information about the Cumul.io dashboard;
Cumul.io provides a way to create dashboards within the platform or through its API. In either case, the dashboard can be used within the platform, separate from the application you want to integrate it into, so it can be maintained entirely separately.
On your login page, you will see your dashboard and can create a new dashboard:
You can open one and drag and drop any chart you want:
You can connect data, and then you can drag and drop it into these charts:
Moreover, the data can be one of many things. For example, you can connect to a pre-existing database of Cumul.io, the datasets in the database repository you use, custom built plugins, and more.
Enable custom events
We have enabled these custom events to scatter plots and tables in the dashboard used in this demo, which we will integrate in the next section. If you want to complete this step, feel free to create your own dashboard!
First, all you need to do is add custom events to the chart. To do this, first select the chart in which you want to add events to your dashboard. In the chart settings, select Interactiveness and open Custom Events :
To add an event, click Edit and define its event name and label . The event name is what your application will receive, and the tag is what will be displayed in the dashboard. In our example, we added 2 events; "Add to playlist" and "Song Information":
That's all the settings you need to do for the dashboard to trigger events at the chart level. Before leaving the editor, you will need your dashboard ID to integrate the dashboard later. You can find it in the Settings tab of the dashboard. The rest of the work is still going on at the application level. This will be where we define what we actually want to do once we receive any of these events.
Key Points
- Events work at the chart level and will contain information within the range of the chart information
- To add an event, go to the chart settings for the chart to which you want to add an event
- Defines the name and label of the event. It's done!
- (Don't forget to note the dashboard ID used for integration)
Use custom events in your own platform
Now that you have added some events to your dashboard, the next step is to use them. The key point here is that once you click on an event in the dashboard, the application that integrates the dashboard will receive an event. The Integration API provides a function to listen for these events, and then you define how to handle them. For more information about API and SDK code samples, you can also view relevant developer documentation.
In this section, we also provide an open GitHub code base (separated from the main application's code base) that you can use as a starting project to add custom events.
The structure of the cumulio-spotify-datatalks code base allows you to check out a commit named skeleton to start from scratch. All subsequent submissions will represent the steps we perform here. It is a simplified version of the complete application, focusing on the main parts of the application that demonstrates custom events. I'll skip some steps, such as the Spotify API call in src/spotify.js, to limit this tutorial to the topic of "Add and Use Custom Events".
Useful information for the following steps
- You can use the cumulio-spotify-datatalks code base and check out the "skeleton" commit as a starting point.
- All changes and code additions will be made in src/app.js.
- Dependencies and run instructions are at the end of this article.
Let's see what's going on in our example. We created two events; add_to_playlist and song_info. We want dashboard visitors to be able to add songs to their own playlist of choice in their own Spotify account. To do this, we take the following steps:
- Integrate dashboards with your application
- Listen to incoming events
Integrate dashboards with your application
First, we need to add the dashboard to our app. Here we use the Cumul.io Spotify playlist dashboard as the main dashboard and the Song Info dashboard as the drill -in dashboard (which means we create a new dashboard in the main dashboard that pops up when we trigger an event). If you have checked out a commit named skeleton and run npm start, the application should currently only open an empty "Cumul.io Favorites" tab with a login button in the upper right corner. For instructions on how to run a project locally, go to the bottom of the article:
To integrate the dashboard, we need to use the Cumulio.addDashboard() function. This function requires an object that contains dashboard options. Here are the actions we did when adding a dashboard:
In src/app.js, we create an object that stores the dashboard ID of the main dashboard and the drill-through dashboard that displays song information, and the dashboardOptions object:
<code>// 使用仪表板ID 和dashboardOptions 对象创建仪表板对象// !!!如果您想使用您自己的仪表板,请更改这些ID!!! const dashboards = { playlist: 'f3555bce-a874-4924-8d08-136169855807', songInfo: 'e92c869c-2a94-406f-b18f-d691fd627d34', }; const dashboardOptions = { dashboardId: dashboards.playlist, container: '#dashboard-container', loader: { background: '#111b31', spinnerColor: '#f44069', spinnerBackground: '#0d1425', fontColor: '#ffffff' } };</code>
We create a loadDashboard() function that calls Cumulio.addDashboard(). This function allows you to select the receive container and modify the dashboardOptions object before adding the dashboard to the application.
<code>// 创建一个loadDashboard() 函数,该函数需要一个仪表板ID 和容器const loadDashboard = (id, container) => { dashboardOptions.dashboardId = id; dashboardOptions.container = container || '#dashboard-container'; Cumulio.addDashboard(dashboardOptions); };</code>
Finally, when we load the Cumul.io favorites tab, we use this function to add our playlist dashboard:
<code>export const openPageCumulioFavorites = async () => { ui.openPage('Cumul.io 播放列表可视化', 'cumulio-playlist-viz'); /**************** 集成仪表板****************/ loadDashboard(dashboards.playlist); };</code>
At this point, we have integrated the playlist dashboard and when we click on a point in the energy/dance scatter plot by song , we get two options with custom events we added earlier. However, we haven't done anything to them yet.
Listen to incoming events
Now that we have integrated the dashboard, we can tell our application to perform actions when an event is received. The two charts here with the Add to Playlist and Song Information events are:
First, we need to set up our code to listen for incoming events. To do this, we need to use the Cumulio.onCustomEvent() function. Here we choose to wrap this function in a listenToEvents() function that can be called when we load the Cumul.io favorites tab. Then, we use the if statement to check the events we receive:
<code>const listenToEvents = () => { Cumulio.onCustomEvent((event) => { if (event.data.event === 'add_to_playlist'){ // 执行某些操作} else if (event.data.event === 'song_info'){ // 执行某些操作} }); };</code>
This is the point where things depend on your needs and creativity. For example, you can simply print a line to your console, or design your own behavior around the data you receive from the event. Alternatively, you can also use some helper functions we created that will display a playlist selector to add songs to the playlist and integrate the song information dashboard. This is how we do it;
Add songs to playlist
Here we will use the addToPlaylistSelector() function in src/ui.js. This function requires the song name and ID and will display a window containing the available playlists for the logged-in user. It will then publish a Spotify API request to add the song to the selected playlist. Since the Spotify Web API requires the song's ID to be added, we created a derived name and ID field for use in scatter plots.
The sample event we receive on add_to_playlist will contain the following for a scatter plot:
<code>"name":{"id":"So Far To Go&id=3R8CATui5dGU42Ddbc2ixE","value":"So Far To Go&id=3R8CATui5dGU42Ddbc2ixE","label":"Name & ID"}</code>
And these columns of the table:
<code>"columns":[ {"id":"Weapon Of Choice (feat. Bootsy Collins) - Remastered Version","value":"Weapon Of Choice (feat. Bootsy Collins) - Remastered Version","label":"Name"}, {"id":"Fatboy Slim","value":"Fatboy Slim","label":"Artist"}, // ... {"id":"3qs3aHNUcqFGv7jMYJJCYa","value":"3qs3aHNUcqFGv7jMYJJCYa","label":"ID"} ]</code>
We extract the name and ID of the song from the event through the getSong() function, and then call the ui.addToPlaylistSelector() function:
<code>/*********** 侦听自定义事件并添加额外内容************/ const getSong = (event) => { let songName; let songArtist; let songId; if (event.data.columns === undefined) { songName = event.data.name.id.split('&id=')[0]; songId = event.data.name.id.split('&id=')[1]; } else { songName = event.data.columns[0].value; songArtist = event.data.columns[1].value; songId = event.data.columns[event.data.columns.length - 1].value; } return {id: songId, name: songName, artist: songArtist}; }; const listenToEvents = () => { Cumulio.onCustomEvent(async (event) => { const song = getSong(event); console.log(JSON.stringify(event)); if (event.data.event === 'add_to_playlist'){ await ui.addToPlaylistSelector(song.name, song.id); } else if (event.data.event === 'song_info'){ // 执行某些操作} }); };</code>
The Add to Playlist event now displays a window with available playlists where logged in users can add songs to:
Show more song information
The last thing we want to do is to make the Song Information event show another dashboard when clicked. It will display more information about the selected song and include the option to play the song. This is also a step to get started with more complex use cases for APIs, which may require some background knowledge. Specifically, we use parameterizable filters. The idea is to create a parameter in your dashboard that defines its value when creating an authorization token . We include the parameters as metadata when creating the authorization token.
For this step, we create a songId parameter that is used for filters on the song information dashboard:
Then, we create a getDashboardAuthorizationToken() function. This requires metadata and then publish it to the /authorization endpoint of our server in server/server.js:
<code>const getDashboardAuthorizationToken = async (metadata) => { try { const body = {}; if (metadata && typeof metadata === 'object') { Object.keys(metadata).forEach(key => { body[key] = metadata[key]; }); } /*使用标头中的平台用户访问凭据向后端API 发出调用,以检索此用户的仪表板授权令牌*/ const response = await fetch('/authorization', { method: 'post', body: JSON.stringify(body), headers: { 'Content-Type': 'application/json' } }); // 使用Cumul.io 授权密钥和令牌获取JSON 结果const responseData = await response.json(); return responseData; } catch (e) { return { error: '无法检索仪表板授权令牌。' }; } };</code>
Finally, we load the songInfo dashboard when the song_info event is triggered. To do this, we use the song ID to create a new authorization token:
<code>const loadDashboard = (id, container, key, token) => { dashboardOptions.dashboardId = id; dashboardOptions.container = container || '#dashboard-container'; if (key && token) { dashboardOptions.key = key; dashboardOptions.token = token; } Cumulio.addDashboard(dashboardOptions); };</code>
We have made some modifications to the loadDashboard() function to use the new token:
<code>const loadDashboard = (id, container, key, token) => { dashboardOptions.dashboardId = id; dashboardOptions.container = container || '#dashboard-container'; if (key && token) { dashboardOptions.key = key; dashboardOptions.token = token; } Cumulio.addDashboard(dashboardOptions); };</code>
Then call ui.displaySongInfo(). The final result is as follows:
<code>const listenToEvents = () => { Cumulio.onCustomEvent(async (event) => { const song = getSong(event); if (event.data.event === 'add_to_playlist'){ await ui.addToPlaylistSelector(song.name, song.id); } else if (event.data.event === 'song_info'){ const token = await getDashboardAuthorizationToken({ songId: [song.id] }); loadDashboard(dashboards.songInfo, '#song-info-dashboard', token.id, token.token); await ui.displaySongInfo(song); } }); };</code>
Look! We're done! In this demo, we used a lot of helper functions that I didn't cover in detail, but you can feel free to clone the demo code base and use them. You can even ignore them and build your own functionality around custom events.
in conclusion
For anyone who intends to integrate data visualization and analysis layers in their applications, Cumul.io provides a very simple way to implement it, as I tried to demonstrate in this demo. The dashboard is still an entity separated from the application and can then be managed separately. If you look at integrated analytics in a business environment and you would rather not always have developers back and fiddle with the dashboard, this will be a big advantage.
On the other hand, you can trigger and listen for events in its host application from the dashboard, which allows you to define implementations based on the information in these separate dashboards. This can be anything from playing a song in our example to triggering the specific email to send. In this sense, the world is your oyster and you decide how to use data from your analytical layer. In other words, you can reuse data in the dashboard, and it doesn't have to just stay in its dashboard and analytics world?
Steps to run this project
Before starting:
- You will need a Cumul.io account.
- You need to register your application in the Spotify Developer Dashboard
- Use npm install to clone the cumulio-spotify-datatalks codebase
- Create a .env file in the root directory and add the following from your Cumul.io and Spotify developer accounts:
- From Cumul.io: CUMULIO_API_KEY=xxx CUMULIO_API_TOKEN=xxx
- From Spotify: SPOTIFY_CLIENT_ID=xxx SPOTIFY_CLIENT_SECRET=xxx ACCESS_TOKEN=xxx REFRESH_TOKEN=xxx
- Run npm start
- On your browser, go to https://www.php.cn/link/b41fa11badee2d79d4fbe0a53f8d7a15 and log in to your Spotify account?
Live demo try Cumul.io
The above is the detailed content of Embedding an Interactive Analytics Component with Cumul.io and Any Web Framework. For more information, please follow other related articles on the PHP Chinese website!
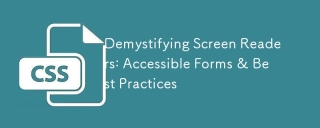
This is the 3rd post in a small series we did on form accessibility. If you missed the second post, check out "Managing User Focus with :focus-visible". In
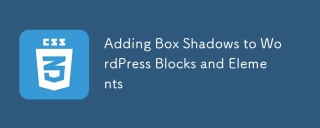
The CSS box-shadow and outline properties gained theme.json support in WordPress 6.1. Let's look at a few examples of how it works in real themes, and what options we have to apply these styles to WordPress blocks and elements.
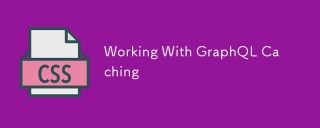
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
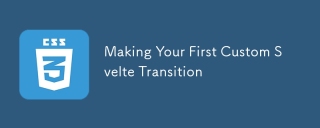
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
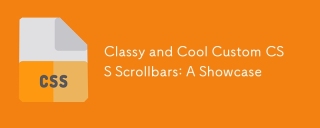
In this article we will be diving into the world of scrollbars. I know, it doesn’t sound too glamorous, but trust me, a well-designed page goes hand-in-hand
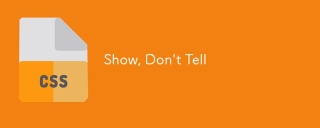
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
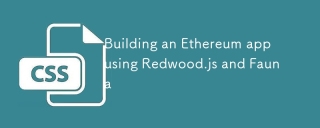
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
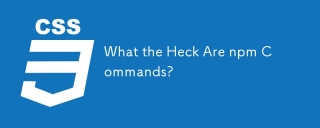
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
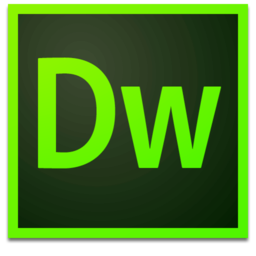
Dreamweaver Mac version
Visual web development tools
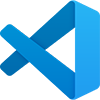
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
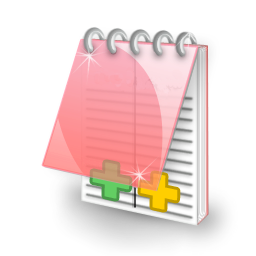
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function